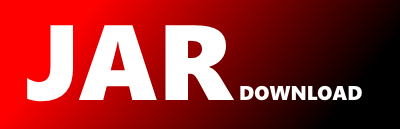
io.qt.core.QDeclarableSignals Maven / Gradle / Ivy
The newest version!
/****************************************************************************
**
** Copyright (C) 2009-2022 Dr. Peter Droste, Omix Visualization GmbH & Co. KG. All rights reserved.
**
** This file is part of Qt Jambi.
**
** ** $BEGIN_LICENSE$
** GNU Lesser General Public License Usage
** This file may be used under the terms of the GNU Lesser
** General Public License version 2.1 as published by the Free Software
** Foundation and appearing in the file LICENSE.LGPL included in the
** packaging of this file. Please review the following information to
** ensure the GNU Lesser General Public License version 2.1 requirements
** will be met: http://www.gnu.org/licenses/old-licenses/lgpl-2.1.html.
**
** GNU General Public License Usage
** Alternatively, this file may be used under the terms of the GNU
** General Public License version 3.0 as published by the Free Software
** Foundation and appearing in the file LICENSE.GPL included in the
** packaging of this file. Please review the following information to
** ensure the GNU General Public License version 3.0 requirements will be
** met: http://www.gnu.org/copyleft/gpl.html.
** $END_LICENSE$
**
** This file is provided AS IS with NO WARRANTY OF ANY KIND, INCLUDING THE
** WARRANTY OF DESIGN, MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE.
**
****************************************************************************/
package io.qt.core;
import java.util.Arrays;
import java.util.function.Consumer;
import java.util.function.Supplier;
import io.qt.QNoDefaultValueException;
import io.qt.QtUninvokable;
import io.qt.core.QMetaObject.*;
public final class QDeclarableSignals {
private QDeclarableSignals() {}
/**
* Use this signal class to declare a local signal.
*/
public static final class Signal0 extends QMetaObject.AbstractPublicSignal0 {
public Signal0() {
super(new Class>[0]);
}
public Signal0(String signalName) {
super(signalName, new Class>[0]);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
*/
public static final class Signal1 extends QMetaObject.AbstractPublicSignal1 {
public Signal1(Class typeA) {
super(typeA);
}
public Signal1(String signalName, Class typeA) {
super(signalName, typeA);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal2 extends QMetaObject.AbstractPublicSignal2 {
public Signal2(Class typeA, Class typeB) {
super(typeA, typeB);
}
public Signal2(String signalName, Class typeA, Class typeB) {
super(signalName, typeA, typeB);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal3 extends QMetaObject.AbstractPublicSignal3 {
public Signal3(Class typeA, Class typeB, Class typeC) {
super(typeA, typeB, typeC);
}
public Signal3(String signalName, Class typeA, Class typeB, Class typeC) {
super(signalName, typeA, typeB, typeC);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal4 extends QMetaObject.AbstractPublicSignal4 {
public Signal4(Class typeA, Class typeB, Class typeC, Class typeD) {
super(typeA, typeB, typeC, typeD);
}
public Signal4(String signalName, Class typeA, Class typeB, Class typeC, Class typeD) {
super(signalName, typeA, typeB, typeC, typeD);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal5 extends QMetaObject.AbstractPublicSignal5 {
public Signal5(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE) {
super(typeA, typeB, typeC, typeD, typeE);
}
public Signal5(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Class typeE) {
super(signalName, typeA, typeB, typeC, typeD, typeE);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal6 extends QMetaObject.AbstractPublicSignal6 {
public Signal6(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF) {
super(typeA, typeB, typeC, typeD, typeE, typeF);
}
public Signal6(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF) {
super(signalName, typeA, typeB, typeC, typeD, typeE, typeF);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal7 extends QMetaObject.AbstractPublicSignal7 {
public Signal7(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF, Class typeG) {
super(typeA, typeB, typeC, typeD, typeE, typeF, typeG);
}
public Signal7(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF, Class typeG) {
super(signalName, typeA, typeB, typeC, typeD, typeE, typeF, typeG);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal8 extends QMetaObject.AbstractPublicSignal8 {
public Signal8(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF, Class typeG, Class typeH) {
super(typeA, typeB, typeC, typeD, typeE, typeF, typeG, typeH);
}
public Signal8(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF, Class typeG, Class typeH) {
super(signalName, typeA, typeB, typeC, typeD, typeE, typeF, typeG, typeH);
}
}
/**
* Use this signal class to declare a local signal.
*
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
* @param The type of the single parameter of the signal.
*/
public static final class Signal9 extends QMetaObject.AbstractPublicSignal9 {
public Signal9(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF, Class typeG, Class typeH, Class typeI) {
super(typeA, typeB, typeC, typeD, typeE, typeF, typeG, typeH, typeI);
}
public Signal9(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Class typeF, Class typeG, Class typeH, Class typeI) {
super(signalName, typeA, typeB, typeC, typeD, typeE, typeF, typeG, typeH, typeI);
}
}
public static final class Signal1Default1 extends QMetaObject.AbstractPublicSignal1 implements QMetaObject.Emitable0, QMetaObject.Connectable0 {
public Signal1Default1(Class typeA, Supplier arg1Default){
super(typeA);
if(arg1Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
public Signal1Default1(String signalName, Class typeA, Supplier arg1Default){
super(signalName, typeA);
if(arg1Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
private final Supplier arg1Default;
@Override public final void emit() {
emitDefaultSignal(arg1Default);
}
}
public static final class Signal2Default1 extends QMetaObject.AbstractSignal2Default1{
public Signal2Default1(Class typeA, Class typeB, Supplier arg2Default) {
super(arg2Default, typeA, typeB);
}
public Signal2Default1(String signalName, Class typeA, Class typeB, Supplier arg2Default) {
super(signalName, arg2Default, typeA, typeB);
}
}
public static final class Signal2Default2 extends QMetaObject.AbstractSignal2Default1 implements QMetaObject.Emitable0, QMetaObject.Connectable0 {
public Signal2Default2(Class typeA, Class typeB, Supplier arg1Default, Supplier arg2Default){
super(arg2Default, typeA, typeB);
if(arg1Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
public Signal2Default2(String signalName, Class typeA, Class typeB, Supplier arg1Default, Supplier arg2Default){
super(signalName, arg2Default, typeA, typeB);
if(arg1Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
private final Supplier arg1Default;
@Override public final void emit() {
emitDefaultSignal(arg1Default);
}
}
public static final class Signal3Default1 extends QMetaObject.AbstractSignal3Default1 {
public Signal3Default1(String signalName, Class typeA, Class typeB, Class typeC, Supplier arg3Default) {
super(signalName, arg3Default, typeA, typeB, typeC);
}
public Signal3Default1(Class typeA, Class typeB, Class typeC, Supplier arg3Default) {
super(arg3Default, typeA, typeB, typeC);
}
}
public static final class Signal3Default2 extends QMetaObject.AbstractSignal3Default2 {
public Signal3Default2(String signalName, Class typeA, Class typeB, Class typeC, Supplier arg2Default, Supplier arg3Default){
super(signalName, arg2Default, arg3Default, typeA, typeB, typeC);
}
public Signal3Default2(Class typeA, Class typeB, Class typeC, Supplier arg2Default, Supplier arg3Default){
super(arg2Default, arg3Default, typeA, typeB, typeC);
}
}
public static final class Signal3Default3 extends QMetaObject.AbstractSignal3Default2 implements QMetaObject.Emitable0, QMetaObject.Connectable0 {
public Signal3Default3(String signalName, Class typeA, Class typeB, Class typeC, Supplier arg1Default, Supplier arg2Default, Supplier arg3Default){
super(signalName, arg2Default, arg3Default, typeA, typeB, typeC);
if(arg2Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
public Signal3Default3(Class typeA, Class typeB, Class typeC, Supplier arg1Default, Supplier arg2Default, Supplier arg3Default){
super(arg2Default, arg3Default, typeA, typeB, typeC);
if(arg2Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
private final Supplier arg1Default;
@Override public final void emit() {
emitDefaultSignal(arg1Default);
}
}
public static final class Signal4Default1 extends QMetaObject.AbstractSignal4Default1{
public Signal4Default1(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Supplier arg4Default){
super(signalName, arg4Default, typeA, typeB, typeC, typeD);
}
public Signal4Default1(Class typeA, Class typeB, Class typeC, Class typeD, Supplier arg4Default){
super(arg4Default, typeA, typeB, typeC, typeD);
}
}
public static final class Signal4Default2 extends QMetaObject.AbstractSignal4Default2{
public Signal4Default2(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Supplier arg3Default, Supplier arg4Default){
super(signalName, arg3Default, arg4Default, typeA, typeB, typeC, typeD);
}
public Signal4Default2(Class typeA, Class typeB, Class typeC, Class typeD, Supplier arg3Default, Supplier arg4Default){
super(arg3Default, arg4Default, typeA, typeB, typeC, typeD);
}
}
public static final class Signal4Default3 extends QMetaObject.AbstractSignal4Default3{
public Signal4Default3(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Supplier arg2Default, Supplier arg3Default, Supplier arg4Default){
super(signalName, arg2Default, arg3Default, arg4Default, typeA, typeB, typeC, typeD);
}
public Signal4Default3(Class typeA, Class typeB, Class typeC, Class typeD, Supplier arg2Default, Supplier arg3Default, Supplier arg4Default){
super(arg2Default, arg3Default, arg4Default, typeA, typeB, typeC, typeD);
}
}
public static final class Signal4Default4 extends QMetaObject.AbstractSignal4Default3 implements QMetaObject.Emitable0, QMetaObject.Connectable0 {
public Signal4Default4(String signalName, Class typeA, Class typeB, Class typeC, Class typeD,
Supplier arg1Default, Supplier arg2Default,
Supplier arg3Default, Supplier arg4Default){
super(signalName, arg2Default, arg3Default, arg4Default, typeA, typeB, typeC, typeD);
if(arg1Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
public Signal4Default4(Class typeA, Class typeB, Class typeC, Class typeD,
Supplier arg1Default, Supplier arg2Default,
Supplier arg3Default, Supplier arg4Default){
super(arg2Default, arg3Default, arg4Default, typeA, typeB, typeC, typeD);
if(arg1Default!=null){
this.arg1Default = arg1Default;
}else{
throw new QNoDefaultValueException(1);
}
}
private final Supplier arg1Default;
@Override public final void emit() {
emitDefaultSignal(arg1Default);
}
}
public static final class Signal5Default1 extends QMetaObject.AbstractSignal5Default1{
public Signal5Default1(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Supplier arg5Default){
super(arg5Default, typeA, typeB, typeC, typeD, typeE);
}
public Signal5Default1(String signalName, Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Supplier arg5Default){
super(signalName, arg5Default, typeA, typeB, typeC, typeD, typeE);
}
}
public static final class Signal5Default2 extends QMetaObject.AbstractSignal5Default2{
public Signal5Default2(Class typeA, Class typeB, Class typeC, Class typeD, Class typeE, Supplier arg4Default, Supplier arg5Default){
super(arg4Default, arg5Default, typeA, typeB, typeC, typeD, typeE);
}
public Signal5Default2(String signalName, Class typeA, Class typeB, Class typeC, Class