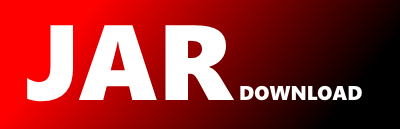
io.qt.core.QFileDevice Maven / Gradle / Ivy
package io.qt.core;
/**
* Interface for reading from and writing to open files
* Java wrapper for Qt class QFileDevice
*/
public class QFileDevice extends io.qt.core.QIODevice
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QFileDevice.class);
/**
* Java wrapper for Qt enum QFileDevice::FileError
*/
@io.qt.QtUnlistedEnum
public enum FileError implements io.qt.QtEnumerator {
NoError(0),
ReadError(1),
WriteError(2),
FatalError(3),
ResourceError(4),
OpenError(5),
AbortError(6),
TimeOutError(7),
UnspecifiedError(8),
RemoveError(9),
RenameError(10),
PositionError(11),
ResizeError(12),
PermissionsError(13),
CopyError(14);
private FileError(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FileError resolve(int value) {
switch (value) {
case 0: return NoError;
case 1: return ReadError;
case 2: return WriteError;
case 3: return FatalError;
case 4: return ResourceError;
case 5: return OpenError;
case 6: return AbortError;
case 7: return TimeOutError;
case 8: return UnspecifiedError;
case 9: return RemoveError;
case 10: return RenameError;
case 11: return PositionError;
case 12: return ResizeError;
case 13: return PermissionsError;
case 14: return CopyError;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QFileDevice::FileHandleFlag
*
* @see FileHandleFlags
*/
public enum FileHandleFlag implements io.qt.QtFlagEnumerator {
AutoCloseHandle(1),
DontCloseHandle(0);
private FileHandleFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public FileHandleFlags asFlags() {
return new FileHandleFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public FileHandleFlags combined(FileHandleFlag e) {
return new FileHandleFlags(this, e);
}
/**
* Creates a new {@link FileHandleFlags} from the entries.
* @param values entries
* @return new flag
*/
public static FileHandleFlags flags(FileHandleFlag ... values) {
return new FileHandleFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FileHandleFlag resolve(int value) {
switch (value) {
case 1: return AutoCloseHandle;
case 0: return DontCloseHandle;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link FileHandleFlag}
*/
public static final class FileHandleFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x16a492cebc911dceL;
/**
* Creates a new FileHandleFlags where the flags in args
are set.
* @param args enum entries
*/
public FileHandleFlags(FileHandleFlag ... args){
super(args);
}
/**
* Creates a new FileHandleFlags with given value
.
* @param value
*/
public FileHandleFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new FileHandleFlags
*/
@Override
public final FileHandleFlags combined(FileHandleFlag e){
return new FileHandleFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final FileHandleFlags setFlag(FileHandleFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final FileHandleFlags setFlag(FileHandleFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this FileHandleFlags.
* @return array of enum entries
*/
@Override
public final FileHandleFlag[] flags(){
return super.flags(FileHandleFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final FileHandleFlags clone(){
return new FileHandleFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(FileHandleFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum QFileDevice::FileTime
*/
@io.qt.QtUnlistedEnum
public enum FileTime implements io.qt.QtEnumerator {
FileAccessTime(0),
FileBirthTime(1),
FileMetadataChangeTime(2),
FileModificationTime(3);
private FileTime(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static FileTime resolve(int value) {
switch (value) {
case 0: return FileAccessTime;
case 1: return FileBirthTime;
case 2: return FileMetadataChangeTime;
case 3: return FileModificationTime;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QFileDevice::MemoryMapFlag
*
* @see MemoryMapFlags
*/
public enum MemoryMapFlag implements io.qt.QtFlagEnumerator {
NoOptions(0),
MapPrivateOption(1);
private MemoryMapFlag(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public MemoryMapFlags asFlags() {
return new MemoryMapFlags(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public MemoryMapFlags combined(MemoryMapFlag e) {
return new MemoryMapFlags(this, e);
}
/**
* Creates a new {@link MemoryMapFlags} from the entries.
* @param values entries
* @return new flag
*/
public static MemoryMapFlags flags(MemoryMapFlag ... values) {
return new MemoryMapFlags(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static MemoryMapFlag resolve(int value) {
switch (value) {
case 0: return NoOptions;
case 1: return MapPrivateOption;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link MemoryMapFlag}
*/
public static final class MemoryMapFlags extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xbc441f9cb881eefbL;
/**
* Creates a new MemoryMapFlags where the flags in args
are set.
* @param args enum entries
*/
public MemoryMapFlags(MemoryMapFlag ... args){
super(args);
}
/**
* Creates a new MemoryMapFlags with given value
.
* @param value
*/
public MemoryMapFlags(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new MemoryMapFlags
*/
@Override
public final MemoryMapFlags combined(MemoryMapFlag e){
return new MemoryMapFlags(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final MemoryMapFlags setFlag(MemoryMapFlag e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final MemoryMapFlags setFlag(MemoryMapFlag e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this MemoryMapFlags.
* @return array of enum entries
*/
@Override
public final MemoryMapFlag[] flags(){
return super.flags(MemoryMapFlag.values());
}
/**
* {@inheritDoc}
*/
@Override
public final MemoryMapFlags clone(){
return new MemoryMapFlags(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(MemoryMapFlags other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum QFileDevice::Permission
*
* @see Permissions
*/
public enum Permission implements io.qt.QtFlagEnumerator {
ReadOwner(16384),
WriteOwner(8192),
ExeOwner(4096),
ReadUser(1024),
WriteUser(512),
ExeUser(256),
ReadGroup(64),
WriteGroup(32),
ExeGroup(16),
ReadOther(4),
WriteOther(2),
ExeOther(1);
private Permission(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public Permissions asFlags() {
return new Permissions(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public Permissions combined(Permission e) {
return new Permissions(this, e);
}
/**
* Creates a new {@link Permissions} from the entries.
* @param values entries
* @return new flag
*/
public static Permissions flags(Permission ... values) {
return new Permissions(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Permission resolve(int value) {
switch (value) {
case 16384: return ReadOwner;
case 8192: return WriteOwner;
case 4096: return ExeOwner;
case 1024: return ReadUser;
case 512: return WriteUser;
case 256: return ExeUser;
case 64: return ReadGroup;
case 32: return WriteGroup;
case 16: return ExeGroup;
case 4: return ReadOther;
case 2: return WriteOther;
case 1: return ExeOther;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link Permission}
*/
public static final class Permissions extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xd4f58fe6ba34a0ecL;
/**
* Creates a new Permissions where the flags in args
are set.
* @param args enum entries
*/
public Permissions(Permission ... args){
super(args);
}
/**
* Creates a new Permissions with given value
.
* @param value
*/
public Permissions(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new Permissions
*/
@Override
public final Permissions combined(Permission e){
return new Permissions(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final Permissions setFlag(Permission e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final Permissions setFlag(Permission e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this Permissions.
* @return array of enum entries
*/
@Override
public final Permission[] flags(){
return super.flags(Permission.values());
}
/**
* {@inheritDoc}
*/
@Override
public final Permissions clone(){
return new Permissions(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(Permissions other){
return Integer.compare(value(), other.value());
}
}
protected QFileDevice(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QFileDevice instance);
protected QFileDevice(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QFileDevice instance, io.qt.core.QObject parent);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.core.QFileDevice.FileError error(){
return io.qt.core.QFileDevice.FileError.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QFileDevice::fileTime(QFileDevice::FileTime)const
*/
@io.qt.QtUninvokable
public final io.qt.core.QDateTime fileTime(io.qt.core.QFileDevice.FileTime time){
return fileTime_native_QFileDevice_FileTime_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), time.value());
}
@io.qt.QtUninvokable
private native io.qt.core.QDateTime fileTime_native_QFileDevice_FileTime_constfct(long __this__nativeId, int time);
/**
*
*/
@io.qt.QtUninvokable
public final boolean flush(){
return flush_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean flush_native(long __this__nativeId);
/**
* See QFileDevice::handle()const
*/
@io.qt.QtUninvokable
public final int handle(){
return handle_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int handle_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #map(long, long, io.qt.core.QFileDevice.MemoryMapFlags)}.
*/
@io.qt.QtUninvokable
public final java.nio.ByteBuffer map(long offset, long size, io.qt.core.QFileDevice.MemoryMapFlag ... flags){
return map(offset, size, new io.qt.core.QFileDevice.MemoryMapFlags(flags));
}
/**
* Overloaded function for {@link #map(long, long, io.qt.core.QFileDevice.MemoryMapFlags)}
* with flags = new io.qt.core.QFileDevice.MemoryMapFlags(0)
.
*/
@io.qt.QtUninvokable
public final java.nio.ByteBuffer map(long offset, long size) {
return map(offset, size, new io.qt.core.QFileDevice.MemoryMapFlags(0));
}
/**
* See QFileDevice::map(qint64,qint64,MemoryMapFlags)
*/
@io.qt.QtUninvokable
public final java.nio.ByteBuffer map(long offset, long size, io.qt.core.QFileDevice.MemoryMapFlags flags){
return map_native_long_long_long_long_QFlags_QFileDevice_MemoryMapFlag_(QtJambi_LibraryUtilities.internal.nativeId(this), offset, size, flags.value());
}
@io.qt.QtUninvokable
private native java.nio.ByteBuffer map_native_long_long_long_long_QFlags_QFileDevice_MemoryMapFlag_(long __this__nativeId, long offset, long size, int flags);
/**
* See QFileDevice::setFileTime(QDateTime,QFileDevice::FileTime)
*/
@io.qt.QtUninvokable
public final boolean setFileTime(io.qt.core.QDateTime newDate, io.qt.core.QFileDevice.FileTime fileTime){
return setFileTime_native_cref_QDateTime_QFileDevice_FileTime(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(newDate), fileTime.value());
}
@io.qt.QtUninvokable
private native boolean setFileTime_native_cref_QDateTime_QFileDevice_FileTime(long __this__nativeId, long newDate, int fileTime);
/**
* See QFileDevice::unmap(uchar*)
*/
@io.qt.QtUninvokable
public final boolean unmap(java.nio.ByteBuffer address){
return unmap_native_unsigned_char_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), address);
}
@io.qt.QtUninvokable
private native boolean unmap_native_unsigned_char_ptr(long __this__nativeId, java.nio.ByteBuffer address);
/**
*
*/
@io.qt.QtUninvokable
public final void unsetError(){
unsetError_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void unsetError_native(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public boolean atEnd(){
return atEnd_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean atEnd_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public void close(){
close_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void close_native(long __this__nativeId);
/**
* See QFileDevice::fileName()const
*/
@io.qt.QtUninvokable
public java.lang.String fileName(){
return fileName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String fileName_native_constfct(long __this__nativeId);
/**
* See QIODevice::isSequential()const
*/
@io.qt.QtUninvokable
public boolean isSequential(){
return isSequential_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isSequential_native_constfct(long __this__nativeId);
/**
* See QFileDevice::permissions()const
*/
@io.qt.QtUninvokable
public io.qt.core.QFileDevice.Permissions permissions(){
return new io.qt.core.QFileDevice.Permissions(permissions_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int permissions_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public long pos(){
return pos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long pos_native_constfct(long __this__nativeId);
/**
* See QIODevice::readData(char*,qint64)
*/
@io.qt.QtUninvokable
protected int readData(byte[] data){
return readData_native_char_ptr_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), data);
}
@io.qt.QtUninvokable
private native int readData_native_char_ptr_long_long(long __this__nativeId, byte[] data);
/**
* See QIODevice::readLineData(char*,qint64)
*/
@io.qt.QtUninvokable
protected int readLineData(byte[] data){
return readLineData_native_char_ptr_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), data);
}
@io.qt.QtUninvokable
private native int readLineData_native_char_ptr_long_long(long __this__nativeId, byte[] data);
/**
* See QFileDevice::resize(qint64)
*/
@io.qt.QtUninvokable
public boolean resize(long sz){
return resize_native_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), sz);
}
@io.qt.QtUninvokable
private native boolean resize_native_long_long(long __this__nativeId, long sz);
/**
*
*/
@io.qt.QtUninvokable
public boolean seek(long offset){
return seek_native_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), offset);
}
@io.qt.QtUninvokable
private native boolean seek_native_long_long(long __this__nativeId, long offset);
/**
* Overloaded function for {@link #setPermissions(io.qt.core.QFileDevice.Permissions)}.
*/
@io.qt.QtUninvokable
public final boolean setPermissions(io.qt.core.QFileDevice.Permission ... permissionSpec){
return setPermissions(new io.qt.core.QFileDevice.Permissions(permissionSpec));
}
/**
* See QFileDevice::setPermissions(Permissions)
*/
@io.qt.QtUninvokable
public boolean setPermissions(io.qt.core.QFileDevice.Permissions permissionSpec){
return setPermissions_native_QFlags_QFileDevice_Permission_(QtJambi_LibraryUtilities.internal.nativeId(this), permissionSpec.value());
}
@io.qt.QtUninvokable
private native boolean setPermissions_native_QFlags_QFileDevice_Permission_(long __this__nativeId, int permissionSpec);
/**
*
*/
@io.qt.QtUninvokable
public long size(){
return size_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long size_native_constfct(long __this__nativeId);
/**
* See QIODevice::writeData(const char*,qint64)
*/
@io.qt.QtUninvokable
protected int writeData(byte[] data){
return writeData_native_const_char_ptr_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), data);
}
@io.qt.QtUninvokable
private native int writeData_native_const_char_ptr_long_long(long __this__nativeId, byte[] data);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QFileDevice(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QFileDevice(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QFileDevice instance, QDeclarativeConstructor constructor);
}