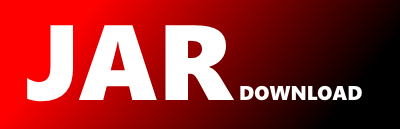
io.qt.widgets.QMessageBox Maven / Gradle / Ivy
package io.qt.widgets;
/**
* Modal dialog for informing the user or for asking the user a question and receiving an answer
* Java wrapper for Qt class QMessageBox
*/
public class QMessageBox extends io.qt.widgets.QDialog
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QMessageBox.class);
/**
* Java wrapper for Qt enum QMessageBox::ButtonRole
*/
@io.qt.QtUnlistedEnum
public enum ButtonRole implements io.qt.QtEnumerator {
InvalidRole(-1),
AcceptRole(0),
RejectRole(1),
DestructiveRole(2),
ActionRole(3),
HelpRole(4),
YesRole(5),
NoRole(6),
ResetRole(7),
ApplyRole(8),
NRoles(9);
private ButtonRole(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ButtonRole resolve(int value) {
switch (value) {
case -1: return InvalidRole;
case 0: return AcceptRole;
case 1: return RejectRole;
case 2: return DestructiveRole;
case 3: return ActionRole;
case 4: return HelpRole;
case 5: return YesRole;
case 6: return NoRole;
case 7: return ResetRole;
case 8: return ApplyRole;
case 9: return NRoles;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QMessageBox::Icon
*/
public enum Icon implements io.qt.QtEnumerator {
NoIcon(0),
Information(1),
Warning(2),
Critical(3),
Question(4);
private Icon(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Icon resolve(int value) {
switch (value) {
case 0: return NoIcon;
case 1: return Information;
case 2: return Warning;
case 3: return Critical;
case 4: return Question;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QMessageBox::StandardButton
*
* @see StandardButtons
*/
@io.qt.QtRejectedEntries({"FirstButton", "LastButton", "YesAll", "NoAll"})
public enum StandardButton implements io.qt.QtFlagEnumerator {
NoButton(0),
Ok(1024),
Save(2048),
SaveAll(4096),
Open(8192),
Yes(16384),
YesToAll(32768),
No(65536),
NoToAll(131072),
Abort(262144),
Retry(524288),
Ignore(1048576),
Close(2097152),
Cancel(4194304),
Discard(8388608),
Help(16777216),
Apply(33554432),
Reset(67108864),
RestoreDefaults(134217728),
FirstButton(1024),
LastButton(134217728),
YesAll(32768),
NoAll(131072),
Default(256),
Escape(512),
FlagMask(768),
ButtonMask(-769);
private StandardButton(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public StandardButtons asFlags() {
return new StandardButtons(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public StandardButtons combined(StandardButton e) {
return new StandardButtons(this, e);
}
/**
* Creates a new {@link StandardButtons} from the entries.
* @param values entries
* @return new flag
*/
public static StandardButtons flags(StandardButton ... values) {
return new StandardButtons(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static StandardButton resolve(int value) {
switch (value) {
case 0: return NoButton;
case 1024: return Ok;
case 2048: return Save;
case 4096: return SaveAll;
case 8192: return Open;
case 16384: return Yes;
case 32768: return YesToAll;
case 65536: return No;
case 131072: return NoToAll;
case 262144: return Abort;
case 524288: return Retry;
case 1048576: return Ignore;
case 2097152: return Close;
case 4194304: return Cancel;
case 8388608: return Discard;
case 16777216: return Help;
case 33554432: return Apply;
case 67108864: return Reset;
case 134217728: return RestoreDefaults;
case 256: return Default;
case 512: return Escape;
case 768: return FlagMask;
case -769: return ButtonMask;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link StandardButton}
*/
public static final class StandardButtons extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xbc3dfa9cca5951bdL;
/**
* Creates a new StandardButtons where the flags in args
are set.
* @param args enum entries
*/
public StandardButtons(StandardButton ... args){
super(args);
}
/**
* Creates a new StandardButtons with given value
.
* @param value
*/
public StandardButtons(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new StandardButtons
*/
@Override
public final StandardButtons combined(StandardButton e){
return new StandardButtons(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final StandardButtons setFlag(StandardButton e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final StandardButtons setFlag(StandardButton e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this StandardButtons.
* @return array of enum entries
*/
@Override
public final StandardButton[] flags(){
return super.flags(StandardButton.values());
}
/**
* {@inheritDoc}
*/
@Override
public final StandardButtons clone(){
return new StandardButtons(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(StandardButtons other){
return Integer.compare(value(), other.value());
}
}
/**
* See QMessageBox::buttonClicked(QAbstractButton*)
*/
public final Signal1 buttonClicked = new Signal1<>();
/**
* Overloaded constructor for {@link #QMessageBox(io.qt.widgets.QMessageBox.Icon, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}.
*/
public QMessageBox(io.qt.widgets.QMessageBox.Icon icon, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QWidget parent, io.qt.core.Qt.WindowType ... flags){
this(icon, title, text, buttons, parent, new io.qt.core.Qt.WindowFlags(flags));
}
/**
* Overloaded constructor for {@link #QMessageBox(io.qt.widgets.QMessageBox.Icon, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}
* with flags = new io.qt.core.Qt.WindowFlags(259)
.
*/
public QMessageBox(io.qt.widgets.QMessageBox.Icon icon, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QWidget parent) {
this(icon, title, text, buttons, parent, new io.qt.core.Qt.WindowFlags(259));
}
/**
* Overloaded constructor for {@link #QMessageBox(io.qt.widgets.QMessageBox.Icon, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}
* with:
* parent = null
* flags = new io.qt.core.Qt.WindowFlags(259)
*
*/
public QMessageBox(io.qt.widgets.QMessageBox.Icon icon, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons) {
this(icon, title, text, buttons, (io.qt.widgets.QWidget)null, new io.qt.core.Qt.WindowFlags(259));
}
/**
* Overloaded constructor for {@link #QMessageBox(io.qt.widgets.QMessageBox.Icon, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}
* with:
* buttons = new io.qt.widgets.QMessageBox.StandardButtons(0)
* parent = null
* flags = new io.qt.core.Qt.WindowFlags(259)
*
*/
public QMessageBox(io.qt.widgets.QMessageBox.Icon icon, java.lang.String title, java.lang.String text) {
this(icon, title, text, new io.qt.widgets.QMessageBox.StandardButtons(0), (io.qt.widgets.QWidget)null, new io.qt.core.Qt.WindowFlags(259));
}
/**
* See QMessageBox::QMessageBox(QMessageBox::Icon,QString,QString,StandardButtons,QWidget*,Qt::WindowFlags)
*/
public QMessageBox(io.qt.widgets.QMessageBox.Icon icon, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QWidget parent, io.qt.core.Qt.WindowFlags flags){
super((QPrivateConstructor)null);
initialize_native(this, icon, title, text, buttons, parent, flags);
}
private native static void initialize_native(QMessageBox instance, io.qt.widgets.QMessageBox.Icon icon, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QWidget parent, io.qt.core.Qt.WindowFlags flags);
/**
* Overloaded constructor for {@link #QMessageBox(io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QMessageBox() {
this((io.qt.widgets.QWidget)null);
}
/**
* See QMessageBox::QMessageBox(QWidget*)
*/
public QMessageBox(io.qt.widgets.QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QMessageBox instance, io.qt.widgets.QWidget parent);
/**
* See QMessageBox::addButton(QAbstractButton*,QMessageBox::ButtonRole)
*/
@io.qt.QtUninvokable
public final void addButton(io.qt.widgets.QAbstractButton button, io.qt.widgets.QMessageBox.ButtonRole role){
addButton_native_QAbstractButton_ptr_QMessageBox_ButtonRole(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(button), role.value());
}
@io.qt.QtUninvokable
private native void addButton_native_QAbstractButton_ptr_QMessageBox_ButtonRole(long __this__nativeId, long button, int role);
/**
* See QMessageBox::addButton(QMessageBox::StandardButton)
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QPushButton addButton(io.qt.widgets.QMessageBox.StandardButton button){
return addButton_native_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.nativeId(this), button.value());
}
@io.qt.QtUninvokable
private native io.qt.widgets.QPushButton addButton_native_QMessageBox_StandardButton(long __this__nativeId, int button);
/**
* See QMessageBox::addButton(QString,QMessageBox::ButtonRole)
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QPushButton addButton(java.lang.String text, io.qt.widgets.QMessageBox.ButtonRole role){
return addButton_native_cref_QString_QMessageBox_ButtonRole(QtJambi_LibraryUtilities.internal.nativeId(this), text, role.value());
}
@io.qt.QtUninvokable
private native io.qt.widgets.QPushButton addButton_native_cref_QString_QMessageBox_ButtonRole(long __this__nativeId, java.lang.String text, int role);
/**
* See QMessageBox::button(QMessageBox::StandardButton)const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QAbstractButton button(io.qt.widgets.QMessageBox.StandardButton which){
return button_native_QMessageBox_StandardButton_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), which.value());
}
@io.qt.QtUninvokable
private native io.qt.widgets.QAbstractButton button_native_QMessageBox_StandardButton_constfct(long __this__nativeId, int which);
/**
* See QMessageBox::buttonRole(QAbstractButton*)const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QMessageBox.ButtonRole buttonRole(io.qt.widgets.QAbstractButton button){
return io.qt.widgets.QMessageBox.ButtonRole.resolve(buttonRole_native_QAbstractButton_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(button)));
}
@io.qt.QtUninvokable
private native int buttonRole_native_QAbstractButton_ptr_constfct(long __this__nativeId, long button);
/**
* See QMessageBox::buttons()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList buttons(){
return buttons_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList buttons_native_constfct(long __this__nativeId);
/**
* See QMessageBox::checkBox()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QCheckBox checkBox(){
return checkBox_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QCheckBox checkBox_native_constfct(long __this__nativeId);
/**
* See QMessageBox::clickedButton()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QAbstractButton clickedButton(){
return clickedButton_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QAbstractButton clickedButton_native_constfct(long __this__nativeId);
/**
* See QMessageBox::defaultButton()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QPushButton defaultButton(){
return defaultButton_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QPushButton defaultButton_native_constfct(long __this__nativeId);
/**
* See QMessageBox::detailedText()const
*/
@io.qt.QtPropertyReader(name="detailedText")
@io.qt.QtUninvokable
public final java.lang.String detailedText(){
return detailedText_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String detailedText_native_constfct(long __this__nativeId);
/**
* See QMessageBox::escapeButton()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QAbstractButton escapeButton(){
return escapeButton_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QAbstractButton escapeButton_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtPropertyReader(name="icon")
@io.qt.QtUninvokable
public final io.qt.widgets.QMessageBox.Icon icon(){
return io.qt.widgets.QMessageBox.Icon.resolve(icon_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int icon_native_constfct(long __this__nativeId);
/**
* See QMessageBox::iconPixmap()const
*/
@io.qt.QtPropertyReader(name="iconPixmap")
@io.qt.QtUninvokable
public final io.qt.gui.QPixmap iconPixmap(){
return iconPixmap_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.gui.QPixmap iconPixmap_native_constfct(long __this__nativeId);
/**
* See QMessageBox::informativeText()const
*/
@io.qt.QtPropertyReader(name="informativeText")
@io.qt.QtUninvokable
public final java.lang.String informativeText(){
return informativeText_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String informativeText_native_constfct(long __this__nativeId);
/**
* See QMessageBox::removeButton(QAbstractButton*)
*/
@io.qt.QtUninvokable
public final void removeButton(io.qt.widgets.QAbstractButton button){
removeButton_native_QAbstractButton_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(button));
}
@io.qt.QtUninvokable
private native void removeButton_native_QAbstractButton_ptr(long __this__nativeId, long button);
/**
* See QMessageBox::setCheckBox(QCheckBox*)
*/
@io.qt.QtUninvokable
public final void setCheckBox(io.qt.widgets.QCheckBox cb){
setCheckBox_native_QCheckBox_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(cb));
QtJambi_LibraryUtilities.internal.setCppOwnership(cb);
}
@io.qt.QtUninvokable
private native void setCheckBox_native_QCheckBox_ptr(long __this__nativeId, long cb);
/**
* See QMessageBox::setDefaultButton(QMessageBox::StandardButton)
*/
@io.qt.QtUninvokable
public final void setDefaultButton(io.qt.widgets.QMessageBox.StandardButton button){
setDefaultButton_native_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.nativeId(this), button.value());
}
@io.qt.QtUninvokable
private native void setDefaultButton_native_QMessageBox_StandardButton(long __this__nativeId, int button);
/**
* See QMessageBox::setDefaultButton(QPushButton*)
*/
@io.qt.QtUninvokable
public final void setDefaultButton(io.qt.widgets.QPushButton button){
setDefaultButton_native_QPushButton_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(button));
}
@io.qt.QtUninvokable
private native void setDefaultButton_native_QPushButton_ptr(long __this__nativeId, long button);
/**
* See QMessageBox::setDetailedText(QString)
*/
@io.qt.QtPropertyWriter(name="detailedText")
@io.qt.QtUninvokable
public final void setDetailedText(java.lang.String text){
setDetailedText_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), text);
}
@io.qt.QtUninvokable
private native void setDetailedText_native_cref_QString(long __this__nativeId, java.lang.String text);
/**
* See QMessageBox::setEscapeButton(QAbstractButton*)
*/
@io.qt.QtUninvokable
public final void setEscapeButton(io.qt.widgets.QAbstractButton button){
setEscapeButton_native_QAbstractButton_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(button));
}
@io.qt.QtUninvokable
private native void setEscapeButton_native_QAbstractButton_ptr(long __this__nativeId, long button);
/**
* See QMessageBox::setEscapeButton(QMessageBox::StandardButton)
*/
@io.qt.QtUninvokable
public final void setEscapeButton(io.qt.widgets.QMessageBox.StandardButton button){
setEscapeButton_native_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.nativeId(this), button.value());
}
@io.qt.QtUninvokable
private native void setEscapeButton_native_QMessageBox_StandardButton(long __this__nativeId, int button);
/**
* See QMessageBox::setIcon(QMessageBox::Icon)
*/
@io.qt.QtPropertyWriter(name="icon")
@io.qt.QtUninvokable
public final void setIcon(io.qt.widgets.QMessageBox.Icon arg__1){
setIcon_native_QMessageBox_Icon(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void setIcon_native_QMessageBox_Icon(long __this__nativeId, int arg__1);
/**
* See QMessageBox::setIconPixmap(QPixmap)
*/
@io.qt.QtPropertyWriter(name="iconPixmap")
@io.qt.QtUninvokable
public final void setIconPixmap(io.qt.gui.QPixmap pixmap){
setIconPixmap_native_cref_QPixmap(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(pixmap));
}
@io.qt.QtUninvokable
private native void setIconPixmap_native_cref_QPixmap(long __this__nativeId, long pixmap);
/**
* See QMessageBox::setInformativeText(QString)
*/
@io.qt.QtPropertyWriter(name="informativeText")
@io.qt.QtUninvokable
public final void setInformativeText(java.lang.String text){
setInformativeText_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), text);
}
@io.qt.QtUninvokable
private native void setInformativeText_native_cref_QString(long __this__nativeId, java.lang.String text);
/**
* Overloaded function for {@link #setStandardButtons(io.qt.widgets.QMessageBox.StandardButtons)}.
*/
@io.qt.QtUninvokable
public final void setStandardButtons(io.qt.widgets.QMessageBox.StandardButton ... buttons){
setStandardButtons(new io.qt.widgets.QMessageBox.StandardButtons(buttons));
}
/**
* See QMessageBox::setStandardButtons(StandardButtons)
*/
@io.qt.QtPropertyWriter(name="standardButtons")
@io.qt.QtUninvokable
public final void setStandardButtons(io.qt.widgets.QMessageBox.StandardButtons buttons){
setStandardButtons_native_QFlags_QMessageBox_StandardButton_(QtJambi_LibraryUtilities.internal.nativeId(this), buttons.value());
}
@io.qt.QtUninvokable
private native void setStandardButtons_native_QFlags_QMessageBox_StandardButton_(long __this__nativeId, int buttons);
/**
* See QMessageBox::setText(QString)
*/
@io.qt.QtPropertyWriter(name="text")
@io.qt.QtUninvokable
public final void setText(java.lang.String text){
setText_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), text);
}
@io.qt.QtUninvokable
private native void setText_native_cref_QString(long __this__nativeId, java.lang.String text);
/**
* See QMessageBox::setTextFormat(Qt::TextFormat)
*/
@io.qt.QtPropertyWriter(name="textFormat")
@io.qt.QtUninvokable
public final void setTextFormat(io.qt.core.Qt.TextFormat format){
setTextFormat_native_Qt_TextFormat(QtJambi_LibraryUtilities.internal.nativeId(this), format.value());
}
@io.qt.QtUninvokable
private native void setTextFormat_native_Qt_TextFormat(long __this__nativeId, int format);
/**
* Overloaded function for {@link #setTextInteractionFlags(io.qt.core.Qt.TextInteractionFlags)}.
*/
@io.qt.QtUninvokable
public final void setTextInteractionFlags(io.qt.core.Qt.TextInteractionFlag ... flags){
setTextInteractionFlags(new io.qt.core.Qt.TextInteractionFlags(flags));
}
/**
* See QMessageBox::setTextInteractionFlags(Qt::TextInteractionFlags)
*/
@io.qt.QtPropertyWriter(name="textInteractionFlags")
@io.qt.QtUninvokable
public final void setTextInteractionFlags(io.qt.core.Qt.TextInteractionFlags flags){
setTextInteractionFlags_native_QFlags_Qt_TextInteractionFlag_(QtJambi_LibraryUtilities.internal.nativeId(this), flags.value());
}
@io.qt.QtUninvokable
private native void setTextInteractionFlags_native_QFlags_Qt_TextInteractionFlag_(long __this__nativeId, int flags);
/**
* See QMessageBox::standardButton(QAbstractButton*)const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QMessageBox.StandardButton standardButton(io.qt.widgets.QAbstractButton button){
return io.qt.widgets.QMessageBox.StandardButton.resolve(standardButton_native_QAbstractButton_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(button)));
}
@io.qt.QtUninvokable
private native int standardButton_native_QAbstractButton_ptr_constfct(long __this__nativeId, long button);
/**
* See QMessageBox::standardButtons()const
*/
@io.qt.QtPropertyReader(name="standardButtons")
@io.qt.QtUninvokable
public final io.qt.widgets.QMessageBox.StandardButtons standardButtons(){
return new io.qt.widgets.QMessageBox.StandardButtons(standardButtons_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int standardButtons_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtPropertyReader(name="text")
@io.qt.QtUninvokable
public final java.lang.String text(){
return text_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String text_native_constfct(long __this__nativeId);
/**
* See QMessageBox::textFormat()const
*/
@io.qt.QtPropertyReader(name="textFormat")
@io.qt.QtUninvokable
public final io.qt.core.Qt.TextFormat textFormat(){
return io.qt.core.Qt.TextFormat.resolve(textFormat_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int textFormat_native_constfct(long __this__nativeId);
/**
* See QMessageBox::textInteractionFlags()const
*/
@io.qt.QtPropertyReader(name="textInteractionFlags")
@io.qt.QtUninvokable
public final io.qt.core.Qt.TextInteractionFlags textInteractionFlags(){
return new io.qt.core.Qt.TextInteractionFlags(textInteractionFlags_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int textInteractionFlags_native_constfct(long __this__nativeId);
/**
* See QWidget::changeEvent(QEvent*)
*/
@io.qt.QtUninvokable
protected void changeEvent(io.qt.core.QEvent event){
changeEvent_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void changeEvent_native_QEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::closeEvent(QCloseEvent*)
*/
@io.qt.QtUninvokable
protected void closeEvent(io.qt.gui.QCloseEvent event){
closeEvent_native_QCloseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void closeEvent_native_QCloseEvent_ptr(long __this__nativeId, long event);
/**
*
*/
@io.qt.QtUninvokable
public boolean event(io.qt.core.QEvent e){
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(e));
}
@io.qt.QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long e);
/**
* See QWidget::keyPressEvent(QKeyEvent*)
*/
@io.qt.QtUninvokable
protected void keyPressEvent(io.qt.gui.QKeyEvent event){
keyPressEvent_native_QKeyEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void keyPressEvent_native_QKeyEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::resizeEvent(QResizeEvent*)
*/
@io.qt.QtUninvokable
protected void resizeEvent(io.qt.gui.QResizeEvent event){
resizeEvent_native_QResizeEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void resizeEvent_native_QResizeEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::showEvent(QShowEvent*)
*/
@io.qt.QtUninvokable
protected void showEvent(io.qt.gui.QShowEvent event){
showEvent_native_QShowEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void showEvent_native_QShowEvent_ptr(long __this__nativeId, long event);
/**
* See QMessageBox::about(QWidget*,QString,QString)
*/
public static void about(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text){
about_native_QWidget_ptr_cref_QString_cref_QString(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text);
}
private native static void about_native_QWidget_ptr_cref_QString_cref_QString(long parent, java.lang.String title, java.lang.String text);
/**
* Overloaded function for {@link #aboutQt(io.qt.widgets.QWidget, java.lang.String)}
* with title = ""
.
*/
public static void aboutQt(io.qt.widgets.QWidget parent) {
aboutQt(parent, "");
}
/**
* See QMessageBox::aboutQt(QWidget*,QString)
*/
public static void aboutQt(io.qt.widgets.QWidget parent, java.lang.String title){
aboutQt_native_QWidget_ptr_cref_QString(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title);
}
private native static void aboutQt_native_QWidget_ptr_cref_QString(long parent, java.lang.String title);
/**
* Overloaded function for {@link #critical(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
.
*/
public static io.qt.widgets.QMessageBox.StandardButton critical(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons) {
return critical(parent, title, text, buttons, io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* Overloaded function for {@link #critical(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with:
* buttons = new io.qt.widgets.QMessageBox.StandardButtons(1024)
* defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
*
*/
public static io.qt.widgets.QMessageBox.StandardButton critical(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text) {
return critical(parent, title, text, new io.qt.widgets.QMessageBox.StandardButtons(1024), io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* See QMessageBox::critical(QWidget*,QString,QString,StandardButtons,QMessageBox::StandardButton)
*/
public static io.qt.widgets.QMessageBox.StandardButton critical(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QMessageBox.StandardButton defaultButton){
return io.qt.widgets.QMessageBox.StandardButton.resolve(critical_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, buttons.value(), defaultButton.value()));
}
private native static int critical_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int buttons, int defaultButton);
/**
*
*/
public static int critical(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButton button0, io.qt.widgets.QMessageBox.StandardButton button1){
return critical_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, button0.value(), button1.value());
}
private native static int critical_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int button0, int button1);
/**
* Overloaded function for {@link #information(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
.
*/
public static io.qt.widgets.QMessageBox.StandardButton information(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons) {
return information(parent, title, text, buttons, io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* Overloaded function for {@link #information(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with:
* buttons = new io.qt.widgets.QMessageBox.StandardButtons(1024)
* defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
*
*/
public static io.qt.widgets.QMessageBox.StandardButton information(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text) {
return information(parent, title, text, new io.qt.widgets.QMessageBox.StandardButtons(1024), io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* See QMessageBox::information(QWidget*,QString,QString,StandardButtons,QMessageBox::StandardButton)
*/
public static io.qt.widgets.QMessageBox.StandardButton information(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QMessageBox.StandardButton defaultButton){
return io.qt.widgets.QMessageBox.StandardButton.resolve(information_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, buttons.value(), defaultButton.value()));
}
private native static int information_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int buttons, int defaultButton);
/**
* Overloaded function for {@link #information(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButton, io.qt.widgets.QMessageBox.StandardButton)}
* with button1 = io.qt.widgets.QMessageBox.StandardButton.NoButton
.
*/
public static io.qt.widgets.QMessageBox.StandardButton information(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButton button0) {
return information(parent, title, text, button0, io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
*
*/
public static io.qt.widgets.QMessageBox.StandardButton information(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButton button0, io.qt.widgets.QMessageBox.StandardButton button1){
return io.qt.widgets.QMessageBox.StandardButton.resolve(information_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, button0.value(), button1.value()));
}
private native static int information_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int button0, int button1);
/**
* Overloaded function for {@link #question(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
.
*/
public static io.qt.widgets.QMessageBox.StandardButton question(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons) {
return question(parent, title, text, buttons, io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* Overloaded function for {@link #question(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with:
* buttons = new io.qt.widgets.QMessageBox.StandardButtons(81920)
* defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
*
*/
public static io.qt.widgets.QMessageBox.StandardButton question(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text) {
return question(parent, title, text, new io.qt.widgets.QMessageBox.StandardButtons(81920), io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* See QMessageBox::question(QWidget*,QString,QString,StandardButtons,QMessageBox::StandardButton)
*/
public static io.qt.widgets.QMessageBox.StandardButton question(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QMessageBox.StandardButton defaultButton){
return io.qt.widgets.QMessageBox.StandardButton.resolve(question_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, buttons.value(), defaultButton.value()));
}
private native static int question_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int buttons, int defaultButton);
/**
*
*/
public static int question(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButton button0, io.qt.widgets.QMessageBox.StandardButton button1){
return question_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, button0.value(), button1.value());
}
private native static int question_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int button0, int button1);
/**
* Overloaded function for {@link #warning(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
.
*/
public static io.qt.widgets.QMessageBox.StandardButton warning(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons) {
return warning(parent, title, text, buttons, io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* Overloaded function for {@link #warning(io.qt.widgets.QWidget, java.lang.String, java.lang.String, io.qt.widgets.QMessageBox.StandardButtons, io.qt.widgets.QMessageBox.StandardButton)}
* with:
* buttons = new io.qt.widgets.QMessageBox.StandardButtons(1024)
* defaultButton = io.qt.widgets.QMessageBox.StandardButton.NoButton
*
*/
public static io.qt.widgets.QMessageBox.StandardButton warning(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text) {
return warning(parent, title, text, new io.qt.widgets.QMessageBox.StandardButtons(1024), io.qt.widgets.QMessageBox.StandardButton.NoButton);
}
/**
* See QMessageBox::warning(QWidget*,QString,QString,StandardButtons,QMessageBox::StandardButton)
*/
public static io.qt.widgets.QMessageBox.StandardButton warning(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButtons buttons, io.qt.widgets.QMessageBox.StandardButton defaultButton){
return io.qt.widgets.QMessageBox.StandardButton.resolve(warning_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, buttons.value(), defaultButton.value()));
}
private native static int warning_native_QWidget_ptr_cref_QString_cref_QString_QFlags_QMessageBox_StandardButton__QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int buttons, int defaultButton);
/**
*
*/
public static int warning(io.qt.widgets.QWidget parent, java.lang.String title, java.lang.String text, io.qt.widgets.QMessageBox.StandardButton button0, io.qt.widgets.QMessageBox.StandardButton button1){
return warning_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(QtJambi_LibraryUtilities.internal.checkedNativeId(parent), title, text, button0.value(), button1.value());
}
private native static int warning_native_QWidget_ptr_cref_QString_cref_QString_QMessageBox_StandardButton_QMessageBox_StandardButton(long parent, java.lang.String title, java.lang.String text, int button0, int button1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QMessageBox(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QMessageBox(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QMessageBox instance, QDeclarativeConstructor constructor);
}