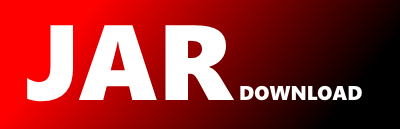
io.qt.core.QItemSelectionModel Maven / Gradle / Ivy
package io.qt.core;
/**
* Keeps track of a view's selected items
* Java wrapper for Qt class QItemSelectionModel
*/
public class QItemSelectionModel extends io.qt.core.QObject
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QItemSelectionModel.class);
/**
* Java wrapper for Qt enum QItemSelectionModel::SelectionFlag
*
* @see SelectionFlags
*/
public enum SelectionFlag implements io.qt.QtFlagEnumerator {
NoUpdate(0),
Clear(1),
Select(2),
Deselect(4),
Toggle(8),
Current(16),
Rows(32),
Columns(64),
SelectCurrent(18),
ToggleCurrent(24),
ClearAndSelect(3);
private SelectionFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public SelectionFlags asFlags() {
return new SelectionFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public SelectionFlags combined(SelectionFlag e) {
return new SelectionFlags(this, e);
}
/**
* Creates a new {@link SelectionFlags} from the entries.
* @param values entries
* @return new flag
*/
public static SelectionFlags flags(SelectionFlag ... values) {
return new SelectionFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SelectionFlag resolve(int value) {
switch (value) {
case 0: return NoUpdate;
case 1: return Clear;
case 2: return Select;
case 4: return Deselect;
case 8: return Toggle;
case 16: return Current;
case 32: return Rows;
case 64: return Columns;
case 18: return SelectCurrent;
case 24: return ToggleCurrent;
case 3: return ClearAndSelect;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link SelectionFlag}
*/
public static final class SelectionFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x94dda626326e2012L;
/**
* Creates a new SelectionFlags where the flags in args
are set.
* @param args enum entries
*/
public SelectionFlags(SelectionFlag ... args){
super(args);
}
/**
* Creates a new SelectionFlags with given value
.
* @param value
*/
public SelectionFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new SelectionFlags
*/
@Override
public final SelectionFlags combined(SelectionFlag e){
return new SelectionFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final SelectionFlags setFlag(SelectionFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final SelectionFlags setFlag(SelectionFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this SelectionFlags.
* @return array of enum entries
*/
@Override
public final SelectionFlag[] flags(){
return super.flags(SelectionFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final SelectionFlags clone(){
return new SelectionFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(SelectionFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* See QItemSelectionModel::currentChanged(QModelIndex,QModelIndex)
*/
@io.qt.QtPropertyNotify(name="currentIndex")
public final Signal2 currentChanged = new Signal2<>();
/**
* See QItemSelectionModel::currentColumnChanged(QModelIndex,QModelIndex)
*/
public final Signal2 currentColumnChanged = new Signal2<>();
/**
* See QItemSelectionModel::currentRowChanged(QModelIndex,QModelIndex)
*/
public final Signal2 currentRowChanged = new Signal2<>();
/**
* See QItemSelectionModel::modelChanged(QAbstractItemModel*)
*/
@io.qt.QtPropertyNotify(name="model")
public final Signal1 modelChanged = new Signal1<>();
/**
* See QItemSelectionModel::selectionChanged(QItemSelection,QItemSelection)
*/
@io.qt.QtPropertyNotify(name="hasSelection")
public final Signal2 selectionChanged = new Signal2<>();
/**
* Overloaded constructor for {@link #QItemSelectionModel(io.qt.core.QAbstractItemModel)}
* with model = null
.
*/
public QItemSelectionModel() {
this((io.qt.core.QAbstractItemModel)null);
}
/**
* See QItemSelectionModel::QItemSelectionModel(QAbstractItemModel*)
*/
public QItemSelectionModel(io.qt.core.QAbstractItemModel model){
super((QPrivateConstructor)null);
initialize_native(this, model);
}
private native static void initialize_native(QItemSelectionModel instance, io.qt.core.QAbstractItemModel model);
/**
* See QItemSelectionModel::QItemSelectionModel(QAbstractItemModel*,QObject*)
*/
public QItemSelectionModel(io.qt.core.QAbstractItemModel model, io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, model, parent);
}
private native static void initialize_native(QItemSelectionModel instance, io.qt.core.QAbstractItemModel model, io.qt.core.QObject parent);
@io.qt.QtPropertyBindable(name="model")
@io.qt.QtUninvokable
public final io.qt.core.QBindable bindableModel(){
return bindableModel_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QBindable bindableModel_native(long __this__nativeId);
/**
* See QItemSelectionModel::clearSelection()
*/
public final void clearSelection(){
clearSelection_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void clearSelection_native(long __this__nativeId);
/**
* Overloaded function for {@link #columnIntersectsSelection(int, io.qt.core.QModelIndex)}
* with parent = null
.
