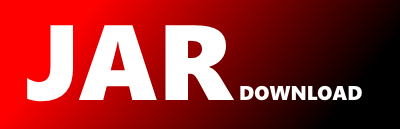
io.qt.core.Qt Maven / Gradle / Ivy
package io.qt.core;
/**
* Contains miscellaneous identifiers used throughout the Qt library
* Java wrapper for Qt class Qt
*/
public final class Qt
{
static {
QtJambi_LibraryUtilities.initialize();
}
private Qt() throws java.lang.InstantiationError { throw new java.lang.InstantiationError("Cannot instantiate namespace Qt."); }
/**
* Java wrapper for Qt enum Qt::AlignmentFlag
*
* @see Alignment
*/
@io.qt.QtRejectedEntries({"AlignLeading", "AlignTrailing"})
public enum AlignmentFlag implements io.qt.QtFlagEnumerator {
AlignLeft(1),
AlignLeading(1),
AlignRight(2),
AlignTrailing(2),
AlignHCenter(4),
AlignJustify(8),
AlignAbsolute(16),
AlignHorizontal_Mask(31),
AlignTop(32),
AlignBottom(64),
AlignVCenter(128),
AlignBaseline(256),
AlignVertical_Mask(480),
AlignCenter(132);
private AlignmentFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public Alignment asFlags() {
return new Alignment(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public Alignment combined(AlignmentFlag e) {
return new Alignment(this, e);
}
/**
* Creates a new {@link Alignment} from the entries.
* @param values entries
* @return new flag
*/
public static Alignment flags(AlignmentFlag ... values) {
return new Alignment(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AlignmentFlag resolve(int value) {
switch (value) {
case 1: return AlignLeft;
case 2: return AlignRight;
case 4: return AlignHCenter;
case 8: return AlignJustify;
case 16: return AlignAbsolute;
case 31: return AlignHorizontal_Mask;
case 32: return AlignTop;
case 64: return AlignBottom;
case 128: return AlignVCenter;
case 256: return AlignBaseline;
case 480: return AlignVertical_Mask;
case 132: return AlignCenter;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link AlignmentFlag}
*/
public static final class Alignment extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xb582c1bff5b386cdL;
/**
* Creates a new Alignment where the flags in args
are set.
* @param args enum entries
*/
public Alignment(AlignmentFlag ... args){
super(args);
}
/**
* Creates a new Alignment with given value
.
* @param value
*/
public Alignment(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new Alignment
*/
@Override
public final Alignment combined(AlignmentFlag e){
return new Alignment(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final Alignment setFlag(AlignmentFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final Alignment setFlag(AlignmentFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this Alignment.
* @return array of enum entries
*/
@Override
public final AlignmentFlag[] flags(){
return super.flags(AlignmentFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final Alignment clone(){
return new Alignment(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(Alignment other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::AnchorPoint
*/
@io.qt.QtUnlistedEnum
public enum AnchorPoint implements io.qt.QtEnumerator {
AnchorLeft(0),
AnchorHorizontalCenter(1),
AnchorRight(2),
AnchorTop(3),
AnchorVerticalCenter(4),
AnchorBottom(5);
private AnchorPoint(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AnchorPoint resolve(int value) {
switch (value) {
case 0: return AnchorLeft;
case 1: return AnchorHorizontalCenter;
case 2: return AnchorRight;
case 3: return AnchorTop;
case 4: return AnchorVerticalCenter;
case 5: return AnchorBottom;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ApplicationAttribute
*/
public enum ApplicationAttribute implements io.qt.QtEnumerator {
AA_DontShowIconsInMenus(2),
AA_NativeWindows(3),
AA_DontCreateNativeWidgetSiblings(4),
AA_PluginApplication(5),
AA_DontUseNativeMenuBar(6),
AA_MacDontSwapCtrlAndMeta(7),
AA_Use96Dpi(8),
AA_DisableNativeVirtualKeyboard(9),
AA_SynthesizeTouchForUnhandledMouseEvents(11),
AA_SynthesizeMouseForUnhandledTouchEvents(12),
/**
* @deprecated "High-DPI pixmaps are always enabled. " \
This attribute no longer has any effect.
*/
@Deprecated
AA_UseHighDpiPixmaps(13),
AA_ForceRasterWidgets(14),
AA_UseDesktopOpenGL(15),
AA_UseOpenGLES(16),
AA_UseSoftwareOpenGL(17),
AA_ShareOpenGLContexts(18),
AA_SetPalette(19),
/**
* @deprecated "High-DPI scaling is always enabled. " \
This attribute no longer has any effect.
*/
@Deprecated
AA_EnableHighDpiScaling(20),
/**
* @deprecated "High-DPI scaling is always enabled. " \
This attribute no longer has any effect.
*/
@Deprecated
AA_DisableHighDpiScaling(21),
AA_UseStyleSheetPropagationInWidgetStyles(22),
AA_DontUseNativeDialogs(23),
AA_SynthesizeMouseForUnhandledTabletEvents(24),
AA_CompressHighFrequencyEvents(25),
AA_DontCheckOpenGLContextThreadAffinity(26),
AA_DisableShaderDiskCache(27),
AA_DontShowShortcutsInContextMenus(28),
AA_CompressTabletEvents(29),
AA_DisableSessionManager(31),
AA_AttributeCount(32);
private ApplicationAttribute(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ApplicationAttribute resolve(int value) {
switch (value) {
case 2: return AA_DontShowIconsInMenus;
case 3: return AA_NativeWindows;
case 4: return AA_DontCreateNativeWidgetSiblings;
case 5: return AA_PluginApplication;
case 6: return AA_DontUseNativeMenuBar;
case 7: return AA_MacDontSwapCtrlAndMeta;
case 8: return AA_Use96Dpi;
case 9: return AA_DisableNativeVirtualKeyboard;
case 11: return AA_SynthesizeTouchForUnhandledMouseEvents;
case 12: return AA_SynthesizeMouseForUnhandledTouchEvents;
case 13: return AA_UseHighDpiPixmaps;
case 14: return AA_ForceRasterWidgets;
case 15: return AA_UseDesktopOpenGL;
case 16: return AA_UseOpenGLES;
case 17: return AA_UseSoftwareOpenGL;
case 18: return AA_ShareOpenGLContexts;
case 19: return AA_SetPalette;
case 20: return AA_EnableHighDpiScaling;
case 21: return AA_DisableHighDpiScaling;
case 22: return AA_UseStyleSheetPropagationInWidgetStyles;
case 23: return AA_DontUseNativeDialogs;
case 24: return AA_SynthesizeMouseForUnhandledTabletEvents;
case 25: return AA_CompressHighFrequencyEvents;
case 26: return AA_DontCheckOpenGLContextThreadAffinity;
case 27: return AA_DisableShaderDiskCache;
case 28: return AA_DontShowShortcutsInContextMenus;
case 29: return AA_CompressTabletEvents;
case 31: return AA_DisableSessionManager;
case 32: return AA_AttributeCount;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ApplicationState
*
* @see ApplicationStates
*/
public enum ApplicationState implements io.qt.QtFlagEnumerator {
ApplicationSuspended(0),
ApplicationHidden(1),
ApplicationInactive(2),
ApplicationActive(4);
private ApplicationState(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public ApplicationStates asFlags() {
return new ApplicationStates(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public ApplicationStates combined(ApplicationState e) {
return new ApplicationStates(this, e);
}
/**
* Creates a new {@link ApplicationStates} from the entries.
* @param values entries
* @return new flag
*/
public static ApplicationStates flags(ApplicationState ... values) {
return new ApplicationStates(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ApplicationState resolve(int value) {
switch (value) {
case 0: return ApplicationSuspended;
case 1: return ApplicationHidden;
case 2: return ApplicationInactive;
case 4: return ApplicationActive;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link ApplicationState}
*/
public static final class ApplicationStates extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xe687331abc8d9d6eL;
/**
* Creates a new ApplicationStates where the flags in args
are set.
* @param args enum entries
*/
public ApplicationStates(ApplicationState ... args){
super(args);
}
/**
* Creates a new ApplicationStates with given value
.
* @param value
*/
public ApplicationStates(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new ApplicationStates
*/
@Override
public final ApplicationStates combined(ApplicationState e){
return new ApplicationStates(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final ApplicationStates setFlag(ApplicationState e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final ApplicationStates setFlag(ApplicationState e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this ApplicationStates.
* @return array of enum entries
*/
@Override
public final ApplicationState[] flags(){
return super.flags(ApplicationState.values());
}
/**
* {@inheritDoc}
*/
@Override
public final ApplicationStates clone(){
return new ApplicationStates(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(ApplicationStates other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::ArrowType
*/
public enum ArrowType implements io.qt.QtEnumerator {
NoArrow(0),
UpArrow(1),
DownArrow(2),
LeftArrow(3),
RightArrow(4);
private ArrowType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ArrowType resolve(int value) {
switch (value) {
case 0: return NoArrow;
case 1: return UpArrow;
case 2: return DownArrow;
case 3: return LeftArrow;
case 4: return RightArrow;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::AspectRatioMode
*/
public enum AspectRatioMode implements io.qt.QtEnumerator {
IgnoreAspectRatio(0),
KeepAspectRatio(1),
KeepAspectRatioByExpanding(2);
private AspectRatioMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AspectRatioMode resolve(int value) {
switch (value) {
case 0: return IgnoreAspectRatio;
case 1: return KeepAspectRatio;
case 2: return KeepAspectRatioByExpanding;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::Axis
*/
public enum Axis implements io.qt.QtEnumerator {
XAxis(0),
YAxis(1),
ZAxis(2);
private Axis(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Axis resolve(int value) {
switch (value) {
case 0: return XAxis;
case 1: return YAxis;
case 2: return ZAxis;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::BGMode
*/
public enum BGMode implements io.qt.QtEnumerator {
TransparentMode(0),
OpaqueMode(1);
private BGMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static BGMode resolve(int value) {
switch (value) {
case 0: return TransparentMode;
case 1: return OpaqueMode;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::BrushStyle
*/
public enum BrushStyle implements io.qt.QtEnumerator {
NoBrush(0),
SolidPattern(1),
Dense1Pattern(2),
Dense2Pattern(3),
Dense3Pattern(4),
Dense4Pattern(5),
Dense5Pattern(6),
Dense6Pattern(7),
Dense7Pattern(8),
HorPattern(9),
VerPattern(10),
CrossPattern(11),
BDiagPattern(12),
FDiagPattern(13),
DiagCrossPattern(14),
LinearGradientPattern(15),
RadialGradientPattern(16),
ConicalGradientPattern(17),
TexturePattern(24);
private BrushStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static BrushStyle resolve(int value) {
switch (value) {
case 0: return NoBrush;
case 1: return SolidPattern;
case 2: return Dense1Pattern;
case 3: return Dense2Pattern;
case 4: return Dense3Pattern;
case 5: return Dense4Pattern;
case 6: return Dense5Pattern;
case 7: return Dense6Pattern;
case 8: return Dense7Pattern;
case 9: return HorPattern;
case 10: return VerPattern;
case 11: return CrossPattern;
case 12: return BDiagPattern;
case 13: return FDiagPattern;
case 14: return DiagCrossPattern;
case 15: return LinearGradientPattern;
case 16: return RadialGradientPattern;
case 17: return ConicalGradientPattern;
case 24: return TexturePattern;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::CaseSensitivity
*/
public enum CaseSensitivity implements io.qt.QtEnumerator {
CaseInsensitive(0),
CaseSensitive(1);
private CaseSensitivity(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static CaseSensitivity resolve(int value) {
switch (value) {
case 0: return CaseInsensitive;
case 1: return CaseSensitive;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::CheckState
*/
public enum CheckState implements io.qt.QtEnumerator {
Unchecked(0),
PartiallyChecked(1),
Checked(2);
private CheckState(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static CheckState resolve(int value) {
switch (value) {
case 0: return Unchecked;
case 1: return PartiallyChecked;
case 2: return Checked;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ChecksumType
*/
public enum ChecksumType implements io.qt.QtEnumerator {
ChecksumIso3309(0),
ChecksumItuV41(1);
private ChecksumType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ChecksumType resolve(int value) {
switch (value) {
case 0: return ChecksumIso3309;
case 1: return ChecksumItuV41;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ClipOperation
*/
public enum ClipOperation implements io.qt.QtEnumerator {
NoClip(0),
ReplaceClip(1),
IntersectClip(2);
private ClipOperation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ClipOperation resolve(int value) {
switch (value) {
case 0: return NoClip;
case 1: return ReplaceClip;
case 2: return IntersectClip;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ConnectionType
*/
public enum ConnectionType implements io.qt.QtEnumerator {
AutoConnection(0),
DirectConnection(1),
QueuedConnection(2),
BlockingQueuedConnection(3),
UniqueConnection(128),
SingleShotConnection(256);
private ConnectionType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ConnectionType resolve(int value) {
switch (value) {
case 0: return AutoConnection;
case 1: return DirectConnection;
case 2: return QueuedConnection;
case 3: return BlockingQueuedConnection;
case 128: return UniqueConnection;
case 256: return SingleShotConnection;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ContextMenuPolicy
*/
public enum ContextMenuPolicy implements io.qt.QtEnumerator {
NoContextMenu(0),
DefaultContextMenu(1),
ActionsContextMenu(2),
CustomContextMenu(3),
PreventContextMenu(4);
private ContextMenuPolicy(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ContextMenuPolicy resolve(int value) {
switch (value) {
case 0: return NoContextMenu;
case 1: return DefaultContextMenu;
case 2: return ActionsContextMenu;
case 3: return CustomContextMenu;
case 4: return PreventContextMenu;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::CoordinateSystem
*/
@io.qt.QtUnlistedEnum
public enum CoordinateSystem implements io.qt.QtEnumerator {
DeviceCoordinates(0),
LogicalCoordinates(1);
private CoordinateSystem(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static CoordinateSystem resolve(int value) {
switch (value) {
case 0: return DeviceCoordinates;
case 1: return LogicalCoordinates;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::Corner
*/
public enum Corner implements io.qt.QtEnumerator {
TopLeftCorner(0),
TopRightCorner(1),
BottomLeftCorner(2),
BottomRightCorner(3);
private Corner(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Corner resolve(int value) {
switch (value) {
case 0: return TopLeftCorner;
case 1: return TopRightCorner;
case 2: return BottomLeftCorner;
case 3: return BottomRightCorner;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::CursorMoveStyle
*/
public enum CursorMoveStyle implements io.qt.QtEnumerator {
LogicalMoveStyle(0),
VisualMoveStyle(1);
private CursorMoveStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static CursorMoveStyle resolve(int value) {
switch (value) {
case 0: return LogicalMoveStyle;
case 1: return VisualMoveStyle;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::CursorShape
*/
@io.qt.QtRejectedEntries({"LastCursor"})
public enum CursorShape implements io.qt.QtEnumerator {
ArrowCursor(0),
UpArrowCursor(1),
CrossCursor(2),
WaitCursor(3),
IBeamCursor(4),
SizeVerCursor(5),
SizeHorCursor(6),
SizeBDiagCursor(7),
SizeFDiagCursor(8),
SizeAllCursor(9),
BlankCursor(10),
SplitVCursor(11),
SplitHCursor(12),
PointingHandCursor(13),
ForbiddenCursor(14),
WhatsThisCursor(15),
BusyCursor(16),
OpenHandCursor(17),
ClosedHandCursor(18),
DragCopyCursor(19),
DragMoveCursor(20),
DragLinkCursor(21),
LastCursor(21),
BitmapCursor(24),
CustomCursor(25);
private CursorShape(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static CursorShape resolve(int value) {
switch (value) {
case 0: return ArrowCursor;
case 1: return UpArrowCursor;
case 2: return CrossCursor;
case 3: return WaitCursor;
case 4: return IBeamCursor;
case 5: return SizeVerCursor;
case 6: return SizeHorCursor;
case 7: return SizeBDiagCursor;
case 8: return SizeFDiagCursor;
case 9: return SizeAllCursor;
case 10: return BlankCursor;
case 11: return SplitVCursor;
case 12: return SplitHCursor;
case 13: return PointingHandCursor;
case 14: return ForbiddenCursor;
case 15: return WhatsThisCursor;
case 16: return BusyCursor;
case 17: return OpenHandCursor;
case 18: return ClosedHandCursor;
case 19: return DragCopyCursor;
case 20: return DragMoveCursor;
case 21: return DragLinkCursor;
case 24: return BitmapCursor;
case 25: return CustomCursor;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::DateFormat
*/
public enum DateFormat implements io.qt.QtEnumerator {
TextDate(0),
ISODate(1),
RFC2822Date(8),
ISODateWithMs(9);
private DateFormat(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static DateFormat resolve(int value) {
switch (value) {
case 0: return TextDate;
case 1: return ISODate;
case 8: return RFC2822Date;
case 9: return ISODateWithMs;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::DayOfWeek
*/
public enum DayOfWeek implements io.qt.QtEnumerator {
Monday(1),
Tuesday(2),
Wednesday(3),
Thursday(4),
Friday(5),
Saturday(6),
Sunday(7);
private DayOfWeek(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static DayOfWeek resolve(int value) {
switch (value) {
case 1: return Monday;
case 2: return Tuesday;
case 3: return Wednesday;
case 4: return Thursday;
case 5: return Friday;
case 6: return Saturday;
case 7: return Sunday;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::DockWidgetArea
*
* @see DockWidgetAreas
*/
@io.qt.QtRejectedEntries({"AllDockWidgetAreas"})
public enum DockWidgetArea implements io.qt.QtFlagEnumerator {
LeftDockWidgetArea(1),
RightDockWidgetArea(2),
TopDockWidgetArea(4),
BottomDockWidgetArea(8),
DockWidgetArea_Mask(15),
AllDockWidgetAreas(15),
NoDockWidgetArea(0);
private DockWidgetArea(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public DockWidgetAreas asFlags() {
return new DockWidgetAreas(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public DockWidgetAreas combined(DockWidgetArea e) {
return new DockWidgetAreas(this, e);
}
/**
* Creates a new {@link DockWidgetAreas} from the entries.
* @param values entries
* @return new flag
*/
public static DockWidgetAreas flags(DockWidgetArea ... values) {
return new DockWidgetAreas(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static DockWidgetArea resolve(int value) {
switch (value) {
case 1: return LeftDockWidgetArea;
case 2: return RightDockWidgetArea;
case 4: return TopDockWidgetArea;
case 8: return BottomDockWidgetArea;
case 15: return DockWidgetArea_Mask;
case 0: return NoDockWidgetArea;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link DockWidgetArea}
*/
public static final class DockWidgetAreas extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x552b51f58f9b406cL;
/**
* Creates a new DockWidgetAreas where the flags in args
are set.
* @param args enum entries
*/
public DockWidgetAreas(DockWidgetArea ... args){
super(args);
}
/**
* Creates a new DockWidgetAreas with given value
.
* @param value
*/
public DockWidgetAreas(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new DockWidgetAreas
*/
@Override
public final DockWidgetAreas combined(DockWidgetArea e){
return new DockWidgetAreas(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final DockWidgetAreas setFlag(DockWidgetArea e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final DockWidgetAreas setFlag(DockWidgetArea e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this DockWidgetAreas.
* @return array of enum entries
*/
@Override
public final DockWidgetArea[] flags(){
return super.flags(DockWidgetArea.values());
}
/**
* {@inheritDoc}
*/
@Override
public final DockWidgetAreas clone(){
return new DockWidgetAreas(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(DockWidgetAreas other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::DockWidgetAreaSizes
*/
@io.qt.QtUnlistedEnum
public enum DockWidgetAreaSizes implements io.qt.QtEnumerator {
NDockWidgetAreas(4);
private DockWidgetAreaSizes(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static DockWidgetAreaSizes resolve(int value) {
switch (value) {
case 4: return NDockWidgetAreas;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::DropAction
*
* @see DropActions
*/
public enum DropAction implements io.qt.QtFlagEnumerator {
CopyAction(1),
MoveAction(2),
LinkAction(4),
ActionMask(255),
TargetMoveAction(32770),
IgnoreAction(0);
private DropAction(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public DropActions asFlags() {
return new DropActions(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public DropActions combined(DropAction e) {
return new DropActions(this, e);
}
/**
* Creates a new {@link DropActions} from the entries.
* @param values entries
* @return new flag
*/
public static DropActions flags(DropAction ... values) {
return new DropActions(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static DropAction resolve(int value) {
switch (value) {
case 1: return CopyAction;
case 2: return MoveAction;
case 4: return LinkAction;
case 255: return ActionMask;
case 32770: return TargetMoveAction;
case 0: return IgnoreAction;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link DropAction}
*/
public static final class DropActions extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xadd267923fb12d1cL;
/**
* Creates a new DropActions where the flags in args
are set.
* @param args enum entries
*/
public DropActions(DropAction ... args){
super(args);
}
/**
* Creates a new DropActions with given value
.
* @param value
*/
public DropActions(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new DropActions
*/
@Override
public final DropActions combined(DropAction e){
return new DropActions(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final DropActions setFlag(DropAction e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final DropActions setFlag(DropAction e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this DropActions.
* @return array of enum entries
*/
@Override
public final DropAction[] flags(){
return super.flags(DropAction.values());
}
/**
* {@inheritDoc}
*/
@Override
public final DropActions clone(){
return new DropActions(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(DropActions other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::Edge
*
* @see Edges
*/
public enum Edge implements io.qt.QtFlagEnumerator {
TopEdge(1),
LeftEdge(2),
RightEdge(4),
BottomEdge(8);
private Edge(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public Edges asFlags() {
return new Edges(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public Edges combined(Edge e) {
return new Edges(this, e);
}
/**
* Creates a new {@link Edges} from the entries.
* @param values entries
* @return new flag
*/
public static Edges flags(Edge ... values) {
return new Edges(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Edge resolve(int value) {
switch (value) {
case 1: return TopEdge;
case 2: return LeftEdge;
case 4: return RightEdge;
case 8: return BottomEdge;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link Edge}
*/
public static final class Edges extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x20fd89038447ec2dL;
/**
* Creates a new Edges where the flags in args
are set.
* @param args enum entries
*/
public Edges(Edge ... args){
super(args);
}
/**
* Creates a new Edges with given value
.
* @param value
*/
public Edges(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new Edges
*/
@Override
public final Edges combined(Edge e){
return new Edges(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final Edges setFlag(Edge e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final Edges setFlag(Edge e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this Edges.
* @return array of enum entries
*/
@Override
public final Edge[] flags(){
return super.flags(Edge.values());
}
/**
* {@inheritDoc}
*/
@Override
public final Edges clone(){
return new Edges(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(Edges other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::EnterKeyType
*/
public enum EnterKeyType implements io.qt.QtEnumerator {
EnterKeyDefault(0),
EnterKeyReturn(1),
EnterKeyDone(2),
EnterKeyGo(3),
EnterKeySend(4),
EnterKeySearch(5),
EnterKeyNext(6),
EnterKeyPrevious(7);
private EnterKeyType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static EnterKeyType resolve(int value) {
switch (value) {
case 0: return EnterKeyDefault;
case 1: return EnterKeyReturn;
case 2: return EnterKeyDone;
case 3: return EnterKeyGo;
case 4: return EnterKeySend;
case 5: return EnterKeySearch;
case 6: return EnterKeyNext;
case 7: return EnterKeyPrevious;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::EventPriority
*/
@io.qt.QtUnlistedEnum
public enum EventPriority implements io.qt.QtEnumerator {
HighEventPriority(1),
NormalEventPriority(0),
LowEventPriority(-1);
private EventPriority(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static EventPriority resolve(int value) {
switch (value) {
case 1: return HighEventPriority;
case 0: return NormalEventPriority;
case -1: return LowEventPriority;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::FillRule
*/
public enum FillRule implements io.qt.QtEnumerator {
OddEvenFill(0),
WindingFill(1);
private FillRule(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FillRule resolve(int value) {
switch (value) {
case 0: return OddEvenFill;
case 1: return WindingFill;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::FindChildOption
*
* @see FindChildOptions
*/
public enum FindChildOption implements io.qt.QtFlagEnumerator {
FindDirectChildrenOnly(0),
FindChildrenRecursively(1);
private FindChildOption(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public FindChildOptions asFlags() {
return new FindChildOptions(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public FindChildOptions combined(FindChildOption e) {
return new FindChildOptions(this, e);
}
/**
* Creates a new {@link FindChildOptions} from the entries.
* @param values entries
* @return new flag
*/
public static FindChildOptions flags(FindChildOption ... values) {
return new FindChildOptions(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FindChildOption resolve(int value) {
switch (value) {
case 0: return FindDirectChildrenOnly;
case 1: return FindChildrenRecursively;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link FindChildOption}
*/
public static final class FindChildOptions extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x77feb73874bdf5baL;
/**
* Creates a new FindChildOptions where the flags in args
are set.
* @param args enum entries
*/
public FindChildOptions(FindChildOption ... args){
super(args);
}
/**
* Creates a new FindChildOptions with given value
.
* @param value
*/
public FindChildOptions(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new FindChildOptions
*/
@Override
public final FindChildOptions combined(FindChildOption e){
return new FindChildOptions(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final FindChildOptions setFlag(FindChildOption e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final FindChildOptions setFlag(FindChildOption e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this FindChildOptions.
* @return array of enum entries
*/
@Override
public final FindChildOption[] flags(){
return super.flags(FindChildOption.values());
}
/**
* {@inheritDoc}
*/
@Override
public final FindChildOptions clone(){
return new FindChildOptions(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(FindChildOptions other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::FocusPolicy
*/
public enum FocusPolicy implements io.qt.QtEnumerator {
NoFocus(0),
TabFocus(1),
ClickFocus(2),
StrongFocus(11),
WheelFocus(15);
private FocusPolicy(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FocusPolicy resolve(int value) {
switch (value) {
case 0: return NoFocus;
case 1: return TabFocus;
case 2: return ClickFocus;
case 11: return StrongFocus;
case 15: return WheelFocus;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::FocusReason
*/
public enum FocusReason implements io.qt.QtEnumerator {
MouseFocusReason(0),
TabFocusReason(1),
BacktabFocusReason(2),
ActiveWindowFocusReason(3),
PopupFocusReason(4),
ShortcutFocusReason(5),
MenuBarFocusReason(6),
OtherFocusReason(7),
NoFocusReason(8);
private FocusReason(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FocusReason resolve(int value) {
switch (value) {
case 0: return MouseFocusReason;
case 1: return TabFocusReason;
case 2: return BacktabFocusReason;
case 3: return ActiveWindowFocusReason;
case 4: return PopupFocusReason;
case 5: return ShortcutFocusReason;
case 6: return MenuBarFocusReason;
case 7: return OtherFocusReason;
case 8: return NoFocusReason;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::GestureFlag
*
* @see GestureFlags
*/
public enum GestureFlag implements io.qt.QtFlagEnumerator {
DontStartGestureOnChildren(1),
ReceivePartialGestures(2),
IgnoredGesturesPropagateToParent(4);
private GestureFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public GestureFlags asFlags() {
return new GestureFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public GestureFlags combined(GestureFlag e) {
return new GestureFlags(this, e);
}
/**
* Creates a new {@link GestureFlags} from the entries.
* @param values entries
* @return new flag
*/
public static GestureFlags flags(GestureFlag ... values) {
return new GestureFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static GestureFlag resolve(int value) {
switch (value) {
case 1: return DontStartGestureOnChildren;
case 2: return ReceivePartialGestures;
case 4: return IgnoredGesturesPropagateToParent;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link GestureFlag}
*/
public static final class GestureFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xcf327bb14988470aL;
/**
* Creates a new GestureFlags where the flags in args
are set.
* @param args enum entries
*/
public GestureFlags(GestureFlag ... args){
super(args);
}
/**
* Creates a new GestureFlags with given value
.
* @param value
*/
public GestureFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new GestureFlags
*/
@Override
public final GestureFlags combined(GestureFlag e){
return new GestureFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final GestureFlags setFlag(GestureFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final GestureFlags setFlag(GestureFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this GestureFlags.
* @return array of enum entries
*/
@Override
public final GestureFlag[] flags(){
return super.flags(GestureFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final GestureFlags clone(){
return new GestureFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(GestureFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::GestureState
*/
public enum GestureState implements io.qt.QtEnumerator {
NoGesture(0),
GestureStarted(1),
GestureUpdated(2),
GestureFinished(3),
GestureCanceled(4);
private GestureState(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static GestureState resolve(int value) {
switch (value) {
case 0: return NoGesture;
case 1: return GestureStarted;
case 2: return GestureUpdated;
case 3: return GestureFinished;
case 4: return GestureCanceled;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::GestureType
*/
public enum GestureType implements io.qt.QtEnumerator {
TapGesture(1),
TapAndHoldGesture(2),
PanGesture(3),
PinchGesture(4),
SwipeGesture(5),
CustomGesture(256),
LastGestureType(-1);
private GestureType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static GestureType resolve(int value) {
switch (value) {
case 1: return TapGesture;
case 2: return TapAndHoldGesture;
case 3: return PanGesture;
case 4: return PinchGesture;
case 5: return SwipeGesture;
case 256: return CustomGesture;
case -1: return LastGestureType;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::GlobalColor
*/
public enum GlobalColor implements io.qt.QtEnumerator {
color0(0),
color1(1),
black(2),
white(3),
darkGray(4),
gray(5),
lightGray(6),
red(7),
green(8),
blue(9),
cyan(10),
magenta(11),
yellow(12),
darkRed(13),
darkGreen(14),
darkBlue(15),
darkCyan(16),
darkMagenta(17),
darkYellow(18),
transparent(19);
private GlobalColor(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static GlobalColor resolve(int value) {
switch (value) {
case 0: return color0;
case 1: return color1;
case 2: return black;
case 3: return white;
case 4: return darkGray;
case 5: return gray;
case 6: return lightGray;
case 7: return red;
case 8: return green;
case 9: return blue;
case 10: return cyan;
case 11: return magenta;
case 12: return yellow;
case 13: return darkRed;
case 14: return darkGreen;
case 15: return darkBlue;
case 16: return darkCyan;
case 17: return darkMagenta;
case 18: return darkYellow;
case 19: return transparent;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::HighDpiScaleFactorRoundingPolicy
*/
public enum HighDpiScaleFactorRoundingPolicy implements io.qt.QtEnumerator {
Unset(0),
Round(1),
Ceil(2),
Floor(3),
RoundPreferFloor(4),
PassThrough(5);
private HighDpiScaleFactorRoundingPolicy(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static HighDpiScaleFactorRoundingPolicy resolve(int value) {
switch (value) {
case 0: return Unset;
case 1: return Round;
case 2: return Ceil;
case 3: return Floor;
case 4: return RoundPreferFloor;
case 5: return PassThrough;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::HitTestAccuracy
*/
@io.qt.QtUnlistedEnum
public enum HitTestAccuracy implements io.qt.QtEnumerator {
ExactHit(0),
FuzzyHit(1);
private HitTestAccuracy(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static HitTestAccuracy resolve(int value) {
switch (value) {
case 0: return ExactHit;
case 1: return FuzzyHit;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ImageConversionFlag
*
* @see ImageConversionFlags
*/
@io.qt.QtRejectedEntries({"ColorOnly", "ThresholdAlphaDither", "NoAlpha", "DiffuseDither", "AutoDither"})
public enum ImageConversionFlag implements io.qt.QtFlagEnumerator {
ColorMode_Mask(3),
AutoColor(0),
ColorOnly(3),
MonoOnly(2),
AlphaDither_Mask(12),
ThresholdAlphaDither(0),
OrderedAlphaDither(4),
DiffuseAlphaDither(8),
NoAlpha(12),
Dither_Mask(48),
DiffuseDither(0),
OrderedDither(16),
ThresholdDither(32),
DitherMode_Mask(192),
AutoDither(0),
PreferDither(64),
AvoidDither(128),
NoOpaqueDetection(256),
NoFormatConversion(512);
private ImageConversionFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public ImageConversionFlags asFlags() {
return new ImageConversionFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public ImageConversionFlags combined(ImageConversionFlag e) {
return new ImageConversionFlags(this, e);
}
/**
* Creates a new {@link ImageConversionFlags} from the entries.
* @param values entries
* @return new flag
*/
public static ImageConversionFlags flags(ImageConversionFlag ... values) {
return new ImageConversionFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ImageConversionFlag resolve(int value) {
switch (value) {
case 3: return ColorMode_Mask;
case 0: return AutoColor;
case 2: return MonoOnly;
case 12: return AlphaDither_Mask;
case 4: return OrderedAlphaDither;
case 8: return DiffuseAlphaDither;
case 48: return Dither_Mask;
case 16: return OrderedDither;
case 32: return ThresholdDither;
case 192: return DitherMode_Mask;
case 64: return PreferDither;
case 128: return AvoidDither;
case 256: return NoOpaqueDetection;
case 512: return NoFormatConversion;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link ImageConversionFlag}
*/
public static final class ImageConversionFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x42fe882ed9d09702L;
/**
* Creates a new ImageConversionFlags where the flags in args
are set.
* @param args enum entries
*/
public ImageConversionFlags(ImageConversionFlag ... args){
super(args);
}
/**
* Creates a new ImageConversionFlags with given value
.
* @param value
*/
public ImageConversionFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new ImageConversionFlags
*/
@Override
public final ImageConversionFlags combined(ImageConversionFlag e){
return new ImageConversionFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final ImageConversionFlags setFlag(ImageConversionFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final ImageConversionFlags setFlag(ImageConversionFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this ImageConversionFlags.
* @return array of enum entries
*/
@Override
public final ImageConversionFlag[] flags(){
return super.flags(ImageConversionFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final ImageConversionFlags clone(){
return new ImageConversionFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(ImageConversionFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::InputMethodHint
*
* @see InputMethodHints
*/
public enum InputMethodHint implements io.qt.QtFlagEnumerator {
ImhNone(0),
ImhHiddenText(1),
ImhSensitiveData(2),
ImhNoAutoUppercase(4),
ImhPreferNumbers(8),
ImhPreferUppercase(16),
ImhPreferLowercase(32),
ImhNoPredictiveText(64),
ImhDate(128),
ImhTime(256),
ImhPreferLatin(512),
ImhMultiLine(1024),
ImhNoEditMenu(2048),
ImhNoTextHandles(4096),
ImhDigitsOnly(65536),
ImhFormattedNumbersOnly(131072),
ImhUppercaseOnly(262144),
ImhLowercaseOnly(524288),
ImhDialableCharactersOnly(1048576),
ImhEmailCharactersOnly(2097152),
ImhUrlCharactersOnly(4194304),
ImhLatinOnly(8388608),
ImhExclusiveInputMask(-65536);
private InputMethodHint(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public InputMethodHints asFlags() {
return new InputMethodHints(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public InputMethodHints combined(InputMethodHint e) {
return new InputMethodHints(this, e);
}
/**
* Creates a new {@link InputMethodHints} from the entries.
* @param values entries
* @return new flag
*/
public static InputMethodHints flags(InputMethodHint ... values) {
return new InputMethodHints(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static InputMethodHint resolve(int value) {
switch (value) {
case 0: return ImhNone;
case 1: return ImhHiddenText;
case 2: return ImhSensitiveData;
case 4: return ImhNoAutoUppercase;
case 8: return ImhPreferNumbers;
case 16: return ImhPreferUppercase;
case 32: return ImhPreferLowercase;
case 64: return ImhNoPredictiveText;
case 128: return ImhDate;
case 256: return ImhTime;
case 512: return ImhPreferLatin;
case 1024: return ImhMultiLine;
case 2048: return ImhNoEditMenu;
case 4096: return ImhNoTextHandles;
case 65536: return ImhDigitsOnly;
case 131072: return ImhFormattedNumbersOnly;
case 262144: return ImhUppercaseOnly;
case 524288: return ImhLowercaseOnly;
case 1048576: return ImhDialableCharactersOnly;
case 2097152: return ImhEmailCharactersOnly;
case 4194304: return ImhUrlCharactersOnly;
case 8388608: return ImhLatinOnly;
case -65536: return ImhExclusiveInputMask;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link InputMethodHint}
*/
public static final class InputMethodHints extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x422c18840ddc0d4bL;
/**
* Creates a new InputMethodHints where the flags in args
are set.
* @param args enum entries
*/
public InputMethodHints(InputMethodHint ... args){
super(args);
}
/**
* Creates a new InputMethodHints with given value
.
* @param value
*/
public InputMethodHints(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new InputMethodHints
*/
@Override
public final InputMethodHints combined(InputMethodHint e){
return new InputMethodHints(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final InputMethodHints setFlag(InputMethodHint e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final InputMethodHints setFlag(InputMethodHint e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this InputMethodHints.
* @return array of enum entries
*/
@Override
public final InputMethodHint[] flags(){
return super.flags(InputMethodHint.values());
}
/**
* {@inheritDoc}
*/
@Override
public final InputMethodHints clone(){
return new InputMethodHints(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(InputMethodHints other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::InputMethodQuery
*
* @see InputMethodQueries
*/
public enum InputMethodQuery implements io.qt.QtFlagEnumerator {
ImEnabled(1),
ImCursorRectangle(2),
ImFont(4),
ImCursorPosition(8),
ImSurroundingText(16),
ImCurrentSelection(32),
ImMaximumTextLength(64),
ImAnchorPosition(128),
ImHints(256),
ImPreferredLanguage(512),
ImAbsolutePosition(1024),
ImTextBeforeCursor(2048),
ImTextAfterCursor(4096),
ImEnterKeyType(8192),
ImAnchorRectangle(16384),
ImInputItemClipRectangle(32768),
ImReadOnly(65536),
ImPlatformData(-2147483648),
ImQueryInput(16570),
ImQueryAll(-1);
private InputMethodQuery(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public InputMethodQueries asFlags() {
return new InputMethodQueries(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public InputMethodQueries combined(InputMethodQuery e) {
return new InputMethodQueries(this, e);
}
/**
* Creates a new {@link InputMethodQueries} from the entries.
* @param values entries
* @return new flag
*/
public static InputMethodQueries flags(InputMethodQuery ... values) {
return new InputMethodQueries(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static InputMethodQuery resolve(int value) {
switch (value) {
case 1: return ImEnabled;
case 2: return ImCursorRectangle;
case 4: return ImFont;
case 8: return ImCursorPosition;
case 16: return ImSurroundingText;
case 32: return ImCurrentSelection;
case 64: return ImMaximumTextLength;
case 128: return ImAnchorPosition;
case 256: return ImHints;
case 512: return ImPreferredLanguage;
case 1024: return ImAbsolutePosition;
case 2048: return ImTextBeforeCursor;
case 4096: return ImTextAfterCursor;
case 8192: return ImEnterKeyType;
case 16384: return ImAnchorRectangle;
case 32768: return ImInputItemClipRectangle;
case 65536: return ImReadOnly;
case -2147483648: return ImPlatformData;
case 16570: return ImQueryInput;
case -1: return ImQueryAll;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link InputMethodQuery}
*/
public static final class InputMethodQueries extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x8141066a98439968L;
/**
* Creates a new InputMethodQueries where the flags in args
are set.
* @param args enum entries
*/
public InputMethodQueries(InputMethodQuery ... args){
super(args);
}
/**
* Creates a new InputMethodQueries with given value
.
* @param value
*/
public InputMethodQueries(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new InputMethodQueries
*/
@Override
public final InputMethodQueries combined(InputMethodQuery e){
return new InputMethodQueries(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final InputMethodQueries setFlag(InputMethodQuery e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final InputMethodQueries setFlag(InputMethodQuery e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this InputMethodQueries.
* @return array of enum entries
*/
@Override
public final InputMethodQuery[] flags(){
return super.flags(InputMethodQuery.values());
}
/**
* {@inheritDoc}
*/
@Override
public final InputMethodQueries clone(){
return new InputMethodQueries(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(InputMethodQueries other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::ItemDataRole
*/
public static class ItemDataRole{
public static final int DisplayRole = 0;
public static final int DecorationRole = 1;
public static final int EditRole = 2;
public static final int ToolTipRole = 3;
public static final int StatusTipRole = 4;
public static final int WhatsThisRole = 5;
public static final int FontRole = 6;
public static final int TextAlignmentRole = 7;
public static final int BackgroundRole = 8;
public static final int ForegroundRole = 9;
public static final int CheckStateRole = 10;
public static final int AccessibleTextRole = 11;
public static final int AccessibleDescriptionRole = 12;
public static final int SizeHintRole = 13;
public static final int InitialSortOrderRole = 14;
public static final int DisplayPropertyRole = 27;
public static final int DecorationPropertyRole = 28;
public static final int ToolTipPropertyRole = 29;
public static final int StatusTipPropertyRole = 30;
public static final int WhatsThisPropertyRole = 31;
public static final int UserRole = 256;
} // end of enum ItemDataRole
/**
* Java wrapper for Qt enum Qt::ItemFlag
*
* @see ItemFlags
*/
public enum ItemFlag implements io.qt.QtFlagEnumerator {
NoItemFlags(0),
ItemIsSelectable(1),
ItemIsEditable(2),
ItemIsDragEnabled(4),
ItemIsDropEnabled(8),
ItemIsUserCheckable(16),
ItemIsEnabled(32),
ItemIsAutoTristate(64),
ItemNeverHasChildren(128),
ItemIsUserTristate(256);
private ItemFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public ItemFlags asFlags() {
return new ItemFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public ItemFlags combined(ItemFlag e) {
return new ItemFlags(this, e);
}
/**
* Creates a new {@link ItemFlags} from the entries.
* @param values entries
* @return new flag
*/
public static ItemFlags flags(ItemFlag ... values) {
return new ItemFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ItemFlag resolve(int value) {
switch (value) {
case 0: return NoItemFlags;
case 1: return ItemIsSelectable;
case 2: return ItemIsEditable;
case 4: return ItemIsDragEnabled;
case 8: return ItemIsDropEnabled;
case 16: return ItemIsUserCheckable;
case 32: return ItemIsEnabled;
case 64: return ItemIsAutoTristate;
case 128: return ItemNeverHasChildren;
case 256: return ItemIsUserTristate;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link ItemFlag}
*/
public static final class ItemFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x931af29c02ccfee8L;
/**
* Creates a new ItemFlags where the flags in args
are set.
* @param args enum entries
*/
public ItemFlags(ItemFlag ... args){
super(args);
}
/**
* Creates a new ItemFlags with given value
.
* @param value
*/
public ItemFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new ItemFlags
*/
@Override
public final ItemFlags combined(ItemFlag e){
return new ItemFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final ItemFlags setFlag(ItemFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final ItemFlags setFlag(ItemFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this ItemFlags.
* @return array of enum entries
*/
@Override
public final ItemFlag[] flags(){
return super.flags(ItemFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final ItemFlags clone(){
return new ItemFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(ItemFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::ItemSelectionMode
*/
public enum ItemSelectionMode implements io.qt.QtEnumerator {
ContainsItemShape(0),
IntersectsItemShape(1),
ContainsItemBoundingRect(2),
IntersectsItemBoundingRect(3);
private ItemSelectionMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ItemSelectionMode resolve(int value) {
switch (value) {
case 0: return ContainsItemShape;
case 1: return IntersectsItemShape;
case 2: return ContainsItemBoundingRect;
case 3: return IntersectsItemBoundingRect;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ItemSelectionOperation
*/
public enum ItemSelectionOperation implements io.qt.QtEnumerator {
ReplaceSelection(0),
AddToSelection(1);
private ItemSelectionOperation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ItemSelectionOperation resolve(int value) {
switch (value) {
case 0: return ReplaceSelection;
case 1: return AddToSelection;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::Key
*/
@io.qt.QtRejectedEntries({"Key_Any"})
public enum Key implements io.qt.QtEnumerator {
Key_Space(32),
Key_Any(32),
Key_Exclam(33),
Key_QuoteDbl(34),
Key_NumberSign(35),
Key_Dollar(36),
Key_Percent(37),
Key_Ampersand(38),
Key_Apostrophe(39),
Key_ParenLeft(40),
Key_ParenRight(41),
Key_Asterisk(42),
Key_Plus(43),
Key_Comma(44),
Key_Minus(45),
Key_Period(46),
Key_Slash(47),
Key_0(48),
Key_1(49),
Key_2(50),
Key_3(51),
Key_4(52),
Key_5(53),
Key_6(54),
Key_7(55),
Key_8(56),
Key_9(57),
Key_Colon(58),
Key_Semicolon(59),
Key_Less(60),
Key_Equal(61),
Key_Greater(62),
Key_Question(63),
Key_At(64),
Key_A(65),
Key_B(66),
Key_C(67),
Key_D(68),
Key_E(69),
Key_F(70),
Key_G(71),
Key_H(72),
Key_I(73),
Key_J(74),
Key_K(75),
Key_L(76),
Key_M(77),
Key_N(78),
Key_O(79),
Key_P(80),
Key_Q(81),
Key_R(82),
Key_S(83),
Key_T(84),
Key_U(85),
Key_V(86),
Key_W(87),
Key_X(88),
Key_Y(89),
Key_Z(90),
Key_BracketLeft(91),
Key_Backslash(92),
Key_BracketRight(93),
Key_AsciiCircum(94),
Key_Underscore(95),
Key_QuoteLeft(96),
Key_BraceLeft(123),
Key_Bar(124),
Key_BraceRight(125),
Key_AsciiTilde(126),
Key_nobreakspace(160),
Key_exclamdown(161),
Key_cent(162),
Key_sterling(163),
Key_currency(164),
Key_yen(165),
Key_brokenbar(166),
Key_section(167),
Key_diaeresis(168),
Key_copyright(169),
Key_ordfeminine(170),
Key_guillemotleft(171),
Key_notsign(172),
Key_hyphen(173),
Key_registered(174),
Key_macron(175),
Key_degree(176),
Key_plusminus(177),
Key_twosuperior(178),
Key_threesuperior(179),
Key_acute(180),
Key_mu(181),
Key_paragraph(182),
Key_periodcentered(183),
Key_cedilla(184),
Key_onesuperior(185),
Key_masculine(186),
Key_guillemotright(187),
Key_onequarter(188),
Key_onehalf(189),
Key_threequarters(190),
Key_questiondown(191),
Key_Agrave(192),
Key_Aacute(193),
Key_Acircumflex(194),
Key_Atilde(195),
Key_Adiaeresis(196),
Key_Aring(197),
Key_AE(198),
Key_Ccedilla(199),
Key_Egrave(200),
Key_Eacute(201),
Key_Ecircumflex(202),
Key_Ediaeresis(203),
Key_Igrave(204),
Key_Iacute(205),
Key_Icircumflex(206),
Key_Idiaeresis(207),
Key_ETH(208),
Key_Ntilde(209),
Key_Ograve(210),
Key_Oacute(211),
Key_Ocircumflex(212),
Key_Otilde(213),
Key_Odiaeresis(214),
Key_multiply(215),
Key_Ooblique(216),
Key_Ugrave(217),
Key_Uacute(218),
Key_Ucircumflex(219),
Key_Udiaeresis(220),
Key_Yacute(221),
Key_THORN(222),
Key_ssharp(223),
Key_division(247),
Key_ydiaeresis(255),
Key_Escape(16777216),
Key_Tab(16777217),
Key_Backtab(16777218),
Key_Backspace(16777219),
Key_Return(16777220),
Key_Enter(16777221),
Key_Insert(16777222),
Key_Delete(16777223),
Key_Pause(16777224),
Key_Print(16777225),
Key_SysReq(16777226),
Key_Clear(16777227),
Key_Home(16777232),
Key_End(16777233),
Key_Left(16777234),
Key_Up(16777235),
Key_Right(16777236),
Key_Down(16777237),
Key_PageUp(16777238),
Key_PageDown(16777239),
Key_Shift(16777248),
Key_Control(16777249),
Key_Meta(16777250),
Key_Alt(16777251),
Key_CapsLock(16777252),
Key_NumLock(16777253),
Key_ScrollLock(16777254),
Key_F1(16777264),
Key_F2(16777265),
Key_F3(16777266),
Key_F4(16777267),
Key_F5(16777268),
Key_F6(16777269),
Key_F7(16777270),
Key_F8(16777271),
Key_F9(16777272),
Key_F10(16777273),
Key_F11(16777274),
Key_F12(16777275),
Key_F13(16777276),
Key_F14(16777277),
Key_F15(16777278),
Key_F16(16777279),
Key_F17(16777280),
Key_F18(16777281),
Key_F19(16777282),
Key_F20(16777283),
Key_F21(16777284),
Key_F22(16777285),
Key_F23(16777286),
Key_F24(16777287),
Key_F25(16777288),
Key_F26(16777289),
Key_F27(16777290),
Key_F28(16777291),
Key_F29(16777292),
Key_F30(16777293),
Key_F31(16777294),
Key_F32(16777295),
Key_F33(16777296),
Key_F34(16777297),
Key_F35(16777298),
Key_Super_L(16777299),
Key_Super_R(16777300),
Key_Menu(16777301),
Key_Hyper_L(16777302),
Key_Hyper_R(16777303),
Key_Help(16777304),
Key_Direction_L(16777305),
Key_Direction_R(16777312),
Key_AltGr(16781571),
Key_Multi_key(16781600),
Key_Codeinput(16781623),
Key_SingleCandidate(16781628),
Key_MultipleCandidate(16781629),
Key_PreviousCandidate(16781630),
Key_Mode_switch(16781694),
Key_Kanji(16781601),
Key_Muhenkan(16781602),
Key_Henkan(16781603),
Key_Romaji(16781604),
Key_Hiragana(16781605),
Key_Katakana(16781606),
Key_Hiragana_Katakana(16781607),
Key_Zenkaku(16781608),
Key_Hankaku(16781609),
Key_Zenkaku_Hankaku(16781610),
Key_Touroku(16781611),
Key_Massyo(16781612),
Key_Kana_Lock(16781613),
Key_Kana_Shift(16781614),
Key_Eisu_Shift(16781615),
Key_Eisu_toggle(16781616),
Key_Hangul(16781617),
Key_Hangul_Start(16781618),
Key_Hangul_End(16781619),
Key_Hangul_Hanja(16781620),
Key_Hangul_Jamo(16781621),
Key_Hangul_Romaja(16781622),
Key_Hangul_Jeonja(16781624),
Key_Hangul_Banja(16781625),
Key_Hangul_PreHanja(16781626),
Key_Hangul_PostHanja(16781627),
Key_Hangul_Special(16781631),
Key_Dead_Grave(16781904),
Key_Dead_Acute(16781905),
Key_Dead_Circumflex(16781906),
Key_Dead_Tilde(16781907),
Key_Dead_Macron(16781908),
Key_Dead_Breve(16781909),
Key_Dead_Abovedot(16781910),
Key_Dead_Diaeresis(16781911),
Key_Dead_Abovering(16781912),
Key_Dead_Doubleacute(16781913),
Key_Dead_Caron(16781914),
Key_Dead_Cedilla(16781915),
Key_Dead_Ogonek(16781916),
Key_Dead_Iota(16781917),
Key_Dead_Voiced_Sound(16781918),
Key_Dead_Semivoiced_Sound(16781919),
Key_Dead_Belowdot(16781920),
Key_Dead_Hook(16781921),
Key_Dead_Horn(16781922),
Key_Dead_Stroke(16781923),
Key_Dead_Abovecomma(16781924),
Key_Dead_Abovereversedcomma(16781925),
Key_Dead_Doublegrave(16781926),
Key_Dead_Belowring(16781927),
Key_Dead_Belowmacron(16781928),
Key_Dead_Belowcircumflex(16781929),
Key_Dead_Belowtilde(16781930),
Key_Dead_Belowbreve(16781931),
Key_Dead_Belowdiaeresis(16781932),
Key_Dead_Invertedbreve(16781933),
Key_Dead_Belowcomma(16781934),
Key_Dead_Currency(16781935),
Key_Dead_a(16781952),
Key_Dead_A(16781953),
Key_Dead_e(16781954),
Key_Dead_E(16781955),
Key_Dead_i(16781956),
Key_Dead_I(16781957),
Key_Dead_o(16781958),
Key_Dead_O(16781959),
Key_Dead_u(16781960),
Key_Dead_U(16781961),
Key_Dead_Small_Schwa(16781962),
Key_Dead_Capital_Schwa(16781963),
Key_Dead_Greek(16781964),
Key_Dead_Lowline(16781968),
Key_Dead_Aboveverticalline(16781969),
Key_Dead_Belowverticalline(16781970),
Key_Dead_Longsolidusoverlay(16781971),
Key_Back(16777313),
Key_Forward(16777314),
Key_Stop(16777315),
Key_Refresh(16777316),
Key_VolumeDown(16777328),
Key_VolumeMute(16777329),
Key_VolumeUp(16777330),
Key_BassBoost(16777331),
Key_BassUp(16777332),
Key_BassDown(16777333),
Key_TrebleUp(16777334),
Key_TrebleDown(16777335),
Key_MediaPlay(16777344),
Key_MediaStop(16777345),
Key_MediaPrevious(16777346),
Key_MediaNext(16777347),
Key_MediaRecord(16777348),
Key_MediaPause(16777349),
Key_MediaTogglePlayPause(16777350),
Key_HomePage(16777360),
Key_Favorites(16777361),
Key_Search(16777362),
Key_Standby(16777363),
Key_OpenUrl(16777364),
Key_LaunchMail(16777376),
Key_LaunchMedia(16777377),
Key_Launch0(16777378),
Key_Launch1(16777379),
Key_Launch2(16777380),
Key_Launch3(16777381),
Key_Launch4(16777382),
Key_Launch5(16777383),
Key_Launch6(16777384),
Key_Launch7(16777385),
Key_Launch8(16777386),
Key_Launch9(16777387),
Key_LaunchA(16777388),
Key_LaunchB(16777389),
Key_LaunchC(16777390),
Key_LaunchD(16777391),
Key_LaunchE(16777392),
Key_LaunchF(16777393),
Key_MonBrightnessUp(16777394),
Key_MonBrightnessDown(16777395),
Key_KeyboardLightOnOff(16777396),
Key_KeyboardBrightnessUp(16777397),
Key_KeyboardBrightnessDown(16777398),
Key_PowerOff(16777399),
Key_WakeUp(16777400),
Key_Eject(16777401),
Key_ScreenSaver(16777402),
Key_WWW(16777403),
Key_Memo(16777404),
Key_LightBulb(16777405),
Key_Shop(16777406),
Key_History(16777407),
Key_AddFavorite(16777408),
Key_HotLinks(16777409),
Key_BrightnessAdjust(16777410),
Key_Finance(16777411),
Key_Community(16777412),
Key_AudioRewind(16777413),
Key_BackForward(16777414),
Key_ApplicationLeft(16777415),
Key_ApplicationRight(16777416),
Key_Book(16777417),
Key_CD(16777418),
Key_Calculator(16777419),
Key_ToDoList(16777420),
Key_ClearGrab(16777421),
Key_Close(16777422),
Key_Copy(16777423),
Key_Cut(16777424),
Key_Display(16777425),
Key_DOS(16777426),
Key_Documents(16777427),
Key_Excel(16777428),
Key_Explorer(16777429),
Key_Game(16777430),
Key_Go(16777431),
Key_iTouch(16777432),
Key_LogOff(16777433),
Key_Market(16777434),
Key_Meeting(16777435),
Key_MenuKB(16777436),
Key_MenuPB(16777437),
Key_MySites(16777438),
Key_News(16777439),
Key_OfficeHome(16777440),
Key_Option(16777441),
Key_Paste(16777442),
Key_Phone(16777443),
Key_Calendar(16777444),
Key_Reply(16777445),
Key_Reload(16777446),
Key_RotateWindows(16777447),
Key_RotationPB(16777448),
Key_RotationKB(16777449),
Key_Save(16777450),
Key_Send(16777451),
Key_Spell(16777452),
Key_SplitScreen(16777453),
Key_Support(16777454),
Key_TaskPane(16777455),
Key_Terminal(16777456),
Key_Tools(16777457),
Key_Travel(16777458),
Key_Video(16777459),
Key_Word(16777460),
Key_Xfer(16777461),
Key_ZoomIn(16777462),
Key_ZoomOut(16777463),
Key_Away(16777464),
Key_Messenger(16777465),
Key_WebCam(16777466),
Key_MailForward(16777467),
Key_Pictures(16777468),
Key_Music(16777469),
Key_Battery(16777470),
Key_Bluetooth(16777471),
Key_WLAN(16777472),
Key_UWB(16777473),
Key_AudioForward(16777474),
Key_AudioRepeat(16777475),
Key_AudioRandomPlay(16777476),
Key_Subtitle(16777477),
Key_AudioCycleTrack(16777478),
Key_Time(16777479),
Key_Hibernate(16777480),
Key_View(16777481),
Key_TopMenu(16777482),
Key_PowerDown(16777483),
Key_Suspend(16777484),
Key_ContrastAdjust(16777485),
Key_LaunchG(16777486),
Key_LaunchH(16777487),
Key_TouchpadToggle(16777488),
Key_TouchpadOn(16777489),
Key_TouchpadOff(16777490),
Key_MicMute(16777491),
Key_Red(16777492),
Key_Green(16777493),
Key_Yellow(16777494),
Key_Blue(16777495),
Key_ChannelUp(16777496),
Key_ChannelDown(16777497),
Key_Guide(16777498),
Key_Info(16777499),
Key_Settings(16777500),
Key_MicVolumeUp(16777501),
Key_MicVolumeDown(16777502),
Key_New(16777504),
Key_Open(16777505),
Key_Find(16777506),
Key_Undo(16777507),
Key_Redo(16777508),
Key_MediaLast(16842751),
Key_Select(16842752),
Key_Yes(16842753),
Key_No(16842754),
Key_Cancel(16908289),
Key_Printer(16908290),
Key_Execute(16908291),
Key_Sleep(16908292),
Key_Play(16908293),
Key_Zoom(16908294),
Key_Exit(16908298),
Key_Context1(17825792),
Key_Context2(17825793),
Key_Context3(17825794),
Key_Context4(17825795),
Key_Call(17825796),
Key_Hangup(17825797),
Key_Flip(17825798),
Key_ToggleCallHangup(17825799),
Key_VoiceDial(17825800),
Key_LastNumberRedial(17825801),
Key_Camera(17825824),
Key_CameraFocus(17825825),
Key_unknown(33554431);
private Key(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Key resolve(int value) {
switch (value) {
case 32: return Key_Space;
case 33: return Key_Exclam;
case 34: return Key_QuoteDbl;
case 35: return Key_NumberSign;
case 36: return Key_Dollar;
case 37: return Key_Percent;
case 38: return Key_Ampersand;
case 39: return Key_Apostrophe;
case 40: return Key_ParenLeft;
case 41: return Key_ParenRight;
case 42: return Key_Asterisk;
case 43: return Key_Plus;
case 44: return Key_Comma;
case 45: return Key_Minus;
case 46: return Key_Period;
case 47: return Key_Slash;
case 48: return Key_0;
case 49: return Key_1;
case 50: return Key_2;
case 51: return Key_3;
case 52: return Key_4;
case 53: return Key_5;
case 54: return Key_6;
case 55: return Key_7;
case 56: return Key_8;
case 57: return Key_9;
case 58: return Key_Colon;
case 59: return Key_Semicolon;
case 60: return Key_Less;
case 61: return Key_Equal;
case 62: return Key_Greater;
case 63: return Key_Question;
case 64: return Key_At;
case 65: return Key_A;
case 66: return Key_B;
case 67: return Key_C;
case 68: return Key_D;
case 69: return Key_E;
case 70: return Key_F;
case 71: return Key_G;
case 72: return Key_H;
case 73: return Key_I;
case 74: return Key_J;
case 75: return Key_K;
case 76: return Key_L;
case 77: return Key_M;
case 78: return Key_N;
case 79: return Key_O;
case 80: return Key_P;
case 81: return Key_Q;
case 82: return Key_R;
case 83: return Key_S;
case 84: return Key_T;
case 85: return Key_U;
case 86: return Key_V;
case 87: return Key_W;
case 88: return Key_X;
case 89: return Key_Y;
case 90: return Key_Z;
case 91: return Key_BracketLeft;
case 92: return Key_Backslash;
case 93: return Key_BracketRight;
case 94: return Key_AsciiCircum;
case 95: return Key_Underscore;
case 96: return Key_QuoteLeft;
case 123: return Key_BraceLeft;
case 124: return Key_Bar;
case 125: return Key_BraceRight;
case 126: return Key_AsciiTilde;
case 160: return Key_nobreakspace;
case 161: return Key_exclamdown;
case 162: return Key_cent;
case 163: return Key_sterling;
case 164: return Key_currency;
case 165: return Key_yen;
case 166: return Key_brokenbar;
case 167: return Key_section;
case 168: return Key_diaeresis;
case 169: return Key_copyright;
case 170: return Key_ordfeminine;
case 171: return Key_guillemotleft;
case 172: return Key_notsign;
case 173: return Key_hyphen;
case 174: return Key_registered;
case 175: return Key_macron;
case 176: return Key_degree;
case 177: return Key_plusminus;
case 178: return Key_twosuperior;
case 179: return Key_threesuperior;
case 180: return Key_acute;
case 181: return Key_mu;
case 182: return Key_paragraph;
case 183: return Key_periodcentered;
case 184: return Key_cedilla;
case 185: return Key_onesuperior;
case 186: return Key_masculine;
case 187: return Key_guillemotright;
case 188: return Key_onequarter;
case 189: return Key_onehalf;
case 190: return Key_threequarters;
case 191: return Key_questiondown;
case 192: return Key_Agrave;
case 193: return Key_Aacute;
case 194: return Key_Acircumflex;
case 195: return Key_Atilde;
case 196: return Key_Adiaeresis;
case 197: return Key_Aring;
case 198: return Key_AE;
case 199: return Key_Ccedilla;
case 200: return Key_Egrave;
case 201: return Key_Eacute;
case 202: return Key_Ecircumflex;
case 203: return Key_Ediaeresis;
case 204: return Key_Igrave;
case 205: return Key_Iacute;
case 206: return Key_Icircumflex;
case 207: return Key_Idiaeresis;
case 208: return Key_ETH;
case 209: return Key_Ntilde;
case 210: return Key_Ograve;
case 211: return Key_Oacute;
case 212: return Key_Ocircumflex;
case 213: return Key_Otilde;
case 214: return Key_Odiaeresis;
case 215: return Key_multiply;
case 216: return Key_Ooblique;
case 217: return Key_Ugrave;
case 218: return Key_Uacute;
case 219: return Key_Ucircumflex;
case 220: return Key_Udiaeresis;
case 221: return Key_Yacute;
case 222: return Key_THORN;
case 223: return Key_ssharp;
case 247: return Key_division;
case 255: return Key_ydiaeresis;
case 16777216: return Key_Escape;
case 16777217: return Key_Tab;
case 16777218: return Key_Backtab;
case 16777219: return Key_Backspace;
case 16777220: return Key_Return;
case 16777221: return Key_Enter;
case 16777222: return Key_Insert;
case 16777223: return Key_Delete;
case 16777224: return Key_Pause;
case 16777225: return Key_Print;
case 16777226: return Key_SysReq;
case 16777227: return Key_Clear;
case 16777232: return Key_Home;
case 16777233: return Key_End;
case 16777234: return Key_Left;
case 16777235: return Key_Up;
case 16777236: return Key_Right;
case 16777237: return Key_Down;
case 16777238: return Key_PageUp;
case 16777239: return Key_PageDown;
case 16777248: return Key_Shift;
case 16777249: return Key_Control;
case 16777250: return Key_Meta;
case 16777251: return Key_Alt;
case 16777252: return Key_CapsLock;
case 16777253: return Key_NumLock;
case 16777254: return Key_ScrollLock;
case 16777264: return Key_F1;
case 16777265: return Key_F2;
case 16777266: return Key_F3;
case 16777267: return Key_F4;
case 16777268: return Key_F5;
case 16777269: return Key_F6;
case 16777270: return Key_F7;
case 16777271: return Key_F8;
case 16777272: return Key_F9;
case 16777273: return Key_F10;
case 16777274: return Key_F11;
case 16777275: return Key_F12;
case 16777276: return Key_F13;
case 16777277: return Key_F14;
case 16777278: return Key_F15;
case 16777279: return Key_F16;
case 16777280: return Key_F17;
case 16777281: return Key_F18;
case 16777282: return Key_F19;
case 16777283: return Key_F20;
case 16777284: return Key_F21;
case 16777285: return Key_F22;
case 16777286: return Key_F23;
case 16777287: return Key_F24;
case 16777288: return Key_F25;
case 16777289: return Key_F26;
case 16777290: return Key_F27;
case 16777291: return Key_F28;
case 16777292: return Key_F29;
case 16777293: return Key_F30;
case 16777294: return Key_F31;
case 16777295: return Key_F32;
case 16777296: return Key_F33;
case 16777297: return Key_F34;
case 16777298: return Key_F35;
case 16777299: return Key_Super_L;
case 16777300: return Key_Super_R;
case 16777301: return Key_Menu;
case 16777302: return Key_Hyper_L;
case 16777303: return Key_Hyper_R;
case 16777304: return Key_Help;
case 16777305: return Key_Direction_L;
case 16777312: return Key_Direction_R;
case 16781571: return Key_AltGr;
case 16781600: return Key_Multi_key;
case 16781623: return Key_Codeinput;
case 16781628: return Key_SingleCandidate;
case 16781629: return Key_MultipleCandidate;
case 16781630: return Key_PreviousCandidate;
case 16781694: return Key_Mode_switch;
case 16781601: return Key_Kanji;
case 16781602: return Key_Muhenkan;
case 16781603: return Key_Henkan;
case 16781604: return Key_Romaji;
case 16781605: return Key_Hiragana;
case 16781606: return Key_Katakana;
case 16781607: return Key_Hiragana_Katakana;
case 16781608: return Key_Zenkaku;
case 16781609: return Key_Hankaku;
case 16781610: return Key_Zenkaku_Hankaku;
case 16781611: return Key_Touroku;
case 16781612: return Key_Massyo;
case 16781613: return Key_Kana_Lock;
case 16781614: return Key_Kana_Shift;
case 16781615: return Key_Eisu_Shift;
case 16781616: return Key_Eisu_toggle;
case 16781617: return Key_Hangul;
case 16781618: return Key_Hangul_Start;
case 16781619: return Key_Hangul_End;
case 16781620: return Key_Hangul_Hanja;
case 16781621: return Key_Hangul_Jamo;
case 16781622: return Key_Hangul_Romaja;
case 16781624: return Key_Hangul_Jeonja;
case 16781625: return Key_Hangul_Banja;
case 16781626: return Key_Hangul_PreHanja;
case 16781627: return Key_Hangul_PostHanja;
case 16781631: return Key_Hangul_Special;
case 16781904: return Key_Dead_Grave;
case 16781905: return Key_Dead_Acute;
case 16781906: return Key_Dead_Circumflex;
case 16781907: return Key_Dead_Tilde;
case 16781908: return Key_Dead_Macron;
case 16781909: return Key_Dead_Breve;
case 16781910: return Key_Dead_Abovedot;
case 16781911: return Key_Dead_Diaeresis;
case 16781912: return Key_Dead_Abovering;
case 16781913: return Key_Dead_Doubleacute;
case 16781914: return Key_Dead_Caron;
case 16781915: return Key_Dead_Cedilla;
case 16781916: return Key_Dead_Ogonek;
case 16781917: return Key_Dead_Iota;
case 16781918: return Key_Dead_Voiced_Sound;
case 16781919: return Key_Dead_Semivoiced_Sound;
case 16781920: return Key_Dead_Belowdot;
case 16781921: return Key_Dead_Hook;
case 16781922: return Key_Dead_Horn;
case 16781923: return Key_Dead_Stroke;
case 16781924: return Key_Dead_Abovecomma;
case 16781925: return Key_Dead_Abovereversedcomma;
case 16781926: return Key_Dead_Doublegrave;
case 16781927: return Key_Dead_Belowring;
case 16781928: return Key_Dead_Belowmacron;
case 16781929: return Key_Dead_Belowcircumflex;
case 16781930: return Key_Dead_Belowtilde;
case 16781931: return Key_Dead_Belowbreve;
case 16781932: return Key_Dead_Belowdiaeresis;
case 16781933: return Key_Dead_Invertedbreve;
case 16781934: return Key_Dead_Belowcomma;
case 16781935: return Key_Dead_Currency;
case 16781952: return Key_Dead_a;
case 16781953: return Key_Dead_A;
case 16781954: return Key_Dead_e;
case 16781955: return Key_Dead_E;
case 16781956: return Key_Dead_i;
case 16781957: return Key_Dead_I;
case 16781958: return Key_Dead_o;
case 16781959: return Key_Dead_O;
case 16781960: return Key_Dead_u;
case 16781961: return Key_Dead_U;
case 16781962: return Key_Dead_Small_Schwa;
case 16781963: return Key_Dead_Capital_Schwa;
case 16781964: return Key_Dead_Greek;
case 16781968: return Key_Dead_Lowline;
case 16781969: return Key_Dead_Aboveverticalline;
case 16781970: return Key_Dead_Belowverticalline;
case 16781971: return Key_Dead_Longsolidusoverlay;
case 16777313: return Key_Back;
case 16777314: return Key_Forward;
case 16777315: return Key_Stop;
case 16777316: return Key_Refresh;
case 16777328: return Key_VolumeDown;
case 16777329: return Key_VolumeMute;
case 16777330: return Key_VolumeUp;
case 16777331: return Key_BassBoost;
case 16777332: return Key_BassUp;
case 16777333: return Key_BassDown;
case 16777334: return Key_TrebleUp;
case 16777335: return Key_TrebleDown;
case 16777344: return Key_MediaPlay;
case 16777345: return Key_MediaStop;
case 16777346: return Key_MediaPrevious;
case 16777347: return Key_MediaNext;
case 16777348: return Key_MediaRecord;
case 16777349: return Key_MediaPause;
case 16777350: return Key_MediaTogglePlayPause;
case 16777360: return Key_HomePage;
case 16777361: return Key_Favorites;
case 16777362: return Key_Search;
case 16777363: return Key_Standby;
case 16777364: return Key_OpenUrl;
case 16777376: return Key_LaunchMail;
case 16777377: return Key_LaunchMedia;
case 16777378: return Key_Launch0;
case 16777379: return Key_Launch1;
case 16777380: return Key_Launch2;
case 16777381: return Key_Launch3;
case 16777382: return Key_Launch4;
case 16777383: return Key_Launch5;
case 16777384: return Key_Launch6;
case 16777385: return Key_Launch7;
case 16777386: return Key_Launch8;
case 16777387: return Key_Launch9;
case 16777388: return Key_LaunchA;
case 16777389: return Key_LaunchB;
case 16777390: return Key_LaunchC;
case 16777391: return Key_LaunchD;
case 16777392: return Key_LaunchE;
case 16777393: return Key_LaunchF;
case 16777394: return Key_MonBrightnessUp;
case 16777395: return Key_MonBrightnessDown;
case 16777396: return Key_KeyboardLightOnOff;
case 16777397: return Key_KeyboardBrightnessUp;
case 16777398: return Key_KeyboardBrightnessDown;
case 16777399: return Key_PowerOff;
case 16777400: return Key_WakeUp;
case 16777401: return Key_Eject;
case 16777402: return Key_ScreenSaver;
case 16777403: return Key_WWW;
case 16777404: return Key_Memo;
case 16777405: return Key_LightBulb;
case 16777406: return Key_Shop;
case 16777407: return Key_History;
case 16777408: return Key_AddFavorite;
case 16777409: return Key_HotLinks;
case 16777410: return Key_BrightnessAdjust;
case 16777411: return Key_Finance;
case 16777412: return Key_Community;
case 16777413: return Key_AudioRewind;
case 16777414: return Key_BackForward;
case 16777415: return Key_ApplicationLeft;
case 16777416: return Key_ApplicationRight;
case 16777417: return Key_Book;
case 16777418: return Key_CD;
case 16777419: return Key_Calculator;
case 16777420: return Key_ToDoList;
case 16777421: return Key_ClearGrab;
case 16777422: return Key_Close;
case 16777423: return Key_Copy;
case 16777424: return Key_Cut;
case 16777425: return Key_Display;
case 16777426: return Key_DOS;
case 16777427: return Key_Documents;
case 16777428: return Key_Excel;
case 16777429: return Key_Explorer;
case 16777430: return Key_Game;
case 16777431: return Key_Go;
case 16777432: return Key_iTouch;
case 16777433: return Key_LogOff;
case 16777434: return Key_Market;
case 16777435: return Key_Meeting;
case 16777436: return Key_MenuKB;
case 16777437: return Key_MenuPB;
case 16777438: return Key_MySites;
case 16777439: return Key_News;
case 16777440: return Key_OfficeHome;
case 16777441: return Key_Option;
case 16777442: return Key_Paste;
case 16777443: return Key_Phone;
case 16777444: return Key_Calendar;
case 16777445: return Key_Reply;
case 16777446: return Key_Reload;
case 16777447: return Key_RotateWindows;
case 16777448: return Key_RotationPB;
case 16777449: return Key_RotationKB;
case 16777450: return Key_Save;
case 16777451: return Key_Send;
case 16777452: return Key_Spell;
case 16777453: return Key_SplitScreen;
case 16777454: return Key_Support;
case 16777455: return Key_TaskPane;
case 16777456: return Key_Terminal;
case 16777457: return Key_Tools;
case 16777458: return Key_Travel;
case 16777459: return Key_Video;
case 16777460: return Key_Word;
case 16777461: return Key_Xfer;
case 16777462: return Key_ZoomIn;
case 16777463: return Key_ZoomOut;
case 16777464: return Key_Away;
case 16777465: return Key_Messenger;
case 16777466: return Key_WebCam;
case 16777467: return Key_MailForward;
case 16777468: return Key_Pictures;
case 16777469: return Key_Music;
case 16777470: return Key_Battery;
case 16777471: return Key_Bluetooth;
case 16777472: return Key_WLAN;
case 16777473: return Key_UWB;
case 16777474: return Key_AudioForward;
case 16777475: return Key_AudioRepeat;
case 16777476: return Key_AudioRandomPlay;
case 16777477: return Key_Subtitle;
case 16777478: return Key_AudioCycleTrack;
case 16777479: return Key_Time;
case 16777480: return Key_Hibernate;
case 16777481: return Key_View;
case 16777482: return Key_TopMenu;
case 16777483: return Key_PowerDown;
case 16777484: return Key_Suspend;
case 16777485: return Key_ContrastAdjust;
case 16777486: return Key_LaunchG;
case 16777487: return Key_LaunchH;
case 16777488: return Key_TouchpadToggle;
case 16777489: return Key_TouchpadOn;
case 16777490: return Key_TouchpadOff;
case 16777491: return Key_MicMute;
case 16777492: return Key_Red;
case 16777493: return Key_Green;
case 16777494: return Key_Yellow;
case 16777495: return Key_Blue;
case 16777496: return Key_ChannelUp;
case 16777497: return Key_ChannelDown;
case 16777498: return Key_Guide;
case 16777499: return Key_Info;
case 16777500: return Key_Settings;
case 16777501: return Key_MicVolumeUp;
case 16777502: return Key_MicVolumeDown;
case 16777504: return Key_New;
case 16777505: return Key_Open;
case 16777506: return Key_Find;
case 16777507: return Key_Undo;
case 16777508: return Key_Redo;
case 16842751: return Key_MediaLast;
case 16842752: return Key_Select;
case 16842753: return Key_Yes;
case 16842754: return Key_No;
case 16908289: return Key_Cancel;
case 16908290: return Key_Printer;
case 16908291: return Key_Execute;
case 16908292: return Key_Sleep;
case 16908293: return Key_Play;
case 16908294: return Key_Zoom;
case 16908298: return Key_Exit;
case 17825792: return Key_Context1;
case 17825793: return Key_Context2;
case 17825794: return Key_Context3;
case 17825795: return Key_Context4;
case 17825796: return Key_Call;
case 17825797: return Key_Hangup;
case 17825798: return Key_Flip;
case 17825799: return Key_ToggleCallHangup;
case 17825800: return Key_VoiceDial;
case 17825801: return Key_LastNumberRedial;
case 17825824: return Key_Camera;
case 17825825: return Key_CameraFocus;
case 33554431: return Key_unknown;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::KeyboardModifier
*
* @see KeyboardModifiers
*/
public enum KeyboardModifier implements io.qt.QtFlagEnumerator {
NoModifier(0),
ShiftModifier(33554432),
ControlModifier(67108864),
AltModifier(134217728),
MetaModifier(268435456),
KeypadModifier(536870912),
GroupSwitchModifier(1073741824),
KeyboardModifierMask(-33554432);
private KeyboardModifier(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public KeyboardModifiers asFlags() {
return new KeyboardModifiers(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public KeyboardModifiers combined(KeyboardModifier e) {
return new KeyboardModifiers(this, e);
}
/**
* Creates a new {@link KeyboardModifiers} from the entries.
* @param values entries
* @return new flag
*/
public static KeyboardModifiers flags(KeyboardModifier ... values) {
return new KeyboardModifiers(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static KeyboardModifier resolve(int value) {
switch (value) {
case 0: return NoModifier;
case 33554432: return ShiftModifier;
case 67108864: return ControlModifier;
case 134217728: return AltModifier;
case 268435456: return MetaModifier;
case 536870912: return KeypadModifier;
case 1073741824: return GroupSwitchModifier;
case -33554432: return KeyboardModifierMask;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link KeyboardModifier}
*/
public static final class KeyboardModifiers extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x1e5b543af4a5c2cL;
/**
* Creates a new KeyboardModifiers where the flags in args
are set.
* @param args enum entries
*/
public KeyboardModifiers(KeyboardModifier ... args){
super(args);
}
/**
* Creates a new KeyboardModifiers with given value
.
* @param value
*/
public KeyboardModifiers(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new KeyboardModifiers
*/
@Override
public final KeyboardModifiers combined(KeyboardModifier e){
return new KeyboardModifiers(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final KeyboardModifiers setFlag(KeyboardModifier e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final KeyboardModifiers setFlag(KeyboardModifier e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this KeyboardModifiers.
