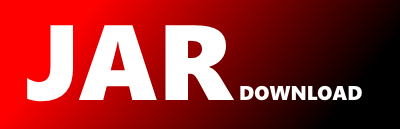
io.qt.multimedia.QMediaFormat Maven / Gradle / Ivy
Show all versions of qtjambi-multimedia Show documentation
package io.qt.multimedia;
import io.qt.*;
/**
* Describes an encoding format for a multimedia file or stream
* Java wrapper for Qt class QMediaFormat
*/
public class QMediaFormat extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QMediaFormat.class);
/**
* Java wrapper for Qt enum QMediaFormat::AudioCodec
*/
@QtRejectedEntries({"LastAudioCodec"})
public enum AudioCodec implements QtEnumerator {
/**
* Representing QMediaFormat:: AudioCodec:: Unspecified
*/
Unspecified(-1),
/**
* Representing QMediaFormat:: AudioCodec:: MP3
*/
MP3(0),
/**
* Representing QMediaFormat:: AudioCodec:: AAC
*/
AAC(1),
/**
* Representing QMediaFormat:: AudioCodec:: AC3
*/
AC3(2),
/**
* Representing QMediaFormat:: AudioCodec:: EAC3
*/
EAC3(3),
/**
* Representing QMediaFormat:: AudioCodec:: FLAC
*/
FLAC(4),
/**
* Representing QMediaFormat:: AudioCodec:: DolbyTrueHD
*/
DolbyTrueHD(5),
/**
* Representing QMediaFormat:: AudioCodec:: Opus
*/
Opus(6),
/**
* Representing QMediaFormat:: AudioCodec:: Vorbis
*/
Vorbis(7),
/**
* Representing QMediaFormat:: AudioCodec:: Wave
*/
Wave(8),
/**
* Representing QMediaFormat:: AudioCodec:: WMA
*/
WMA(9),
/**
* Representing QMediaFormat:: AudioCodec:: ALAC
*/
ALAC(10),
/**
* Representing QMediaFormat:: AudioCodec:: LastAudioCodec
*/
LastAudioCodec(10);
static {
QtJambi_LibraryUtilities.initialize();
}
private AudioCodec(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull AudioCodec resolve(int value) {
switch (value) {
case -1: return Unspecified;
case 0: return MP3;
case 1: return AAC;
case 2: return AC3;
case 3: return EAC3;
case 4: return FLAC;
case 5: return DolbyTrueHD;
case 6: return Opus;
case 7: return Vorbis;
case 8: return Wave;
case 9: return WMA;
case 10: return ALAC;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QMediaFormat::ConversionMode
*/
public enum ConversionMode implements QtEnumerator {
/**
* Representing QMediaFormat:: Encode
*/
Encode(0),
/**
* Representing QMediaFormat:: Decode
*/
Decode(1);
static {
QtJambi_LibraryUtilities.initialize();
}
private ConversionMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull ConversionMode resolve(int value) {
switch (value) {
case 0: return Encode;
case 1: return Decode;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QMediaFormat::FileFormat
*/
@QtRejectedEntries({"LastFileFormat"})
public enum FileFormat implements QtEnumerator {
/**
* Representing QMediaFormat:: UnspecifiedFormat
*/
UnspecifiedFormat(-1),
/**
* Representing QMediaFormat:: WMV
*/
WMV(0),
/**
* Representing QMediaFormat:: AVI
*/
AVI(1),
/**
* Representing QMediaFormat:: Matroska
*/
Matroska(2),
/**
* Representing QMediaFormat:: MPEG4
*/
MPEG4(3),
/**
* Representing QMediaFormat:: Ogg
*/
Ogg(4),
/**
* Representing QMediaFormat:: QuickTime
*/
QuickTime(5),
/**
* Representing QMediaFormat:: WebM
*/
WebM(6),
/**
* Representing QMediaFormat:: Mpeg4Audio
*/
Mpeg4Audio(7),
/**
* Representing QMediaFormat:: AAC
*/
AAC(8),
/**
* Representing QMediaFormat:: WMA
*/
WMA(9),
/**
* Representing QMediaFormat:: MP3
*/
MP3(10),
/**
* Representing QMediaFormat:: FLAC
*/
FLAC(11),
/**
* Representing QMediaFormat:: Wave
*/
Wave(12),
/**
* Representing QMediaFormat:: LastFileFormat
*/
LastFileFormat(12);
static {
QtJambi_LibraryUtilities.initialize();
