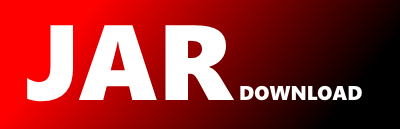
io.qt.multimedia.widgets.QGraphicsVideoItem Maven / Gradle / Ivy
Show all versions of qtjambi-multimediawidgets Show documentation
package io.qt.multimedia.widgets;
import io.qt.*;
/**
* Graphics item which display video produced by a QMediaPlayer or QCamera
* Java wrapper for Qt class QGraphicsVideoItem
*/
public class QGraphicsVideoItem extends io.qt.widgets.QGraphicsObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QGraphicsVideoItem.class);
/**
* See QGraphicsVideoItem:: nativeSizeChanged(QSizeF)
*/
@QtPropertyNotify(name="nativeSize")
public final @NonNull Signal1 nativeSizeChanged = new Signal1<>();
/**
* See QGraphicsVideoItem:: QGraphicsVideoItem(QGraphicsItem*)
*/
public QGraphicsVideoItem(io.qt.widgets.@Nullable QGraphicsItem parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QGraphicsVideoItem instance, io.qt.widgets.QGraphicsItem parent);
/**
* See QGraphicsVideoItem:: aspectRatioMode()const
*/
@QtPropertyReader(name="aspectRatioMode")
@QtUninvokable
public final io.qt.core.Qt.@NonNull AspectRatioMode aspectRatioMode(){
return io.qt.core.Qt.AspectRatioMode.resolve(aspectRatioMode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int aspectRatioMode_native_constfct(long __this__nativeId);
/**
* See QGraphicsVideoItem:: nativeSize()const
*/
@QtPropertyReader(name="nativeSize")
@QtUninvokable
public final io.qt.core.@NonNull QSizeF nativeSize(){
return nativeSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QSizeF nativeSize_native_constfct(long __this__nativeId);
/**
* See QGraphicsVideoItem:: offset()const
*/
@QtPropertyReader(name="offset")
@QtUninvokable
public final io.qt.core.@NonNull QPointF offset(){
return offset_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QPointF offset_native_constfct(long __this__nativeId);
/**
* See QGraphicsVideoItem:: setAspectRatioMode(Qt::AspectRatioMode)
*/
@QtPropertyWriter(name="aspectRatioMode")
@QtUninvokable
public final void setAspectRatioMode(io.qt.core.Qt.@NonNull AspectRatioMode mode){
setAspectRatioMode_native_Qt_AspectRatioMode(QtJambi_LibraryUtilities.internal.nativeId(this), mode.value());
}
@QtUninvokable
private native void setAspectRatioMode_native_Qt_AspectRatioMode(long __this__nativeId, int mode);
/**
* See QGraphicsVideoItem:: setOffset(QPointF)
*/
@QtPropertyWriter(name="offset")
@QtUninvokable
public final void setOffset(io.qt.core.@NonNull QPointF offset){
setOffset_native_cref_QPointF(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(offset));
}
@QtUninvokable
private native void setOffset_native_cref_QPointF(long __this__nativeId, long offset);
/**
* See QGraphicsVideoItem:: setSize(QSizeF)
*/
@QtPropertyWriter(name="size")
@QtUninvokable
public final void setSize(io.qt.core.@NonNull QSizeF size){
setSize_native_cref_QSizeF(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(size));
}
@QtUninvokable
private native void setSize_native_cref_QSizeF(long __this__nativeId, long size);
/**
* See QGraphicsVideoItem:: size()const
*/
@QtPropertyReader(name="size")
@QtUninvokable
public final io.qt.core.@NonNull QSizeF size(){
return size_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QSizeF size_native_constfct(long __this__nativeId);
/**
* See QGraphicsVideoItem:: type()const
*/
@QtUninvokable
public final int type(){
return type_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int type_native_constfct(long __this__nativeId);
/**
* See QGraphicsVideoItem:: videoSink()const
*/
@QtPropertyReader(name="videoSink")
@QtPropertyConstant
@SuppressWarnings({"exports"})
public final io.qt.multimedia.@Nullable QVideoSink videoSink(){
return videoSink_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native io.qt.multimedia.QVideoSink videoSink_native_constfct(long __this__nativeId);
/**
* See QGraphicsItem:: boundingRect()const
*/
@QtUninvokable
@Override
public io.qt.core.@NonNull QRectF boundingRect(){
return boundingRect_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static io.qt.core.QRectF boundingRect_native_constfct(long __this__nativeId);
/**
* See QGraphicsItem:: itemChange(QGraphicsItem::GraphicsItemChange, QVariant)
*/
@QtUninvokable
@Override
protected java.lang.Object itemChange(io.qt.widgets.QGraphicsItem.@NonNull GraphicsItemChange change, java.lang.Object value){
return itemChange_native_QGraphicsItem_GraphicsItemChange_cref_QVariant(QtJambi_LibraryUtilities.internal.nativeId(this), change.value(), value);
}
private native static java.lang.Object itemChange_native_QGraphicsItem_GraphicsItemChange_cref_QVariant(long __this__nativeId, int change, java.lang.Object value);
/**
* See QGraphicsItem:: paint(QPainter*, const QStyleOptionGraphicsItem*, QWidget*)
*/
@QtUninvokable
@Override
public void paint(io.qt.gui.@Nullable QPainter painter, io.qt.widgets.@Nullable QStyleOptionGraphicsItem option, io.qt.widgets.@Nullable QWidget widget){
paint_native_QPainter_ptr_const_QStyleOptionGraphicsItem_ptr_QWidget_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(painter), QtJambi_LibraryUtilities.internal.checkedNativeId(option), QtJambi_LibraryUtilities.internal.checkedNativeId(widget));
}
private native static void paint_native_QPainter_ptr_const_QStyleOptionGraphicsItem_ptr_QWidget_ptr(long __this__nativeId, long painter, long option, long widget);
/**
* See QObject:: timerEvent(QTimerEvent*)
*/
@QtUninvokable
@Override
protected void timerEvent(io.qt.core.@Nullable QTimerEvent event){
timerEvent_native_QTimerEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void timerEvent_native_QTimerEvent_ptr(long __this__nativeId, long event);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QGraphicsVideoItem(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QGraphicsVideoItem(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QGraphicsVideoItem instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QGraphicsVideoItem(io.qt.widgets.QGraphicsItem)}
* with parent = null
.
*/
public QGraphicsVideoItem() {
this((io.qt.widgets.QGraphicsItem)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #aspectRatioMode()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.Qt.@NonNull AspectRatioMode getAspectRatioMode() {
return aspectRatioMode();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #nativeSize()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QSizeF getNativeSize() {
return nativeSize();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #offset()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QPointF getOffset() {
return offset();
}
/**
* Overloaded function for {@link #setOffset(io.qt.core.QPointF)}.
*/
@QtUninvokable
public final void setOffset(io.qt.core.@NonNull QPoint offset) {
setOffset(new io.qt.core.QPointF(offset));
}
/**
* Overloaded function for {@link #setSize(io.qt.core.QSizeF)}.
*/
@QtUninvokable
public final void setSize(io.qt.core.@NonNull QSize size) {
setSize(new io.qt.core.QSizeF(size));
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #size()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QSizeF getSize() {
return size();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #videoSink()} instead.
*/
@QtPropertyReader(enabled=false)
@SuppressWarnings({"exports"})
public final io.qt.multimedia.@Nullable QVideoSink getVideoSink() {
return videoSink();
}
}