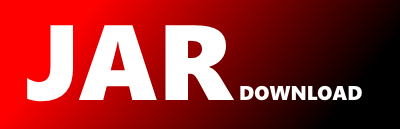
io.qt.widgets.QSizeGrip Maven / Gradle / Ivy
The newest version!
package io.qt.widgets;
/**
* Resize handle for resizing top-level windows
* Java wrapper for Qt class QSizeGrip
*/
public class QSizeGrip extends io.qt.widgets.QWidget
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QSizeGrip.class);
/**
* See QSizeGrip::QSizeGrip(QWidget*)
*/
public QSizeGrip(io.qt.widgets.QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QSizeGrip instance, io.qt.widgets.QWidget parent);
/**
*
*/
@io.qt.QtUninvokable
public boolean event(io.qt.core.QEvent arg__1){
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QObject::eventFilter(QObject*,QEvent*)
*/
@io.qt.QtUninvokable
public boolean eventFilter(io.qt.core.QObject arg__1, io.qt.core.QEvent arg__2){
return eventFilter_native_QObject_ptr_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__2));
}
@io.qt.QtUninvokable
private native boolean eventFilter_native_QObject_ptr_QEvent_ptr(long __this__nativeId, long arg__1, long arg__2);
/**
* See QWidget::hideEvent(QHideEvent*)
*/
@io.qt.QtUninvokable
protected void hideEvent(io.qt.gui.QHideEvent hideEvent){
hideEvent_native_QHideEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(hideEvent));
}
@io.qt.QtUninvokable
private native void hideEvent_native_QHideEvent_ptr(long __this__nativeId, long hideEvent);
/**
* See QWidget::mouseMoveEvent(QMouseEvent*)
*/
@io.qt.QtUninvokable
protected void mouseMoveEvent(io.qt.gui.QMouseEvent arg__1){
mouseMoveEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void mouseMoveEvent_native_QMouseEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget::mousePressEvent(QMouseEvent*)
*/
@io.qt.QtUninvokable
protected void mousePressEvent(io.qt.gui.QMouseEvent arg__1){
mousePressEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void mousePressEvent_native_QMouseEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget::mouseReleaseEvent(QMouseEvent*)
*/
@io.qt.QtUninvokable
protected void mouseReleaseEvent(io.qt.gui.QMouseEvent mouseEvent){
mouseReleaseEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(mouseEvent));
}
@io.qt.QtUninvokable
private native void mouseReleaseEvent_native_QMouseEvent_ptr(long __this__nativeId, long mouseEvent);
/**
* See QWidget::moveEvent(QMoveEvent*)
*/
@io.qt.QtUninvokable
protected void moveEvent(io.qt.gui.QMoveEvent moveEvent){
moveEvent_native_QMoveEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(moveEvent));
}
@io.qt.QtUninvokable
private native void moveEvent_native_QMoveEvent_ptr(long __this__nativeId, long moveEvent);
/**
* See QWidget::paintEvent(QPaintEvent*)
*/
@io.qt.QtUninvokable
protected void paintEvent(io.qt.gui.QPaintEvent arg__1){
paintEvent_native_QPaintEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@io.qt.QtUninvokable
private native void paintEvent_native_QPaintEvent_ptr(long __this__nativeId, long arg__1);
/**
*
*/
@io.qt.QtUninvokable
public void setVisible(boolean arg__1){
setVisible_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setVisible_native_bool(long __this__nativeId, boolean arg__1);
/**
* See QWidget::showEvent(QShowEvent*)
*/
@io.qt.QtUninvokable
protected void showEvent(io.qt.gui.QShowEvent showEvent){
showEvent_native_QShowEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(showEvent));
}
@io.qt.QtUninvokable
private native void showEvent_native_QShowEvent_ptr(long __this__nativeId, long showEvent);
/**
*
*/
@io.qt.QtUninvokable
public io.qt.core.QSize sizeHint(){
return sizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QSize sizeHint_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QSizeGrip(QPrivateConstructor p) { super(p); }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy