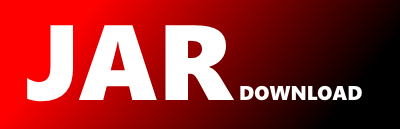
io.qt.gamepad.QGamepadManager Maven / Gradle / Ivy
Show all versions of qtjambi-gamepad Show documentation
package io.qt.gamepad;
import io.qt.*;
/**
* Queries attached gamepads and related events
* Java wrapper for Qt class QGamepadManager
*/
public final class QGamepadManager extends io.qt.core.QObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QGamepadManager.class);
/**
* Java wrapper for Qt enum QGamepadManager::GamepadAxis
*
* @see GamepadAxes
*/
public enum GamepadAxis implements QtFlagEnumerator {
/**
* Representing QGamepadManager:: AxisInvalid
*/
AxisInvalid(-1),
/**
* Representing QGamepadManager:: AxisLeftX
*/
AxisLeftX(0),
/**
* Representing QGamepadManager:: AxisLeftY
*/
AxisLeftY(1),
/**
* Representing QGamepadManager:: AxisRightX
*/
AxisRightX(2),
/**
* Representing QGamepadManager:: AxisRightY
*/
AxisRightY(3);
static {
QtJambi_LibraryUtilities.initialize();
}
private GamepadAxis(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
@Override
public @NonNull GamepadAxes asFlags() {
return new GamepadAxes(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public @NonNull GamepadAxes combined(@NonNull GamepadAxis e) {
return asFlags().setFlag(e, true);
}
/**
* Excludes other enum entry from a flag of this entry.
* @param e enum entry
* @return new flag
*/
public @NonNull GamepadAxes cleared(@NonNull GamepadAxis e) {
return asFlags().setFlag(e, false);
}
/**
* Creates a new {@link GamepadAxes} from the entries.
* @param values entries
* @return new flag
*/
public static @NonNull GamepadAxes flags(@Nullable GamepadAxis @NonNull... values) {
return new GamepadAxes(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull GamepadAxis resolve(int value) {
switch (value) {
case -1: return AxisInvalid;
case 0: return AxisLeftX;
case 1: return AxisLeftY;
case 2: return AxisRightX;
case 3: return AxisRightY;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* {@link QFlags} type for enum {@link GamepadAxis}
*/
public static final class GamepadAxes extends QFlags implements Comparable {
private static final long serialVersionUID = 0xda70e9601c72f2ddL;
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Creates a new GamepadAxes where the flags in args
are set.
* @param args enum entries
*/
public GamepadAxes(@Nullable GamepadAxis @NonNull... args){
super(args);
}
/**
* Creates a new GamepadAxes with given value
.
* @param value
*/
public GamepadAxes(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new GamepadAxes
*/
@Override
public final @NonNull GamepadAxes combined(@StrictNonNull GamepadAxis e){
return new GamepadAxes(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
@Override
public final @NonNull GamepadAxes setFlag(@Nullable GamepadAxis e){
return setFlag(e, true);
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
@Override
public final @NonNull GamepadAxes setFlag(@Nullable GamepadAxis e, boolean on){
if (on) {
setValue(value() | e.value());
}else {
setValue(value() & ~e.value());
}
return this;
}
/**
* Returns an array of flag objects represented by this GamepadAxes.
* @return array of enum entries
*/
@Override
public final @NonNull GamepadAxis @NonNull[] flags(){
return super.flags(GamepadAxis.values());
}
/**
* {@inheritDoc}
*/
@Override
public final @NonNull GamepadAxes clone(){
return new GamepadAxes(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(@StrictNonNull GamepadAxes other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum QGamepadManager::GamepadButton
*
* @see GamepadButtons
*/
public enum GamepadButton implements QtFlagEnumerator {
/**
* Representing QGamepadManager:: ButtonInvalid
*/
ButtonInvalid(-1),
/**
* Representing QGamepadManager:: ButtonA
*/
ButtonA(0),
/**
* Representing QGamepadManager:: ButtonB
*/
ButtonB(1),
/**
* Representing QGamepadManager:: ButtonX
*/
ButtonX(2),
/**
* Representing QGamepadManager:: ButtonY
*/
ButtonY(3),
/**
* Representing QGamepadManager:: ButtonL1
*/
ButtonL1(4),
/**
* Representing QGamepadManager:: ButtonR1
*/
ButtonR1(5),
/**
* Representing QGamepadManager:: ButtonL2
*/
ButtonL2(6),
/**
* Representing QGamepadManager:: ButtonR2
*/
ButtonR2(7),
/**
* Representing QGamepadManager:: ButtonSelect
*/
ButtonSelect(8),
/**
* Representing QGamepadManager:: ButtonStart
*/
ButtonStart(9),
/**
* Representing QGamepadManager:: ButtonL3
*/
ButtonL3(10),
/**
* Representing QGamepadManager:: ButtonR3
*/
ButtonR3(11),
/**
* Representing QGamepadManager:: ButtonUp
*/
ButtonUp(12),
/**
* Representing QGamepadManager:: ButtonDown
*/
ButtonDown(13),
/**
* Representing QGamepadManager:: ButtonRight
*/
ButtonRight(14),
/**
* Representing QGamepadManager:: ButtonLeft
*/
ButtonLeft(15),
/**
* Representing QGamepadManager:: ButtonCenter
*/
ButtonCenter(16),
/**
* Representing QGamepadManager:: ButtonGuide
*/
ButtonGuide(17);
static {
QtJambi_LibraryUtilities.initialize();
}
private GamepadButton(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
@Override
public @NonNull GamepadButtons asFlags() {
return new GamepadButtons(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public @NonNull GamepadButtons combined(@NonNull GamepadButton e) {
return asFlags().setFlag(e, true);
}
/**
* Excludes other enum entry from a flag of this entry.
* @param e enum entry
* @return new flag
*/
public @NonNull GamepadButtons cleared(@NonNull GamepadButton e) {
return asFlags().setFlag(e, false);
}
/**
* Creates a new {@link GamepadButtons} from the entries.
* @param values entries
* @return new flag
*/
public static @NonNull GamepadButtons flags(@Nullable GamepadButton @NonNull... values) {
return new GamepadButtons(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull GamepadButton resolve(int value) {
switch (value) {
case -1: return ButtonInvalid;
case 0: return ButtonA;
case 1: return ButtonB;
case 2: return ButtonX;
case 3: return ButtonY;
case 4: return ButtonL1;
case 5: return ButtonR1;
case 6: return ButtonL2;
case 7: return ButtonR2;
case 8: return ButtonSelect;
case 9: return ButtonStart;
case 10: return ButtonL3;
case 11: return ButtonR3;
case 12: return ButtonUp;
case 13: return ButtonDown;
case 14: return ButtonRight;
case 15: return ButtonLeft;
case 16: return ButtonCenter;
case 17: return ButtonGuide;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* {@link QFlags} type for enum {@link GamepadButton}
*/
public static final class GamepadButtons extends QFlags implements Comparable {
private static final long serialVersionUID = 0x5ce4d2185530f07L;
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Creates a new GamepadButtons where the flags in args
are set.
* @param args enum entries
*/
public GamepadButtons(@Nullable GamepadButton @NonNull... args){
super(args);
}
/**
* Creates a new GamepadButtons with given value
.
* @param value
*/
public GamepadButtons(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new GamepadButtons
*/
@Override
public final @NonNull GamepadButtons combined(@StrictNonNull GamepadButton e){
return new GamepadButtons(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
@Override
public final @NonNull GamepadButtons setFlag(@Nullable GamepadButton e){
return setFlag(e, true);
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
@Override
public final @NonNull GamepadButtons setFlag(@Nullable GamepadButton e, boolean on){
if (on) {
setValue(value() | e.value());
}else {
setValue(value() & ~e.value());
}
return this;
}
/**
* Returns an array of flag objects represented by this GamepadButtons.
* @return array of enum entries
*/
@Override
public final @NonNull GamepadButton @NonNull[] flags(){
return super.flags(GamepadButton.values());
}
/**
* {@inheritDoc}
*/
@Override
public final @NonNull GamepadButtons clone(){
return new GamepadButtons(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(@StrictNonNull GamepadButtons other){
return Integer.compare(value(), other.value());
}
}
/**
* See QGamepadManager:: axisConfigured(int, QGamepadManager::GamepadAxis)
*/
public final @NonNull Signal2 axisConfigured = new Signal2<>();
/**
* See QGamepadManager:: buttonConfigured(int, QGamepadManager::GamepadButton)
*/
public final @NonNull Signal2 buttonConfigured = new Signal2<>();
/**
* See QGamepadManager:: configurationCanceled(int)
*/
public final @NonNull Signal1 configurationCanceled = new Signal1<>();
/**
* See QGamepadManager:: connectedGamepadsChanged()
*/
@QtPropertyNotify(name="connectedGamepads")
public final @NonNull Signal0 connectedGamepadsChanged = new Signal0();
/**
* See QGamepadManager:: gamepadAxisEvent(int, QGamepadManager::GamepadAxis, double)
*/
public final @NonNull Signal3 gamepadAxisEvent = new Signal3<>();
/**
* See QGamepadManager:: gamepadButtonPressEvent(int, QGamepadManager::GamepadButton, double)
*/
public final @NonNull Signal3 gamepadButtonPressEvent = new Signal3<>();
/**
* See QGamepadManager:: gamepadButtonReleaseEvent(int, QGamepadManager::GamepadButton)
*/
public final @NonNull Signal2 gamepadButtonReleaseEvent = new Signal2<>();
/**
* See QGamepadManager:: gamepadConnected(int)
*/
public final @NonNull Signal1 gamepadConnected = new Signal1<>();
/**
* See QGamepadManager:: gamepadDisconnected(int)
*/
public final @NonNull Signal1 gamepadDisconnected = new Signal1<>();
/**
* See QGamepadManager:: gamepadNameChanged(int, QString)
*/
public final @NonNull Signal2 gamepadNameChanged = new Signal2<>();
/**
* See QGamepadManager:: configureAxis(int, QGamepadManager::GamepadAxis)
* @param deviceId
* @param axis
* @return
*/
public final boolean configureAxis(int deviceId, io.qt.gamepad.QGamepadManager.@NonNull GamepadAxis axis){
return configureAxis_native_int_QGamepadManager_GamepadAxis(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId, axis.value());
}
private native boolean configureAxis_native_int_QGamepadManager_GamepadAxis(long __this__nativeId, int deviceId, int axis);
/**
* See QGamepadManager:: configureButton(int, QGamepadManager::GamepadButton)
* @param deviceId
* @param button
* @return
*/
public final boolean configureButton(int deviceId, io.qt.gamepad.QGamepadManager.@NonNull GamepadButton button){
return configureButton_native_int_QGamepadManager_GamepadButton(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId, button.value());
}
private native boolean configureButton_native_int_QGamepadManager_GamepadButton(long __this__nativeId, int deviceId, int button);
/**
* See QGamepadManager:: connectedGamepads()const
* @return
*/
@QtPropertyReader(name="connectedGamepads")
@QtUninvokable
public final io.qt.core.@NonNull QList connectedGamepads(){
return connectedGamepads_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QList connectedGamepads_native_constfct(long __this__nativeId);
/**
* See QGamepadManager:: gamepadName(int)const
* @since This function was introduced in Qt 5.11.
* @param deviceId
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String gamepadName(int deviceId){
return gamepadName_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId);
}
@QtUninvokable
private native java.lang.String gamepadName_native_int_constfct(long __this__nativeId, int deviceId);
/**
* See QGamepadManager:: isConfigurationNeeded(int)const
* @param deviceId
* @return
*/
public final boolean isConfigurationNeeded(int deviceId){
return isConfigurationNeeded_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId);
}
private native boolean isConfigurationNeeded_native_int_constfct(long __this__nativeId, int deviceId);
/**
* See QGamepadManager:: isGamepadConnected(int)const
* @param deviceId
* @return
*/
@QtUninvokable
public final boolean isGamepadConnected(int deviceId){
return isGamepadConnected_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId);
}
@QtUninvokable
private native boolean isGamepadConnected_native_int_constfct(long __this__nativeId, int deviceId);
/**
* See QGamepadManager:: resetConfiguration(int)
* @param deviceId
*/
public final void resetConfiguration(int deviceId){
resetConfiguration_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId);
}
private native void resetConfiguration_native_int(long __this__nativeId, int deviceId);
/**
* See QGamepadManager:: setCancelConfigureButton(int, QGamepadManager::GamepadButton)
* @param deviceId
* @param button
* @return
*/
public final boolean setCancelConfigureButton(int deviceId, io.qt.gamepad.QGamepadManager.@NonNull GamepadButton button){
return setCancelConfigureButton_native_int_QGamepadManager_GamepadButton(QtJambi_LibraryUtilities.internal.nativeId(this), deviceId, button.value());
}
private native boolean setCancelConfigureButton_native_int_QGamepadManager_GamepadButton(long __this__nativeId, int deviceId, int button);
/**
* See QGamepadManager:: setSettingsFile(QString)
* @param file
*/
public final void setSettingsFile(java.lang.@NonNull String file){
setSettingsFile_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), file);
}
private native void setSettingsFile_native_cref_QString(long __this__nativeId, java.lang.String file);
/**
* See QGamepadManager:: instance()
* @return
*/
public native static io.qt.gamepad.@Nullable QGamepadManager instance();
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
private QGamepadManager(QPrivateConstructor p) { super(p); }
/**
* @hidden
* Kotlin property getter. In Java use {@link #connectedGamepads()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QList getConnectedGamepads() {
return connectedGamepads();
}
}