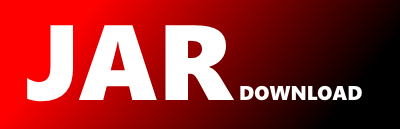
io.qt.widgets.QWizard Maven / Gradle / Ivy
package io.qt.widgets;
/**
* Framework for wizards
* Java wrapper for Qt class QWizard
*/
public class QWizard extends io.qt.widgets.QDialog
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QWizard.class);
/**
* Java wrapper for Qt enum QWizard::WizardButton
*/
@io.qt.QtUnlistedEnum
@io.qt.QtRejectedEntries({"NStandardButtons", "NButtons"})
public enum WizardButton implements io.qt.QtEnumerator {
BackButton(0),
NextButton(1),
CommitButton(2),
FinishButton(3),
CancelButton(4),
HelpButton(5),
CustomButton1(6),
CustomButton2(7),
CustomButton3(8),
Stretch(9),
NoButton(-1),
NStandardButtons(6),
NButtons(9);
private WizardButton(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WizardButton resolve(int value) {
switch (value) {
case 0: return BackButton;
case 1: return NextButton;
case 2: return CommitButton;
case 3: return FinishButton;
case 4: return CancelButton;
case 5: return HelpButton;
case 6: return CustomButton1;
case 7: return CustomButton2;
case 8: return CustomButton3;
case 9: return Stretch;
case -1: return NoButton;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QWizard::WizardOption
*
* @see WizardOptions
*/
public enum WizardOption implements io.qt.QtFlagEnumerator {
IndependentPages(1),
IgnoreSubTitles(2),
ExtendedWatermarkPixmap(4),
NoDefaultButton(8),
NoBackButtonOnStartPage(16),
NoBackButtonOnLastPage(32),
DisabledBackButtonOnLastPage(64),
HaveNextButtonOnLastPage(128),
HaveFinishButtonOnEarlyPages(256),
NoCancelButton(512),
CancelButtonOnLeft(1024),
HaveHelpButton(2048),
HelpButtonOnRight(4096),
HaveCustomButton1(8192),
HaveCustomButton2(16384),
HaveCustomButton3(32768),
NoCancelButtonOnLastPage(65536);
private WizardOption(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public WizardOptions asFlags() {
return new WizardOptions(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public WizardOptions combined(WizardOption e) {
return new WizardOptions(this, e);
}
/**
* Creates a new {@link WizardOptions} from the entries.
* @param values entries
* @return new flag
*/
public static WizardOptions flags(WizardOption ... values) {
return new WizardOptions(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WizardOption resolve(int value) {
switch (value) {
case 1: return IndependentPages;
case 2: return IgnoreSubTitles;
case 4: return ExtendedWatermarkPixmap;
case 8: return NoDefaultButton;
case 16: return NoBackButtonOnStartPage;
case 32: return NoBackButtonOnLastPage;
case 64: return DisabledBackButtonOnLastPage;
case 128: return HaveNextButtonOnLastPage;
case 256: return HaveFinishButtonOnEarlyPages;
case 512: return NoCancelButton;
case 1024: return CancelButtonOnLeft;
case 2048: return HaveHelpButton;
case 4096: return HelpButtonOnRight;
case 8192: return HaveCustomButton1;
case 16384: return HaveCustomButton2;
case 32768: return HaveCustomButton3;
case 65536: return NoCancelButtonOnLastPage;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link WizardOption}
*/
public static final class WizardOptions extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xb37409cf5a8aaa28L;
/**
* Creates a new WizardOptions where the flags in args
are set.
* @param args enum entries
*/
public WizardOptions(WizardOption ... args){
super(args);
}
/**
* Creates a new WizardOptions with given value
.
* @param value
*/
public WizardOptions(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new WizardOptions
*/
@Override
public final WizardOptions combined(WizardOption e){
return new WizardOptions(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final WizardOptions setFlag(WizardOption e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final WizardOptions setFlag(WizardOption e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this WizardOptions.
* @return array of enum entries
*/
@Override
public final WizardOption[] flags(){
return super.flags(WizardOption.values());
}
/**
* {@inheritDoc}
*/
@Override
public final WizardOptions clone(){
return new WizardOptions(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(WizardOptions other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum QWizard::WizardPixmap
*/
@io.qt.QtUnlistedEnum
public enum WizardPixmap implements io.qt.QtEnumerator {
WatermarkPixmap(0),
LogoPixmap(1),
BannerPixmap(2),
BackgroundPixmap(3),
NPixmaps(4);
private WizardPixmap(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WizardPixmap resolve(int value) {
switch (value) {
case 0: return WatermarkPixmap;
case 1: return LogoPixmap;
case 2: return BannerPixmap;
case 3: return BackgroundPixmap;
case 4: return NPixmaps;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QWizard::WizardStyle
*/
public enum WizardStyle implements io.qt.QtEnumerator {
ClassicStyle(0),
ModernStyle(1),
MacStyle(2),
AeroStyle(3),
NStyles(4);
private WizardStyle(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static WizardStyle resolve(int value) {
switch (value) {
case 0: return ClassicStyle;
case 1: return ModernStyle;
case 2: return MacStyle;
case 3: return AeroStyle;
case 4: return NStyles;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QWizard::currentIdChanged(int)
*/
@io.qt.QtPropertyNotify(name="currentId")
public final Signal1<@io.qt.QtPrimitiveType Integer> currentIdChanged = new Signal1<>();
/**
* See QWizard::customButtonClicked(int)
*/
public final Signal1<@io.qt.QtPrimitiveType Integer> customButtonClicked = new Signal1<>();
/**
*
*/
public final Signal0 helpRequested = new Signal0();
/**
*
*/
public final Signal1<@io.qt.QtPrimitiveType Integer> pageAdded = new Signal1<>();
/**
*
*/
public final Signal1<@io.qt.QtPrimitiveType Integer> pageRemoved = new Signal1<>();
/**
* Overloaded constructor for {@link #QWizard(io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}.
*/
public QWizard(io.qt.widgets.QWidget parent, io.qt.core.Qt.WindowType ... flags){
this(parent, new io.qt.core.Qt.WindowFlags(flags));
}
/**
* Overloaded constructor for {@link #QWizard(io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}
* with flags = new io.qt.core.Qt.WindowFlags(0)
.
*/
public QWizard(io.qt.widgets.QWidget parent) {
this(parent, new io.qt.core.Qt.WindowFlags(0));
}
/**
* Overloaded constructor for {@link #QWizard(io.qt.widgets.QWidget, io.qt.core.Qt.WindowFlags)}
* with:
* parent = null
* flags = new io.qt.core.Qt.WindowFlags(0)
*
*/
public QWizard() {
this((io.qt.widgets.QWidget)null, new io.qt.core.Qt.WindowFlags(0));
}
/**
* See QWizard::QWizard(QWidget*,Qt::WindowFlags)
*/
public QWizard(io.qt.widgets.QWidget parent, io.qt.core.Qt.WindowFlags flags){
super((QPrivateConstructor)null);
initialize_native(this, parent, flags);
}
private native static void initialize_native(QWizard instance, io.qt.widgets.QWidget parent, io.qt.core.Qt.WindowFlags flags);
/**
* See QWizard::addPage(QWizardPage*)
*/
@io.qt.QtUninvokable
public final int addPage(io.qt.widgets.QWizardPage page){
java.util.Objects.requireNonNull(page, "Argument 'page': null not expected.");
return addPage_native_QWizardPage_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(page));
}
@io.qt.QtUninvokable
private native int addPage_native_QWizardPage_ptr(long __this__nativeId, long page);
/**
* See QWizard::back()
*/
public final void back(){
back_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void back_native(long __this__nativeId);
/**
* See QWizard::button(QWizard::WizardButton)const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QAbstractButton button(io.qt.widgets.QWizard.WizardButton which){
return button_native_QWizard_WizardButton_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), which.value());
}
@io.qt.QtUninvokable
private native io.qt.widgets.QAbstractButton button_native_QWizard_WizardButton_constfct(long __this__nativeId, int which);
/**
* See QWizard::buttonText(QWizard::WizardButton)const
*/
@io.qt.QtUninvokable
public final java.lang.String buttonText(io.qt.widgets.QWizard.WizardButton which){
return buttonText_native_QWizard_WizardButton_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), which.value());
}
@io.qt.QtUninvokable
private native java.lang.String buttonText_native_QWizard_WizardButton_constfct(long __this__nativeId, int which);
/**
*
*/
@io.qt.QtPropertyReader(name="currentId")
@io.qt.QtUninvokable
public final int currentId(){
return currentId_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int currentId_native_constfct(long __this__nativeId);
/**
* See QWizard::currentPage()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QWizardPage currentPage(){
return currentPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QWizardPage currentPage_native_constfct(long __this__nativeId);
/**
* See QWizard::field(QString)const
*/
@io.qt.QtUninvokable
public final java.lang.Object field(java.lang.String name){
return field_native_cref_QString_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), name);
}
@io.qt.QtUninvokable
private native java.lang.Object field_native_cref_QString_constfct(long __this__nativeId, java.lang.String name);
/**
* See QWizard::hasVisitedPage(int)const
*/
@io.qt.QtUninvokable
public final boolean hasVisitedPage(int id){
return hasVisitedPage_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@io.qt.QtUninvokable
private native boolean hasVisitedPage_native_int_constfct(long __this__nativeId, int id);
/**
* See QWizard::next()
*/
public final void next(){
next_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void next_native(long __this__nativeId);
/**
*
*/
@io.qt.QtPropertyReader(name="options")
@io.qt.QtUninvokable
public final io.qt.widgets.QWizard.WizardOptions options(){
return new io.qt.widgets.QWizard.WizardOptions(options_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int options_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QWizardPage page(int id){
return page_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@io.qt.QtUninvokable
private native io.qt.widgets.QWizardPage page_native_int_constfct(long __this__nativeId, int id);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.core.QList pageIds(){
return pageIds_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList pageIds_native_constfct(long __this__nativeId);
/**
* See QWizard::pixmap(QWizard::WizardPixmap)const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPixmap pixmap(io.qt.widgets.QWizard.WizardPixmap which){
return pixmap_native_QWizard_WizardPixmap_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), which.value());
}
@io.qt.QtUninvokable
private native io.qt.gui.QPixmap pixmap_native_QWizard_WizardPixmap_constfct(long __this__nativeId, int which);
/**
*
*/
@io.qt.QtUninvokable
public final void removePage(int id){
removePage_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@io.qt.QtUninvokable
private native void removePage_native_int(long __this__nativeId, int id);
/**
*
*/
public final void restart(){
restart_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void restart_native(long __this__nativeId);
/**
* See QWizard::setButton(QWizard::WizardButton,QAbstractButton*)
*/
@io.qt.QtUninvokable
public final void setButton(io.qt.widgets.QWizard.WizardButton which, io.qt.widgets.QAbstractButton button){
setButton_native_QWizard_WizardButton_QAbstractButton_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), which.value(), QtJambi_LibraryUtilities.internal.checkedNativeId(button));
}
@io.qt.QtUninvokable
private native void setButton_native_QWizard_WizardButton_QAbstractButton_ptr(long __this__nativeId, int which, long button);
/**
* See QWizard::setButtonLayout(QList<QWizard::WizardButton>)
*/
@io.qt.QtUninvokable
public final void setButtonLayout(java.util.Collection layout){
setButtonLayout_native_cref_QList(QtJambi_LibraryUtilities.internal.nativeId(this), layout);
}
@io.qt.QtUninvokable
private native void setButtonLayout_native_cref_QList(long __this__nativeId, java.util.Collection layout);
/**
* See QWizard::setButtonText(QWizard::WizardButton,QString)
*/
@io.qt.QtUninvokable
public final void setButtonText(io.qt.widgets.QWizard.WizardButton which, java.lang.String text){
setButtonText_native_QWizard_WizardButton_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), which.value(), text);
}
@io.qt.QtUninvokable
private native void setButtonText_native_QWizard_WizardButton_cref_QString(long __this__nativeId, int which, java.lang.String text);
/**
* See QWizard::setField(QString,QVariant)
*/
@io.qt.QtUninvokable
public final void setField(java.lang.String name, java.lang.Object value){
setField_native_cref_QString_cref_QVariant(QtJambi_LibraryUtilities.internal.nativeId(this), name, value);
}
@io.qt.QtUninvokable
private native void setField_native_cref_QString_cref_QVariant(long __this__nativeId, java.lang.String name, java.lang.Object value);
/**
* Overloaded function for {@link #setOption(io.qt.widgets.QWizard.WizardOption, boolean)}
* with on = true
.
*/
@io.qt.QtUninvokable
public final void setOption(io.qt.widgets.QWizard.WizardOption option) {
setOption(option, (boolean)true);
}
/**
* See QWizard::setOption(QWizard::WizardOption,bool)
*/
@io.qt.QtUninvokable
public final void setOption(io.qt.widgets.QWizard.WizardOption option, boolean on){
setOption_native_QWizard_WizardOption_bool(QtJambi_LibraryUtilities.internal.nativeId(this), option.value(), on);
}
@io.qt.QtUninvokable
private native void setOption_native_QWizard_WizardOption_bool(long __this__nativeId, int option, boolean on);
/**
* Overloaded function for {@link #setOptions(io.qt.widgets.QWizard.WizardOptions)}.
*/
@io.qt.QtUninvokable
public final void setOptions(io.qt.widgets.QWizard.WizardOption ... options){
setOptions(new io.qt.widgets.QWizard.WizardOptions(options));
}
/**
* See QWizard::setOptions(WizardOptions)
*/
@io.qt.QtPropertyWriter(name="options")
@io.qt.QtUninvokable
public final void setOptions(io.qt.widgets.QWizard.WizardOptions options){
setOptions_native_QFlags_QWizard_WizardOption_(QtJambi_LibraryUtilities.internal.nativeId(this), options.value());
}
@io.qt.QtUninvokable
private native void setOptions_native_QFlags_QWizard_WizardOption_(long __this__nativeId, int options);
/**
* See QWizard::setPage(int,QWizardPage*)
*/
@io.qt.QtUninvokable
public final void setPage(int id, io.qt.widgets.QWizardPage page){
java.util.Objects.requireNonNull(page, "Argument 'page': null not expected.");
setPage_native_int_QWizardPage_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), id, QtJambi_LibraryUtilities.internal.checkedNativeId(page));
}
@io.qt.QtUninvokable
private native void setPage_native_int_QWizardPage_ptr(long __this__nativeId, int id, long page);
/**
* See QWizard::setPixmap(QWizard::WizardPixmap,QPixmap)
*/
@io.qt.QtUninvokable
public final void setPixmap(io.qt.widgets.QWizard.WizardPixmap which, io.qt.gui.QPixmap pixmap){
setPixmap_native_QWizard_WizardPixmap_cref_QPixmap(QtJambi_LibraryUtilities.internal.nativeId(this), which.value(), QtJambi_LibraryUtilities.internal.checkedNativeId(pixmap));
}
@io.qt.QtUninvokable
private native void setPixmap_native_QWizard_WizardPixmap_cref_QPixmap(long __this__nativeId, int which, long pixmap);
/**
* See QWizard::setSideWidget(QWidget*)
*/
@io.qt.QtUninvokable
public final void setSideWidget(io.qt.widgets.QWidget widget){
setSideWidget_native_QWidget_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(widget));
}
@io.qt.QtUninvokable
private native void setSideWidget_native_QWidget_ptr(long __this__nativeId, long widget);
/**
*
*/
@io.qt.QtPropertyWriter(name="startId")
@io.qt.QtUninvokable
public final void setStartId(int id){
setStartId_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@io.qt.QtUninvokable
private native void setStartId_native_int(long __this__nativeId, int id);
/**
* See QWizard::setSubTitleFormat(Qt::TextFormat)
*/
@io.qt.QtPropertyWriter(name="subTitleFormat")
@io.qt.QtUninvokable
public final void setSubTitleFormat(io.qt.core.Qt.TextFormat format){
setSubTitleFormat_native_Qt_TextFormat(QtJambi_LibraryUtilities.internal.nativeId(this), format.value());
}
@io.qt.QtUninvokable
private native void setSubTitleFormat_native_Qt_TextFormat(long __this__nativeId, int format);
/**
* See QWizard::setTitleFormat(Qt::TextFormat)
*/
@io.qt.QtPropertyWriter(name="titleFormat")
@io.qt.QtUninvokable
public final void setTitleFormat(io.qt.core.Qt.TextFormat format){
setTitleFormat_native_Qt_TextFormat(QtJambi_LibraryUtilities.internal.nativeId(this), format.value());
}
@io.qt.QtUninvokable
private native void setTitleFormat_native_Qt_TextFormat(long __this__nativeId, int format);
/**
* See QWizard::setWizardStyle(QWizard::WizardStyle)
*/
@io.qt.QtPropertyWriter(name="wizardStyle")
@io.qt.QtUninvokable
public final void setWizardStyle(io.qt.widgets.QWizard.WizardStyle style){
setWizardStyle_native_QWizard_WizardStyle(QtJambi_LibraryUtilities.internal.nativeId(this), style.value());
}
@io.qt.QtUninvokable
private native void setWizardStyle_native_QWizard_WizardStyle(long __this__nativeId, int style);
/**
* See QWizard::sideWidget()const
*/
@io.qt.QtUninvokable
public final io.qt.widgets.QWidget sideWidget(){
return sideWidget_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.widgets.QWidget sideWidget_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtPropertyReader(name="startId")
@io.qt.QtUninvokable
public final int startId(){
return startId_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int startId_native_constfct(long __this__nativeId);
/**
* See QWizard::subTitleFormat()const
*/
@io.qt.QtPropertyReader(name="subTitleFormat")
@io.qt.QtUninvokable
public final io.qt.core.Qt.TextFormat subTitleFormat(){
return io.qt.core.Qt.TextFormat.resolve(subTitleFormat_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int subTitleFormat_native_constfct(long __this__nativeId);
/**
* See QWizard::testOption(QWizard::WizardOption)const
*/
@io.qt.QtUninvokable
public final boolean testOption(io.qt.widgets.QWizard.WizardOption option){
return testOption_native_QWizard_WizardOption_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), option.value());
}
@io.qt.QtUninvokable
private native boolean testOption_native_QWizard_WizardOption_constfct(long __this__nativeId, int option);
/**
* See QWizard::titleFormat()const
*/
@io.qt.QtPropertyReader(name="titleFormat")
@io.qt.QtUninvokable
public final io.qt.core.Qt.TextFormat titleFormat(){
return io.qt.core.Qt.TextFormat.resolve(titleFormat_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int titleFormat_native_constfct(long __this__nativeId);
/**
* See QWizard::visitedIds()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList visitedIds(){
return visitedIds_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList visitedIds_native_constfct(long __this__nativeId);
/**
* See QWizard::wizardStyle()const
*/
@io.qt.QtPropertyReader(name="wizardStyle")
@io.qt.QtUninvokable
public final io.qt.widgets.QWizard.WizardStyle wizardStyle(){
return io.qt.widgets.QWizard.WizardStyle.resolve(wizardStyle_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int wizardStyle_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
protected void cleanupPage(int id){
cleanupPage_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@io.qt.QtUninvokable
private native void cleanupPage_native_int(long __this__nativeId, int id);
/**
*
*/
@io.qt.QtUninvokable
public void done(int result){
done_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), result);
}
@io.qt.QtUninvokable
private native void done_native_int(long __this__nativeId, int result);
/**
*
*/
@io.qt.QtUninvokable
public boolean event(io.qt.core.QEvent event){
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long event);
/**
* See QWizard::initializePage(int)
*/
@io.qt.QtUninvokable
protected void initializePage(int id){
initializePage_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@io.qt.QtUninvokable
private native void initializePage_native_int(long __this__nativeId, int id);
/**
*
*/
@io.qt.QtUninvokable
public int nextId(){
return nextId_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int nextId_native_constfct(long __this__nativeId);
/**
* See QWidget::paintEvent(QPaintEvent*)
*/
@io.qt.QtUninvokable
protected void paintEvent(io.qt.gui.QPaintEvent event){
paintEvent_native_QPaintEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void paintEvent_native_QPaintEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::resizeEvent(QResizeEvent*)
*/
@io.qt.QtUninvokable
protected void resizeEvent(io.qt.gui.QResizeEvent event){
resizeEvent_native_QResizeEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native void resizeEvent_native_QResizeEvent_ptr(long __this__nativeId, long event);
/**
*
*/
@io.qt.QtUninvokable
public void setVisible(boolean visible){
setVisible_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), visible);
}
@io.qt.QtUninvokable
private native void setVisible_native_bool(long __this__nativeId, boolean visible);
/**
*
*/
@io.qt.QtUninvokable
public io.qt.core.QSize sizeHint(){
return sizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QSize sizeHint_native_constfct(long __this__nativeId);
/**
* See QWizard::validateCurrentPage()
*/
@io.qt.QtUninvokable
public boolean validateCurrentPage(){
return validateCurrentPage_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean validateCurrentPage_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QWizard(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QWizard(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QWizard instance, QDeclarativeConstructor constructor);
}