* @return array of enum entries
*/
@Override
public final KeyboardModifier[] flags(){
return super.flags(KeyboardModifier.values());
}
/**
* {@inheritDoc}
*/
@Override
public final KeyboardModifiers clone(){
return new KeyboardModifiers(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(KeyboardModifiers other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::LayoutDirection
*/
public enum LayoutDirection implements io.qt.QtEnumerator {
LeftToRight(0),
RightToLeft(1),
LayoutDirectionAuto(2);
private LayoutDirection(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static LayoutDirection resolve(int value) {
switch (value) {
case 0: return LeftToRight;
case 1: return RightToLeft;
case 2: return LayoutDirectionAuto;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::MaskMode
*/
public enum MaskMode implements io.qt.QtEnumerator {
MaskInColor(0),
MaskOutColor(1);
private MaskMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static MaskMode resolve(int value) {
switch (value) {
case 0: return MaskInColor;
case 1: return MaskOutColor;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::MatchFlag
*
* @see MatchFlags
*/
public enum MatchFlag implements io.qt.QtFlagEnumerator {
MatchExactly(0),
MatchContains(1),
MatchStartsWith(2),
MatchEndsWith(3),
MatchRegularExpression(4),
MatchWildcard(5),
MatchFixedString(8),
MatchTypeMask(15),
MatchCaseSensitive(16),
MatchWrap(32),
MatchRecursive(64);
private MatchFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public MatchFlags asFlags() {
return new MatchFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public MatchFlags combined(MatchFlag e) {
return new MatchFlags(this, e);
}
/**
* Creates a new {@link MatchFlags} from the entries.
* @param values entries
* @return new flag
*/
public static MatchFlags flags(MatchFlag ... values) {
return new MatchFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static MatchFlag resolve(int value) {
switch (value) {
case 0: return MatchExactly;
case 1: return MatchContains;
case 2: return MatchStartsWith;
case 3: return MatchEndsWith;
case 4: return MatchRegularExpression;
case 5: return MatchWildcard;
case 8: return MatchFixedString;
case 15: return MatchTypeMask;
case 16: return MatchCaseSensitive;
case 32: return MatchWrap;
case 64: return MatchRecursive;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link MatchFlag}
*/
public static final class MatchFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xe52b74c57cb33994L;
/**
* Creates a new MatchFlags where the flags in args
are set.
* @param args enum entries
*/
public MatchFlags(MatchFlag ... args){
super(args);
}
/**
* Creates a new MatchFlags with given value
.
* @param value
*/
public MatchFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new MatchFlags
*/
@Override
public final MatchFlags combined(MatchFlag e){
return new MatchFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final MatchFlags setFlag(MatchFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final MatchFlags setFlag(MatchFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this MatchFlags.
* @return array of enum entries
*/
@Override
public final MatchFlag[] flags(){
return super.flags(MatchFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final MatchFlags clone(){
return new MatchFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(MatchFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::MouseButton
*
* @see MouseButtons
*/
@io.qt.QtRejectedEntries({"BackButton", "XButton1", "ForwardButton", "XButton2", "TaskButton", "MaxMouseButton"})
public enum MouseButton implements io.qt.QtFlagEnumerator {
NoButton(0),
LeftButton(1),
RightButton(2),
MiddleButton(4),
BackButton(8),
XButton1(8),
ExtraButton1(8),
ForwardButton(16),
XButton2(16),
ExtraButton2(16),
TaskButton(32),
ExtraButton3(32),
ExtraButton4(64),
ExtraButton5(128),
ExtraButton6(256),
ExtraButton7(512),
ExtraButton8(1024),
ExtraButton9(2048),
ExtraButton10(4096),
ExtraButton11(8192),
ExtraButton12(16384),
ExtraButton13(32768),
ExtraButton14(65536),
ExtraButton15(131072),
ExtraButton16(262144),
ExtraButton17(524288),
ExtraButton18(1048576),
ExtraButton19(2097152),
ExtraButton20(4194304),
ExtraButton21(8388608),
ExtraButton22(16777216),
ExtraButton23(33554432),
ExtraButton24(67108864),
AllButtons(134217727),
MaxMouseButton(67108864),
MouseButtonMask(-1);
private MouseButton(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public MouseButtons asFlags() {
return new MouseButtons(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public MouseButtons combined(MouseButton e) {
return new MouseButtons(this, e);
}
/**
* Creates a new {@link MouseButtons} from the entries.
* @param values entries
* @return new flag
*/
public static MouseButtons flags(MouseButton ... values) {
return new MouseButtons(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static MouseButton resolve(int value) {
switch (value) {
case 0: return NoButton;
case 1: return LeftButton;
case 2: return RightButton;
case 4: return MiddleButton;
case 8: return ExtraButton1;
case 16: return ExtraButton2;
case 32: return ExtraButton3;
case 64: return ExtraButton4;
case 128: return ExtraButton5;
case 256: return ExtraButton6;
case 512: return ExtraButton7;
case 1024: return ExtraButton8;
case 2048: return ExtraButton9;
case 4096: return ExtraButton10;
case 8192: return ExtraButton11;
case 16384: return ExtraButton12;
case 32768: return ExtraButton13;
case 65536: return ExtraButton14;
case 131072: return ExtraButton15;
case 262144: return ExtraButton16;
case 524288: return ExtraButton17;
case 1048576: return ExtraButton18;
case 2097152: return ExtraButton19;
case 4194304: return ExtraButton20;
case 8388608: return ExtraButton21;
case 16777216: return ExtraButton22;
case 33554432: return ExtraButton23;
case 67108864: return ExtraButton24;
case 134217727: return AllButtons;
case -1: return MouseButtonMask;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link MouseButton}
*/
public static final class MouseButtons extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xd0ea232122520ba8L;
/**
* Creates a new MouseButtons where the flags in args
are set.
* @param args enum entries
*/
public MouseButtons(MouseButton ... args){
super(args);
}
/**
* Creates a new MouseButtons with given value
.
* @param value
*/
public MouseButtons(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new MouseButtons
*/
@Override
public final MouseButtons combined(MouseButton e){
return new MouseButtons(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final MouseButtons setFlag(MouseButton e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final MouseButtons setFlag(MouseButton e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this MouseButtons.
* @return array of enum entries
*/
@Override
public final MouseButton[] flags(){
return super.flags(MouseButton.values());
}
/**
* {@inheritDoc}
*/
@Override
public final MouseButtons clone(){
return new MouseButtons(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(MouseButtons other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::MouseEventFlag
*
* @see MouseEventFlags
*/
public enum MouseEventFlag implements io.qt.QtFlagEnumerator {
NoMouseEventFlag(0),
MouseEventCreatedDoubleClick(1),
MouseEventFlagMask(255);
private MouseEventFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public MouseEventFlags asFlags() {
return new MouseEventFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public MouseEventFlags combined(MouseEventFlag e) {
return new MouseEventFlags(this, e);
}
/**
* Creates a new {@link MouseEventFlags} from the entries.
* @param values entries
* @return new flag
*/
public static MouseEventFlags flags(MouseEventFlag ... values) {
return new MouseEventFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static MouseEventFlag resolve(int value) {
switch (value) {
case 0: return NoMouseEventFlag;
case 1: return MouseEventCreatedDoubleClick;
case 255: return MouseEventFlagMask;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link MouseEventFlag}
*/
public static final class MouseEventFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x16a152411e32883fL;
/**
* Creates a new MouseEventFlags where the flags in args
are set.
* @param args enum entries
*/
public MouseEventFlags(MouseEventFlag ... args){
super(args);
}
/**
* Creates a new MouseEventFlags with given value
.
* @param value
*/
public MouseEventFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new MouseEventFlags
*/
@Override
public final MouseEventFlags combined(MouseEventFlag e){
return new MouseEventFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final MouseEventFlags setFlag(MouseEventFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final MouseEventFlags setFlag(MouseEventFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this MouseEventFlags.
* @return array of enum entries
*/
@Override
public final MouseEventFlag[] flags(){
return super.flags(MouseEventFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final MouseEventFlags clone(){
return new MouseEventFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(MouseEventFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::MouseEventSource
*/
public enum MouseEventSource implements io.qt.QtEnumerator {
MouseEventNotSynthesized(0),
MouseEventSynthesizedBySystem(1),
MouseEventSynthesizedByQt(2),
MouseEventSynthesizedByApplication(3);
private MouseEventSource(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static MouseEventSource resolve(int value) {
switch (value) {
case 0: return MouseEventNotSynthesized;
case 1: return MouseEventSynthesizedBySystem;
case 2: return MouseEventSynthesizedByQt;
case 3: return MouseEventSynthesizedByApplication;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::NativeGestureType
*/
public enum NativeGestureType implements io.qt.QtEnumerator {
BeginNativeGesture(0),
EndNativeGesture(1),
PanNativeGesture(2),
ZoomNativeGesture(3),
SmartZoomNativeGesture(4),
RotateNativeGesture(5),
SwipeNativeGesture(6);
private NativeGestureType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static NativeGestureType resolve(int value) {
switch (value) {
case 0: return BeginNativeGesture;
case 1: return EndNativeGesture;
case 2: return PanNativeGesture;
case 3: return ZoomNativeGesture;
case 4: return SmartZoomNativeGesture;
case 5: return RotateNativeGesture;
case 6: return SwipeNativeGesture;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::NavigationMode
*/
@io.qt.QtUnlistedEnum
public enum NavigationMode implements io.qt.QtEnumerator {
NavigationModeNone(0),
NavigationModeKeypadTabOrder(1),
NavigationModeKeypadDirectional(2),
NavigationModeCursorAuto(3),
NavigationModeCursorForceVisible(4);
private NavigationMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static NavigationMode resolve(int value) {
switch (value) {
case 0: return NavigationModeNone;
case 1: return NavigationModeKeypadTabOrder;
case 2: return NavigationModeKeypadDirectional;
case 3: return NavigationModeCursorAuto;
case 4: return NavigationModeCursorForceVisible;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::Orientation
*
* @see Orientations
*/
public enum Orientation implements io.qt.QtFlagEnumerator {
Horizontal(1),
Vertical(2);
private Orientation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public Orientations asFlags() {
return new Orientations(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public Orientations combined(Orientation e) {
return new Orientations(this, e);
}
/**
* Creates a new {@link Orientations} from the entries.
* @param values entries
* @return new flag
*/
public static Orientations flags(Orientation ... values) {
return new Orientations(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Orientation resolve(int value) {
switch (value) {
case 1: return Horizontal;
case 2: return Vertical;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link Orientation}
*/
public static final class Orientations extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x86e5ea6bbe4bd92fL;
/**
* Creates a new Orientations where the flags in args
are set.
* @param args enum entries
*/
public Orientations(Orientation ... args){
super(args);
}
/**
* Creates a new Orientations with given value
.
* @param value
*/
public Orientations(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new Orientations
*/
@Override
public final Orientations combined(Orientation e){
return new Orientations(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final Orientations setFlag(Orientation e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final Orientations setFlag(Orientation e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this Orientations.
* @return array of enum entries
*/
@Override
public final Orientation[] flags(){
return super.flags(Orientation.values());
}
/**
* {@inheritDoc}
*/
@Override
public final Orientations clone(){
return new Orientations(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(Orientations other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::PenCapStyle
*/
public enum PenCapStyle implements io.qt.QtEnumerator {
FlatCap(0),
SquareCap(16),
RoundCap(32),
MPenCapStyle(48);
private PenCapStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PenCapStyle resolve(int value) {
switch (value) {
case 0: return FlatCap;
case 16: return SquareCap;
case 32: return RoundCap;
case 48: return MPenCapStyle;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::PenJoinStyle
*/
public enum PenJoinStyle implements io.qt.QtEnumerator {
MiterJoin(0),
BevelJoin(64),
RoundJoin(128),
SvgMiterJoin(256),
MPenJoinStyle(448);
private PenJoinStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PenJoinStyle resolve(int value) {
switch (value) {
case 0: return MiterJoin;
case 64: return BevelJoin;
case 128: return RoundJoin;
case 256: return SvgMiterJoin;
case 448: return MPenJoinStyle;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::PenStyle
*/
public enum PenStyle implements io.qt.QtEnumerator {
NoPen(0),
SolidLine(1),
DashLine(2),
DotLine(3),
DashDotLine(4),
DashDotDotLine(5),
CustomDashLine(6),
MPenStyle(15);
private PenStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PenStyle resolve(int value) {
switch (value) {
case 0: return NoPen;
case 1: return SolidLine;
case 2: return DashLine;
case 3: return DotLine;
case 4: return DashDotLine;
case 5: return DashDotDotLine;
case 6: return CustomDashLine;
case 15: return MPenStyle;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ScreenOrientation
*
* @see ScreenOrientations
*/
public enum ScreenOrientation implements io.qt.QtFlagEnumerator {
PrimaryOrientation(0),
PortraitOrientation(1),
LandscapeOrientation(2),
InvertedPortraitOrientation(4),
InvertedLandscapeOrientation(8);
private ScreenOrientation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public ScreenOrientations asFlags() {
return new ScreenOrientations(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public ScreenOrientations combined(ScreenOrientation e) {
return new ScreenOrientations(this, e);
}
/**
* Creates a new {@link ScreenOrientations} from the entries.
* @param values entries
* @return new flag
*/
public static ScreenOrientations flags(ScreenOrientation ... values) {
return new ScreenOrientations(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ScreenOrientation resolve(int value) {
switch (value) {
case 0: return PrimaryOrientation;
case 1: return PortraitOrientation;
case 2: return LandscapeOrientation;
case 4: return InvertedPortraitOrientation;
case 8: return InvertedLandscapeOrientation;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link ScreenOrientation}
*/
public static final class ScreenOrientations extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x9f66ee8fc16dc747L;
/**
* Creates a new ScreenOrientations where the flags in args
are set.
* @param args enum entries
*/
public ScreenOrientations(ScreenOrientation ... args){
super(args);
}
/**
* Creates a new ScreenOrientations with given value
.
* @param value
*/
public ScreenOrientations(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new ScreenOrientations
*/
@Override
public final ScreenOrientations combined(ScreenOrientation e){
return new ScreenOrientations(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final ScreenOrientations setFlag(ScreenOrientation e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final ScreenOrientations setFlag(ScreenOrientation e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this ScreenOrientations.
* @return array of enum entries
*/
@Override
public final ScreenOrientation[] flags(){
return super.flags(ScreenOrientation.values());
}
/**
* {@inheritDoc}
*/
@Override
public final ScreenOrientations clone(){
return new ScreenOrientations(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(ScreenOrientations other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::ScrollBarPolicy
*/
public enum ScrollBarPolicy implements io.qt.QtEnumerator {
ScrollBarAsNeeded(0),
ScrollBarAlwaysOff(1),
ScrollBarAlwaysOn(2);
private ScrollBarPolicy(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ScrollBarPolicy resolve(int value) {
switch (value) {
case 0: return ScrollBarAsNeeded;
case 1: return ScrollBarAlwaysOff;
case 2: return ScrollBarAlwaysOn;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ScrollPhase
*/
public enum ScrollPhase implements io.qt.QtEnumerator {
NoScrollPhase(0),
ScrollBegin(1),
ScrollUpdate(2),
ScrollEnd(3),
ScrollMomentum(4);
private ScrollPhase(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ScrollPhase resolve(int value) {
switch (value) {
case 0: return NoScrollPhase;
case 1: return ScrollBegin;
case 2: return ScrollUpdate;
case 3: return ScrollEnd;
case 4: return ScrollMomentum;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ShortcutContext
*/
public enum ShortcutContext implements io.qt.QtEnumerator {
WidgetShortcut(0),
WindowShortcut(1),
ApplicationShortcut(2),
WidgetWithChildrenShortcut(3);
private ShortcutContext(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ShortcutContext resolve(int value) {
switch (value) {
case 0: return WidgetShortcut;
case 1: return WindowShortcut;
case 2: return ApplicationShortcut;
case 3: return WidgetWithChildrenShortcut;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::SizeHint
*/
public enum SizeHint implements io.qt.QtEnumerator {
MinimumSize(0),
PreferredSize(1),
MaximumSize(2),
MinimumDescent(3),
NSizeHints(4);
private SizeHint(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SizeHint resolve(int value) {
switch (value) {
case 0: return MinimumSize;
case 1: return PreferredSize;
case 2: return MaximumSize;
case 3: return MinimumDescent;
case 4: return NSizeHints;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::SizeMode
*/
public enum SizeMode implements io.qt.QtEnumerator {
AbsoluteSize(0),
RelativeSize(1);
private SizeMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SizeMode resolve(int value) {
switch (value) {
case 0: return AbsoluteSize;
case 1: return RelativeSize;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::SortOrder
*/
public enum SortOrder implements io.qt.QtEnumerator {
AscendingOrder(0),
DescendingOrder(1);
private SortOrder(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SortOrder resolve(int value) {
switch (value) {
case 0: return AscendingOrder;
case 1: return DescendingOrder;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::SplitBehaviorFlags
*
* @see SplitBehavior
*/
public enum SplitBehaviorFlags implements io.qt.QtFlagEnumerator {
KeepEmptyParts(0),
SkipEmptyParts(1);
private SplitBehaviorFlags(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public SplitBehavior asFlags() {
return new SplitBehavior(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public SplitBehavior combined(SplitBehaviorFlags e) {
return new SplitBehavior(this, e);
}
/**
* Creates a new {@link SplitBehavior} from the entries.
* @param values entries
* @return new flag
*/
public static SplitBehavior flags(SplitBehaviorFlags ... values) {
return new SplitBehavior(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SplitBehaviorFlags resolve(int value) {
switch (value) {
case 0: return KeepEmptyParts;
case 1: return SkipEmptyParts;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link SplitBehaviorFlags}
*/
public static final class SplitBehavior extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x7383c0ed51069a39L;
/**
* Creates a new SplitBehavior where the flags in args
are set.
* @param args enum entries
*/
public SplitBehavior(SplitBehaviorFlags ... args){
super(args);
}
/**
* Creates a new SplitBehavior with given value
.
* @param value
*/
public SplitBehavior(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new SplitBehavior
*/
@Override
public final SplitBehavior combined(SplitBehaviorFlags e){
return new SplitBehavior(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final SplitBehavior setFlag(SplitBehaviorFlags e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final SplitBehavior setFlag(SplitBehaviorFlags e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this SplitBehavior.
* @return array of enum entries
*/
@Override
public final SplitBehaviorFlags[] flags(){
return super.flags(SplitBehaviorFlags.values());
}
/**
* {@inheritDoc}
*/
@Override
public final SplitBehavior clone(){
return new SplitBehavior(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(SplitBehavior other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::TabFocusBehavior
*/
public enum TabFocusBehavior implements io.qt.QtEnumerator {
NoTabFocus(0),
TabFocusTextControls(1),
TabFocusListControls(2),
TabFocusAllControls(255);
private TabFocusBehavior(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TabFocusBehavior resolve(int value) {
switch (value) {
case 0: return NoTabFocus;
case 1: return TabFocusTextControls;
case 2: return TabFocusListControls;
case 255: return TabFocusAllControls;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TextElideMode
*/
public enum TextElideMode implements io.qt.QtEnumerator {
ElideLeft(0),
ElideRight(1),
ElideMiddle(2),
ElideNone(3);
private TextElideMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TextElideMode resolve(int value) {
switch (value) {
case 0: return ElideLeft;
case 1: return ElideRight;
case 2: return ElideMiddle;
case 3: return ElideNone;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TextFlag
*/
public enum TextFlag implements io.qt.QtEnumerator {
TextSingleLine(256),
TextDontClip(512),
TextExpandTabs(1024),
TextShowMnemonic(2048),
TextWordWrap(4096),
TextWrapAnywhere(8192),
TextDontPrint(16384),
TextIncludeTrailingSpaces(134217728),
TextHideMnemonic(32768),
TextJustificationForced(65536),
TextForceLeftToRight(131072),
TextForceRightToLeft(262144),
TextLongestVariant(524288);
private TextFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TextFlag resolve(int value) {
switch (value) {
case 256: return TextSingleLine;
case 512: return TextDontClip;
case 1024: return TextExpandTabs;
case 2048: return TextShowMnemonic;
case 4096: return TextWordWrap;
case 8192: return TextWrapAnywhere;
case 16384: return TextDontPrint;
case 134217728: return TextIncludeTrailingSpaces;
case 32768: return TextHideMnemonic;
case 65536: return TextJustificationForced;
case 131072: return TextForceLeftToRight;
case 262144: return TextForceRightToLeft;
case 524288: return TextLongestVariant;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TextFormat
*/
public enum TextFormat implements io.qt.QtEnumerator {
PlainText(0),
RichText(1),
AutoText(2),
MarkdownText(3);
private TextFormat(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TextFormat resolve(int value) {
switch (value) {
case 0: return PlainText;
case 1: return RichText;
case 2: return AutoText;
case 3: return MarkdownText;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TextInteractionFlag
*
* @see TextInteractionFlags
*/
public enum TextInteractionFlag implements io.qt.QtFlagEnumerator {
NoTextInteraction(0),
TextSelectableByMouse(1),
TextSelectableByKeyboard(2),
LinksAccessibleByMouse(4),
LinksAccessibleByKeyboard(8),
TextEditable(16),
TextEditorInteraction(19),
TextBrowserInteraction(13);
private TextInteractionFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public TextInteractionFlags asFlags() {
return new TextInteractionFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public TextInteractionFlags combined(TextInteractionFlag e) {
return new TextInteractionFlags(this, e);
}
/**
* Creates a new {@link TextInteractionFlags} from the entries.
* @param values entries
* @return new flag
*/
public static TextInteractionFlags flags(TextInteractionFlag ... values) {
return new TextInteractionFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TextInteractionFlag resolve(int value) {
switch (value) {
case 0: return NoTextInteraction;
case 1: return TextSelectableByMouse;
case 2: return TextSelectableByKeyboard;
case 4: return LinksAccessibleByMouse;
case 8: return LinksAccessibleByKeyboard;
case 16: return TextEditable;
case 19: return TextEditorInteraction;
case 13: return TextBrowserInteraction;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link TextInteractionFlag}
*/
public static final class TextInteractionFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xd88b7287f6140537L;
/**
* Creates a new TextInteractionFlags where the flags in args
are set.
* @param args enum entries
*/
public TextInteractionFlags(TextInteractionFlag ... args){
super(args);
}
/**
* Creates a new TextInteractionFlags with given value
.
* @param value
*/
public TextInteractionFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new TextInteractionFlags
*/
@Override
public final TextInteractionFlags combined(TextInteractionFlag e){
return new TextInteractionFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final TextInteractionFlags setFlag(TextInteractionFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final TextInteractionFlags setFlag(TextInteractionFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this TextInteractionFlags.
* @return array of enum entries
*/
@Override
public final TextInteractionFlag[] flags(){
return super.flags(TextInteractionFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final TextInteractionFlags clone(){
return new TextInteractionFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(TextInteractionFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::TileRule
*/
@io.qt.QtUnlistedEnum
public enum TileRule implements io.qt.QtEnumerator {
StretchTile(0),
RepeatTile(1),
RoundTile(2);
private TileRule(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TileRule resolve(int value) {
switch (value) {
case 0: return StretchTile;
case 1: return RepeatTile;
case 2: return RoundTile;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TimeSpec
*/
public enum TimeSpec implements io.qt.QtEnumerator {
LocalTime(0),
UTC(1),
OffsetFromUTC(2),
TimeZone(3);
private TimeSpec(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TimeSpec resolve(int value) {
switch (value) {
case 0: return LocalTime;
case 1: return UTC;
case 2: return OffsetFromUTC;
case 3: return TimeZone;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TimerType
*/
public enum TimerType implements io.qt.QtEnumerator {
PreciseTimer(0),
CoarseTimer(1),
VeryCoarseTimer(2);
private TimerType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TimerType resolve(int value) {
switch (value) {
case 0: return PreciseTimer;
case 1: return CoarseTimer;
case 2: return VeryCoarseTimer;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ToolBarArea
*
* @see ToolBarAreas
*/
@io.qt.QtRejectedEntries({"AllToolBarAreas"})
public enum ToolBarArea implements io.qt.QtFlagEnumerator {
LeftToolBarArea(1),
RightToolBarArea(2),
TopToolBarArea(4),
BottomToolBarArea(8),
ToolBarArea_Mask(15),
AllToolBarAreas(15),
NoToolBarArea(0);
private ToolBarArea(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public ToolBarAreas asFlags() {
return new ToolBarAreas(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public ToolBarAreas combined(ToolBarArea e) {
return new ToolBarAreas(this, e);
}
/**
* Creates a new {@link ToolBarAreas} from the entries.
* @param values entries
* @return new flag
*/
public static ToolBarAreas flags(ToolBarArea ... values) {
return new ToolBarAreas(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ToolBarArea resolve(int value) {
switch (value) {
case 1: return LeftToolBarArea;
case 2: return RightToolBarArea;
case 4: return TopToolBarArea;
case 8: return BottomToolBarArea;
case 15: return ToolBarArea_Mask;
case 0: return NoToolBarArea;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link ToolBarArea}
*/
public static final class ToolBarAreas extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x8a1d8360260b7e0fL;
/**
* Creates a new ToolBarAreas where the flags in args
are set.
* @param args enum entries
*/
public ToolBarAreas(ToolBarArea ... args){
super(args);
}
/**
* Creates a new ToolBarAreas with given value
.
* @param value
*/
public ToolBarAreas(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new ToolBarAreas
*/
@Override
public final ToolBarAreas combined(ToolBarArea e){
return new ToolBarAreas(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final ToolBarAreas setFlag(ToolBarArea e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final ToolBarAreas setFlag(ToolBarArea e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this ToolBarAreas.
* @return array of enum entries
*/
@Override
public final ToolBarArea[] flags(){
return super.flags(ToolBarArea.values());
}
/**
* {@inheritDoc}
*/
@Override
public final ToolBarAreas clone(){
return new ToolBarAreas(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(ToolBarAreas other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::ToolBarAreaSizes
*/
@io.qt.QtUnlistedEnum
public enum ToolBarAreaSizes implements io.qt.QtEnumerator {
NToolBarAreas(4);
private ToolBarAreaSizes(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ToolBarAreaSizes resolve(int value) {
switch (value) {
case 4: return NToolBarAreas;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::ToolButtonStyle
*/
public enum ToolButtonStyle implements io.qt.QtEnumerator {
ToolButtonIconOnly(0),
ToolButtonTextOnly(1),
ToolButtonTextBesideIcon(2),
ToolButtonTextUnderIcon(3),
ToolButtonFollowStyle(4);
private ToolButtonStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ToolButtonStyle resolve(int value) {
switch (value) {
case 0: return ToolButtonIconOnly;
case 1: return ToolButtonTextOnly;
case 2: return ToolButtonTextBesideIcon;
case 3: return ToolButtonTextUnderIcon;
case 4: return ToolButtonFollowStyle;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::TouchPointState
*
* @see TouchPointStates
*/
public enum TouchPointState implements io.qt.QtFlagEnumerator {
TouchPointUnknownState(0),
TouchPointPressed(1),
TouchPointMoved(2),
TouchPointStationary(4),
TouchPointReleased(8);
private TouchPointState(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public TouchPointStates asFlags() {
return new TouchPointStates(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public TouchPointStates combined(TouchPointState e) {
return new TouchPointStates(this, e);
}
/**
* Creates a new {@link TouchPointStates} from the entries.
* @param values entries
* @return new flag
*/
public static TouchPointStates flags(TouchPointState ... values) {
return new TouchPointStates(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TouchPointState resolve(int value) {
switch (value) {
case 0: return TouchPointUnknownState;
case 1: return TouchPointPressed;
case 2: return TouchPointMoved;
case 4: return TouchPointStationary;
case 8: return TouchPointReleased;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link TouchPointState}
*/
public static final class TouchPointStates extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xf76302d6bf84f56dL;
/**
* Creates a new TouchPointStates where the flags in args
are set.
* @param args enum entries
*/
public TouchPointStates(TouchPointState ... args){
super(args);
}
/**
* Creates a new TouchPointStates with given value
.
* @param value
*/
public TouchPointStates(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new TouchPointStates
*/
@Override
public final TouchPointStates combined(TouchPointState e){
return new TouchPointStates(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final TouchPointStates setFlag(TouchPointState e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final TouchPointStates setFlag(TouchPointState e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this TouchPointStates.
* @return array of enum entries
*/
@Override
public final TouchPointState[] flags(){
return super.flags(TouchPointState.values());
}
/**
* {@inheritDoc}
*/
@Override
public final TouchPointStates clone(){
return new TouchPointStates(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(TouchPointStates other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::TransformationMode
*/
public enum TransformationMode implements io.qt.QtEnumerator {
FastTransformation(0),
SmoothTransformation(1);
private TransformationMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static TransformationMode resolve(int value) {
switch (value) {
case 0: return FastTransformation;
case 1: return SmoothTransformation;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::UIEffect
*/
@io.qt.QtUnlistedEnum
public enum UIEffect implements io.qt.QtEnumerator {
UI_General(0),
UI_AnimateMenu(1),
UI_FadeMenu(2),
UI_AnimateCombo(3),
UI_AnimateTooltip(4),
UI_FadeTooltip(5),
UI_AnimateToolBox(6);
private UIEffect(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static UIEffect resolve(int value) {
switch (value) {
case 0: return UI_General;
case 1: return UI_AnimateMenu;
case 2: return UI_FadeMenu;
case 3: return UI_AnimateCombo;
case 4: return UI_AnimateTooltip;
case 5: return UI_FadeTooltip;
case 6: return UI_AnimateToolBox;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::WhiteSpaceMode
*/
@io.qt.QtUnlistedEnum
public enum WhiteSpaceMode implements io.qt.QtEnumerator {
WhiteSpaceNormal(0),
WhiteSpacePre(1),
WhiteSpaceNoWrap(2),
WhiteSpaceModeUndefined(-1);
private WhiteSpaceMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WhiteSpaceMode resolve(int value) {
switch (value) {
case 0: return WhiteSpaceNormal;
case 1: return WhiteSpacePre;
case 2: return WhiteSpaceNoWrap;
case -1: return WhiteSpaceModeUndefined;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::WidgetAttribute
*/
public enum WidgetAttribute implements io.qt.QtEnumerator {
WA_Disabled(0),
WA_UnderMouse(1),
WA_MouseTracking(2),
WA_OpaquePaintEvent(4),
WA_StaticContents(5),
WA_LaidOut(7),
WA_PaintOnScreen(8),
WA_NoSystemBackground(9),
WA_UpdatesDisabled(10),
WA_Mapped(11),
WA_InputMethodEnabled(14),
WA_WState_Visible(15),
WA_WState_Hidden(16),
WA_ForceDisabled(32),
WA_KeyCompression(33),
WA_PendingMoveEvent(34),
WA_PendingResizeEvent(35),
WA_SetPalette(36),
WA_SetFont(37),
WA_SetCursor(38),
WA_NoChildEventsFromChildren(39),
WA_WindowModified(41),
WA_Resized(42),
WA_Moved(43),
WA_PendingUpdate(44),
WA_InvalidSize(45),
WA_CustomWhatsThis(47),
WA_LayoutOnEntireRect(48),
WA_OutsideWSRange(49),
WA_GrabbedShortcut(50),
WA_TransparentForMouseEvents(51),
WA_PaintUnclipped(52),
WA_SetWindowIcon(53),
WA_NoMouseReplay(54),
WA_DeleteOnClose(55),
WA_RightToLeft(56),
WA_SetLayoutDirection(57),
WA_NoChildEventsForParent(58),
WA_ForceUpdatesDisabled(59),
WA_WState_Created(60),
WA_WState_CompressKeys(61),
WA_WState_InPaintEvent(62),
WA_WState_Reparented(63),
WA_WState_ConfigPending(64),
WA_WState_Polished(66),
WA_WState_OwnSizePolicy(68),
WA_WState_ExplicitShowHide(69),
WA_ShowModal(70),
WA_MouseNoMask(71),
WA_NoMousePropagation(73),
WA_Hover(74),
WA_InputMethodTransparent(75),
WA_QuitOnClose(76),
WA_KeyboardFocusChange(77),
WA_AcceptDrops(78),
WA_DropSiteRegistered(79),
WA_WindowPropagation(80),
WA_NoX11EventCompression(81),
WA_TintedBackground(82),
WA_X11OpenGLOverlay(83),
WA_AlwaysShowToolTips(84),
WA_MacOpaqueSizeGrip(85),
WA_SetStyle(86),
WA_SetLocale(87),
WA_MacShowFocusRect(88),
WA_MacNormalSize(89),
WA_MacSmallSize(90),
WA_MacMiniSize(91),
WA_LayoutUsesWidgetRect(92),
WA_StyledBackground(93),
WA_CanHostQMdiSubWindowTitleBar(95),
WA_MacAlwaysShowToolWindow(96),
WA_StyleSheet(97),
WA_ShowWithoutActivating(98),
WA_X11BypassTransientForHint(99),
WA_NativeWindow(100),
WA_DontCreateNativeAncestors(101),
WA_DontShowOnScreen(103),
WA_X11NetWmWindowTypeDesktop(104),
WA_X11NetWmWindowTypeDock(105),
WA_X11NetWmWindowTypeToolBar(106),
WA_X11NetWmWindowTypeMenu(107),
WA_X11NetWmWindowTypeUtility(108),
WA_X11NetWmWindowTypeSplash(109),
WA_X11NetWmWindowTypeDialog(110),
WA_X11NetWmWindowTypeDropDownMenu(111),
WA_X11NetWmWindowTypePopupMenu(112),
WA_X11NetWmWindowTypeToolTip(113),
WA_X11NetWmWindowTypeNotification(114),
WA_X11NetWmWindowTypeCombo(115),
WA_X11NetWmWindowTypeDND(116),
WA_SetWindowModality(118),
WA_WState_WindowOpacitySet(119),
WA_TranslucentBackground(120),
WA_AcceptTouchEvents(121),
WA_WState_AcceptedTouchBeginEvent(122),
WA_TouchPadAcceptSingleTouchEvents(123),
WA_X11DoNotAcceptFocus(126),
WA_AlwaysStackOnTop(128),
WA_TabletTracking(129),
WA_ContentsMarginsRespectsSafeArea(130),
WA_StyleSheetTarget(131),
WA_AttributeCount(132);
private WidgetAttribute(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WidgetAttribute resolve(int value) {
switch (value) {
case 0: return WA_Disabled;
case 1: return WA_UnderMouse;
case 2: return WA_MouseTracking;
case 4: return WA_OpaquePaintEvent;
case 5: return WA_StaticContents;
case 7: return WA_LaidOut;
case 8: return WA_PaintOnScreen;
case 9: return WA_NoSystemBackground;
case 10: return WA_UpdatesDisabled;
case 11: return WA_Mapped;
case 14: return WA_InputMethodEnabled;
case 15: return WA_WState_Visible;
case 16: return WA_WState_Hidden;
case 32: return WA_ForceDisabled;
case 33: return WA_KeyCompression;
case 34: return WA_PendingMoveEvent;
case 35: return WA_PendingResizeEvent;
case 36: return WA_SetPalette;
case 37: return WA_SetFont;
case 38: return WA_SetCursor;
case 39: return WA_NoChildEventsFromChildren;
case 41: return WA_WindowModified;
case 42: return WA_Resized;
case 43: return WA_Moved;
case 44: return WA_PendingUpdate;
case 45: return WA_InvalidSize;
case 47: return WA_CustomWhatsThis;
case 48: return WA_LayoutOnEntireRect;
case 49: return WA_OutsideWSRange;
case 50: return WA_GrabbedShortcut;
case 51: return WA_TransparentForMouseEvents;
case 52: return WA_PaintUnclipped;
case 53: return WA_SetWindowIcon;
case 54: return WA_NoMouseReplay;
case 55: return WA_DeleteOnClose;
case 56: return WA_RightToLeft;
case 57: return WA_SetLayoutDirection;
case 58: return WA_NoChildEventsForParent;
case 59: return WA_ForceUpdatesDisabled;
case 60: return WA_WState_Created;
case 61: return WA_WState_CompressKeys;
case 62: return WA_WState_InPaintEvent;
case 63: return WA_WState_Reparented;
case 64: return WA_WState_ConfigPending;
case 66: return WA_WState_Polished;
case 68: return WA_WState_OwnSizePolicy;
case 69: return WA_WState_ExplicitShowHide;
case 70: return WA_ShowModal;
case 71: return WA_MouseNoMask;
case 73: return WA_NoMousePropagation;
case 74: return WA_Hover;
case 75: return WA_InputMethodTransparent;
case 76: return WA_QuitOnClose;
case 77: return WA_KeyboardFocusChange;
case 78: return WA_AcceptDrops;
case 79: return WA_DropSiteRegistered;
case 80: return WA_WindowPropagation;
case 81: return WA_NoX11EventCompression;
case 82: return WA_TintedBackground;
case 83: return WA_X11OpenGLOverlay;
case 84: return WA_AlwaysShowToolTips;
case 85: return WA_MacOpaqueSizeGrip;
case 86: return WA_SetStyle;
case 87: return WA_SetLocale;
case 88: return WA_MacShowFocusRect;
case 89: return WA_MacNormalSize;
case 90: return WA_MacSmallSize;
case 91: return WA_MacMiniSize;
case 92: return WA_LayoutUsesWidgetRect;
case 93: return WA_StyledBackground;
case 95: return WA_CanHostQMdiSubWindowTitleBar;
case 96: return WA_MacAlwaysShowToolWindow;
case 97: return WA_StyleSheet;
case 98: return WA_ShowWithoutActivating;
case 99: return WA_X11BypassTransientForHint;
case 100: return WA_NativeWindow;
case 101: return WA_DontCreateNativeAncestors;
case 103: return WA_DontShowOnScreen;
case 104: return WA_X11NetWmWindowTypeDesktop;
case 105: return WA_X11NetWmWindowTypeDock;
case 106: return WA_X11NetWmWindowTypeToolBar;
case 107: return WA_X11NetWmWindowTypeMenu;
case 108: return WA_X11NetWmWindowTypeUtility;
case 109: return WA_X11NetWmWindowTypeSplash;
case 110: return WA_X11NetWmWindowTypeDialog;
case 111: return WA_X11NetWmWindowTypeDropDownMenu;
case 112: return WA_X11NetWmWindowTypePopupMenu;
case 113: return WA_X11NetWmWindowTypeToolTip;
case 114: return WA_X11NetWmWindowTypeNotification;
case 115: return WA_X11NetWmWindowTypeCombo;
case 116: return WA_X11NetWmWindowTypeDND;
case 118: return WA_SetWindowModality;
case 119: return WA_WState_WindowOpacitySet;
case 120: return WA_TranslucentBackground;
case 121: return WA_AcceptTouchEvents;
case 122: return WA_WState_AcceptedTouchBeginEvent;
case 123: return WA_TouchPadAcceptSingleTouchEvents;
case 126: return WA_X11DoNotAcceptFocus;
case 128: return WA_AlwaysStackOnTop;
case 129: return WA_TabletTracking;
case 130: return WA_ContentsMarginsRespectsSafeArea;
case 131: return WA_StyleSheetTarget;
case 132: return WA_AttributeCount;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::WindowFrameSection
*/
@io.qt.QtUnlistedEnum
public enum WindowFrameSection implements io.qt.QtEnumerator {
NoSection(0),
LeftSection(1),
TopLeftSection(2),
TopSection(3),
TopRightSection(4),
RightSection(5),
BottomRightSection(6),
BottomSection(7),
BottomLeftSection(8),
TitleBarArea(9);
private WindowFrameSection(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WindowFrameSection resolve(int value) {
switch (value) {
case 0: return NoSection;
case 1: return LeftSection;
case 2: return TopLeftSection;
case 3: return TopSection;
case 4: return TopRightSection;
case 5: return RightSection;
case 6: return BottomRightSection;
case 7: return BottomSection;
case 8: return BottomLeftSection;
case 9: return TitleBarArea;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::WindowModality
*/
public enum WindowModality implements io.qt.QtEnumerator {
NonModal(0),
WindowModal(1),
ApplicationModal(2);
private WindowModality(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WindowModality resolve(int value) {
switch (value) {
case 0: return NonModal;
case 1: return WindowModal;
case 2: return ApplicationModal;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum Qt::WindowState
*
* @see WindowStates
*/
public enum WindowState implements io.qt.QtFlagEnumerator {
WindowNoState(0),
WindowMinimized(1),
WindowMaximized(2),
WindowFullScreen(4),
WindowActive(8);
private WindowState(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public WindowStates asFlags() {
return new WindowStates(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public WindowStates combined(WindowState e) {
return new WindowStates(this, e);
}
/**
* Creates a new {@link WindowStates} from the entries.
* @param values entries
* @return new flag
*/
public static WindowStates flags(WindowState ... values) {
return new WindowStates(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WindowState resolve(int value) {
switch (value) {
case 0: return WindowNoState;
case 1: return WindowMinimized;
case 2: return WindowMaximized;
case 4: return WindowFullScreen;
case 8: return WindowActive;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link WindowState}
*/
public static final class WindowStates extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x47389fc27c17f83cL;
/**
* Creates a new WindowStates where the flags in args
are set.
* @param args enum entries
*/
public WindowStates(WindowState ... args){
super(args);
}
/**
* Creates a new WindowStates with given value
.
* @param value
*/
public WindowStates(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new WindowStates
*/
@Override
public final WindowStates combined(WindowState e){
return new WindowStates(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final WindowStates setFlag(WindowState e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final WindowStates setFlag(WindowState e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this WindowStates.
* @return array of enum entries
*/
@Override
public final WindowState[] flags(){
return super.flags(WindowState.values());
}
/**
* {@inheritDoc}
*/
@Override
public final WindowStates clone(){
return new WindowStates(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(WindowStates other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum Qt::WindowType
*
* @see WindowFlags
*/
@io.qt.QtRejectedEntries({"X11BypassWindowManagerHint"})
public enum WindowType implements io.qt.QtFlagEnumerator {
Widget(0),
Window(1),
Dialog(3),
Sheet(5),
Drawer(7),
Popup(9),
Tool(11),
ToolTip(13),
SplashScreen(15),
Desktop(17),
SubWindow(18),
ForeignWindow(33),
CoverWindow(65),
WindowType_Mask(255),
MSWindowsFixedSizeDialogHint(256),
MSWindowsOwnDC(512),
BypassWindowManagerHint(1024),
X11BypassWindowManagerHint(1024),
FramelessWindowHint(2048),
WindowTitleHint(4096),
WindowSystemMenuHint(8192),
WindowMinimizeButtonHint(16384),
WindowMaximizeButtonHint(32768),
WindowMinMaxButtonsHint(49152),
WindowContextHelpButtonHint(65536),
WindowShadeButtonHint(131072),
WindowStaysOnTopHint(262144),
WindowTransparentForInput(524288),
WindowOverridesSystemGestures(1048576),
WindowDoesNotAcceptFocus(2097152),
MaximizeUsingFullscreenGeometryHint(4194304),
CustomizeWindowHint(33554432),
WindowStaysOnBottomHint(67108864),
WindowCloseButtonHint(134217728),
MacWindowToolBarButtonHint(268435456),
BypassGraphicsProxyWidget(536870912),
NoDropShadowWindowHint(1073741824),
WindowFullscreenButtonHint(-2147483648);
private WindowType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public WindowFlags asFlags() {
return new WindowFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public WindowFlags combined(WindowType e) {
return new WindowFlags(this, e);
}
/**
* Creates a new {@link WindowFlags} from the entries.
* @param values entries
* @return new flag
*/
public static WindowFlags flags(WindowType ... values) {
return new WindowFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WindowType resolve(int value) {
switch (value) {
case 0: return Widget;
case 1: return Window;
case 3: return Dialog;
case 5: return Sheet;
case 7: return Drawer;
case 9: return Popup;
case 11: return Tool;
case 13: return ToolTip;
case 15: return SplashScreen;
case 17: return Desktop;
case 18: return SubWindow;
case 33: return ForeignWindow;
case 65: return CoverWindow;
case 255: return WindowType_Mask;
case 256: return MSWindowsFixedSizeDialogHint;
case 512: return MSWindowsOwnDC;
case 1024: return BypassWindowManagerHint;
case 2048: return FramelessWindowHint;
case 4096: return WindowTitleHint;
case 8192: return WindowSystemMenuHint;
case 16384: return WindowMinimizeButtonHint;
case 32768: return WindowMaximizeButtonHint;
case 49152: return WindowMinMaxButtonsHint;
case 65536: return WindowContextHelpButtonHint;
case 131072: return WindowShadeButtonHint;
case 262144: return WindowStaysOnTopHint;
case 524288: return WindowTransparentForInput;
case 1048576: return WindowOverridesSystemGestures;
case 2097152: return WindowDoesNotAcceptFocus;
case 4194304: return MaximizeUsingFullscreenGeometryHint;
case 33554432: return CustomizeWindowHint;
case 67108864: return WindowStaysOnBottomHint;
case 134217728: return WindowCloseButtonHint;
case 268435456: return MacWindowToolBarButtonHint;
case 536870912: return BypassGraphicsProxyWidget;
case 1073741824: return NoDropShadowWindowHint;
case -2147483648: return WindowFullscreenButtonHint;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link WindowType}
*/
public static final class WindowFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x4b60224f25ec75acL;
/**
* Creates a new WindowFlags where the flags in args
are set.
* @param args enum entries
*/
public WindowFlags(WindowType ... args){
super(args);
}
/**
* Creates a new WindowFlags with given value
.
* @param value
*/
public WindowFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new WindowFlags
*/
@Override
public final WindowFlags combined(WindowType e){
return new WindowFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final WindowFlags setFlag(WindowType e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final WindowFlags setFlag(WindowType e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this WindowFlags.
* @return array of enum entries
*/
@Override
public final WindowType[] flags(){
return super.flags(WindowType.values());
}
/**
* {@inheritDoc}
*/
@Override
public final WindowFlags clone(){
return new WindowFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(WindowFlags other){
return Integer.compare(value(), other.value());
}
}
public native static void beginPropertyUpdateGroup();
public static io.qt.core.QTextStream bin(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return bin_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream bin_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream bom(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return bom_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream bom_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream center(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return center_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream center_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream dec(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return dec_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream dec_native_ref_QTextStream(long s);
public native static void endPropertyUpdateGroup();
public static io.qt.core.QTextStream endl(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return endl_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream endl_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream fixed(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return fixed_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream fixed_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream flush(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return flush_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream flush_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream forcepoint(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return forcepoint_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream forcepoint_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream forcesign(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return forcesign_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream forcesign_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream hex(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return hex_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream hex_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream left(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return left_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream left_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream lowercasebase(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return lowercasebase_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream lowercasebase_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream lowercasedigits(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return lowercasedigits_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream lowercasedigits_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream noforcepoint(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return noforcepoint_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream noforcepoint_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream noforcesign(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return noforcesign_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream noforcesign_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream noshowbase(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return noshowbase_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream noshowbase_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream oct(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return oct_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream oct_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream reset(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return reset_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream reset_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream right(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return right_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream right_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream scientific(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return scientific_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream scientific_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream showbase(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return showbase_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream showbase_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream uppercasebase(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return uppercasebase_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream uppercasebase_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream uppercasedigits(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return uppercasedigits_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream uppercasedigits_native_ref_QTextStream(long s);
public static io.qt.core.QTextStream ws(io.qt.core.QTextStream s){
java.util.Objects.requireNonNull(s, "Argument 's': null not expected.");
return ws_native_ref_QTextStream(QtJambi_LibraryUtilities.internal.checkedNativeId(s));
}
private native static io.qt.core.QTextStream ws_native_ref_QTextStream(long s);
public final static QMetaObject staticMetaObject = QMetaObject.forType(Qt.class);
}