}
private FileFormat(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull FileFormat resolve(int value) {
switch (value) {
case -1: return UnspecifiedFormat;
case 0: return WMV;
case 1: return AVI;
case 2: return Matroska;
case 3: return MPEG4;
case 4: return Ogg;
case 5: return QuickTime;
case 6: return WebM;
case 7: return Mpeg4Audio;
case 8: return AAC;
case 9: return WMA;
case 10: return MP3;
case 11: return FLAC;
case 12: return Wave;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QMediaFormat::ResolveFlags
*/
public enum ResolveFlags implements QtEnumerator {
/**
* Representing QMediaFormat:: NoFlags
*/
NoFlags(0),
/**
* Representing QMediaFormat:: RequiresVideo
*/
RequiresVideo(1);
static {
QtJambi_LibraryUtilities.initialize();
}
private ResolveFlags(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull ResolveFlags resolve(int value) {
switch (value) {
case 0: return NoFlags;
case 1: return RequiresVideo;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QMediaFormat::VideoCodec
*/
@QtRejectedEntries({"LastVideoCodec"})
public enum VideoCodec implements QtEnumerator {
/**
* Representing QMediaFormat:: VideoCodec:: Unspecified
*/
Unspecified(-1),
/**
* Representing QMediaFormat:: VideoCodec:: MPEG1
*/
MPEG1(0),
/**
* Representing QMediaFormat:: VideoCodec:: MPEG2
*/
MPEG2(1),
/**
* Representing QMediaFormat:: VideoCodec:: MPEG4
*/
MPEG4(2),
/**
* Representing QMediaFormat:: VideoCodec:: H264
*/
H264(3),
/**
* Representing QMediaFormat:: VideoCodec:: H265
*/
H265(4),
/**
* Representing QMediaFormat:: VideoCodec:: VP8
*/
VP8(5),
/**
* Representing QMediaFormat:: VideoCodec:: VP9
*/
VP9(6),
/**
* Representing QMediaFormat:: VideoCodec:: AV1
*/
AV1(7),
/**
* Representing QMediaFormat:: VideoCodec:: Theora
*/
Theora(8),
/**
* Representing QMediaFormat:: VideoCodec:: WMV
*/
WMV(9),
/**
* Representing QMediaFormat:: VideoCodec:: MotionJPEG
*/
MotionJPEG(10),
/**
* Representing QMediaFormat:: VideoCodec:: LastVideoCodec
*/
LastVideoCodec(10);
static {
QtJambi_LibraryUtilities.initialize();
}
private VideoCodec(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull VideoCodec resolve(int value) {
switch (value) {
case -1: return Unspecified;
case 0: return MPEG1;
case 1: return MPEG2;
case 2: return MPEG4;
case 3: return H264;
case 4: return H265;
case 5: return VP8;
case 6: return VP9;
case 7: return AV1;
case 8: return Theora;
case 9: return WMV;
case 10: return MotionJPEG;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QMediaFormat:: QMediaFormat(QMediaFormat)
* @param other
*/
public QMediaFormat(io.qt.multimedia.@NonNull QMediaFormat other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QMediaFormat instance, io.qt.multimedia.QMediaFormat other);
/**
* See QMediaFormat:: QMediaFormat(QMediaFormat::FileFormat)
* @param format
*/
public QMediaFormat(io.qt.multimedia.QMediaFormat.@NonNull FileFormat format){
super((QPrivateConstructor)null);
initialize_native(this, format);
}
private native static void initialize_native(QMediaFormat instance, io.qt.multimedia.QMediaFormat.FileFormat format);
/**
* See QMediaFormat:: audioCodec()const
* @return
*/
@QtPropertyReader(name="audioCodec")
@QtUninvokable
public final io.qt.multimedia.QMediaFormat.@NonNull AudioCodec audioCodec(){
return io.qt.multimedia.QMediaFormat.AudioCodec.resolve(audioCodec_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int audioCodec_native_constfct(long __this__nativeId);
/**
* See QMediaFormat:: fileFormat()const
* @return
*/
@QtPropertyReader(name="fileFormat")
@QtUninvokable
public final io.qt.multimedia.QMediaFormat.@NonNull FileFormat fileFormat(){
return io.qt.multimedia.QMediaFormat.FileFormat.resolve(fileFormat_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int fileFormat_native_constfct(long __this__nativeId);
/**
* See QMediaFormat:: isSupported(QMediaFormat::ConversionMode)const
* @param mode
* @return
*/
public final boolean isSupported(io.qt.multimedia.QMediaFormat.@NonNull ConversionMode mode){
return isSupported_native_QMediaFormat_ConversionMode_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), mode.value());
}
private native boolean isSupported_native_QMediaFormat_ConversionMode_constfct(long __this__nativeId, int mode);
/**
* See QMediaFormat:: mimeType()const
* @return
*/
@QtUninvokable
public final io.qt.core.@NonNull QMimeType mimeType(){
return mimeType_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QMimeType mimeType_native_constfct(long __this__nativeId);
/**
* See QMediaFormat:: operator=(QMediaFormat)
* @param other
*/
@QtUninvokable
public final void assign(io.qt.multimedia.@NonNull QMediaFormat other){
assign_native_cref_QMediaFormat(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void assign_native_cref_QMediaFormat(long __this__nativeId, long other);
/**
* See QMediaFormat:: operator==(QMediaFormat)const
* @param other
* @return
*/
@QtUninvokable
public final boolean equals(io.qt.multimedia.@NonNull QMediaFormat other){
return equals_native_cref_QMediaFormat_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native boolean equals_native_cref_QMediaFormat_constfct(long __this__nativeId, long other);
/**
* See QMediaFormat:: resolveForEncoding(QMediaFormat::ResolveFlags)
* @param flags
*/
@QtUninvokable
public final void resolveForEncoding(io.qt.multimedia.QMediaFormat.@NonNull ResolveFlags flags){
resolveForEncoding_native_QMediaFormat_ResolveFlags(QtJambi_LibraryUtilities.internal.nativeId(this), flags.value());
}
@QtUninvokable
private native void resolveForEncoding_native_QMediaFormat_ResolveFlags(long __this__nativeId, int flags);
/**
* See QMediaFormat:: setAudioCodec(QMediaFormat::AudioCodec)
* @param codec
*/
@QtPropertyWriter(name="audioCodec")
@QtUninvokable
public final void setAudioCodec(io.qt.multimedia.QMediaFormat.@NonNull AudioCodec codec){
setAudioCodec_native_QMediaFormat_AudioCodec(QtJambi_LibraryUtilities.internal.nativeId(this), codec.value());
}
@QtUninvokable
private native void setAudioCodec_native_QMediaFormat_AudioCodec(long __this__nativeId, int codec);
/**
* See QMediaFormat:: setFileFormat(QMediaFormat::FileFormat)
* @param f
*/
@QtPropertyWriter(name="fileFormat")
@QtUninvokable
public final void setFileFormat(io.qt.multimedia.QMediaFormat.@NonNull FileFormat f){
setFileFormat_native_QMediaFormat_FileFormat(QtJambi_LibraryUtilities.internal.nativeId(this), f.value());
}
@QtUninvokable
private native void setFileFormat_native_QMediaFormat_FileFormat(long __this__nativeId, int f);
/**
* See QMediaFormat:: setVideoCodec(QMediaFormat::VideoCodec)
* @param codec
*/
@QtPropertyWriter(name="videoCodec")
@QtUninvokable
public final void setVideoCodec(io.qt.multimedia.QMediaFormat.@NonNull VideoCodec codec){
setVideoCodec_native_QMediaFormat_VideoCodec(QtJambi_LibraryUtilities.internal.nativeId(this), codec.value());
}
@QtUninvokable
private native void setVideoCodec_native_QMediaFormat_VideoCodec(long __this__nativeId, int codec);
/**
* See QMediaFormat:: supportedAudioCodecs(QMediaFormat::ConversionMode)
* @param m
* @return
*/
public final io.qt.core.@NonNull QList supportedAudioCodecs(io.qt.multimedia.QMediaFormat.@NonNull ConversionMode m){
return supportedAudioCodecs_native_QMediaFormat_ConversionMode(QtJambi_LibraryUtilities.internal.nativeId(this), m.value());
}
private native io.qt.core.QList supportedAudioCodecs_native_QMediaFormat_ConversionMode(long __this__nativeId, int m);
/**
* See QMediaFormat:: supportedFileFormats(QMediaFormat::ConversionMode)
* @param m
* @return
*/
public final io.qt.core.@NonNull QList supportedFileFormats(io.qt.multimedia.QMediaFormat.@NonNull ConversionMode m){
return supportedFileFormats_native_QMediaFormat_ConversionMode(QtJambi_LibraryUtilities.internal.nativeId(this), m.value());
}
private native io.qt.core.QList supportedFileFormats_native_QMediaFormat_ConversionMode(long __this__nativeId, int m);
/**
* See QMediaFormat:: supportedVideoCodecs(QMediaFormat::ConversionMode)
* @param m
* @return
*/
public final io.qt.core.@NonNull QList supportedVideoCodecs(io.qt.multimedia.QMediaFormat.@NonNull ConversionMode m){
return supportedVideoCodecs_native_QMediaFormat_ConversionMode(QtJambi_LibraryUtilities.internal.nativeId(this), m.value());
}
private native io.qt.core.QList supportedVideoCodecs_native_QMediaFormat_ConversionMode(long __this__nativeId, int m);
/**
* See QMediaFormat:: swap(QMediaFormat&)
* @param other
*/
@QtUninvokable
public final void swap(io.qt.multimedia.@StrictNonNull QMediaFormat other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QMediaFormat(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void swap_native_ref_QMediaFormat(long __this__nativeId, long other);
/**
* See QMediaFormat:: videoCodec()const
* @return
*/
@QtPropertyReader(name="videoCodec")
@QtUninvokable
public final io.qt.multimedia.QMediaFormat.@NonNull VideoCodec videoCodec(){
return io.qt.multimedia.QMediaFormat.VideoCodec.resolve(videoCodec_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int videoCodec_native_constfct(long __this__nativeId);
/**
* See QMediaFormat:: audioCodecDescription(QMediaFormat::AudioCodec)
* @param codec
* @return
*/
public static java.lang.@NonNull String audioCodecDescription(io.qt.multimedia.QMediaFormat.@NonNull AudioCodec codec){
return audioCodecDescription_native_QMediaFormat_AudioCodec(codec.value());
}
private native static java.lang.String audioCodecDescription_native_QMediaFormat_AudioCodec(int codec);
/**
* See QMediaFormat:: audioCodecName(QMediaFormat::AudioCodec)
* @param codec
* @return
*/
public static java.lang.@NonNull String audioCodecName(io.qt.multimedia.QMediaFormat.@NonNull AudioCodec codec){
return audioCodecName_native_QMediaFormat_AudioCodec(codec.value());
}
private native static java.lang.String audioCodecName_native_QMediaFormat_AudioCodec(int codec);
/**
* See QMediaFormat:: fileFormatDescription(QMediaFormat::FileFormat)
* @param fileFormat
* @return
*/
public static java.lang.@NonNull String fileFormatDescription(io.qt.multimedia.QMediaFormat.@NonNull FileFormat fileFormat){
return fileFormatDescription_native_QMediaFormat_FileFormat(fileFormat.value());
}
private native static java.lang.String fileFormatDescription_native_QMediaFormat_FileFormat(int fileFormat);
/**
* See QMediaFormat:: fileFormatName(QMediaFormat::FileFormat)
* @param fileFormat
* @return
*/
public static java.lang.@NonNull String fileFormatName(io.qt.multimedia.QMediaFormat.@NonNull FileFormat fileFormat){
return fileFormatName_native_QMediaFormat_FileFormat(fileFormat.value());
}
private native static java.lang.String fileFormatName_native_QMediaFormat_FileFormat(int fileFormat);
/**
* See QMediaFormat:: videoCodecDescription(QMediaFormat::VideoCodec)
* @param codec
* @return
*/
public static java.lang.@NonNull String videoCodecDescription(io.qt.multimedia.QMediaFormat.@NonNull VideoCodec codec){
return videoCodecDescription_native_QMediaFormat_VideoCodec(codec.value());
}
private native static java.lang.String videoCodecDescription_native_QMediaFormat_VideoCodec(int codec);
/**
* See QMediaFormat:: videoCodecName(QMediaFormat::VideoCodec)
* @param codec
* @return
*/
public static java.lang.@NonNull String videoCodecName(io.qt.multimedia.QMediaFormat.@NonNull VideoCodec codec){
return videoCodecName_native_QMediaFormat_VideoCodec(codec.value());
}
private native static java.lang.String videoCodecName_native_QMediaFormat_VideoCodec(int codec);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QMediaFormat(QPrivateConstructor p) { super(p); }
/**
* See QMediaFormat:: operator==(QMediaFormat)const
*/
@Override
@QtUninvokable
public boolean equals(Object other) {
if (other==null || other instanceof io.qt.multimedia.QMediaFormat) {
return equals((io.qt.multimedia.QMediaFormat) other);
}
return false;
}
/**
* Returns the objects's hash code computed by qHash(QMediaFormat)
.
*/
@QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native static int hashCode_native(long __this_nativeId);
/**
* Creates and returns a copy of this object.
See QMediaFormat:: QMediaFormat(QMediaFormat)
*/
@QtUninvokable
@Override
public QMediaFormat clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native QMediaFormat clone_native(long __this_nativeId);
/**
* Overloaded constructor for {@link #QMediaFormat(io.qt.multimedia.QMediaFormat.FileFormat)}
* with format = io.qt.multimedia.QMediaFormat.FileFormat.UnspecifiedFormat
.
*/
public QMediaFormat() {
this(io.qt.multimedia.QMediaFormat.FileFormat.UnspecifiedFormat);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #audioCodec()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.QMediaFormat.@NonNull AudioCodec getAudioCodec() {
return audioCodec();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #fileFormat()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.QMediaFormat.@NonNull FileFormat getFileFormat() {
return fileFormat();
}
/**
* Overloaded function for {@link #assign(io.qt.multimedia.QMediaFormat)}.
*/
@QtUninvokable
public final void assign(io.qt.multimedia.QMediaFormat.@NonNull FileFormat other) {
assign(new io.qt.multimedia.QMediaFormat(other));
}
/**
* Overloaded function for {@link #equals(io.qt.multimedia.QMediaFormat)}.
*/
@QtUninvokable
public final boolean equals(io.qt.multimedia.QMediaFormat.@NonNull FileFormat other) {
return equals(new io.qt.multimedia.QMediaFormat(other));
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #videoCodec()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.QMediaFormat.@NonNull VideoCodec getVideoCodec() {
return videoCodec();
}
}