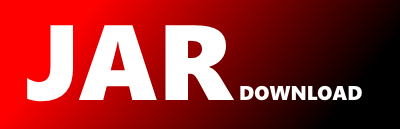
io.qt.pdf.QPdfSelection Maven / Gradle / Ivy
Show all versions of qtjambi-pdf Show documentation
package io.qt.pdf;
import io.qt.*;
/**
* Defines a range of text that has been selected on one page in a PDF document, and its geometric boundaries
* Java wrapper for Qt class QPdfSelection
*/
public class QPdfSelection extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QPdfSelection.class);
/**
* See QPdfSelection:: QPdfSelection(QPdfSelection)
*/
public QPdfSelection(io.qt.pdf.@StrictNonNull QPdfSelection other){
super((QPrivateConstructor)null);
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
initialize_native(this, other);
}
private native static void initialize_native(QPdfSelection instance, io.qt.pdf.QPdfSelection other);
/**
* See QPdfSelection:: boundingRectangle()const
*/
@QtPropertyReader(name="boundingRectangle")
@QtUninvokable
public final io.qt.core.@NonNull QRectF boundingRectangle(){
return boundingRectangle_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QRectF boundingRectangle_native_constfct(long __this__nativeId);
/**
* See QPdfSelection:: bounds()const
*/
@QtPropertyReader(name="bounds")
@QtUninvokable
public final io.qt.core.@NonNull QList bounds(){
return bounds_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QList bounds_native_constfct(long __this__nativeId);
/**
* See QPdfSelection:: copyToClipboard(QClipboard::Mode)const
*/
@QtUninvokable
public final void copyToClipboard(io.qt.gui.QClipboard.@NonNull Mode mode){
copyToClipboard_native_QClipboard_Mode_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), mode.value());
}
@QtUninvokable
private native void copyToClipboard_native_QClipboard_Mode_constfct(long __this__nativeId, int mode);
/**
* See QPdfSelection:: endIndex()const
*/
@QtPropertyReader(name="endIndex")
@QtUninvokable
public final int endIndex(){
return endIndex_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int endIndex_native_constfct(long __this__nativeId);
/**
* See QPdfSelection:: isValid()const
*/
@QtPropertyReader(name="valid")
@QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QPdfSelection:: operator=(QPdfSelection)
*/
@QtUninvokable
public final void assign(io.qt.pdf.@StrictNonNull QPdfSelection other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
assign_native_cref_QPdfSelection(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void assign_native_cref_QPdfSelection(long __this__nativeId, long other);
/**
* See operator==(QPdfSelection, QPdfSelection)
*/
@QtUninvokable
public final boolean equals(io.qt.pdf.@StrictNonNull QPdfSelection value2){
java.util.Objects.requireNonNull(value2, "Argument 'value2': null not expected.");
return equals_native_cref_QPdfSelection(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(value2));
}
@QtUninvokable
private native boolean equals_native_cref_QPdfSelection(long __this__nativeId, long value2);
/**
* See QPdfSelection:: startIndex()const
*/
@QtPropertyReader(name="startIndex")
@QtUninvokable
public final int startIndex(){
return startIndex_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int startIndex_native_constfct(long __this__nativeId);
/**
* See QPdfSelection:: swap(QPdfSelection&)
*/
@QtUninvokable
public final void swap(io.qt.pdf.@StrictNonNull QPdfSelection other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QPdfSelection(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void swap_native_ref_QPdfSelection(long __this__nativeId, long other);
/**
* See QPdfSelection:: text()const
*/
@QtPropertyReader(name="text")
@QtUninvokable
public final java.lang.@NonNull String text(){
return text_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String text_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QPdfSelection(QPrivateConstructor p) { super(p); }
/**
* See operator==(QPdfSelection, QPdfSelection)
*/
@Override
@QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.pdf.QPdfSelection) {
return equals((io.qt.pdf.QPdfSelection) other);
}
return false;
}
/**
* Returns the objects's hash code computed by qHash(QPdfSelection)
.
*/
@QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native static int hashCode_native(long __this_nativeId);
/**
* Creates and returns a copy of this object.
See QPdfSelection:: QPdfSelection(QPdfSelection)
*/
@QtUninvokable
@Override
public QPdfSelection clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native QPdfSelection clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #boundingRectangle()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QRectF getBoundingRectangle() {
return boundingRectangle();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #bounds()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QList getBounds() {
return bounds();
}
/**
* Overloaded function for {@link #copyToClipboard(io.qt.gui.QClipboard.Mode)}
* with mode = io.qt.gui.QClipboard.Mode.Clipboard
.
*/
@QtUninvokable
public final void copyToClipboard() {
copyToClipboard(io.qt.gui.QClipboard.Mode.Clipboard);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #endIndex()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getEndIndex() {
return endIndex();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #isValid()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final boolean getValid() {
return isValid();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #startIndex()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getStartIndex() {
return startIndex();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #text()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final java.lang.@NonNull String getText() {
return text();
}
}