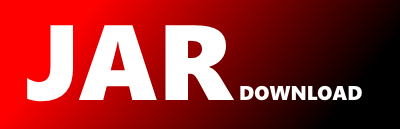
io.qt.core.QApplicationPermission Maven / Gradle / Ivy
package io.qt.core;
/**
* Java wrapper for Qt class QApplicationPermission
*/
public final class QApplicationPermission
{
static {
QtJambi_LibraryUtilities.initialize();
}
private QApplicationPermission() throws java.lang.InstantiationError { throw new java.lang.InstantiationError("Cannot instantiate namespace QApplicationPermission."); }
/**
* Java wrapper for Qt enum QApplicationPermission::PermissionResult
*/
public enum PermissionResult implements io.qt.QtEnumerator {
Undetermined(0),
Authorized(1),
Denied(2);
private PermissionResult(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PermissionResult resolve(int value) {
switch (value) {
case 0: return Undetermined;
case 1: return Authorized;
case 2: return Denied;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QApplicationPermission::PermissionType
*/
public enum PermissionType implements io.qt.QtEnumerator {
Camera(0),
Microphone(1),
Bluetooth(2),
Location(3),
PreciseLocation(4),
BackgroundLocation(5),
PreciseBackgroundLocation(6),
BodySensors(7),
PhysicalActivity(8),
Contacts(9),
Storage(10),
WriteStorage(11),
Calendar(12);
private PermissionType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PermissionType resolve(int value) {
switch (value) {
case 0: return Camera;
case 1: return Microphone;
case 2: return Bluetooth;
case 3: return Location;
case 4: return PreciseLocation;
case 5: return BackgroundLocation;
case 6: return PreciseBackgroundLocation;
case 7: return BodySensors;
case 8: return PhysicalActivity;
case 9: return Contacts;
case 10: return Storage;
case 11: return WriteStorage;
case 12: return Calendar;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy