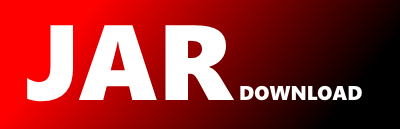
io.qt.core.QPointF Maven / Gradle / Ivy
The newest version!
package io.qt.core;
/**
* Defines a point in the plane using floating point precision
* Java wrapper for Qt class QPointF
*/
public class QPointF extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
*
*/
public QPointF(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QPointF instance);
/**
*
*/
public QPointF(io.qt.core.QPoint p){
super((QPrivateConstructor)null);
initialize_native(this, p);
}
private native static void initialize_native(QPointF instance, io.qt.core.QPoint p);
/**
* See QPointF::QPointF(qreal,qreal)
*/
public QPointF(double xpos, double ypos){
super((QPrivateConstructor)null);
initialize_native(this, xpos, ypos);
}
private native static void initialize_native(QPointF instance, double xpos, double ypos);
/**
*
*/
@io.qt.QtUninvokable
public final boolean isNull(){
return isNull_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isNull_native_constfct(long __this__nativeId);
/**
* See QPointF::manhattanLength()const
*/
@io.qt.QtUninvokable
public final double manhattanLength(){
return manhattanLength_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double manhattanLength_native_constfct(long __this__nativeId);
/**
* See QPointF::operator*=(qreal)
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF multiply(double c){
multiply_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), c);
return this;
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF multiply_native_qtjambireal(long __this__nativeId, double c);
/**
* See QPointF::operator+=(QPointF)
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF add(io.qt.core.QPointF p){
add_native_cref_QPointF(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(p));
return this;
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF add_native_cref_QPointF(long __this__nativeId, long p);
/**
* See QPointF::operator-=(QPointF)
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF subtract(io.qt.core.QPointF p){
subtract_native_cref_QPointF(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(p));
return this;
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF subtract_native_cref_QPointF(long __this__nativeId, long p);
/**
* See QPointF::operator/=(qreal)
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF divide(double c){
divide_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), c);
return this;
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF divide_native_qtjambireal(long __this__nativeId, double c);
@io.qt.QtUninvokable
public void writeTo(io.qt.core.QDataStream arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
writeTo_native_ref_QDataStream(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void writeTo_native_ref_QDataStream(long __this__nativeId, long arg__1);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.core.QPointF p2){
return operator_equal_native_cref_QPointF(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(p2));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QPointF(long __this__nativeId, long p2);
@io.qt.QtUninvokable
public void readFrom(io.qt.core.QDataStream arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
readFrom_native_ref_QDataStream(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void readFrom_native_ref_QDataStream(long __this__nativeId, long arg__1);
/**
*
*/
@io.qt.QtUninvokable
public final void setX(double x){
setX_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), x);
}
@io.qt.QtUninvokable
private native void setX_native_qtjambireal(long __this__nativeId, double x);
/**
*
*/
@io.qt.QtUninvokable
public final void setY(double y){
setY_native_qtjambireal(QtJambi_LibraryUtilities.internal.nativeId(this), y);
}
@io.qt.QtUninvokable
private native void setY_native_qtjambireal(long __this__nativeId, double y);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.core.QPoint toPoint(){
return toPoint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QPoint toPoint_native_constfct(long __this__nativeId);
/**
* See QPointF::transposed()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QPointF transposed(){
return transposed_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QPointF transposed_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final double x(){
return x_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double x_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final double y(){
return y_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double y_native_constfct(long __this__nativeId);
/**
* See QPointF::dotProduct(QPointF,QPointF)
*/
public static double dotProduct(io.qt.core.QPointF p1, io.qt.core.QPointF p2){
return dotProduct_native_cref_QPointF_cref_QPointF(QtJambi_LibraryUtilities.internal.checkedNativeId(p1), QtJambi_LibraryUtilities.internal.checkedNativeId(p2));
}
private native static double dotProduct_native_cref_QPointF_cref_QPointF(long p1, long p2);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QPointF(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.core.QPointF) {
return operator_equal((io.qt.core.QPointF) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
@io.qt.QtUninvokable
public String toString() {
return toString_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private static native String toString_native(long __this_nativeId);
@Override
public QPointF clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QPointF clone_native(long __this_nativeId);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy