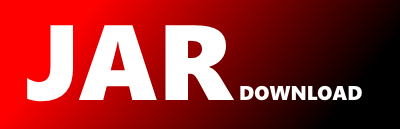
io.qt.multimedia.QMediaCaptureSession Maven / Gradle / Ivy
Show all versions of qtjambi-multimedia Show documentation
package io.qt.multimedia;
import io.qt.*;
/**
* Allows capturing of audio and video content
* Java wrapper for Qt class QMediaCaptureSession
*/
public class QMediaCaptureSession extends io.qt.core.QObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
@QtPropertyMember(enabled=false)
private Object __rcAudioBufferInput;
@QtPropertyMember(enabled=false)
private Object __rcAudioInput;
@QtPropertyMember(enabled=false)
private Object __rcAudioOutput;
@QtPropertyMember(enabled=false)
private Object __rcCamera;
@QtPropertyMember(enabled=false)
private Object __rcImageCapture;
@QtPropertyMember(enabled=false)
private Object __rcRecorder;
@QtPropertyMember(enabled=false)
private Object __rcScreenCapture;
@QtPropertyMember(enabled=false)
private Object __rcVideoFrameInput;
@QtPropertyMember(enabled=false)
private Object __rcVideoOutput;
@QtPropertyMember(enabled=false)
private Object __rcVideoSink;
@QtPropertyMember(enabled=false)
private Object __rcWindowCapture;
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QMediaCaptureSession.class);
/**
* See QMediaCaptureSession:: audioBufferInputChanged()
*/
@QtPropertyNotify(name="audioBufferInput")
public final @NonNull Signal0 audioBufferInputChanged = new Signal0();
/**
* See QMediaCaptureSession:: audioInputChanged()
*/
@QtPropertyNotify(name="audioInput")
public final @NonNull Signal0 audioInputChanged = new Signal0();
/**
* See QMediaCaptureSession:: audioOutputChanged()
*/
@QtPropertyNotify(name="audioOutput")
public final @NonNull Signal0 audioOutputChanged = new Signal0();
/**
* See QMediaCaptureSession:: cameraChanged()
*/
@QtPropertyNotify(name="camera")
public final @NonNull Signal0 cameraChanged = new Signal0();
/**
* See QMediaCaptureSession:: imageCaptureChanged()
*/
@QtPropertyNotify(name="imageCapture")
public final @NonNull Signal0 imageCaptureChanged = new Signal0();
/**
* See QMediaCaptureSession:: recorderChanged()
*/
@QtPropertyNotify(name="recorder")
public final @NonNull Signal0 recorderChanged = new Signal0();
/**
* See QMediaCaptureSession:: screenCaptureChanged()
*/
@QtPropertyNotify(name="screenCapture")
public final @NonNull Signal0 screenCaptureChanged = new Signal0();
/**
* See QMediaCaptureSession:: videoFrameInputChanged()
*/
@QtPropertyNotify(name="videoFrameInput")
public final @NonNull Signal0 videoFrameInputChanged = new Signal0();
/**
* See QMediaCaptureSession:: videoOutputChanged()
*/
@QtPropertyNotify(name="videoOutput")
public final @NonNull Signal0 videoOutputChanged = new Signal0();
/**
* See QMediaCaptureSession:: windowCaptureChanged()
*/
@QtPropertyNotify(name="windowCapture")
public final @NonNull Signal0 windowCaptureChanged = new Signal0();
/**
* See QMediaCaptureSession:: QMediaCaptureSession(QObject*)
* @param parent
*/
public QMediaCaptureSession(io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QMediaCaptureSession instance, io.qt.core.QObject parent);
/**
* See QMediaCaptureSession:: audioBufferInput()const
* @return
*/
@QtPropertyReader(name="audioBufferInput")
@QtUninvokable
public final io.qt.multimedia.@Nullable QAudioBufferInput audioBufferInput(){
return audioBufferInput_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QAudioBufferInput audioBufferInput_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: audioInput()const
* @return
*/
@QtPropertyReader(name="audioInput")
@QtUninvokable
public final io.qt.multimedia.@Nullable QAudioInput audioInput(){
return audioInput_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QAudioInput audioInput_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: audioOutput()const
* @return
*/
@QtPropertyReader(name="audioOutput")
@QtUninvokable
public final io.qt.multimedia.@Nullable QAudioOutput audioOutput(){
return audioOutput_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QAudioOutput audioOutput_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: camera()const
* @return
*/
@QtPropertyReader(name="camera")
@QtUninvokable
public final io.qt.multimedia.@Nullable QCamera camera(){
return camera_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QCamera camera_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: imageCapture()
* @return
*/
@QtPropertyReader(name="imageCapture")
@QtUninvokable
public final io.qt.multimedia.@Nullable QImageCapture imageCapture(){
return imageCapture_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QImageCapture imageCapture_native(long __this__nativeId);
/**
* See QMediaCaptureSession:: recorder()
* @return
*/
@QtPropertyReader(name="recorder")
@QtUninvokable
public final io.qt.multimedia.@Nullable QMediaRecorder recorder(){
return recorder_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QMediaRecorder recorder_native(long __this__nativeId);
/**
* See QMediaCaptureSession:: screenCapture()
* @return
*/
@QtPropertyReader(name="screenCapture")
@QtUninvokable
public final io.qt.multimedia.@Nullable QScreenCapture screenCapture(){
return screenCapture_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QScreenCapture screenCapture_native(long __this__nativeId);
/**
* See QMediaCaptureSession:: setAudioBufferInput(QAudioBufferInput*)
* @param input
*/
@QtPropertyWriter(name="audioBufferInput")
@QtUninvokable
public final void setAudioBufferInput(io.qt.multimedia.@Nullable QAudioBufferInput input){
InternalAccess.NativeIdInfo __input__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(input);
setAudioBufferInput_native_QAudioBufferInput_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __input__NativeIdInfo.nativeId());
if (__input__NativeIdInfo.needsReferenceCounting()) {
__rcAudioBufferInput = input;
}else{
__rcAudioBufferInput = null;
}
}
@QtUninvokable
private native void setAudioBufferInput_native_QAudioBufferInput_ptr(long __this__nativeId, long input);
/**
* See QMediaCaptureSession:: setAudioInput(QAudioInput*)
* @param input
*/
@QtPropertyWriter(name="audioInput")
@QtUninvokable
public final void setAudioInput(io.qt.multimedia.@Nullable QAudioInput input){
InternalAccess.NativeIdInfo __input__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(input);
setAudioInput_native_QAudioInput_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __input__NativeIdInfo.nativeId());
if (__input__NativeIdInfo.needsReferenceCounting()) {
__rcAudioInput = input;
}else{
__rcAudioInput = null;
}
}
@QtUninvokable
private native void setAudioInput_native_QAudioInput_ptr(long __this__nativeId, long input);
/**
* See QMediaCaptureSession:: setAudioOutput(QAudioOutput*)
* @param output
*/
@QtPropertyWriter(name="audioOutput")
@QtUninvokable
public final void setAudioOutput(io.qt.multimedia.@Nullable QAudioOutput output){
InternalAccess.NativeIdInfo __output__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(output);
setAudioOutput_native_QAudioOutput_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __output__NativeIdInfo.nativeId());
if (__output__NativeIdInfo.needsReferenceCounting()) {
__rcAudioOutput = output;
}else{
__rcAudioOutput = null;
}
}
@QtUninvokable
private native void setAudioOutput_native_QAudioOutput_ptr(long __this__nativeId, long output);
/**
* See QMediaCaptureSession:: setCamera(QCamera*)
* @param camera
*/
@QtPropertyWriter(name="camera")
@QtUninvokable
public final void setCamera(io.qt.multimedia.@Nullable QCamera camera){
InternalAccess.NativeIdInfo __camera__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(camera);
setCamera_native_QCamera_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __camera__NativeIdInfo.nativeId());
if (__camera__NativeIdInfo.needsReferenceCounting()) {
__rcCamera = camera;
}else{
__rcCamera = null;
}
}
@QtUninvokable
private native void setCamera_native_QCamera_ptr(long __this__nativeId, long camera);
/**
* See QMediaCaptureSession:: setImageCapture(QImageCapture*)
* @param imageCapture
*/
@QtPropertyWriter(name="imageCapture")
@QtUninvokable
public final void setImageCapture(io.qt.multimedia.@Nullable QImageCapture imageCapture){
InternalAccess.NativeIdInfo __imageCapture__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(imageCapture);
setImageCapture_native_QImageCapture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __imageCapture__NativeIdInfo.nativeId());
if (__imageCapture__NativeIdInfo.needsReferenceCounting()) {
__rcImageCapture = imageCapture;
}else{
__rcImageCapture = null;
}
}
@QtUninvokable
private native void setImageCapture_native_QImageCapture_ptr(long __this__nativeId, long imageCapture);
/**
* See QMediaCaptureSession:: setRecorder(QMediaRecorder*)
* @param recorder
*/
@QtPropertyWriter(name="recorder")
@QtUninvokable
public final void setRecorder(io.qt.multimedia.@Nullable QMediaRecorder recorder){
InternalAccess.NativeIdInfo __recorder__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(recorder);
setRecorder_native_QMediaRecorder_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __recorder__NativeIdInfo.nativeId());
if (__recorder__NativeIdInfo.needsReferenceCounting()) {
__rcRecorder = recorder;
}else{
__rcRecorder = null;
}
}
@QtUninvokable
private native void setRecorder_native_QMediaRecorder_ptr(long __this__nativeId, long recorder);
/**
* See QMediaCaptureSession:: setScreenCapture(QScreenCapture*)
* @param screenCapture
*/
@QtPropertyWriter(name="screenCapture")
@QtUninvokable
public final void setScreenCapture(io.qt.multimedia.@Nullable QScreenCapture screenCapture){
InternalAccess.NativeIdInfo __screenCapture__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(screenCapture);
setScreenCapture_native_QScreenCapture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __screenCapture__NativeIdInfo.nativeId());
if (__screenCapture__NativeIdInfo.needsReferenceCounting()) {
__rcScreenCapture = screenCapture;
}else{
__rcScreenCapture = null;
}
}
@QtUninvokable
private native void setScreenCapture_native_QScreenCapture_ptr(long __this__nativeId, long screenCapture);
/**
* See QMediaCaptureSession:: setVideoFrameInput(QVideoFrameInput*)
* @param input
*/
@QtPropertyWriter(name="videoFrameInput")
@QtUninvokable
public final void setVideoFrameInput(io.qt.multimedia.@Nullable QVideoFrameInput input){
InternalAccess.NativeIdInfo __input__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(input);
setVideoFrameInput_native_QVideoFrameInput_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __input__NativeIdInfo.nativeId());
if (__input__NativeIdInfo.needsReferenceCounting()) {
__rcVideoFrameInput = input;
}else{
__rcVideoFrameInput = null;
}
}
@QtUninvokable
private native void setVideoFrameInput_native_QVideoFrameInput_ptr(long __this__nativeId, long input);
/**
* See QMediaCaptureSession:: setVideoOutput(QObject*)
* @param output
*/
@QtPropertyWriter(name="videoOutput")
@QtUninvokable
public final void setVideoOutput(io.qt.core.@Nullable QObject output){
InternalAccess.NativeIdInfo __output__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(output);
setVideoOutput_native_QObject_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __output__NativeIdInfo.nativeId());
if (__output__NativeIdInfo.needsReferenceCounting()) {
__rcVideoOutput = output;
}else{
__rcVideoOutput = null;
}
}
@QtUninvokable
private native void setVideoOutput_native_QObject_ptr(long __this__nativeId, long output);
/**
* See QMediaCaptureSession:: setVideoSink(QVideoSink*)
* @param sink
*/
@QtUninvokable
public final void setVideoSink(io.qt.multimedia.@Nullable QVideoSink sink){
InternalAccess.NativeIdInfo __sink__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(sink);
setVideoSink_native_QVideoSink_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __sink__NativeIdInfo.nativeId());
if (__sink__NativeIdInfo.needsReferenceCounting()) {
__rcVideoSink = sink;
}else{
__rcVideoSink = null;
}
}
@QtUninvokable
private native void setVideoSink_native_QVideoSink_ptr(long __this__nativeId, long sink);
/**
* See QMediaCaptureSession:: setWindowCapture(QWindowCapture*)
* @param windowCapture
*/
@QtPropertyWriter(name="windowCapture")
@QtUninvokable
public final void setWindowCapture(io.qt.multimedia.@Nullable QWindowCapture windowCapture){
InternalAccess.NativeIdInfo __windowCapture__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(windowCapture);
setWindowCapture_native_QWindowCapture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __windowCapture__NativeIdInfo.nativeId());
if (__windowCapture__NativeIdInfo.needsReferenceCounting()) {
__rcWindowCapture = windowCapture;
}else{
__rcWindowCapture = null;
}
}
@QtUninvokable
private native void setWindowCapture_native_QWindowCapture_ptr(long __this__nativeId, long windowCapture);
/**
* See QMediaCaptureSession:: videoFrameInput()const
* @return
*/
@QtPropertyReader(name="videoFrameInput")
@QtUninvokable
public final io.qt.multimedia.@Nullable QVideoFrameInput videoFrameInput(){
return videoFrameInput_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QVideoFrameInput videoFrameInput_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: videoOutput()const
* @return
*/
@QtPropertyReader(name="videoOutput")
@QtUninvokable
public final io.qt.core.@Nullable QObject videoOutput(){
return videoOutput_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QObject videoOutput_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: videoSink()const
* @return
*/
@QtUninvokable
public final io.qt.multimedia.@Nullable QVideoSink videoSink(){
return videoSink_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QVideoSink videoSink_native_constfct(long __this__nativeId);
/**
* See QMediaCaptureSession:: windowCapture()
* @return
*/
@QtPropertyReader(name="windowCapture")
@QtUninvokable
public final io.qt.multimedia.@Nullable QWindowCapture windowCapture(){
return windowCapture_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QWindowCapture windowCapture_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QMediaCaptureSession(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QMediaCaptureSession(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QMediaCaptureSession instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QMediaCaptureSession(io.qt.core.QObject)}
* with parent = null
.
*/
public QMediaCaptureSession() {
this((io.qt.core.QObject)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #audioBufferInput()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QAudioBufferInput getAudioBufferInput() {
return audioBufferInput();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #audioInput()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QAudioInput getAudioInput() {
return audioInput();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #audioOutput()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QAudioOutput getAudioOutput() {
return audioOutput();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #camera()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QCamera getCamera() {
return camera();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #imageCapture()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QImageCapture getImageCapture() {
return imageCapture();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #recorder()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QMediaRecorder getRecorder() {
return recorder();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #screenCapture()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QScreenCapture getScreenCapture() {
return screenCapture();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #videoFrameInput()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QVideoFrameInput getVideoFrameInput() {
return videoFrameInput();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #videoOutput()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@Nullable QObject getVideoOutput() {
return videoOutput();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #windowCapture()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@Nullable QWindowCapture getWindowCapture() {
return windowCapture();
}
}