*/
public final boolean columnIntersectsSelection(int column) {
return columnIntersectsSelection(column, (io.qt.core.QModelIndex)null);
}
/**
* See QItemSelectionModel::columnIntersectsSelection(int,QModelIndex)const
*/
public final boolean columnIntersectsSelection(int column, io.qt.core.QModelIndex parent){
return columnIntersectsSelection_native_int_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), column, parent);
}
private native boolean columnIntersectsSelection_native_int_cref_QModelIndex_constfct(long __this__nativeId, int column, io.qt.core.QModelIndex parent);
/**
* See QItemSelectionModel::currentIndex()const
*/
@io.qt.QtPropertyReader(name="currentIndex")
@io.qt.QtPropertyDesignable("false")
@io.qt.QtPropertyStored("false")
@io.qt.QtUninvokable
public final io.qt.core.QModelIndex currentIndex(){
return currentIndex_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QModelIndex currentIndex_native_constfct(long __this__nativeId);
/**
* See QItemSelectionModel::emitSelectionChanged(QItemSelection,QItemSelection)
*/
@io.qt.QtUninvokable
protected final void emitSelectionChanged(io.qt.core.QItemSelection newSelection, io.qt.core.QItemSelection oldSelection){
emitSelectionChanged_native_cref_QItemSelection_cref_QItemSelection(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(newSelection), QtJambi_LibraryUtilities.internal.checkedNativeId(oldSelection));
}
@io.qt.QtUninvokable
private native void emitSelectionChanged_native_cref_QItemSelection_cref_QItemSelection(long __this__nativeId, long newSelection, long oldSelection);
/**
* See QItemSelectionModel::hasSelection()const
*/
@io.qt.QtPropertyReader(name="hasSelection")
@io.qt.QtPropertyDesignable("false")
@io.qt.QtPropertyStored("false")
@io.qt.QtUninvokable
public final boolean hasSelection(){
return hasSelection_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean hasSelection_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #isColumnSelected(int, io.qt.core.QModelIndex)}
* with parent = null
.
*/
public final boolean isColumnSelected(int column) {
return isColumnSelected(column, (io.qt.core.QModelIndex)null);
}
/**
* See QItemSelectionModel::isColumnSelected(int,QModelIndex)const
*/
public final boolean isColumnSelected(int column, io.qt.core.QModelIndex parent){
return isColumnSelected_native_int_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), column, parent);
}
private native boolean isColumnSelected_native_int_cref_QModelIndex_constfct(long __this__nativeId, int column, io.qt.core.QModelIndex parent);
/**
* Overloaded function for {@link #isRowSelected(int, io.qt.core.QModelIndex)}
* with parent = null
.
*/
public final boolean isRowSelected(int row) {
return isRowSelected(row, (io.qt.core.QModelIndex)null);
}
/**
* See QItemSelectionModel::isRowSelected(int,QModelIndex)const
*/
public final boolean isRowSelected(int row, io.qt.core.QModelIndex parent){
return isRowSelected_native_int_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), row, parent);
}
private native boolean isRowSelected_native_int_cref_QModelIndex_constfct(long __this__nativeId, int row, io.qt.core.QModelIndex parent);
/**
* See QItemSelectionModel::isSelected(QModelIndex)const
*/
public final boolean isSelected(io.qt.core.QModelIndex index){
return isSelected_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), index);
}
private native boolean isSelected_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex index);
/**
* See QItemSelectionModel::model()
*/
@io.qt.QtPropertyReader(name="model")
@io.qt.QtUninvokable
public final io.qt.core.QAbstractItemModel model(){
return model_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QAbstractItemModel model_native(long __this__nativeId);
/**
* Overloaded function for {@link #rowIntersectsSelection(int, io.qt.core.QModelIndex)}
* with parent = null
.
*/
public final boolean rowIntersectsSelection(int row) {
return rowIntersectsSelection(row, (io.qt.core.QModelIndex)null);
}
/**
* See QItemSelectionModel::rowIntersectsSelection(int,QModelIndex)const
*/
public final boolean rowIntersectsSelection(int row, io.qt.core.QModelIndex parent){
return rowIntersectsSelection_native_int_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), row, parent);
}
private native boolean rowIntersectsSelection_native_int_cref_QModelIndex_constfct(long __this__nativeId, int row, io.qt.core.QModelIndex parent);
/**
* Overloaded function for {@link #selectedColumns(int)}
* with row = 0
.
*/
public final io.qt.core.QList selectedColumns() {
return selectedColumns((int)0);
}
/**
* See QItemSelectionModel::selectedColumns(int)const
*/
public final io.qt.core.QList selectedColumns(int row){
return selectedColumns_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), row);
}
private native io.qt.core.QList selectedColumns_native_int_constfct(long __this__nativeId, int row);
/**
* See QItemSelectionModel::selectedIndexes()const
*/
@io.qt.QtPropertyReader(name="selectedIndexes")
@io.qt.QtPropertyDesignable("false")
@io.qt.QtPropertyStored("false")
@io.qt.QtUninvokable
public final io.qt.core.QList selectedIndexes(){
return selectedIndexes_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList selectedIndexes_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #selectedRows(int)}
* with column = 0
.
*/
public final io.qt.core.QList selectedRows() {
return selectedRows((int)0);
}
/**
* See QItemSelectionModel::selectedRows(int)const
*/
public final io.qt.core.QList selectedRows(int column){
return selectedRows_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), column);
}
private native io.qt.core.QList selectedRows_native_int_constfct(long __this__nativeId, int column);
/**
* See QItemSelectionModel::selection()const
*/
@io.qt.QtPropertyReader(name="selection")
@io.qt.QtPropertyDesignable("false")
@io.qt.QtPropertyStored("false")
@io.qt.QtUninvokable
public final io.qt.core.QItemSelection selection(){
return selection_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QItemSelection selection_native_constfct(long __this__nativeId);
/**
* See QItemSelectionModel::setModel(QAbstractItemModel*)
*/
@io.qt.QtPropertyWriter(name="model")
@io.qt.QtUninvokable
public final void setModel(io.qt.core.QAbstractItemModel model){
setModel_native_QAbstractItemModel_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(model));
}
@io.qt.QtUninvokable
private native void setModel_native_QAbstractItemModel_ptr(long __this__nativeId, long model);
/**
* See QItemSelectionModel::clear()
*/
public void clear(){
clear_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void clear_native(long __this__nativeId);
/**
* See QItemSelectionModel::clearCurrentIndex()
*/
public void clearCurrentIndex(){
clearCurrentIndex_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void clearCurrentIndex_native(long __this__nativeId);
/**
* See QItemSelectionModel::reset()
*/
public void reset(){
reset_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void reset_native(long __this__nativeId);
/**
* Overloaded function for {@link #select(io.qt.core.QItemSelection, io.qt.core.QItemSelectionModel.SelectionFlags)}.
*/
public final void select(io.qt.core.QItemSelection selection, io.qt.core.QItemSelectionModel.SelectionFlag ... command){
select(selection, new io.qt.core.QItemSelectionModel.SelectionFlags(command));
}
/**
* See QItemSelectionModel::select(QItemSelection,QItemSelectionModel::SelectionFlags)
*/
public void select(io.qt.core.QItemSelection selection, io.qt.core.QItemSelectionModel.SelectionFlags command){
select_native_cref_QItemSelection_QFlags_QItemSelectionModel_SelectionFlag_(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(selection), command.value());
}
private native void select_native_cref_QItemSelection_QFlags_QItemSelectionModel_SelectionFlag_(long __this__nativeId, long selection, int command);
/**
* Overloaded function for {@link #select(io.qt.core.QModelIndex, io.qt.core.QItemSelectionModel.SelectionFlags)}.
*/
public final void select(io.qt.core.QModelIndex index, io.qt.core.QItemSelectionModel.SelectionFlag ... command){
select(index, new io.qt.core.QItemSelectionModel.SelectionFlags(command));
}
/**
* See QItemSelectionModel::select(QModelIndex,QItemSelectionModel::SelectionFlags)
*/
public void select(io.qt.core.QModelIndex index, io.qt.core.QItemSelectionModel.SelectionFlags command){
select_native_cref_QModelIndex_QFlags_QItemSelectionModel_SelectionFlag_(QtJambi_LibraryUtilities.internal.nativeId(this), index, command.value());
}
private native void select_native_cref_QModelIndex_QFlags_QItemSelectionModel_SelectionFlag_(long __this__nativeId, io.qt.core.QModelIndex index, int command);
/**
* Overloaded function for {@link #setCurrentIndex(io.qt.core.QModelIndex, io.qt.core.QItemSelectionModel.SelectionFlags)}.
*/
public final void setCurrentIndex(io.qt.core.QModelIndex index, io.qt.core.QItemSelectionModel.SelectionFlag ... command){
setCurrentIndex(index, new io.qt.core.QItemSelectionModel.SelectionFlags(command));
}
/**
* See QItemSelectionModel::setCurrentIndex(QModelIndex,QItemSelectionModel::SelectionFlags)
*/
public void setCurrentIndex(io.qt.core.QModelIndex index, io.qt.core.QItemSelectionModel.SelectionFlags command){
setCurrentIndex_native_cref_QModelIndex_QFlags_QItemSelectionModel_SelectionFlag_(QtJambi_LibraryUtilities.internal.nativeId(this), index, command.value());
}
private native void setCurrentIndex_native_cref_QModelIndex_QFlags_QItemSelectionModel_SelectionFlag_(long __this__nativeId, io.qt.core.QModelIndex index, int command);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QItemSelectionModel(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QItemSelectionModel(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QItemSelectionModel instance, QDeclarativeConstructor constructor);
}