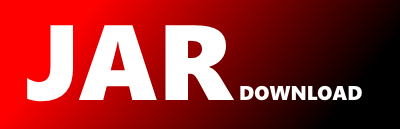
io.qt.help.QHelpSearchQuery Maven / Gradle / Ivy
Show all versions of qtjambi-help Show documentation
package io.qt.help;
import io.qt.*;
/**
* Contains the field name and the associated search term
* Java wrapper for Qt class QHelpSearchQuery
*/
public class QHelpSearchQuery extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QHelpSearchQuery::FieldName
*/
public enum FieldName implements QtEnumerator {
/**
* Representing QHelpSearchQuery:: DEFAULT
*/
DEFAULT(0),
/**
* Representing QHelpSearchQuery:: FUZZY
*/
FUZZY(1),
/**
* Representing QHelpSearchQuery:: WITHOUT
*/
WITHOUT(2),
/**
* Representing QHelpSearchQuery:: PHRASE
*/
PHRASE(3),
/**
* Representing QHelpSearchQuery:: ALL
*/
ALL(4),
/**
* Representing QHelpSearchQuery:: ATLEAST
*/
ATLEAST(5);
static {
QtJambi_LibraryUtilities.initialize();
}
private FieldName(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull FieldName resolve(int value) {
switch (value) {
case 0: return DEFAULT;
case 1: return FUZZY;
case 2: return WITHOUT;
case 3: return PHRASE;
case 4: return ALL;
case 5: return ATLEAST;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QHelpSearchQuery:: QHelpSearchQuery()
*/
public QHelpSearchQuery(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QHelpSearchQuery instance);
/**
* See QHelpSearchQuery:: QHelpSearchQuery(QHelpSearchQuery)
*/
public QHelpSearchQuery(io.qt.help.@NonNull QHelpSearchQuery other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QHelpSearchQuery instance, io.qt.help.QHelpSearchQuery other);
/**
* See QHelpSearchQuery:: QHelpSearchQuery(QHelpSearchQuery::FieldName, QStringList)
*/
public QHelpSearchQuery(io.qt.help.QHelpSearchQuery.@NonNull FieldName field, java.util.@NonNull Collection wordList_){
super((QPrivateConstructor)null);
initialize_native(this, field, wordList_);
}
private native static void initialize_native(QHelpSearchQuery instance, io.qt.help.QHelpSearchQuery.FieldName field, java.util.Collection wordList_);
/**
* See QHelpSearchQuery:: fieldName
*/
@QtUninvokable
public final void setFieldName(io.qt.help.QHelpSearchQuery.@NonNull FieldName fieldName){
setFieldName_native_cref_QHelpSearchQuery_FieldName(QtJambi_LibraryUtilities.internal.nativeId(this), fieldName.value());
}
@QtUninvokable
private native void setFieldName_native_cref_QHelpSearchQuery_FieldName(long __this__nativeId, int fieldName);
/**
* See QHelpSearchQuery:: fieldName
*/
@QtUninvokable
public final io.qt.help.QHelpSearchQuery.@NonNull FieldName fieldName(){
return io.qt.help.QHelpSearchQuery.FieldName.resolve(fieldName_native(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int fieldName_native(long __this__nativeId);
/**
* See QHelpSearchQuery:: wordList
*/
@QtUninvokable
public final void setWordList(java.util.@NonNull Collection wordList){
setWordList_native_cref_QStringList(QtJambi_LibraryUtilities.internal.nativeId(this), wordList);
}
@QtUninvokable
private native void setWordList_native_cref_QStringList(long __this__nativeId, java.util.Collection wordList);
/**
* See QHelpSearchQuery:: wordList
*/
@QtUninvokable
public final io.qt.core.@NonNull QList wordList(){
return wordList_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QList wordList_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QHelpSearchQuery(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See QHelpSearchQuery:: QHelpSearchQuery(QHelpSearchQuery)
*/
@QtUninvokable
@Override
public QHelpSearchQuery clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native QHelpSearchQuery clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #fieldName()} instead.
*/
@QtUninvokable
public final io.qt.help.QHelpSearchQuery.@NonNull FieldName getFieldName() {
return fieldName();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #wordList()} instead.
*/
@QtUninvokable
public final io.qt.core.@NonNull QList getWordList() {
return wordList();
}
}