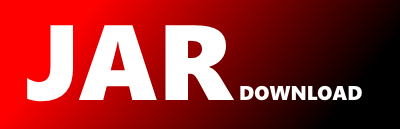
io.army.criteria.postgre.PostgreInsert Maven / Gradle / Ivy
package io.army.criteria.postgre;
import io.army.criteria.*;
import io.army.criteria.dialect.ReturningInsert;
import io.army.meta.*;
import java.util.function.BooleanSupplier;
import java.util.function.Supplier;
/**
*
* This interface representing postgre INSERT statement.
*
* @see Postgre INSERT syntax
* @since 1.0
*/
public interface PostgreInsert extends PostgreStatement {
interface _OverridingValueClause {
R overridingSystemValue();
R overridingUserValue();
R ifOverridingSystemValue(BooleanSupplier predicate);
R ifOverridingUserValue(BooleanSupplier predicate);
}
interface _StaticReturningCommaSpec
extends _StaticInsertReturningCommaClause<_StaticReturningCommaSpec>,
_DqlInsertClause {
}
interface _ReturningSpec
extends _StaticInsertReturningClause<_StaticReturningCommaSpec>,
_DynamicReturningClause<_DqlInsertClause>,
_DmlInsertClause {
}
interface _DoUpdateWhereAndSpec
extends UpdateStatement._UpdateWhereAndClause<_DoUpdateWhereAndSpec>,
_ReturningSpec {
}
interface _DoUpdateWhereClause
extends _WhereClause<_ReturningSpec, _DoUpdateWhereAndSpec>,
_ReturningSpec {
}
interface _DoUpdateSetClause
extends UpdateStatement._StaticRowSetClause, _DoUpdateWhereSpec>,
UpdateStatement._DynamicSetClause>, _DoUpdateWhereClause> {
}
interface _DoUpdateWhereSpec
extends _DoUpdateWhereClause,
_DoUpdateSetClause {
}
interface _ConflictDoNothingClause {
_ReturningSpec doNothing();
}
interface _ConflictActionClause extends _ConflictDoNothingClause {
_DoUpdateSetClause doUpdate();
}
interface _ConflictTargetWhereAndSpec
extends Statement._WhereAndClause<_ConflictTargetWhereAndSpec>,
_ConflictActionClause {
}
interface _ConflictTargetWhereSpec
extends _ConflictActionClause,
Statement._WhereClause<_ConflictActionClause, _ConflictTargetWhereAndSpec> {
}
interface _ConflictTargetCommaSpec
extends Statement._RightParenClause<_ConflictTargetWhereSpec> {
_ConflictCollateSpec comma(IndexFieldMeta indexColumn);
_ConflictCollateSpec comma(Expression indexExpression);
}
interface _ConflictOpClassSpec
extends _ConflictTargetCommaSpec {
/**
* @param operatorClass operator class
*/
_ConflictTargetCommaSpec space(String operatorClass);
/**
* @param supplier provide operator class
*/
_ConflictTargetCommaSpec ifSpace(Supplier supplier);
}
interface _ConflictCollateSpec
extends _ConflictOpClassSpec {
_ConflictOpClassSpec collation(String collationName);
_ConflictOpClassSpec collation(Supplier supplier);
_ConflictOpClassSpec ifCollation(Supplier supplier);
}
interface _ConflictTargetOptionSpec
extends _ConflictDoNothingClause {
_ConflictCollateSpec leftParen(IndexFieldMeta indexColumn);
_ConflictCollateSpec leftParen(Expression indexExpression);
_ConflictActionClause onConstraint(String constraintName);
}
interface _OnConflictSpec extends _ReturningSpec {
_ConflictTargetOptionSpec onConflict();
}
/*-------------------below insert syntax interfaces -------------------*/
interface _PostgreValuesStaticParensClause
extends InsertStatement._ValuesParensClause> {
}
interface _PostgreValuesStaticParensCommaSpec
extends _CommaClause<_PostgreValuesStaticParensClause>, _OnConflictSpec {
}
interface _ValuesDefaultSpec
extends InsertStatement._FullColumnDefaultClause>,
InsertStatement._DomainValueClause>,
InsertStatement._DynamicValuesClause>,
InsertStatement._StaticValuesClause<_PostgreValuesStaticParensClause> {
}
interface _ComplexColumnDefaultSpec
extends _ValuesDefaultSpec,
InsertStatement._QueryInsertSpaceClause>, _OnConflictSpec> {
}
interface _OverridingValueSpec
extends _ComplexColumnDefaultSpec, _OverridingValueClause<_ComplexColumnDefaultSpec> {
}
interface _ColumnListSpec
extends InsertStatement._ColumnListParensClause>,
_OverridingValueSpec {
}
interface _TableAliasSpec
extends Statement._AsClause<_ColumnListSpec>,
_ColumnListSpec {
}
interface _ChildInsertIntoClause extends Item {
_TableAliasSpec insertInto(ComplexTableMeta table);
}
interface _ChildWithCteSpec
extends _PostgreDynamicWithClause<_ChildInsertIntoClause
>,
PostgreQuery._PostgreStaticWithClause<_ChildInsertIntoClause
>,
_ChildInsertIntoClause
{
}
interface _ParentInsert
extends Insert, InsertStatement._ChildPartClause<_ChildWithCteSpec
> {
}
interface _ParentReturnInsert
extends ReturningInsert, InsertStatement._ChildPartClause<_ChildWithCteSpec
> {
}
interface _PrimaryInsertIntoClause extends Item {
_TableAliasSpec insertInto(TableMeta table);
_TableAliasSpec
, _ParentReturnInsert
> insertInto(ParentTableMeta
table);
}
interface _PrimaryWithCteSpec
extends _PostgreDynamicWithClause<_PrimaryInsertIntoClause>,
PostgreQuery._PostgreStaticWithClause<_PrimaryInsertIntoClause>,
_PrimaryInsertIntoClause {
}
interface _PrimaryPreferLiteralSpec extends InsertStatement._PreferLiteralClause<_PrimaryWithCteSpec>,
_PrimaryWithCteSpec {
}
interface _PrimaryNullOptionSpec extends InsertStatement._NullOptionClause<_PrimaryPreferLiteralSpec>,
_PrimaryPreferLiteralSpec {
}
interface _PrimaryOptionSpec extends InsertStatement._MigrationOptionClause<_PrimaryNullOptionSpec>,
InsertStatement._IgnoreReturnIdsOptionClause<_PrimaryNullOptionSpec>,
_PrimaryNullOptionSpec {
}
/*-------------------below complex insert syntax -------------------*/
/**
*
* This interface is used by in multi-statement api.
*
*/
interface _ComplexInsertIntoClause extends Item {
_TableAliasSpec insertInto(TableMeta table);
}
interface _ComplexWithCteSpec
extends _PostgreDynamicWithClause<_ComplexInsertIntoClause>,
PostgreQuery._PostgreStaticWithClause<_ComplexInsertIntoClause>,
_ComplexInsertIntoClause {
}
interface _ComplexPreferLiteralSpec
extends InsertStatement._PreferLiteralClause<_ComplexWithCteSpec>,
_ComplexWithCteSpec {
}
interface _ComplexNullOptionSpec
extends InsertStatement._NullOptionClause<_ComplexPreferLiteralSpec>,
_ComplexPreferLiteralSpec {
}
interface _ComplexOptionSpec
extends InsertStatement._MigrationOptionClause<_ComplexNullOptionSpec>,
InsertStatement._IgnoreReturnIdsOptionClause<_ComplexNullOptionSpec>,
_ComplexNullOptionSpec {
}
/*-------------------below sub insert syntax -------------------*/
interface _CteInsertIntoClause extends Item {
_TableAliasSpec insertInto(TableMeta table);
}
interface _DynamicSubWithSpec extends _PostgreDynamicWithClause<_CteInsertIntoClause>,
PostgreQuery._PostgreStaticWithClause<_CteInsertIntoClause>,
_CteInsertIntoClause {
}
interface _DynamicSubPreferLiteralSpec
extends InsertStatement._PreferLiteralClause<_DynamicSubWithSpec>,
_DynamicSubWithSpec {
}
interface _DynamicSubNullOptionSpec
extends InsertStatement._NullOptionClause<_DynamicSubPreferLiteralSpec>,
_DynamicSubPreferLiteralSpec {
}
interface _DynamicSubOptionSpec
extends InsertStatement._MigrationOptionClause<_DynamicSubNullOptionSpec>,
_DynamicSubNullOptionSpec {
}
interface _InsertDynamicCteAsClause extends _PostgreDynamicCteAsClause<_DynamicSubOptionSpec<_CommaClause>,
_CommaClause> {
}
interface _DynamicCteParensSpec extends _OptionalParensStringClause<_InsertDynamicCteAsClause>, _InsertDynamicCteAsClause {
}
/**
*
* static sub-statement syntax forbid the WITH clause of cte insert,because it destroy the Readability of code.
*
*/
interface _StaticSubPreferLiteralSpec
extends InsertStatement._PreferLiteralClause<_CteInsertIntoClause>,
_CteInsertIntoClause {
}
/**
*
* static sub-statement syntax forbid the WITH clause of cte insert,because it destroy the Readability of code.
*
*/
interface _StaticSubNullOptionSpec
extends InsertStatement._NullOptionClause<_StaticSubPreferLiteralSpec>,
_StaticSubPreferLiteralSpec {
}
/**
*
* static sub-statement syntax forbid the WITH clause of cte insert,because it destroy the Readability of code.
*
*/
interface _StaticSubOptionSpec
extends InsertStatement._MigrationOptionClause<_StaticSubNullOptionSpec>,
_StaticSubNullOptionSpec {
}
}