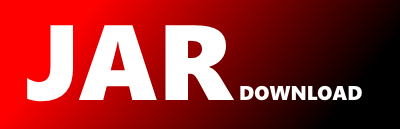
io.qt.printsupport.QAbstractPrintDialog Maven / Gradle / Ivy
Show all versions of qtjambi-printsupport-jre8 Show documentation
package io.qt.printsupport;
/**
* Base implementation for print dialogs used to configure printers
* Java wrapper for Qt class QAbstractPrintDialog
*/
public class QAbstractPrintDialog extends io.qt.widgets.QDialog
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QAbstractPrintDialog.class);
/**
* Java wrapper for Qt enum QAbstractPrintDialog::PrintDialogOption
*
* @see PrintDialogOptions
*/
public enum PrintDialogOption implements io.qt.QtFlagEnumerator {
PrintToFile(1),
PrintSelection(2),
PrintPageRange(4),
PrintShowPageSize(8),
PrintCollateCopies(16),
PrintCurrentPage(64);
private PrintDialogOption(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Create a QFlags of the enum entry.
* @return QFlags
*/
public PrintDialogOptions asFlags() {
return new PrintDialogOptions(value);
}
/**
* Combines this entry with other enum entry.
* @param e enum entry
* @return new flag
*/
public PrintDialogOptions combined(PrintDialogOption e) {
return new PrintDialogOptions(this, e);
}
/**
* Creates a new {@link PrintDialogOptions} from the entries.
* @param values entries
* @return new flag
*/
public static PrintDialogOptions flags(PrintDialogOption ... values) {
return new PrintDialogOptions(values);
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PrintDialogOption resolve(int value) {
switch (value) {
case 1: return PrintToFile;
case 2: return PrintSelection;
case 4: return PrintPageRange;
case 8: return PrintShowPageSize;
case 16: return PrintCollateCopies;
case 64: return PrintCurrentPage;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* QFlags type for enum {@link PrintDialogOption}
*/
public static final class PrintDialogOptions extends io.qt.QFlags implements Comparable {
private static final long serialVersionUID = 0xe8a339595a9c2774L;
/**
* Creates a new PrintDialogOptions where the flags in args
are set.
* @param args enum entries
*/
public PrintDialogOptions(PrintDialogOption ... args){
super(args);
}
/**
* Creates a new PrintDialogOptions with given value
.
* @param value
*/
public PrintDialogOptions(int value) {
super(value);
}
/**
* Combines this flags with enum entry.
* @param e enum entry
* @return new PrintDialogOptions
*/
@Override
public final PrintDialogOptions combined(PrintDialogOption e){
return new PrintDialogOptions(value() | e.value());
}
/**
* Sets the flag e
* @param e enum entry
* @return this
*/
public final PrintDialogOptions setFlag(PrintDialogOption e){
super.setFlag(e);
return this;
}
/**
* Sets or clears the flag flag
* @param e enum entry
* @param on set (true) or clear (false)
* @return this
*/
public final PrintDialogOptions setFlag(PrintDialogOption e, boolean on){
super.setFlag(e, on);
return this;
}
/**
* Returns an array of flag objects represented by this PrintDialogOptions.
* @return array of enum entries
*/
@Override
public final PrintDialogOption[] flags(){
return super.flags(PrintDialogOption.values());
}
/**
* {@inheritDoc}
*/
@Override
public final PrintDialogOptions clone(){
return new PrintDialogOptions(value());
}
/**
* {@inheritDoc}
*/
@Override
public final int compareTo(PrintDialogOptions other){
return Integer.compare(value(), other.value());
}
}
/**
* Java wrapper for Qt enum QAbstractPrintDialog::PrintRange
*/
@io.qt.QtUnlistedEnum
public enum PrintRange implements io.qt.QtEnumerator {
AllPages(0),
Selection(1),
PageRange(2),
CurrentPage(3);
private PrintRange(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PrintRange resolve(int value) {
switch (value) {
case 0: return AllPages;
case 1: return Selection;
case 2: return PageRange;
case 3: return CurrentPage;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Overloaded constructor for {@link #QAbstractPrintDialog(io.qt.printsupport.QPrinter, io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QAbstractPrintDialog(io.qt.printsupport.QPrinter printer) {
this(printer, (io.qt.widgets.QWidget)null);
}
/**
* See QAbstractPrintDialog::QAbstractPrintDialog(QPrinter*,QWidget*)
*/
public QAbstractPrintDialog(io.qt.printsupport.QPrinter printer, io.qt.widgets.QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, printer, parent);
}
private native static void initialize_native(QAbstractPrintDialog instance, io.qt.printsupport.QPrinter printer, io.qt.widgets.QWidget parent);
/**
* See QAbstractPrintDialog::fromPage()const
*/
@io.qt.QtUninvokable
public final int fromPage(){
return fromPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int fromPage_native_constfct(long __this__nativeId);
/**
* See QAbstractPrintDialog::maxPage()const
*/
@io.qt.QtUninvokable
public final int maxPage(){
return maxPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int maxPage_native_constfct(long __this__nativeId);
/**
* See QAbstractPrintDialog::minPage()const
*/
@io.qt.QtUninvokable
public final int minPage(){
return minPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int minPage_native_constfct(long __this__nativeId);
/**
* See QAbstractPrintDialog::printRange()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QAbstractPrintDialog.PrintRange printRange(){
return io.qt.printsupport.QAbstractPrintDialog.PrintRange.resolve(printRange_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int printRange_native_constfct(long __this__nativeId);
/**
* See QAbstractPrintDialog::printer()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter printer(){
return printer_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.printsupport.QPrinter printer_native_constfct(long __this__nativeId);
/**
* See QAbstractPrintDialog::setFromTo(int,int)
*/
@io.qt.QtUninvokable
public final void setFromTo(int fromPage, int toPage){
setFromTo_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), fromPage, toPage);
}
@io.qt.QtUninvokable
private native void setFromTo_native_int_int(long __this__nativeId, int fromPage, int toPage);
/**
* See QAbstractPrintDialog::setMinMax(int,int)
*/
@io.qt.QtUninvokable
public final void setMinMax(int min, int max){
setMinMax_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), min, max);
}
@io.qt.QtUninvokable
private native void setMinMax_native_int_int(long __this__nativeId, int min, int max);
/**
* See QAbstractPrintDialog::setOptionTabs(QList<QWidget*>)
*/
@io.qt.QtUninvokable
public final void setOptionTabs(java.util.Collection tabs){
setOptionTabs_native_cref_QList(QtJambi_LibraryUtilities.internal.nativeId(this), tabs);
}
@io.qt.QtUninvokable
private native void setOptionTabs_native_cref_QList(long __this__nativeId, java.util.Collection tabs);
/**
* See QAbstractPrintDialog::setPrintRange(QAbstractPrintDialog::PrintRange)
*/
@io.qt.QtUninvokable
public final void setPrintRange(io.qt.printsupport.QAbstractPrintDialog.PrintRange range){
setPrintRange_native_QAbstractPrintDialog_PrintRange(QtJambi_LibraryUtilities.internal.nativeId(this), range.value());
}
@io.qt.QtUninvokable
private native void setPrintRange_native_QAbstractPrintDialog_PrintRange(long __this__nativeId, int range);
/**
* See QAbstractPrintDialog::toPage()const
*/
@io.qt.QtUninvokable
public final int toPage(){
return toPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int toPage_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QAbstractPrintDialog(QPrivateConstructor p) { super(p); }
}