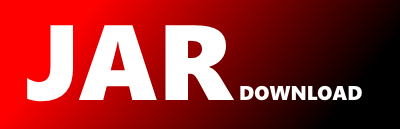
io.qt.bluetooth.QLowEnergyDescriptorData Maven / Gradle / Ivy
package io.qt.bluetooth;
import io.qt.*;
/**
* Used to create GATT service data
* Java wrapper for Qt class QLowEnergyDescriptorData
*/
public class QLowEnergyDescriptorData extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QLowEnergyDescriptorData:: QLowEnergyDescriptorData()
*/
public QLowEnergyDescriptorData(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QLowEnergyDescriptorData instance);
/**
* See QLowEnergyDescriptorData:: QLowEnergyDescriptorData(QBluetoothUuid, QByteArray)
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.@NonNull QBluetoothUuid uuid, io.qt.core.@NonNull QByteArray value){
super((QPrivateConstructor)null);
initialize_native(this, uuid, value);
}
private native static void initialize_native(QLowEnergyDescriptorData instance, io.qt.bluetooth.QBluetoothUuid uuid, io.qt.core.QByteArray value);
/**
* See QLowEnergyDescriptorData:: QLowEnergyDescriptorData(QLowEnergyDescriptorData)
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.@NonNull QLowEnergyDescriptorData other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QLowEnergyDescriptorData instance, io.qt.bluetooth.QLowEnergyDescriptorData other);
/**
* See QLowEnergyDescriptorData:: isReadable()const
*/
@QtUninvokable
public final boolean isReadable(){
return isReadable_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isReadable_native_constfct(long __this__nativeId);
/**
* See QLowEnergyDescriptorData:: isValid()const
*/
@QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QLowEnergyDescriptorData:: isWritable()const
*/
@QtUninvokable
public final boolean isWritable(){
return isWritable_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isWritable_native_constfct(long __this__nativeId);
/**
* See QLowEnergyDescriptorData:: operator=(QLowEnergyDescriptorData)
*/
@QtUninvokable
public final void assign(io.qt.bluetooth.@NonNull QLowEnergyDescriptorData other){
assign_native_cref_QLowEnergyDescriptorData(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void assign_native_cref_QLowEnergyDescriptorData(long __this__nativeId, long other);
/**
* See operator==(QLowEnergyDescriptorData, QLowEnergyDescriptorData)
*/
@QtUninvokable
public final boolean equals(io.qt.bluetooth.@NonNull QLowEnergyDescriptorData d2){
return equals_native_cref_QLowEnergyDescriptorData(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(d2));
}
@QtUninvokable
private native boolean equals_native_cref_QLowEnergyDescriptorData(long __this__nativeId, long d2);
/**
* See QLowEnergyDescriptorData:: readConstraints()const
*/
@QtUninvokable
public final io.qt.bluetooth.QBluetooth.@NonNull AttAccessConstraints readConstraints(){
return new io.qt.bluetooth.QBluetooth.AttAccessConstraints(readConstraints_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int readConstraints_native_constfct(long __this__nativeId);
/**
* See QLowEnergyDescriptorData:: setReadPermissions(bool, QBluetooth::AttAccessConstraints)
*/
@QtUninvokable
public final void setReadPermissions(boolean readable, io.qt.bluetooth.QBluetooth.@NonNull AttAccessConstraints constraints){
setReadPermissions_native_bool_QBluetooth_AttAccessConstraints(QtJambi_LibraryUtilities.internal.nativeId(this), readable, constraints.value());
}
@QtUninvokable
private native void setReadPermissions_native_bool_QBluetooth_AttAccessConstraints(long __this__nativeId, boolean readable, int constraints);
/**
* See QLowEnergyDescriptorData:: setUuid(QBluetoothUuid)
*/
@QtUninvokable
public final void setUuid(io.qt.bluetooth.@NonNull QBluetoothUuid uuid){
setUuid_native_cref_QBluetoothUuid(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(uuid));
}
@QtUninvokable
private native void setUuid_native_cref_QBluetoothUuid(long __this__nativeId, long uuid);
/**
* See QLowEnergyDescriptorData:: setValue(QByteArray)
*/
@QtUninvokable
public final void setValue(io.qt.core.@NonNull QByteArray value){
setValue_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(value));
}
@QtUninvokable
private native void setValue_native_cref_QByteArray(long __this__nativeId, long value);
/**
* See QLowEnergyDescriptorData:: setWritePermissions(bool, QBluetooth::AttAccessConstraints)
*/
@QtUninvokable
public final void setWritePermissions(boolean writable, io.qt.bluetooth.QBluetooth.@NonNull AttAccessConstraints constraints){
setWritePermissions_native_bool_QBluetooth_AttAccessConstraints(QtJambi_LibraryUtilities.internal.nativeId(this), writable, constraints.value());
}
@QtUninvokable
private native void setWritePermissions_native_bool_QBluetooth_AttAccessConstraints(long __this__nativeId, boolean writable, int constraints);
/**
* See QLowEnergyDescriptorData:: swap(QLowEnergyDescriptorData&)
*/
@QtUninvokable
public final void swap(io.qt.bluetooth.@StrictNonNull QLowEnergyDescriptorData other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QLowEnergyDescriptorData(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void swap_native_ref_QLowEnergyDescriptorData(long __this__nativeId, long other);
/**
* See QLowEnergyDescriptorData:: uuid()const
*/
@QtUninvokable
public final io.qt.bluetooth.@NonNull QBluetoothUuid uuid(){
return uuid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.bluetooth.QBluetoothUuid uuid_native_constfct(long __this__nativeId);
/**
* See QLowEnergyDescriptorData:: value()const
*/
@QtUninvokable
public final io.qt.core.@NonNull QByteArray value(){
return value_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QByteArray value_native_constfct(long __this__nativeId);
/**
* See QLowEnergyDescriptorData:: writeConstraints()const
*/
@QtUninvokable
public final io.qt.bluetooth.QBluetooth.@NonNull AttAccessConstraints writeConstraints(){
return new io.qt.bluetooth.QBluetooth.AttAccessConstraints(writeConstraints_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int writeConstraints_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QLowEnergyDescriptorData(QPrivateConstructor p) { super(p); }
/**
* See operator==(QLowEnergyDescriptorData, QLowEnergyDescriptorData)
*/
@Override
@QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.bluetooth.QLowEnergyDescriptorData) {
return equals((io.qt.bluetooth.QLowEnergyDescriptorData) other);
}
return false;
}
/**
* Returns the objects's hash code computed by qHash(QLowEnergyDescriptorData)
.
*/
@QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native static int hashCode_native(long __this_nativeId);
/**
* Creates and returns a copy of this object.
*/
@QtUninvokable
@Override
public QLowEnergyDescriptorData clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native QLowEnergyDescriptorData clone_native(long __this_nativeId);
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull ProtocolUuid uuid, io.qt.core.@NonNull QByteArray value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), value);
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull ServiceClassUuid uuid, io.qt.core.@NonNull QByteArray value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), value);
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull CharacteristicType uuid, io.qt.core.@NonNull QByteArray value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), value);
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull DescriptorType uuid, io.qt.core.@NonNull QByteArray value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), value);
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.core.@NonNull QUuid uuid, io.qt.core.@NonNull QByteArray value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), value);
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.@NonNull QBluetoothUuid uuid, byte @NonNull[] value) {
this(uuid, new io.qt.core.QByteArray(value));
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull ProtocolUuid uuid, byte @NonNull[] value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), new io.qt.core.QByteArray(value));
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull ServiceClassUuid uuid, byte @NonNull[] value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), new io.qt.core.QByteArray(value));
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull CharacteristicType uuid, byte @NonNull[] value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), new io.qt.core.QByteArray(value));
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid.@NonNull DescriptorType uuid, byte @NonNull[] value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), new io.qt.core.QByteArray(value));
}
/**
* Overloaded constructor for {@link #QLowEnergyDescriptorData(io.qt.bluetooth.QBluetoothUuid, io.qt.core.QByteArray)}.
*/
public QLowEnergyDescriptorData(io.qt.core.@NonNull QUuid uuid, byte @NonNull[] value) {
this(new io.qt.bluetooth.QBluetoothUuid(uuid), new io.qt.core.QByteArray(value));
}
/**
* Overloaded function for {@link #setReadPermissions(boolean, io.qt.bluetooth.QBluetooth.AttAccessConstraints)}
* with constraints = new io.qt.bluetooth.QBluetooth.AttAccessConstraints(0)
.
*/
@QtUninvokable
public final void setReadPermissions(boolean readable) {
setReadPermissions(readable, new io.qt.bluetooth.QBluetooth.AttAccessConstraints(0));
}
/**
* Overloaded function for {@link #setReadPermissions(boolean, io.qt.bluetooth.QBluetooth.AttAccessConstraints)}.
*/
@QtUninvokable
public final void setReadPermissions(boolean readable, io.qt.bluetooth.QBluetooth.@NonNull AttAccessConstraint @NonNull... constraints) {
setReadPermissions(readable, new io.qt.bluetooth.QBluetooth.AttAccessConstraints(constraints));
}
/**
* Overloaded function for {@link #setUuid(io.qt.bluetooth.QBluetoothUuid)}.
*/
@QtUninvokable
public final void setUuid(io.qt.bluetooth.QBluetoothUuid.@NonNull ProtocolUuid uuid) {
setUuid(new io.qt.bluetooth.QBluetoothUuid(uuid));
}
/**
* Overloaded function for {@link #setUuid(io.qt.bluetooth.QBluetoothUuid)}.
*/
@QtUninvokable
public final void setUuid(io.qt.bluetooth.QBluetoothUuid.@NonNull ServiceClassUuid uuid) {
setUuid(new io.qt.bluetooth.QBluetoothUuid(uuid));
}
/**
* Overloaded function for {@link #setUuid(io.qt.bluetooth.QBluetoothUuid)}.
*/
@QtUninvokable
public final void setUuid(io.qt.bluetooth.QBluetoothUuid.@NonNull CharacteristicType uuid) {
setUuid(new io.qt.bluetooth.QBluetoothUuid(uuid));
}
/**
* Overloaded function for {@link #setUuid(io.qt.bluetooth.QBluetoothUuid)}.
*/
@QtUninvokable
public final void setUuid(io.qt.bluetooth.QBluetoothUuid.@NonNull DescriptorType uuid) {
setUuid(new io.qt.bluetooth.QBluetoothUuid(uuid));
}
/**
* Overloaded function for {@link #setUuid(io.qt.bluetooth.QBluetoothUuid)}.
*/
@QtUninvokable
public final void setUuid(io.qt.core.@NonNull QUuid uuid) {
setUuid(new io.qt.bluetooth.QBluetoothUuid(uuid));
}
/**
* Overloaded function for {@link #setValue(io.qt.core.QByteArray)}.
*/
@QtUninvokable
public final void setValue(byte @NonNull[] value) {
setValue(new io.qt.core.QByteArray(value));
}
/**
* Overloaded function for {@link #setWritePermissions(boolean, io.qt.bluetooth.QBluetooth.AttAccessConstraints)}
* with constraints = new io.qt.bluetooth.QBluetooth.AttAccessConstraints(0)
.
*/
@QtUninvokable
public final void setWritePermissions(boolean writable) {
setWritePermissions(writable, new io.qt.bluetooth.QBluetooth.AttAccessConstraints(0));
}
/**
* Overloaded function for {@link #setWritePermissions(boolean, io.qt.bluetooth.QBluetooth.AttAccessConstraints)}.
*/
@QtUninvokable
public final void setWritePermissions(boolean writable, io.qt.bluetooth.QBluetooth.@NonNull AttAccessConstraint @NonNull... constraints) {
setWritePermissions(writable, new io.qt.bluetooth.QBluetooth.AttAccessConstraints(constraints));
}
}