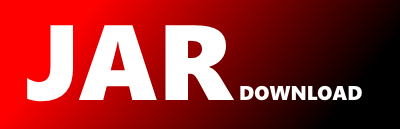
io.qt.gui.QFontInfo Maven / Gradle / Ivy
The newest version!
package io.qt.gui;
/**
* General information about fonts
* Java wrapper for Qt class QFontInfo
*/
public class QFontInfo extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
private static long __qt_expenseCounter = 0;
/**
* See QFontInfo::QFontInfo(QFont)
*/
public QFontInfo(io.qt.gui.QFont arg__1){
super((QPrivateConstructor)null);
initialize_native(this, arg__1);
boolean _dogc;
synchronized(io.qt.gui.QFontInfo.class){
if(_dogc = (__qt_expenseCounter += 1) > 1000)
__qt_expenseCounter = 0;
}
if (_dogc)
System.gc();
}
private native static void initialize_native(QFontInfo instance, io.qt.gui.QFont arg__1);
/**
* See QFontInfo::QFontInfo(QFontInfo)
*/
public QFontInfo(io.qt.gui.QFontInfo arg__1){
super((QPrivateConstructor)null);
initialize_native(this, arg__1);
boolean _dogc;
synchronized(io.qt.gui.QFontInfo.class){
if(_dogc = (__qt_expenseCounter += 1) > 1000)
__qt_expenseCounter = 0;
}
if (_dogc)
System.gc();
}
private native static void initialize_native(QFontInfo instance, io.qt.gui.QFontInfo arg__1);
/**
*
*/
@io.qt.QtUninvokable
public final boolean bold(){
return bold_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean bold_native_constfct(long __this__nativeId);
/**
* See QFontInfo::exactMatch()const
*/
@io.qt.QtUninvokable
public final boolean exactMatch(){
return exactMatch_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean exactMatch_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final java.lang.String family(){
return family_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String family_native_constfct(long __this__nativeId);
/**
* See QFontInfo::fixedPitch()const
*/
@io.qt.QtUninvokable
public final boolean fixedPitch(){
return fixedPitch_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean fixedPitch_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final boolean italic(){
return italic_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean italic_native_constfct(long __this__nativeId);
/**
* @deprecated Use {@link io.qt.gui.QFontInfo#weight()} instead
*/
@Deprecated
@io.qt.QtUninvokable
public final int legacyWeight(){
return legacyWeight_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native int legacyWeight_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean overline(){
return overline_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean overline_native_constfct(long __this__nativeId);
/**
* See QFontInfo::pixelSize()const
*/
@io.qt.QtUninvokable
public final int pixelSize(){
return pixelSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int pixelSize_native_constfct(long __this__nativeId);
/**
* See QFontInfo::pointSize()const
*/
@io.qt.QtUninvokable
public final int pointSize(){
return pointSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int pointSize_native_constfct(long __this__nativeId);
/**
* See QFontInfo::pointSizeF()const
*/
@io.qt.QtUninvokable
public final double pointSizeF(){
return pointSizeF_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native double pointSizeF_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean strikeOut(){
return strikeOut_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean strikeOut_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.gui.QFont.Style style(){
return io.qt.gui.QFont.Style.resolve(style_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int style_native_constfct(long __this__nativeId);
/**
* See QFontInfo::styleHint()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QFont.StyleHint styleHint(){
return io.qt.gui.QFont.StyleHint.resolve(styleHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int styleHint_native_constfct(long __this__nativeId);
/**
* See QFontInfo::styleName()const
*/
@io.qt.QtUninvokable
public final java.lang.String styleName(){
return styleName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String styleName_native_constfct(long __this__nativeId);
/**
* See QFontInfo::swap(QFontInfo&)
*/
@io.qt.QtUninvokable
public final void swap(io.qt.gui.QFontInfo other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QFontInfo(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native void swap_native_ref_QFontInfo(long __this__nativeId, long other);
@io.qt.QtUninvokable
public final boolean underline(){
return underline_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean underline_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final int weight(){
return weight_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int weight_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QFontInfo(QPrivateConstructor p) { super(p); }
@Override
public QFontInfo clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QFontInfo clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy