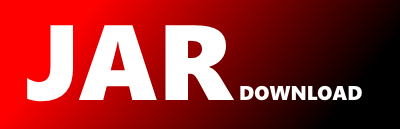
io.qt.internal.PropertyFlags Maven / Gradle / Ivy
The newest version!
package io.qt.internal;
/**
* Java wrapper for Qt enum PropertyFlags
*/
public enum PropertyFlags implements io.qt.QtFlagEnumerator {
Invalid(0),
Readable(1),
Writable(2),
Resettable(4),
EnumOrFlag(8),
Alias(16),
StdCppSet(256),
Constant(1024),
Final(2048),
Designable(4096),
Scriptable(16384),
Stored(65536),
User(1048576),
Required(16777216),
Bindable(33554432);
private PropertyFlags(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public PropertyAttributes asFlags() {
return new PropertyAttributes(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public PropertyAttributes combined(PropertyFlags e) {
return new PropertyAttributes(this, e);
}
/**
* Creates a new {@link PropertyAttributes} from the entries.
* @param values entries
* @return new flag
*/
public static PropertyAttributes flags(PropertyFlags ... values) {
return new PropertyAttributes(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PropertyFlags resolve(int value) {
switch (value) {
case 0: return Invalid;
case 1: return Readable;
case 2: return Writable;
case 4: return Resettable;
case 8: return EnumOrFlag;
case 16: return Alias;
case 256: return StdCppSet;
case 1024: return Constant;
case 2048: return Final;
case 4096: return Designable;
case 16384: return Scriptable;
case 65536: return Stored;
case 1048576: return User;
case 16777216: return Required;
case 33554432: return Bindable;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy