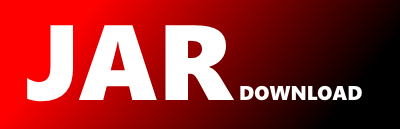
io.qt.multimedia.QWaveDecoder Maven / Gradle / Ivy
Show all versions of qtjambi-multimedia Show documentation
package io.qt.multimedia;
import io.qt.*;
/**
* Java wrapper for Qt class QWaveDecoder
*/
public class QWaveDecoder extends io.qt.core.QIODevice
{
static {
QtJambi_LibraryUtilities.initialize();
}
@QtPropertyMember(enabled=false)
private Object __rcIODevice;
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QWaveDecoder.class);
/**
* See QWaveDecoder:: formatKnown()
*/
public final @NonNull Signal0 formatKnown = new Signal0();
/**
* See QWaveDecoder:: parsingError()
*/
public final @NonNull Signal0 parsingError = new Signal0();
/**
* See QWaveDecoder:: QWaveDecoder(QIODevice*, QAudioFormat, QObject*)
* @param device
* @param format
* @param parent
*/
public QWaveDecoder(io.qt.core.@Nullable QIODevice device, io.qt.multimedia.@NonNull QAudioFormat format, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, device, format, parent);
}
private native static void initialize_native(QWaveDecoder instance, io.qt.core.QIODevice device, io.qt.multimedia.QAudioFormat format, io.qt.core.QObject parent);
/**
* See QWaveDecoder:: QWaveDecoder(QIODevice*, QObject*)
* @param device
* @param parent
*/
public QWaveDecoder(io.qt.core.@Nullable QIODevice device, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, device, parent);
}
private native static void initialize_native(QWaveDecoder instance, io.qt.core.QIODevice device, io.qt.core.QObject parent);
/**
* See QWaveDecoder:: audioFormat()const
* @return
*/
@QtUninvokable
public final io.qt.multimedia.@NonNull QAudioFormat audioFormat(){
return audioFormat_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QAudioFormat audioFormat_native_constfct(long __this__nativeId);
/**
* See QWaveDecoder:: duration()const
* @return
*/
@QtUninvokable
public final int duration(){
return duration_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int duration_native_constfct(long __this__nativeId);
/**
* See QWaveDecoder:: getDevice()
* @return
*/
@QtUninvokable
public final io.qt.core.@Nullable QIODevice getDevice(){
return getDevice_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QIODevice getDevice_native(long __this__nativeId);
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
@QtUninvokable
@Override
protected final int readData(java.nio.@Nullable ByteBuffer data) throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
@QtUninvokable
@Override
protected final int writeData(java.nio.@Nullable ByteBuffer data) throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* See QIODevice:: bytesAvailable()const
* @return
*/
@QtUninvokable
@Override
public long bytesAvailable(){
return bytesAvailable_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long bytesAvailable_native_constfct(long __this__nativeId);
/**
* See QIODevice:: close()
*/
@QtUninvokable
@Override
public void close(){
close_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void close_native(long __this__nativeId);
/**
* See QIODevice:: isSequential()const
* @return
*/
@QtUninvokable
@Override
public boolean isSequential(){
return isSequential_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isSequential_native_constfct(long __this__nativeId);
/**
* See QIODevice:: open(QIODevice::OpenMode)
* @param mode
* @return
*/
@QtUninvokable
@Override
public boolean open(io.qt.core.QIODeviceBase.@NonNull OpenMode mode){
return open_native_QIODeviceBase_OpenMode(QtJambi_LibraryUtilities.internal.nativeId(this), mode.value());
}
@QtUninvokable
private native boolean open_native_QIODeviceBase_OpenMode(long __this__nativeId, int mode);
/**
* See QIODevice:: pos()const
* @return
*/
@QtUninvokable
@Override
public long pos(){
return pos_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long pos_native_constfct(long __this__nativeId);
/**
* See QIODevice:: seek(qint64)
* @param pos
* @return
*/
@QtUninvokable
@Override
public boolean seek(long pos){
return seek_native_qint64(QtJambi_LibraryUtilities.internal.nativeId(this), pos);
}
@QtUninvokable
private native boolean seek_native_qint64(long __this__nativeId, long pos);
/**
* See QIODevice:: size()const
* @return
*/
@QtUninvokable
@Override
public long size(){
return size_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long size_native_constfct(long __this__nativeId);
/**
* See QWaveDecoder:: headerLength()
* @return
*/
public native static long headerLength();
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QWaveDecoder(QPrivateConstructor p) { super(p); }
/**
* Overloaded constructor for {@link #QWaveDecoder(io.qt.core.QIODevice, io.qt.multimedia.QAudioFormat, io.qt.core.QObject)}
* with parent = null
.
*/
public QWaveDecoder(io.qt.core.@Nullable QIODevice device, io.qt.multimedia.@NonNull QAudioFormat format) {
this(device, format, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QWaveDecoder(io.qt.core.QIODevice, io.qt.core.QObject)}
* with parent = null
.
*/
public QWaveDecoder(io.qt.core.@Nullable QIODevice device) {
this(device, (io.qt.core.QObject)null);
}
}