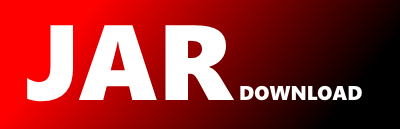
io.qt.multimedia.QSoundEffect Maven / Gradle / Ivy
Show all versions of qtjambi-multimedia Show documentation
package io.qt.multimedia;
import io.qt.*;
/**
* Way to play low latency sound effects
* Java wrapper for Qt class QSoundEffect
*/
public class QSoundEffect extends io.qt.core.QObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QSoundEffect.class);
/**
* Java wrapper for Qt enum QSoundEffect::Loop
*/
public enum Loop implements QtEnumerator {
/**
* Representing QSoundEffect:: Infinite
*/
Infinite(-2);
static {
QtJambi_LibraryUtilities.initialize();
}
private Loop(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Loop resolve(int value) {
switch (value) {
case -2: return Infinite;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QSoundEffect::Status
*/
public enum Status implements QtEnumerator {
/**
* Representing QSoundEffect:: Null
*/
Null(0),
/**
* Representing QSoundEffect:: Loading
*/
Loading(1),
/**
* Representing QSoundEffect:: Ready
*/
Ready(2),
/**
* Representing QSoundEffect:: Error
*/
Error(3);
static {
QtJambi_LibraryUtilities.initialize();
}
private Status(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Status resolve(int value) {
switch (value) {
case 0: return Null;
case 1: return Loading;
case 2: return Ready;
case 3: return Error;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QSoundEffect:: audioDeviceChanged()
*/
@QtPropertyNotify(name="audioDevice")
public final @NonNull Signal0 audioDeviceChanged = new Signal0();
/**
* See QSoundEffect:: loadedChanged()
*/
public final @NonNull Signal0 loadedChanged = new Signal0();
/**
* See QSoundEffect:: loopCountChanged()
*/
@QtPropertyNotify(name="loops")
public final @NonNull Signal0 loopCountChanged = new Signal0();
/**
* See QSoundEffect:: loopsRemainingChanged()
*/
@QtPropertyNotify(name="loopsRemaining")
public final @NonNull Signal0 loopsRemainingChanged = new Signal0();
/**
* See QSoundEffect:: mutedChanged()
*/
@QtPropertyNotify(name="muted")
public final @NonNull Signal0 mutedChanged = new Signal0();
/**
* See QSoundEffect:: playingChanged()
*/
@QtPropertyNotify(name="playing")
public final @NonNull Signal0 playingChanged = new Signal0();
/**
* See QSoundEffect:: sourceChanged()
*/
@QtPropertyNotify(name="source")
public final @NonNull Signal0 sourceChanged = new Signal0();
/**
* See QSoundEffect:: statusChanged()
*/
@QtPropertyNotify(name="status")
public final @NonNull Signal0 statusChanged = new Signal0();
/**
* See QSoundEffect:: volumeChanged()
*/
@QtPropertyNotify(name="volume")
public final @NonNull Signal0 volumeChanged = new Signal0();
/**
* See QSoundEffect:: QSoundEffect(QAudioDevice, QObject*)
* @param audioDevice
* @param parent
*/
public QSoundEffect(io.qt.multimedia.@NonNull QAudioDevice audioDevice, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, audioDevice, parent);
}
private native static void initialize_native(QSoundEffect instance, io.qt.multimedia.QAudioDevice audioDevice, io.qt.core.QObject parent);
/**
* See QSoundEffect:: QSoundEffect(QObject*)
* @param parent
*/
public QSoundEffect(io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QSoundEffect instance, io.qt.core.QObject parent);
/**
* See QSoundEffect:: audioDevice()
* @return
*/
@QtPropertyReader(name="audioDevice")
@QtUninvokable
public final io.qt.multimedia.@NonNull QAudioDevice audioDevice(){
return audioDevice_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QAudioDevice audioDevice_native(long __this__nativeId);
/**
* See QSoundEffect:: isLoaded()const
* @return
*/
@QtUninvokable
public final boolean isLoaded(){
return isLoaded_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isLoaded_native_constfct(long __this__nativeId);
/**
* See QSoundEffect:: isMuted()const
* @return
*/
@QtPropertyReader(name="muted")
@QtUninvokable
public final boolean isMuted(){
return isMuted_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isMuted_native_constfct(long __this__nativeId);
/**
* See QSoundEffect:: isPlaying()const
* @return
*/
@QtPropertyReader(name="playing")
@QtUninvokable
public final boolean isPlaying(){
return isPlaying_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isPlaying_native_constfct(long __this__nativeId);
/**
* See QSoundEffect:: loopCount()const
* @return
*/
@QtPropertyReader(name="loops")
@QtUninvokable
public final int loopCount(){
return loopCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int loopCount_native_constfct(long __this__nativeId);
/**
* See QSoundEffect:: loopsRemaining()const
* @return
*/
@QtPropertyReader(name="loopsRemaining")
@QtUninvokable
public final int loopsRemaining(){
return loopsRemaining_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int loopsRemaining_native_constfct(long __this__nativeId);
/**
*
*/
public final void play(){
play_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void play_native(long __this__nativeId);
/**
* See QSoundEffect:: setAudioDevice(QAudioDevice)
* @param device
*/
@QtPropertyWriter(name="audioDevice")
@QtUninvokable
public final void setAudioDevice(io.qt.multimedia.@NonNull QAudioDevice device){
setAudioDevice_native_cref_QAudioDevice(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(device));
}
@QtUninvokable
private native void setAudioDevice_native_cref_QAudioDevice(long __this__nativeId, long device);
/**
* See QSoundEffect:: setLoopCount(int)
* @param loopCount
*/
@QtPropertyWriter(name="loops")
@QtUninvokable
public final void setLoopCount(int loopCount){
setLoopCount_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), loopCount);
}
@QtUninvokable
private native void setLoopCount_native_int(long __this__nativeId, int loopCount);
/**
* See QSoundEffect:: setMuted(bool)
* @param muted
*/
@QtPropertyWriter(name="muted")
@QtUninvokable
public final void setMuted(boolean muted){
setMuted_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), muted);
}
@QtUninvokable
private native void setMuted_native_bool(long __this__nativeId, boolean muted);
/**
* See QSoundEffect:: setSource(QUrl)
* @param url
*/
@QtPropertyWriter(name="source")
@QtUninvokable
public final void setSource(io.qt.core.@NonNull QUrl url){
setSource_native_cref_QUrl(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(url));
}
@QtUninvokable
private native void setSource_native_cref_QUrl(long __this__nativeId, long url);
/**
* See QSoundEffect:: setVolume(float)
* @param volume
*/
@QtPropertyWriter(name="volume")
@QtUninvokable
public final void setVolume(float volume){
setVolume_native_float(QtJambi_LibraryUtilities.internal.nativeId(this), volume);
}
@QtUninvokable
private native void setVolume_native_float(long __this__nativeId, float volume);
/**
* See QSoundEffect:: source()const
* @return
*/
@QtPropertyReader(name="source")
@QtUninvokable
public final io.qt.core.@NonNull QUrl source(){
return source_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QUrl source_native_constfct(long __this__nativeId);
/**
* See QSoundEffect:: status()const
* @return
*/
@QtPropertyReader(name="status")
@QtUninvokable
public final io.qt.multimedia.QSoundEffect.@NonNull Status status(){
return io.qt.multimedia.QSoundEffect.Status.resolve(status_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int status_native_constfct(long __this__nativeId);
/**
*
*/
public final void stop(){
stop_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void stop_native(long __this__nativeId);
/**
* See QSoundEffect:: volume()const
* @return
*/
@QtPropertyReader(name="volume")
@QtUninvokable
public final float volume(){
return volume_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native float volume_native_constfct(long __this__nativeId);
/**
* See QSoundEffect:: supportedMimeTypes()
* @return
*/
public native static io.qt.core.@NonNull QStringList supportedMimeTypes();
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QSoundEffect(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QSoundEffect(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QSoundEffect instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QSoundEffect(io.qt.multimedia.QAudioDevice, io.qt.core.QObject)}
* with parent = null
.
*/
public QSoundEffect(io.qt.multimedia.@NonNull QAudioDevice audioDevice) {
this(audioDevice, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QSoundEffect(io.qt.core.QObject)}
* with parent = null
.
*/
public QSoundEffect() {
this((io.qt.core.QObject)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #audioDevice()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.@NonNull QAudioDevice getAudioDevice() {
return audioDevice();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #isMuted()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final boolean getMuted() {
return isMuted();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #isPlaying()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final boolean getPlaying() {
return isPlaying();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #loopCount()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getLoops() {
return loopCount();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #loopsRemaining()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getLoopsRemaining() {
return loopsRemaining();
}
/**
* Overloaded function for {@link #setSource(io.qt.core.QUrl)}.
*/
@QtUninvokable
public final void setSource(java.lang.@NonNull String url) {
setSource(new io.qt.core.QUrl(url));
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #source()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QUrl getSource() {
return source();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #status()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.multimedia.QSoundEffect.@NonNull Status getStatus() {
return status();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #volume()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final float getVolume() {
return volume();
}
}