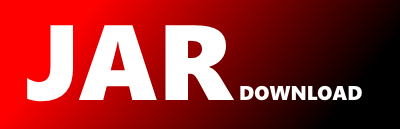
io.qt.multimedia.widgets.QVideoWidget Maven / Gradle / Ivy
Show all versions of qtjambi-multimediawidgets Show documentation
package io.qt.multimedia.widgets;
import io.qt.*;
/**
* Widget which presents video produced by a media object
* Java wrapper for Qt's class QVideoWidget
*/
public class QVideoWidget extends io.qt.widgets.QWidget
implements io.qt.multimedia.QMediaBindableInterface
{
static {
QtJambi_LibraryUtilities.initialize();
}
@QtPropertyMember(enabled=false)
private Object __rcMediaObject = null;
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QVideoWidget.class);
/**
* See QVideoWidget::brightnessChanged(int)
*/
@QtPropertyNotify(name="brightness")
public final @NonNull Signal1 brightnessChanged = new Signal1<>();
/**
* See QVideoWidget::contrastChanged(int)
*/
@QtPropertyNotify(name="contrast")
public final @NonNull Signal1 contrastChanged = new Signal1<>();
/**
* See QVideoWidget::fullScreenChanged(bool)
*/
@QtPropertyNotify(name="fullScreen")
public final @NonNull Signal1 fullScreenChanged = new Signal1<>();
/**
* See QVideoWidget::hueChanged(int)
*/
@QtPropertyNotify(name="hue")
public final @NonNull Signal1 hueChanged = new Signal1<>();
/**
* See QVideoWidget::saturationChanged(int)
*/
@QtPropertyNotify(name="saturation")
public final @NonNull Signal1 saturationChanged = new Signal1<>();
/**
* Overloaded constructor for {@link #QVideoWidget(io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QVideoWidget() {
this((io.qt.widgets.QWidget)null);
}
/**
* See QVideoWidget::QVideoWidget(QWidget*)
*/
public QVideoWidget(io.qt.widgets.@Nullable QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QVideoWidget instance, io.qt.widgets.QWidget parent);
/**
* Kotlin property getter. In Java use {@link #aspectRatioMode()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.Qt.@NonNull AspectRatioMode getAspectRatioMode() {
return aspectRatioMode();
}
/**
* See QVideoWidget::aspectRatioMode()const
*/
@QtPropertyReader(name="aspectRatioMode")
@QtUninvokable
public final io.qt.core.Qt.@NonNull AspectRatioMode aspectRatioMode(){
return io.qt.core.Qt.AspectRatioMode.resolve(aspectRatioMode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int aspectRatioMode_native_constfct(long __this__nativeId);
/**
* Kotlin property getter. In Java use {@link #brightness()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getBrightness() {
return brightness();
}
/**
* See QVideoWidget::brightness()const
*/
@QtPropertyReader(name="brightness")
@QtUninvokable
public final int brightness(){
return brightness_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int brightness_native_constfct(long __this__nativeId);
/**
* Kotlin property getter. In Java use {@link #contrast()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getContrast() {
return contrast();
}
/**
* See QVideoWidget::contrast()const
*/
@QtPropertyReader(name="contrast")
@QtUninvokable
public final int contrast(){
return contrast_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int contrast_native_constfct(long __this__nativeId);
/**
* Kotlin property getter. In Java use {@link #hue()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getHue() {
return hue();
}
/**
*
*/
@QtPropertyReader(name="hue")
@QtUninvokable
public final int hue(){
return hue_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int hue_native_constfct(long __this__nativeId);
/**
* Kotlin property getter. In Java use {@link #saturation()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getSaturation() {
return saturation();
}
/**
* See QVideoWidget::saturation()const
*/
@QtPropertyReader(name="saturation")
@QtUninvokable
public final int saturation(){
return saturation_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int saturation_native_constfct(long __this__nativeId);
/**
* See QVideoWidget::setAspectRatioMode(Qt::AspectRatioMode)
*/
@QtPropertyWriter(name="aspectRatioMode")
public final void setAspectRatioMode(io.qt.core.Qt.@NonNull AspectRatioMode mode){
setAspectRatioMode_native_Qt_AspectRatioMode(QtJambi_LibraryUtilities.internal.nativeId(this), mode.value());
}
private native void setAspectRatioMode_native_Qt_AspectRatioMode(long __this__nativeId, int mode);
/**
* See QVideoWidget::setBrightness(int)
*/
@QtPropertyWriter(name="brightness")
public final void setBrightness(int brightness){
setBrightness_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), brightness);
}
private native void setBrightness_native_int(long __this__nativeId, int brightness);
/**
* See QVideoWidget::setContrast(int)
*/
@QtPropertyWriter(name="contrast")
public final void setContrast(int contrast){
setContrast_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), contrast);
}
private native void setContrast_native_int(long __this__nativeId, int contrast);
/**
* See QVideoWidget::setFullScreen(bool)
*/
@QtPropertyWriter(name="fullScreen")
public final void setFullScreen(boolean fullScreen){
setFullScreen_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), fullScreen);
}
private native void setFullScreen_native_bool(long __this__nativeId, boolean fullScreen);
/**
*
*/
@QtPropertyWriter(name="hue")
public final void setHue(int hue){
setHue_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), hue);
}
private native void setHue_native_int(long __this__nativeId, int hue);
/**
* See QVideoWidget::setSaturation(int)
*/
@QtPropertyWriter(name="saturation")
public final void setSaturation(int saturation){
setSaturation_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), saturation);
}
private native void setSaturation_native_int(long __this__nativeId, int saturation);
/**
* Kotlin property getter. In Java use {@link #videoSurface()} instead.
*/
@QtPropertyReader(enabled=false)
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.multimedia.@Nullable QAbstractVideoSurface getVideoSurface() {
return videoSurface();
}
/**
* See QVideoWidget::videoSurface()const
*/
@QtPropertyReader(name="videoSurface")
@QtPropertyConstant
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.multimedia.@Nullable QAbstractVideoSurface videoSurface(){
return videoSurface_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.multimedia.QAbstractVideoSurface videoSurface_native_constfct(long __this__nativeId);
/**
*
*/
@QtUninvokable
public boolean event(io.qt.core.@Nullable QEvent event){
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::hideEvent(QHideEvent*)
*/
@QtUninvokable
protected void hideEvent(io.qt.gui.@Nullable QHideEvent event){
hideEvent_native_QHideEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void hideEvent_native_QHideEvent_ptr(long __this__nativeId, long event);
/**
* Kotlin property getter. In Java use {@link #mediaObject()} instead.
*/
@QtPropertyReader(enabled=false)
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.multimedia.@Nullable QMediaObject getMediaObject() {
return mediaObject();
}
/**
* See QMediaBindableInterface::mediaObject()const
*/
@QtPropertyReader(name="mediaObject")
@SuppressWarnings({"exports"})
@QtUninvokable
public io.qt.multimedia.@Nullable QMediaObject mediaObject(){
return mediaObject_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static io.qt.multimedia.QMediaObject mediaObject_native_constfct(long __this__nativeId);
/**
* See QWidget::moveEvent(QMoveEvent*)
*/
@QtUninvokable
protected void moveEvent(io.qt.gui.@Nullable QMoveEvent event){
moveEvent_native_QMoveEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void moveEvent_native_QMoveEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::paintEvent(QPaintEvent*)
*/
@QtUninvokable
protected void paintEvent(io.qt.gui.@Nullable QPaintEvent event){
paintEvent_native_QPaintEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void paintEvent_native_QPaintEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget::resizeEvent(QResizeEvent*)
*/
@QtUninvokable
protected void resizeEvent(io.qt.gui.@Nullable QResizeEvent event){
resizeEvent_native_QResizeEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void resizeEvent_native_QResizeEvent_ptr(long __this__nativeId, long event);
/**
* See QMediaBindableInterface::setMediaObject(QMediaObject*)
*/
@QtPropertyWriter(name="mediaObject")
@QtUninvokable
protected boolean setMediaObject(io.qt.multimedia.@Nullable QMediaObject object){
boolean __qt_return_value = setMediaObject_native_QMediaObject_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(object));
__rcMediaObject = object;
return __qt_return_value;
}
private native static boolean setMediaObject_native_QMediaObject_ptr(long __this__nativeId, long object);
/**
* See QWidget::showEvent(QShowEvent*)
*/
@QtUninvokable
protected void showEvent(io.qt.gui.@Nullable QShowEvent event){
showEvent_native_QShowEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void showEvent_native_QShowEvent_ptr(long __this__nativeId, long event);
/**
*
*/
@QtUninvokable
public io.qt.core.@NonNull QSize sizeHint(){
return sizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QSize sizeHint_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@NativeAccess
protected QVideoWidget(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@NativeAccess
protected QVideoWidget(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QVideoWidget instance, QDeclarativeConstructor constructor);
}