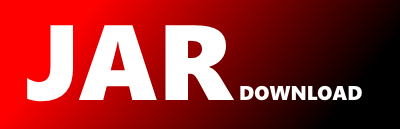
io.qt.qt3d.render.QPaintedTextureImage Maven / Gradle / Ivy
package io.qt.qt3d.render;
import io.qt.*;
/**
* A QAbstractTextureImage that can be written through a QPainter
* Java wrapper for Qt class Qt3DRender::QPaintedTextureImage
*/
public abstract class QPaintedTextureImage extends io.qt.qt3d.render.QAbstractTextureImage
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QPaintedTextureImage.class);
@NativeAccess
private static final class ConcreteWrapper extends QPaintedTextureImage {
@NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
@QtUninvokable
protected void paint(io.qt.gui.@Nullable QPainter painter){
paint_native_QPainter_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(painter));
}
@QtUninvokable
private native void paint_native_QPainter_ptr(long __this__nativeId, long painter);
}
/**
* See Qt3DRender::QPaintedTextureImage:: heightChanged(int)
*/
@QtPropertyNotify(name="height")
public final @NonNull Signal1 heightChanged = new Signal1<>();
/**
* See Qt3DRender::QPaintedTextureImage:: sizeChanged(QSize)
*/
@QtPropertyNotify(name="size")
public final @NonNull Signal1 sizeChanged = new Signal1<>();
/**
* See Qt3DRender::QPaintedTextureImage:: widthChanged(int)
*/
@QtPropertyNotify(name="width")
public final @NonNull Signal1 widthChanged = new Signal1<>();
/**
* See Qt3DRender::QPaintedTextureImage:: QPaintedTextureImage(Qt3DCore::QNode*)
* @param parent
*/
@SuppressWarnings({"exports"})
public QPaintedTextureImage(io.qt.qt3d.core.@Nullable QNode parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QPaintedTextureImage instance, io.qt.qt3d.core.QNode parent);
/**
* Function has no implementation because its native counterpart is private.
*/
@Deprecated
@QtUninvokable
@Override
protected final io.qt.qt3d.render.@Nullable QTextureImageDataGenerator dataGenerator() throws QNoImplementationException {
throw new QNoImplementationException();
}
/**
* See Qt3DRender::QPaintedTextureImage:: height()const
* @return
*/
@QtPropertyReader(name="height")
@QtUninvokable
public final int height(){
return height_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int height_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QPaintedTextureImage:: setHeight(int)
* @param h
*/
@QtPropertyWriter(name="height")
public final void setHeight(int h){
setHeight_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), h);
}
private native void setHeight_native_int(long __this__nativeId, int h);
/**
* See Qt3DRender::QPaintedTextureImage:: setSize(QSize)
* @param size
*/
@QtPropertyWriter(name="size")
public final void setSize(io.qt.core.@NonNull QSize size){
setSize_native_QSize(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(size));
}
private native void setSize_native_QSize(long __this__nativeId, long size);
/**
* See Qt3DRender::QPaintedTextureImage:: setWidth(int)
* @param w
*/
@QtPropertyWriter(name="width")
public final void setWidth(int w){
setWidth_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), w);
}
private native void setWidth_native_int(long __this__nativeId, int w);
/**
* See Qt3DRender::QPaintedTextureImage:: size()const
* @return
*/
@QtPropertyReader(name="size")
@QtUninvokable
public final io.qt.core.@NonNull QSize size(){
return size_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QSize size_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QPaintedTextureImage:: update(QRect)
* @param rect
*/
@QtUninvokable
public final void update(io.qt.core.@NonNull QRect rect){
update_native_cref_QRect(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(rect));
}
@QtUninvokable
private native void update_native_cref_QRect(long __this__nativeId, long rect);
/**
* See Qt3DRender::QPaintedTextureImage:: width()const
* @return
*/
@QtPropertyReader(name="width")
@QtUninvokable
public final int width(){
return width_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int width_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QPaintedTextureImage:: paint(QPainter*)
* @param painter
*/
@QtUninvokable
protected abstract void paint(io.qt.gui.@Nullable QPainter painter);
@QtUninvokable
private native void paint_native_QPainter_ptr(long __this__nativeId, long painter);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QPaintedTextureImage(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QPaintedTextureImage(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QPaintedTextureImage instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QPaintedTextureImage(io.qt.qt3d.core.QNode)}
* with parent = null
.
*/
public QPaintedTextureImage() {
this((io.qt.qt3d.core.QNode)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #height()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getHeight() {
return height();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #size()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QSize getSize() {
return size();
}
/**
* Overloaded function for {@link #update(io.qt.core.QRect)}
* with rect = new io.qt.core.QRect()
.
*/
@QtUninvokable
public final void update() {
update(new io.qt.core.QRect());
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #width()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getWidth() {
return width();
}
}