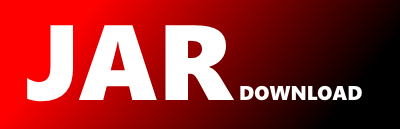
io.qt.pdf.QPdfDocument Maven / Gradle / Ivy
Show all versions of qtjambi-pdf Show documentation
package io.qt.pdf;
import io.qt.*;
/**
* Loads a PDF document and renders pages from it
* Java wrapper for Qt class QPdfDocument
*/
public class QPdfDocument extends io.qt.core.QObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QPdfDocument.class);
/**
* Java wrapper for Qt enum QPdfDocument::Error
*/
public enum Error implements QtEnumerator {
/**
* Representing QPdfDocument:: Error:: None
*/
None(0),
/**
* Representing QPdfDocument:: Error:: Unknown
*/
Unknown(1),
/**
* Representing QPdfDocument:: Error:: DataNotYetAvailable
*/
DataNotYetAvailable(2),
/**
* Representing QPdfDocument:: Error:: FileNotFound
*/
FileNotFound(3),
/**
* Representing QPdfDocument:: Error:: InvalidFileFormat
*/
InvalidFileFormat(4),
/**
* Representing QPdfDocument:: Error:: IncorrectPassword
*/
IncorrectPassword(5),
/**
* Representing QPdfDocument:: Error:: UnsupportedSecurityScheme
*/
UnsupportedSecurityScheme(6);
static {
QtJambi_LibraryUtilities.initialize();
}
private Error(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Error resolve(int value) {
switch (value) {
case 0: return None;
case 1: return Unknown;
case 2: return DataNotYetAvailable;
case 3: return FileNotFound;
case 4: return InvalidFileFormat;
case 5: return IncorrectPassword;
case 6: return UnsupportedSecurityScheme;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPdfDocument::MetaDataField
*/
public enum MetaDataField implements QtEnumerator {
/**
* Representing QPdfDocument:: MetaDataField:: Title
*/
Title(0),
/**
* Representing QPdfDocument:: MetaDataField:: Subject
*/
Subject(1),
/**
* Representing QPdfDocument:: MetaDataField:: Author
*/
Author(2),
/**
* Representing QPdfDocument:: MetaDataField:: Keywords
*/
Keywords(3),
/**
* Representing QPdfDocument:: MetaDataField:: Producer
*/
Producer(4),
/**
* Representing QPdfDocument:: MetaDataField:: Creator
*/
Creator(5),
/**
* Representing QPdfDocument:: MetaDataField:: CreationDate
*/
CreationDate(6),
/**
* Representing QPdfDocument:: MetaDataField:: ModificationDate
*/
ModificationDate(7);
static {
QtJambi_LibraryUtilities.initialize();
}
private MetaDataField(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull MetaDataField resolve(int value) {
switch (value) {
case 0: return Title;
case 1: return Subject;
case 2: return Author;
case 3: return Keywords;
case 4: return Producer;
case 5: return Creator;
case 6: return CreationDate;
case 7: return ModificationDate;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPdfDocument::PageModelRole
*/
public enum PageModelRole implements QtEnumerator {
/**
* Representing QPdfDocument:: PageModelRole:: Label
*/
Label(256),
/**
* Representing QPdfDocument:: PageModelRole:: PointSize
*/
PointSize(257),
/**
* Representing QPdfDocument:: PageModelRole:: NRoles
*/
NRoles(258);
static {
QtJambi_LibraryUtilities.initialize();
}
private PageModelRole(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull PageModelRole resolve(int value) {
switch (value) {
case 256: return Label;
case 257: return PointSize;
case 258: return NRoles;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPdfDocument::Status
*/
public enum Status implements QtEnumerator {
/**
* Representing QPdfDocument:: Status:: Null
*/
Null(0),
/**
* Representing QPdfDocument:: Status:: Loading
*/
Loading(1),
/**
* Representing QPdfDocument:: Status:: Ready
*/
Ready(2),
/**
* Representing QPdfDocument:: Status:: Unloading
*/
Unloading(3),
/**
* Representing QPdfDocument:: Status:: Error
*/
Error(4);
static {
QtJambi_LibraryUtilities.initialize();
}
private Status(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Status resolve(int value) {
switch (value) {
case 0: return Null;
case 1: return Loading;
case 2: return Ready;
case 3: return Unloading;
case 4: return Error;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QPdfDocument:: pageCountChanged(int)
*/
@QtPropertyNotify(name="pageCount")
public final @NonNull Signal1 pageCountChanged = new Signal1<>();
/**
* See QPdfDocument:: pageModelChanged()
*/
@QtPropertyNotify(name="pageModel")
public final @NonNull Signal0 pageModelChanged = new Signal0();
/**
* See QPdfDocument:: passwordChanged()
*/
@QtPropertyNotify(name="password")
public final @NonNull Signal0 passwordChanged = new Signal0();
/**
* See QPdfDocument:: passwordRequired()
*/
public final @NonNull Signal0 passwordRequired = new Signal0();
/**
* See QPdfDocument:: statusChanged(QPdfDocument::Status)
*/
@QtPropertyNotify(name="status")
public final @NonNull Signal1 statusChanged = new Signal1<>();
/**
* See QPdfDocument:: QPdfDocument()
*/
public QPdfDocument(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QPdfDocument instance);
/**
* See QPdfDocument:: QPdfDocument(QObject*)
*/
public QPdfDocument(io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QPdfDocument instance, io.qt.core.QObject parent);
/**
*
*/
@QtUninvokable
public final void close(){
close_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void close_native(long __this__nativeId);
/**
* See QPdfDocument:: error()const
*/
@QtUninvokable
public final io.qt.pdf.QPdfDocument.@NonNull Error error(){
return io.qt.pdf.QPdfDocument.Error.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QPdfDocument:: getAllText(int)
*/
public final io.qt.pdf.@NonNull QPdfSelection getAllText(int page){
return getAllText_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), page);
}
private native io.qt.pdf.QPdfSelection getAllText_native_int(long __this__nativeId, int page);
/**
* See QPdfDocument:: getSelection(int, QPointF, QPointF)
*/
public final io.qt.pdf.@NonNull QPdfSelection getSelection(int page, io.qt.core.@NonNull QPointF start, io.qt.core.@NonNull QPointF end){
return getSelection_native_int_QPointF_QPointF(QtJambi_LibraryUtilities.internal.nativeId(this), page, QtJambi_LibraryUtilities.internal.checkedNativeId(start), QtJambi_LibraryUtilities.internal.checkedNativeId(end));
}
private native io.qt.pdf.QPdfSelection getSelection_native_int_QPointF_QPointF(long __this__nativeId, int page, long start, long end);
/**
* See QPdfDocument:: getSelectionAtIndex(int, int, int)
*/
public final io.qt.pdf.@NonNull QPdfSelection getSelectionAtIndex(int page, int startIndex, int maxLength){
return getSelectionAtIndex_native_int_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), page, startIndex, maxLength);
}
private native io.qt.pdf.QPdfSelection getSelectionAtIndex_native_int_int_int(long __this__nativeId, int page, int startIndex, int maxLength);
/**
* See QPdfDocument:: load(QIODevice*)
*/
@QtUninvokable
public final void load(io.qt.core.@Nullable QIODevice device){
load_native_QIODevice_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(device));
}
@QtUninvokable
private native void load_native_QIODevice_ptr(long __this__nativeId, long device);
/**
* See QPdfDocument:: load(QString)
*/
@QtUninvokable
public final io.qt.pdf.QPdfDocument.@NonNull Error load(java.lang.@NonNull String fileName){
return io.qt.pdf.QPdfDocument.Error.resolve(load_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), fileName));
}
@QtUninvokable
private native int load_native_cref_QString(long __this__nativeId, java.lang.String fileName);
/**
* See QPdfDocument:: metaData(QPdfDocument::MetaDataField)const
*/
@QtUninvokable
public final java.lang.Object metaData(io.qt.pdf.QPdfDocument.@NonNull MetaDataField field){
return metaData_native_QPdfDocument_MetaDataField_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), field.value());
}
@QtUninvokable
private native java.lang.Object metaData_native_QPdfDocument_MetaDataField_constfct(long __this__nativeId, int field);
/**
* See QPdfDocument:: pageCount()const
*/
@QtPropertyReader(name="pageCount")
@QtUninvokable
public final int pageCount(){
return pageCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int pageCount_native_constfct(long __this__nativeId);
/**
* See QPdfDocument:: pageLabel(int)
*/
public final java.lang.@NonNull String pageLabel(int page){
return pageLabel_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), page);
}
private native java.lang.String pageLabel_native_int(long __this__nativeId, int page);
/**
*
*/
@QtPropertyReader(name="pageModel")
@QtUninvokable
public final io.qt.core.@Nullable QAbstractListModel pageModel(){
return pageModel_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QAbstractListModel pageModel_native(long __this__nativeId);
/**
* See QPdfDocument:: pagePointSize(int)const
*/
public final io.qt.core.@NonNull QSizeF pagePointSize(int page){
return pagePointSize_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), page);
}
private native io.qt.core.QSizeF pagePointSize_native_int_constfct(long __this__nativeId, int page);
/**
* See QPdfDocument:: password()const
*/
@QtPropertyReader(name="password")
@QtUninvokable
public final java.lang.@NonNull String password(){
return password_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String password_native_constfct(long __this__nativeId);
/**
* See QPdfDocument:: render(int, QSize, QPdfDocumentRenderOptions)
*/
@QtUninvokable
public final io.qt.gui.@NonNull QImage render(int page, io.qt.core.@NonNull QSize imageSize, io.qt.pdf.@NonNull QPdfDocumentRenderOptions options){
return render_native_int_QSize_QPdfDocumentRenderOptions(QtJambi_LibraryUtilities.internal.nativeId(this), page, QtJambi_LibraryUtilities.internal.checkedNativeId(imageSize), QtJambi_LibraryUtilities.internal.checkedNativeId(options));
}
@QtUninvokable
private native io.qt.gui.QImage render_native_int_QSize_QPdfDocumentRenderOptions(long __this__nativeId, int page, long imageSize, long options);
/**
* See QPdfDocument:: setPassword(QString)
*/
@QtPropertyWriter(name="password")
@QtUninvokable
public final void setPassword(java.lang.@NonNull String password){
setPassword_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), password);
}
@QtUninvokable
private native void setPassword_native_cref_QString(long __this__nativeId, java.lang.String password);
/**
* See QPdfDocument:: status()const
*/
@QtPropertyReader(name="status")
@QtUninvokable
public final io.qt.pdf.QPdfDocument.@NonNull Status status(){
return io.qt.pdf.QPdfDocument.Status.resolve(status_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int status_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QPdfDocument(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QPdfDocument(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QPdfDocument instance, QDeclarativeConstructor constructor);
/**
* Overloaded function for {@link #getSelection(int, io.qt.core.QPointF, io.qt.core.QPointF)}.
*/
public final io.qt.pdf.@NonNull QPdfSelection getSelection(int page, io.qt.core.@NonNull QPoint start, io.qt.core.@NonNull QPointF end) {
return getSelection(page, new io.qt.core.QPointF(start), end);
}
/**
* Overloaded function for {@link #getSelection(int, io.qt.core.QPointF, io.qt.core.QPointF)}.
*/
public final io.qt.pdf.@NonNull QPdfSelection getSelection(int page, io.qt.core.@NonNull QPointF start, io.qt.core.@NonNull QPoint end) {
return getSelection(page, start, new io.qt.core.QPointF(end));
}
/**
* Overloaded function for {@link #getSelection(int, io.qt.core.QPointF, io.qt.core.QPointF)}.
*/
public final io.qt.pdf.@NonNull QPdfSelection getSelection(int page, io.qt.core.@NonNull QPoint start, io.qt.core.@NonNull QPoint end) {
return getSelection(page, new io.qt.core.QPointF(start), new io.qt.core.QPointF(end));
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #pageCount()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getPageCount() {
return pageCount();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #pageModel()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@Nullable QAbstractListModel getPageModel() {
return pageModel();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #password()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final java.lang.@NonNull String getPassword() {
return password();
}
/**
* Overloaded function for {@link #render(int, io.qt.core.QSize, io.qt.pdf.QPdfDocumentRenderOptions)}
* with options = new io.qt.pdf.QPdfDocumentRenderOptions()
.
*/
@QtUninvokable
public final io.qt.gui.@NonNull QImage render(int page, io.qt.core.@NonNull QSize imageSize) {
return render(page, imageSize, new io.qt.pdf.QPdfDocumentRenderOptions());
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #status()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.pdf.QPdfDocument.@NonNull Status getStatus() {
return status();
}
}