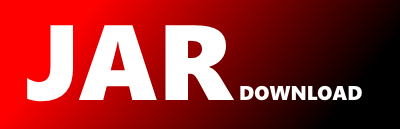
io.qt.core.QTextBoundaryFinder Maven / Gradle / Ivy
package io.qt.core;
/**
* Way of finding Unicode text boundaries in a string
* Java wrapper for Qt class QTextBoundaryFinder
*/
public class QTextBoundaryFinder extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QTextBoundaryFinder::BoundaryReason
*
* @see BoundaryReasons
*/
public enum BoundaryReason implements io.qt.QtFlagEnumerator {
NotAtBoundary(0),
BreakOpportunity(31),
StartOfItem(32),
EndOfItem(64),
MandatoryBreak(128),
SoftHyphen(256);
private BoundaryReason(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public BoundaryReasons asFlags() {
return new BoundaryReasons(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public BoundaryReasons combined(BoundaryReason e) {
return new BoundaryReasons(this, e);
}
/**
* Creates a new {@link BoundaryReasons} from the entries.
* @param values entries
* @return new flag
*/
public static BoundaryReasons flags(BoundaryReason ... values) {
return new BoundaryReasons(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static BoundaryReason resolve(int value) {
switch (value) {
case 0: return NotAtBoundary;
case 31: return BreakOpportunity;
case 32: return StartOfItem;
case 64: return EndOfItem;
case 128: return MandatoryBreak;
case 256: return SoftHyphen;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link BoundaryReason}
*/
public static final class BoundaryReasons extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0x4e12944fa8d67624L;
/**
* Creates a new BoundaryReasons where the flags in args
are set.
* @param args enum entries
*/
public BoundaryReasons(BoundaryReason ... args){
super(args);
}
/**
* Creates a new BoundaryReasons with given value
.
* @param value
*/
public BoundaryReasons(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new BoundaryReasons
*/
@Override
public final BoundaryReasons combined(BoundaryReason e){
return new BoundaryReasons(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final BoundaryReasons setFlag(BoundaryReason e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final BoundaryReasons setFlag(BoundaryReason e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this BoundaryReasons.
* @return array of enum entries
*/
@Override
public final BoundaryReason[] flags(){
return super.flags(BoundaryReason.values());
}
/**
* {@inheritDoc}
*/
@Override
public final BoundaryReasons clone(){
return new BoundaryReasons(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(BoundaryReasons other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum QTextBoundaryFinder::BoundaryType
*/
public enum BoundaryType implements io.qt.QtEnumerator {
Grapheme(0),
Word(1),
Sentence(2),
Line(3);
private BoundaryType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static BoundaryType resolve(int value) {
switch (value) {
case 0: return Grapheme;
case 1: return Word;
case 2: return Sentence;
case 3: return Line;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QTextBoundaryFinder::QTextBoundaryFinder()
*/
public QTextBoundaryFinder(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QTextBoundaryFinder instance);
/**
* See QTextBoundaryFinder::QTextBoundaryFinder(QTextBoundaryFinder::BoundaryType,QString)
*/
public QTextBoundaryFinder(io.qt.core.QTextBoundaryFinder.BoundaryType type, java.lang.String string){
super((QPrivateConstructor)null);
initialize_native(this, type, string);
}
private native static void initialize_native(QTextBoundaryFinder instance, io.qt.core.QTextBoundaryFinder.BoundaryType type, java.lang.String string);
/**
* See QTextBoundaryFinder::QTextBoundaryFinder(QTextBoundaryFinder)
*/
public QTextBoundaryFinder(io.qt.core.QTextBoundaryFinder other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QTextBoundaryFinder instance, io.qt.core.QTextBoundaryFinder other);
/**
* See QTextBoundaryFinder::boundaryReasons()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QTextBoundaryFinder.BoundaryReasons boundaryReasons(){
return new io.qt.core.QTextBoundaryFinder.BoundaryReasons(boundaryReasons_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int boundaryReasons_native_constfct(long __this__nativeId);
/**
* See QTextBoundaryFinder::isAtBoundary()const
*/
@io.qt.QtUninvokable
public final boolean isAtBoundary(){
return isAtBoundary_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isAtBoundary_native_constfct(long __this__nativeId);
/**
* See QTextBoundaryFinder::isValid()const
*/
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QTextBoundaryFinder::position()const
*/
@io.qt.QtUninvokable
public final long position(){
return position_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long position_native_constfct(long __this__nativeId);
/**
* See QTextBoundaryFinder::setPosition(qsizetype)
*/
@io.qt.QtUninvokable
public final void setPosition(long position){
setPosition_native_qsizetype(QtJambi_LibraryUtilities.internal.nativeId(this), position);
}
@io.qt.QtUninvokable
private native void setPosition_native_qsizetype(long __this__nativeId, long position);
/**
* See QTextBoundaryFinder::string()const
*/
@io.qt.QtUninvokable
public final java.lang.String string(){
return string_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String string_native_constfct(long __this__nativeId);
/**
* See QTextBoundaryFinder::toEnd()
*/
@io.qt.QtUninvokable
public final void toEnd(){
toEnd_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void toEnd_native(long __this__nativeId);
/**
* See QTextBoundaryFinder::toNextBoundary()
*/
@io.qt.QtUninvokable
public final long toNextBoundary(){
return toNextBoundary_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long toNextBoundary_native(long __this__nativeId);
/**
* See QTextBoundaryFinder::toPreviousBoundary()
*/
@io.qt.QtUninvokable
public final long toPreviousBoundary(){
return toPreviousBoundary_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long toPreviousBoundary_native(long __this__nativeId);
/**
* See QTextBoundaryFinder::toStart()
*/
@io.qt.QtUninvokable
public final void toStart(){
toStart_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void toStart_native(long __this__nativeId);
/**
* See QTextBoundaryFinder::type()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QTextBoundaryFinder.BoundaryType type(){
return io.qt.core.QTextBoundaryFinder.BoundaryType.resolve(type_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int type_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QTextBoundaryFinder(QPrivateConstructor p) { super(p); }
@Override
public QTextBoundaryFinder clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QTextBoundaryFinder clone_native(long __this_nativeId);
}