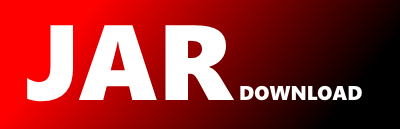
io.qt.gui.QTextItem Maven / Gradle / Ivy
package io.qt.gui;
/**
* All the information required to draw text in a custom paint engine
* Java wrapper for Qt class QTextItem
*/
public class QTextItem extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QTextItem::RenderFlag
*
* @see RenderFlags
*/
public enum RenderFlag implements io.qt.QtFlagEnumerator {
RightToLeft(1),
Overline(16),
Underline(32),
StrikeOut(64),
Dummy(-1);
private RenderFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public RenderFlags asFlags() {
return new RenderFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public RenderFlags combined(RenderFlag e) {
return new RenderFlags(this, e);
}
/**
* Creates a new {@link RenderFlags} from the entries.
* @param values entries
* @return new flag
*/
public static RenderFlags flags(RenderFlag ... values) {
return new RenderFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static RenderFlag resolve(int value) {
switch (value) {
case 1: return RightToLeft;
case 16: return Overline;
case 32: return Underline;
case 64: return StrikeOut;
case -1: return Dummy;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link RenderFlag}
*/
public static final class RenderFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xc5275907bfed457bL;
/**
* Creates a new RenderFlags where the flags in args
are set.
* @param args enum entries
*/
public RenderFlags(RenderFlag ... args){
super(args);
}
/**
* Creates a new RenderFlags with given value
.
* @param value
*/
public RenderFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new RenderFlags
*/
@Override
public final RenderFlags combined(RenderFlag e){
return new RenderFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final RenderFlags setFlag(RenderFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final RenderFlags setFlag(RenderFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this RenderFlags.
* @return array of enum entries
*/
@Override
public final RenderFlag[] flags(){
return super.flags(RenderFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final RenderFlags clone(){
return new RenderFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(RenderFlags other){
return Integer.compare(value(), other.value());
}
}
public QTextItem(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QTextItem instance);
/**
*
*/
@io.qt.QtUninvokable
public final double ascent(){
return ascent_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double ascent_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final double descent(){
return descent_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double descent_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.gui.QFont font(){
return font_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.gui.QFont font_native_constfct(long __this__nativeId);
/**
* See QTextItem::renderFlags()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QTextItem.RenderFlags renderFlags(){
return new io.qt.gui.QTextItem.RenderFlags(renderFlags_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int renderFlags_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final java.lang.String text(){
return text_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String text_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final double width(){
return width_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double width_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QTextItem(QPrivateConstructor p) { super(p); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy