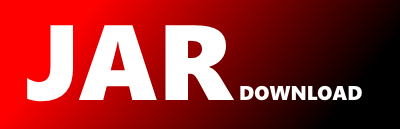
io.qt.printsupport.QPrinter Maven / Gradle / Ivy
package io.qt.printsupport;
/**
* Paint device that paints on a printer
* Java wrapper for Qt class QPrinter
*/
public class QPrinter extends io.qt.QtObject
implements io.qt.gui.QPagedPaintDevice,
io.qt.gui.QPaintDevice
{
static {
QtJambi_LibraryUtilities.initialize();
}
@io.qt.QtPropertyMember(enabled=false)
private Object __rcPaintEngine = null;
@io.qt.QtPropertyMember(enabled=false)
private Object __rcPrintEngine = null;
/**
* Java wrapper for Qt enum QPrinter::ColorMode
*/
public enum ColorMode implements io.qt.QtEnumerator {
GrayScale(0),
Color(1);
private ColorMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ColorMode resolve(int value) {
switch (value) {
case 0: return GrayScale;
case 1: return Color;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::DuplexMode
*/
public enum DuplexMode implements io.qt.QtEnumerator {
DuplexNone(0),
DuplexAuto(1),
DuplexLongSide(2),
DuplexShortSide(3);
private DuplexMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static DuplexMode resolve(int value) {
switch (value) {
case 0: return DuplexNone;
case 1: return DuplexAuto;
case 2: return DuplexLongSide;
case 3: return DuplexShortSide;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::OutputFormat
*/
public enum OutputFormat implements io.qt.QtEnumerator {
NativeFormat(0),
PdfFormat(1);
private OutputFormat(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static OutputFormat resolve(int value) {
switch (value) {
case 0: return NativeFormat;
case 1: return PdfFormat;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::PageOrder
*/
public enum PageOrder implements io.qt.QtEnumerator {
FirstPageFirst(0),
LastPageFirst(1);
private PageOrder(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PageOrder resolve(int value) {
switch (value) {
case 0: return FirstPageFirst;
case 1: return LastPageFirst;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::PaperSource
*/
@io.qt.QtRejectedEntries({"LastPaperSource", "Upper"})
public enum PaperSource implements io.qt.QtEnumerator {
OnlyOne(0),
Lower(1),
Middle(2),
Manual(3),
Envelope(4),
EnvelopeManual(5),
Auto(6),
Tractor(7),
SmallFormat(8),
LargeFormat(9),
LargeCapacity(10),
Cassette(11),
FormSource(12),
MaxPageSource(13),
CustomSource(14),
LastPaperSource(14),
Upper(0);
private PaperSource(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PaperSource resolve(int value) {
switch (value) {
case 0: return OnlyOne;
case 1: return Lower;
case 2: return Middle;
case 3: return Manual;
case 4: return Envelope;
case 5: return EnvelopeManual;
case 6: return Auto;
case 7: return Tractor;
case 8: return SmallFormat;
case 9: return LargeFormat;
case 10: return LargeCapacity;
case 11: return Cassette;
case 12: return FormSource;
case 13: return MaxPageSource;
case 14: return CustomSource;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::PrintRange
*/
public enum PrintRange implements io.qt.QtEnumerator {
AllPages(0),
Selection(1),
PageRange(2),
CurrentPage(3);
private PrintRange(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PrintRange resolve(int value) {
switch (value) {
case 0: return AllPages;
case 1: return Selection;
case 2: return PageRange;
case 3: return CurrentPage;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::PrinterMode
*/
public enum PrinterMode implements io.qt.QtEnumerator {
ScreenResolution(0),
PrinterResolution(1),
HighResolution(2);
private PrinterMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PrinterMode resolve(int value) {
switch (value) {
case 0: return ScreenResolution;
case 1: return PrinterResolution;
case 2: return HighResolution;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::PrinterState
*/
public enum PrinterState implements io.qt.QtEnumerator {
Idle(0),
Active(1),
Aborted(2),
Error(3);
private PrinterState(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static PrinterState resolve(int value) {
switch (value) {
case 0: return Idle;
case 1: return Active;
case 2: return Aborted;
case 3: return Error;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QPrinter::Unit
*/
public enum Unit implements io.qt.QtEnumerator {
Millimeter(0),
Point(1),
Inch(2),
Pica(3),
Didot(4),
Cicero(5),
DevicePixel(6);
private Unit(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Unit resolve(int value) {
switch (value) {
case 0: return Millimeter;
case 1: return Point;
case 2: return Inch;
case 3: return Pica;
case 4: return Didot;
case 5: return Cicero;
case 6: return DevicePixel;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Overloaded constructor for {@link #QPrinter(io.qt.printsupport.QPrinter.PrinterMode)}
* with mode = io.qt.printsupport.QPrinter.PrinterMode.ScreenResolution
.
*/
public QPrinter() {
this(io.qt.printsupport.QPrinter.PrinterMode.ScreenResolution);
}
/**
* See QPrinter::QPrinter(QPrinter::PrinterMode)
*/
public QPrinter(io.qt.printsupport.QPrinter.PrinterMode mode){
super((QPrivateConstructor)null);
if(io.qt.core.QCoreApplication.instance()==null)
throw new IllegalStateException("Cannot create QPrinter before initializing QCoreApplication.");
initialize_native(this, mode);
}
private native static void initialize_native(QPrinter instance, io.qt.printsupport.QPrinter.PrinterMode mode);
/**
* Overloaded constructor for {@link #QPrinter(io.qt.printsupport.QPrinterInfo, io.qt.printsupport.QPrinter.PrinterMode)}
* with mode = io.qt.printsupport.QPrinter.PrinterMode.ScreenResolution
.
*/
public QPrinter(io.qt.printsupport.QPrinterInfo printer) {
this(printer, io.qt.printsupport.QPrinter.PrinterMode.ScreenResolution);
}
/**
* See QPrinter::QPrinter(QPrinterInfo,QPrinter::PrinterMode)
*/
public QPrinter(io.qt.printsupport.QPrinterInfo printer, io.qt.printsupport.QPrinter.PrinterMode mode){
super((QPrivateConstructor)null);
if(io.qt.core.QCoreApplication.instance()==null)
throw new IllegalStateException("Cannot create QPrinter before initializing QCoreApplication.");
initialize_native(this, printer, mode);
}
private native static void initialize_native(QPrinter instance, io.qt.printsupport.QPrinterInfo printer, io.qt.printsupport.QPrinter.PrinterMode mode);
/**
*
*/
@io.qt.QtUninvokable
public final boolean abort(){
return abort_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean abort_native(long __this__nativeId);
/**
* See QPrinter::collateCopies()const
*/
@io.qt.QtUninvokable
public final boolean collateCopies(){
return collateCopies_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean collateCopies_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::colorCount()const
*/
@io.qt.QtUninvokable
public final int colorCount(){
return colorCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int colorCount_native_constfct(long __this__nativeId);
/**
* See QPrinter::colorMode()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.ColorMode colorMode(){
return io.qt.printsupport.QPrinter.ColorMode.resolve(colorMode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int colorMode_native_constfct(long __this__nativeId);
/**
* See QPrinter::copyCount()const
*/
@io.qt.QtUninvokable
public final int copyCount(){
return copyCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int copyCount_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final java.lang.String creator(){
return creator_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String creator_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::depth()const
*/
@io.qt.QtUninvokable
public final int depth(){
return depth_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int depth_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::devicePixelRatio()const
*/
@io.qt.QtUninvokable
public final double devicePixelRatio(){
return devicePixelRatio_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static double devicePixelRatio_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::devicePixelRatioF()const
*/
@io.qt.QtUninvokable
public final double devicePixelRatioF(){
return devicePixelRatioF_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static double devicePixelRatioF_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final java.lang.String docName(){
return docName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String docName_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.DuplexMode duplex(){
return io.qt.printsupport.QPrinter.DuplexMode.resolve(duplex_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int duplex_native_constfct(long __this__nativeId);
/**
* See QPrinter::fontEmbeddingEnabled()const
*/
@io.qt.QtUninvokable
public final boolean fontEmbeddingEnabled(){
return fontEmbeddingEnabled_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean fontEmbeddingEnabled_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final int fromPage(){
return fromPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int fromPage_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final boolean fullPage(){
return fullPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean fullPage_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::height()const
*/
@io.qt.QtUninvokable
public final int height(){
return height_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int height_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::heightMM()const
*/
@io.qt.QtUninvokable
public final int heightMM(){
return heightMM_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int heightMM_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::logicalDpiX()const
*/
@io.qt.QtUninvokable
public final int logicalDpiX(){
return logicalDpiX_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int logicalDpiX_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::logicalDpiY()const
*/
@io.qt.QtUninvokable
public final int logicalDpiY(){
return logicalDpiY_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int logicalDpiY_native_constfct(long __this__nativeId);
/**
* See QPrinter::outputFileName()const
*/
@io.qt.QtUninvokable
public final java.lang.String outputFileName(){
return outputFileName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String outputFileName_native_constfct(long __this__nativeId);
/**
* See QPrinter::outputFormat()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.OutputFormat outputFormat(){
return io.qt.printsupport.QPrinter.OutputFormat.resolve(outputFormat_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int outputFormat_native_constfct(long __this__nativeId);
/**
* See QPagedPaintDevice::pageLayout()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPageLayout pageLayout(){
return pageLayout_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static io.qt.gui.QPageLayout pageLayout_native_constfct(long __this__nativeId);
/**
* See QPrinter::pageOrder()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.PageOrder pageOrder(){
return io.qt.printsupport.QPrinter.PageOrder.resolve(pageOrder_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int pageOrder_native_constfct(long __this__nativeId);
/**
* See QPagedPaintDevice::pageRanges()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPageRanges pageRanges(){
return pageRanges_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static io.qt.gui.QPageRanges pageRanges_native_constfct(long __this__nativeId);
/**
* See QPrinter::pageRect(QPrinter::Unit)const
*/
@io.qt.QtUninvokable
public final io.qt.core.QRectF pageRect(io.qt.printsupport.QPrinter.Unit arg__1){
return pageRect_native_QPrinter_Unit_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native io.qt.core.QRectF pageRect_native_QPrinter_Unit_constfct(long __this__nativeId, int arg__1);
/**
* See QPaintDevice::paintingActive()const
*/
@io.qt.QtUninvokable
public final boolean paintingActive(){
return paintingActive_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static boolean paintingActive_native_constfct(long __this__nativeId);
/**
* See QPrinter::paperRect(QPrinter::Unit)const
*/
@io.qt.QtUninvokable
public final io.qt.core.QRectF paperRect(io.qt.printsupport.QPrinter.Unit arg__1){
return paperRect_native_QPrinter_Unit_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native io.qt.core.QRectF paperRect_native_QPrinter_Unit_constfct(long __this__nativeId, int arg__1);
/**
* See QPrinter::paperSource()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.PaperSource paperSource(){
return io.qt.printsupport.QPrinter.PaperSource.resolve(paperSource_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int paperSource_native_constfct(long __this__nativeId);
/**
* See QPrinter::pdfVersion()const
*/
@io.qt.QtUninvokable
public final io.qt.gui.QPagedPaintDevice.PdfVersion pdfVersion(){
return io.qt.gui.QPagedPaintDevice.PdfVersion.resolve(pdfVersion_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int pdfVersion_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::physicalDpiX()const
*/
@io.qt.QtUninvokable
public final int physicalDpiX(){
return physicalDpiX_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int physicalDpiX_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::physicalDpiY()const
*/
@io.qt.QtUninvokable
public final int physicalDpiY(){
return physicalDpiY_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int physicalDpiY_native_constfct(long __this__nativeId);
/**
* See QPrinter::printEngine()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrintEngine printEngine(){
return printEngine_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.printsupport.QPrintEngine printEngine_native_constfct(long __this__nativeId);
/**
* See QPrinter::printProgram()const
*/
@io.qt.QtUninvokable
public final java.lang.String printProgram(){
return printProgram_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String printProgram_native_constfct(long __this__nativeId);
/**
* See QPrinter::printRange()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.PrintRange printRange(){
return io.qt.printsupport.QPrinter.PrintRange.resolve(printRange_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int printRange_native_constfct(long __this__nativeId);
/**
* See QPrinter::printerName()const
*/
@io.qt.QtUninvokable
public final java.lang.String printerName(){
return printerName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String printerName_native_constfct(long __this__nativeId);
/**
* See QPrinter::printerState()const
*/
@io.qt.QtUninvokable
public final io.qt.printsupport.QPrinter.PrinterState printerState(){
return io.qt.printsupport.QPrinter.PrinterState.resolve(printerState_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int printerState_native_constfct(long __this__nativeId);
/**
* See QPrinter::resolution()const
*/
@io.qt.QtUninvokable
public final int resolution(){
return resolution_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int resolution_native_constfct(long __this__nativeId);
/**
* See QPrinter::setCollateCopies(bool)
*/
@io.qt.QtUninvokable
public final void setCollateCopies(boolean collate){
setCollateCopies_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), collate);
}
@io.qt.QtUninvokable
private native void setCollateCopies_native_bool(long __this__nativeId, boolean collate);
/**
* See QPrinter::setColorMode(QPrinter::ColorMode)
*/
@io.qt.QtUninvokable
public final void setColorMode(io.qt.printsupport.QPrinter.ColorMode arg__1){
setColorMode_native_QPrinter_ColorMode(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void setColorMode_native_QPrinter_ColorMode(long __this__nativeId, int arg__1);
/**
* See QPrinter::setCopyCount(int)
*/
@io.qt.QtUninvokable
public final void setCopyCount(int arg__1){
setCopyCount_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setCopyCount_native_int(long __this__nativeId, int arg__1);
/**
* See QPrinter::setCreator(QString)
*/
@io.qt.QtUninvokable
public final void setCreator(java.lang.String arg__1){
setCreator_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setCreator_native_cref_QString(long __this__nativeId, java.lang.String arg__1);
/**
* See QPrinter::setDocName(QString)
*/
@io.qt.QtUninvokable
public final void setDocName(java.lang.String arg__1){
setDocName_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setDocName_native_cref_QString(long __this__nativeId, java.lang.String arg__1);
/**
* See QPrinter::setDuplex(QPrinter::DuplexMode)
*/
@io.qt.QtUninvokable
public final void setDuplex(io.qt.printsupport.QPrinter.DuplexMode duplex){
setDuplex_native_QPrinter_DuplexMode(QtJambi_LibraryUtilities.internal.nativeId(this), duplex.value());
}
@io.qt.QtUninvokable
private native void setDuplex_native_QPrinter_DuplexMode(long __this__nativeId, int duplex);
/**
* See QPrinter::setEngines(QPrintEngine*,QPaintEngine*)
*/
@io.qt.QtUninvokable
protected final void setEngines(io.qt.printsupport.QPrintEngine printEngine, io.qt.gui.QPaintEngine paintEngine){
setEngines_native_QPrintEngine_ptr_QPaintEngine_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(printEngine), QtJambi_LibraryUtilities.internal.checkedNativeId(paintEngine));
__rcPrintEngine = printEngine;
__rcPaintEngine = paintEngine;
}
@io.qt.QtUninvokable
private native void setEngines_native_QPrintEngine_ptr_QPaintEngine_ptr(long __this__nativeId, long printEngine, long paintEngine);
/**
* See QPrinter::setFontEmbeddingEnabled(bool)
*/
@io.qt.QtUninvokable
public final void setFontEmbeddingEnabled(boolean enable){
setFontEmbeddingEnabled_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), enable);
}
@io.qt.QtUninvokable
private native void setFontEmbeddingEnabled_native_bool(long __this__nativeId, boolean enable);
/**
* See QPrinter::setFromTo(int,int)
*/
@io.qt.QtUninvokable
public final void setFromTo(int fromPage, int toPage){
setFromTo_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), fromPage, toPage);
}
@io.qt.QtUninvokable
private native void setFromTo_native_int_int(long __this__nativeId, int fromPage, int toPage);
/**
* See QPrinter::setFullPage(bool)
*/
@io.qt.QtUninvokable
public final void setFullPage(boolean arg__1){
setFullPage_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setFullPage_native_bool(long __this__nativeId, boolean arg__1);
/**
* See QPrinter::setOutputFileName(QString)
*/
@io.qt.QtUninvokable
public final void setOutputFileName(java.lang.String arg__1){
setOutputFileName_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setOutputFileName_native_cref_QString(long __this__nativeId, java.lang.String arg__1);
/**
* See QPrinter::setOutputFormat(QPrinter::OutputFormat)
*/
@io.qt.QtUninvokable
public final void setOutputFormat(io.qt.printsupport.QPrinter.OutputFormat format){
setOutputFormat_native_QPrinter_OutputFormat(QtJambi_LibraryUtilities.internal.nativeId(this), format.value());
}
@io.qt.QtUninvokable
private native void setOutputFormat_native_QPrinter_OutputFormat(long __this__nativeId, int format);
/**
* See QPrinter::setPageOrder(QPrinter::PageOrder)
*/
@io.qt.QtUninvokable
public final void setPageOrder(io.qt.printsupport.QPrinter.PageOrder arg__1){
setPageOrder_native_QPrinter_PageOrder(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void setPageOrder_native_QPrinter_PageOrder(long __this__nativeId, int arg__1);
/**
* See QPrinter::setPaperSource(QPrinter::PaperSource)
*/
@io.qt.QtUninvokable
public final void setPaperSource(io.qt.printsupport.QPrinter.PaperSource arg__1){
setPaperSource_native_QPrinter_PaperSource(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@io.qt.QtUninvokable
private native void setPaperSource_native_QPrinter_PaperSource(long __this__nativeId, int arg__1);
/**
* See QPrinter::setPdfVersion(QPagedPaintDevice::PdfVersion)
*/
@io.qt.QtUninvokable
public final void setPdfVersion(io.qt.gui.QPagedPaintDevice.PdfVersion version){
setPdfVersion_native_QPagedPaintDevice_PdfVersion(QtJambi_LibraryUtilities.internal.nativeId(this), version.value());
}
@io.qt.QtUninvokable
private native void setPdfVersion_native_QPagedPaintDevice_PdfVersion(long __this__nativeId, int version);
/**
* See QPrinter::setPrintProgram(QString)
*/
@io.qt.QtUninvokable
public final void setPrintProgram(java.lang.String arg__1){
setPrintProgram_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setPrintProgram_native_cref_QString(long __this__nativeId, java.lang.String arg__1);
/**
* See QPrinter::setPrintRange(QPrinter::PrintRange)
*/
@io.qt.QtUninvokable
public final void setPrintRange(io.qt.printsupport.QPrinter.PrintRange range){
setPrintRange_native_QPrinter_PrintRange(QtJambi_LibraryUtilities.internal.nativeId(this), range.value());
}
@io.qt.QtUninvokable
private native void setPrintRange_native_QPrinter_PrintRange(long __this__nativeId, int range);
/**
* See QPrinter::setPrinterName(QString)
*/
@io.qt.QtUninvokable
public final void setPrinterName(java.lang.String arg__1){
setPrinterName_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setPrinterName_native_cref_QString(long __this__nativeId, java.lang.String arg__1);
/**
* See QPrinter::setResolution(int)
*/
@io.qt.QtUninvokable
public final void setResolution(int arg__1){
setResolution_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setResolution_native_int(long __this__nativeId, int arg__1);
/**
* See QPrinter::supportedResolutions()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList supportedResolutions(){
return supportedResolutions_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList supportedResolutions_native_constfct(long __this__nativeId);
/**
* See QPrinter::supportsMultipleCopies()const
*/
@io.qt.QtUninvokable
public final boolean supportsMultipleCopies(){
return supportsMultipleCopies_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean supportsMultipleCopies_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final int toPage(){
return toPage_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int toPage_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::width()const
*/
@io.qt.QtUninvokable
public final int width(){
return width_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int width_native_constfct(long __this__nativeId);
/**
* See QPaintDevice::widthMM()const
*/
@io.qt.QtUninvokable
public final int widthMM(){
return widthMM_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static int widthMM_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected void initPainter(io.qt.gui.QPainter painter){
initPainter_native_QPainter_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(painter));
}
private native static void initPainter_native_QPainter_ptr_constfct(long __this__nativeId, long painter);
/**
* See QPaintDevice::metric(QPaintDevice::PaintDeviceMetric)const
*/
@io.qt.QtUninvokable
protected int metric(io.qt.gui.QPaintDevice.PaintDeviceMetric arg__1){
return metric_native_QPaintDevice_PaintDeviceMetric_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
private native static int metric_native_QPaintDevice_PaintDeviceMetric_constfct(long __this__nativeId, int arg__1);
/**
* See QPagedPaintDevice::newPage()
*/
@io.qt.QtUninvokable
public boolean newPage(){
return newPage_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static boolean newPage_native(long __this__nativeId);
/**
* See QPaintDevice::paintEngine()const
*/
@io.qt.QtUninvokable
public io.qt.gui.QPaintEngine paintEngine(){
return paintEngine_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static io.qt.gui.QPaintEngine paintEngine_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected io.qt.gui.QPaintDevice redirected(io.qt.core.QPoint offset){
return redirected_native_QPoint_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), offset);
}
private native static io.qt.gui.QPaintDevice redirected_native_QPoint_ptr_constfct(long __this__nativeId, io.qt.core.QPoint offset);
/**
* See QPagedPaintDevice::setPageLayout(QPageLayout)
*/
@io.qt.QtUninvokable
public boolean setPageLayout(io.qt.gui.QPageLayout pageLayout){
return setPageLayout_native_cref_QPageLayout(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(pageLayout));
}
private native static boolean setPageLayout_native_cref_QPageLayout(long __this__nativeId, long pageLayout);
/**
* Overloaded function for {@link #setPageMargins(io.qt.core.QMarginsF, io.qt.gui.QPageLayout.Unit)}
* with units = io.qt.gui.QPageLayout.Unit.Millimeter
.
*/
@io.qt.QtUninvokable
public final boolean setPageMargins(io.qt.core.QMarginsF margins) {
return setPageMargins(margins, io.qt.gui.QPageLayout.Unit.Millimeter);
}
/**
* See QPagedPaintDevice::setPageMargins(QMarginsF,QPageLayout::Unit)
*/
@io.qt.QtUninvokable
public boolean setPageMargins(io.qt.core.QMarginsF margins, io.qt.gui.QPageLayout.Unit units){
return setPageMargins_native_cref_QMarginsF_QPageLayout_Unit(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(margins), units.value());
}
private native static boolean setPageMargins_native_cref_QMarginsF_QPageLayout_Unit(long __this__nativeId, long margins, int units);
/**
* See QPagedPaintDevice::setPageOrientation(QPageLayout::Orientation)
*/
@io.qt.QtUninvokable
public boolean setPageOrientation(io.qt.gui.QPageLayout.Orientation orientation){
return setPageOrientation_native_QPageLayout_Orientation(QtJambi_LibraryUtilities.internal.nativeId(this), orientation.value());
}
private native static boolean setPageOrientation_native_QPageLayout_Orientation(long __this__nativeId, int orientation);
/**
* See QPagedPaintDevice::setPageRanges(QPageRanges)
*/
@io.qt.QtUninvokable
public void setPageRanges(io.qt.gui.QPageRanges ranges){
setPageRanges_native_cref_QPageRanges(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(ranges));
}
private native static void setPageRanges_native_cref_QPageRanges(long __this__nativeId, long ranges);
/**
* See QPagedPaintDevice::setPageSize(QPageSize)
*/
@io.qt.QtUninvokable
public boolean setPageSize(io.qt.gui.QPageSize pageSize){
return setPageSize_native_cref_QPageSize(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(pageSize));
}
private native static boolean setPageSize_native_cref_QPageSize(long __this__nativeId, long pageSize);
@io.qt.QtUninvokable
protected io.qt.gui.QPainter sharedPainter(){
return sharedPainter_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native static io.qt.gui.QPainter sharedPainter_native_constfct(long __this__nativeId);
public native static double devicePixelRatioFScale();
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QPrinter(QPrivateConstructor p) { super(p); }
}