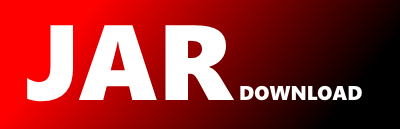
io.qt.gui.QDoubleValidator Maven / Gradle / Ivy
package io.qt.gui;
/**
* Range checking of floating-point numbers
* Java wrapper for Qt class QDoubleValidator
*/
public class QDoubleValidator extends io.qt.gui.QValidator
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QDoubleValidator.class);
/**
* Java wrapper for Qt enum QDoubleValidator::Notation
*/
public enum Notation implements io.qt.QtEnumerator {
StandardNotation(0),
ScientificNotation(1);
private Notation(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Notation resolve(int value) {
switch (value) {
case 0: return StandardNotation;
case 1: return ScientificNotation;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QDoubleValidator::bottomChanged(double)
*/
@io.qt.QtPropertyNotify(name="bottom")
public final Signal1<@io.qt.QtPrimitiveType Double> bottomChanged = new Signal1<>();
/**
* See QDoubleValidator::decimalsChanged(int)
*/
@io.qt.QtPropertyNotify(name="decimals")
public final Signal1<@io.qt.QtPrimitiveType Integer> decimalsChanged = new Signal1<>();
/**
* See QDoubleValidator::notationChanged(QDoubleValidator::Notation)
*/
@io.qt.QtPropertyNotify(name="notation")
public final Signal1 notationChanged = new Signal1<>();
/**
* See QDoubleValidator::topChanged(double)
*/
@io.qt.QtPropertyNotify(name="top")
public final Signal1<@io.qt.QtPrimitiveType Double> topChanged = new Signal1<>();
/**
* Overloaded constructor for {@link #QDoubleValidator(io.qt.core.QObject)}
* with parent = null
.
*/
public QDoubleValidator() {
this((io.qt.core.QObject)null);
}
/**
* See QDoubleValidator::QDoubleValidator(QObject*)
*/
public QDoubleValidator(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QDoubleValidator instance, io.qt.core.QObject parent);
/**
* Overloaded constructor for {@link #QDoubleValidator(double, double, int, io.qt.core.QObject)}
* with parent = null
.
*/
public QDoubleValidator(double bottom, double top, int decimals) {
this(bottom, top, decimals, (io.qt.core.QObject)null);
}
/**
* See QDoubleValidator::QDoubleValidator(double,double,int,QObject*)
*/
public QDoubleValidator(double bottom, double top, int decimals, io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, bottom, top, decimals, parent);
}
private native static void initialize_native(QDoubleValidator instance, double bottom, double top, int decimals, io.qt.core.QObject parent);
/**
* See QDoubleValidator::bottom()const
*/
@io.qt.QtPropertyReader(name="bottom")
@io.qt.QtUninvokable
public final double bottom(){
return bottom_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double bottom_native_constfct(long __this__nativeId);
/**
* See QDoubleValidator::decimals()const
*/
@io.qt.QtPropertyReader(name="decimals")
@io.qt.QtUninvokable
public final int decimals(){
return decimals_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int decimals_native_constfct(long __this__nativeId);
/**
* See QDoubleValidator::notation()const
*/
@io.qt.QtPropertyReader(name="notation")
@io.qt.QtUninvokable
public final io.qt.gui.QDoubleValidator.Notation notation(){
return io.qt.gui.QDoubleValidator.Notation.resolve(notation_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int notation_native_constfct(long __this__nativeId);
/**
* See QDoubleValidator::setBottom(double)
*/
@io.qt.QtPropertyWriter(name="bottom")
@io.qt.QtUninvokable
public final void setBottom(double arg__1){
setBottom_native_double(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setBottom_native_double(long __this__nativeId, double arg__1);
/**
* See QDoubleValidator::setDecimals(int)
*/
@io.qt.QtPropertyWriter(name="decimals")
@io.qt.QtUninvokable
public final void setDecimals(int arg__1){
setDecimals_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setDecimals_native_int(long __this__nativeId, int arg__1);
/**
* See QDoubleValidator::setNotation(QDoubleValidator::Notation)
*/
@io.qt.QtPropertyWriter(name="notation")
@io.qt.QtUninvokable
public final void setNotation(io.qt.gui.QDoubleValidator.Notation arg__1){
setNotation_native_QDoubleValidator_Notation(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void setNotation_native_QDoubleValidator_Notation(long __this__nativeId, int arg__1);
/**
* See QDoubleValidator::setRange(double,double)
*/
@io.qt.QtUninvokable
public final void setRange(double bottom, double top){
setRange_native_double_double(QtJambi_LibraryUtilities.internal.nativeId(this), bottom, top);
}
@io.qt.QtUninvokable
private native void setRange_native_double_double(long __this__nativeId, double bottom, double top);
/**
* See QDoubleValidator::setRange(double,double,int)
*/
@io.qt.QtUninvokable
public final void setRange(double bottom, double top, int decimals){
setRange_native_double_double_int(QtJambi_LibraryUtilities.internal.nativeId(this), bottom, top, decimals);
}
@io.qt.QtUninvokable
private native void setRange_native_double_double_int(long __this__nativeId, double bottom, double top, int decimals);
/**
* See QDoubleValidator::setTop(double)
*/
@io.qt.QtPropertyWriter(name="top")
@io.qt.QtUninvokable
public final void setTop(double arg__1){
setTop_native_double(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setTop_native_double(long __this__nativeId, double arg__1);
/**
* See QDoubleValidator::top()const
*/
@io.qt.QtPropertyReader(name="top")
@io.qt.QtUninvokable
public final double top(){
return top_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double top_native_constfct(long __this__nativeId);
/**
* See QValidator::fixup(QString&)const
*/
@io.qt.QtUninvokable
public java.lang.String fixup(java.lang.String input){
return fixup_native_ref_QString_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), input);
}
@io.qt.QtUninvokable
private native java.lang.String fixup_native_ref_QString_constfct(long __this__nativeId, java.lang.String input);
/**
* See QValidator::validate(QString&,int&)const
*/
@io.qt.QtUninvokable
public io.qt.gui.QValidator.State validate(io.qt.gui.QValidator.QValidationData arg__1){
return io.qt.gui.QValidator.State.resolve(validate_native_ref_QString_ref_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1));
}
@io.qt.QtUninvokable
private native int validate_native_ref_QString_ref_int_constfct(long __this__nativeId, io.qt.gui.QValidator.QValidationData arg__1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QDoubleValidator(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QDoubleValidator(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QDoubleValidator instance, QDeclarativeConstructor constructor);
}