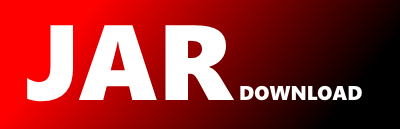
io.qt.core.QPromise Maven / Gradle / Ivy
The newest version!
package io.qt.core;
import java.util.Objects;
import io.qt.NativeAccess;
import io.qt.QNoImplementationException;
import io.qt.QtObject;
/**
* Way to store computation results to be accessed by QFuture
* Java wrapper for Qt class QPromise
*/
public final class QPromise
{
static {
QtJambi_LibraryUtilities.initialize();
}
private static class NativeInstance extends QtObject{
@NativeAccess
private NativeInstance(QPrivateConstructor p) {
super(p);
}
@NativeAccess
private QPromise> promise;
}
private final io.qt.core.QFutureInterfaceBase d;
@NativeAccess
private NativeInstance nativeInstance;
public static QPromise createVoidPromise() {
return new QPromise<>(true);
}
/**
*
*/
public QPromise(){
super();
d = new QFutureInterface<>();
registerPromise(this);
}
private void registerPromise(QPromise> promise) {
QFutureInterfaceBase d = promise.d;
QtJambi_LibraryUtilities.internal.registerCleaner(promise, ()->{if(!d.isDisposed()){
d.reportFinished();
}});
}
private QPromise(boolean isVoid){
super();
d = QFutureInterface.createVoidFutureInterface();
registerPromise(this);
}
public QPromise(io.qt.core.QFutureInterface other){
d = other.clone();
registerPromise(this);
}
@NativeAccess
private QPromise(io.qt.core.QFutureInterfaceBase other, boolean internal){
d = Objects.requireNonNull(other);
}
/**
*
*/
@io.qt.QtUninvokable
public final void finish(){
d.reportFinished();
}
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.core.QFuture future(){
return new QFuture<>(d.clone(), true);
}
/**
* See QPromise::isCanceled()const
*/
@io.qt.QtUninvokable
public final boolean isCanceled(){
return d.isCanceled();
}
/**
* See QPromise::setProgressRange(int,int)
*/
@io.qt.QtUninvokable
public final void setProgressRange(int minimum, int maximum){
d.setProgressRange(minimum, maximum);
}
/**
* See QPromise::setProgressValue(int)
*/
@io.qt.QtUninvokable
public final void setProgressValue(int progressValue){
d.setProgressValue(progressValue);
}
/**
* See QPromise::setProgressValueAndText(int,QString)
*/
@io.qt.QtUninvokable
public final void setProgressValueAndText(int progressValue, java.lang.String progressText){
d.setProgressValueAndText(progressValue, progressText);
}
/**
*
*/
@io.qt.QtUninvokable
public final void start(){
d.reportStarted();
}
/**
* See QPromise::suspendIfRequested()
*/
@io.qt.QtUninvokable
public final void suspendIfRequested(){
d.suspendIfRequested();
}
/**
* See QPromise::swap(QPromise<T>&)
*/
@io.qt.QtUninvokable
public final void swap(io.qt.core.QPromise other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
d.swap(other.d);
}
@io.qt.QtUninvokable
public final void setException(Throwable e) {
d.reportException(e);
}
@io.qt.QtUninvokable
public final boolean addResult(T result) {
try {
return QFutureInterface.reportResult(QtJambi_LibraryUtilities.internal.nativeId(d), result, -1);
} catch (QNoImplementationException e) {
throw new QNoImplementationException("addResult(T) not available for QPromise.");
}
}
@io.qt.QtUninvokable
public final boolean addResult(T result, int index) {
try{
return QFutureInterface.reportResult(QtJambi_LibraryUtilities.internal.nativeId(d), result, index);
} catch (QNoImplementationException e) {
throw new QNoImplementationException("addResult(T, int) not available for QPromise.");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy