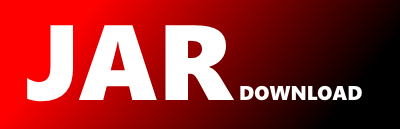
io.qt.qt3d.render.QRayCasterHit Maven / Gradle / Ivy
package io.qt.qt3d.render;
import io.qt.*;
/**
* Details of a hit when casting a ray through a model
* Java wrapper for Qt class Qt3DRender::QRayCasterHit
*/
public class QRayCasterHit extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QRayCasterHit.class);
/**
* Java wrapper for Qt enum Qt3DRender::QRayCasterHit::HitType
*/
public enum HitType implements QtEnumerator {
/**
* Representing Qt3DRender:: QRayCasterHit:: TriangleHit
*/
TriangleHit(0),
/**
* Representing Qt3DRender:: QRayCasterHit:: LineHit
*/
LineHit(1),
/**
* Representing Qt3DRender:: QRayCasterHit:: PointHit
*/
PointHit(2),
/**
* Representing Qt3DRender:: QRayCasterHit:: EntityHit
*/
EntityHit(3);
static {
QtJambi_LibraryUtilities.initialize();
}
private HitType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull HitType resolve(int value) {
switch (value) {
case 0: return TriangleHit;
case 1: return LineHit;
case 2: return PointHit;
case 3: return EntityHit;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See Qt3DRender::QRayCasterHit:: QRayCasterHit()
*/
public QRayCasterHit(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QRayCasterHit instance);
/**
* See Qt3DRender::QRayCasterHit:: QRayCasterHit(Qt3DRender::QRayCasterHit)
* @param other
*/
public QRayCasterHit(io.qt.qt3d.render.@NonNull QRayCasterHit other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QRayCasterHit instance, io.qt.qt3d.render.QRayCasterHit other);
/**
* See Qt3DRender::QRayCasterHit:: QRayCasterHit(Qt3DRender::QRayCasterHit::HitType, Qt3DCore::QNodeId, float, QVector3D, QVector3D, uint, uint, uint, uint)
* @param type
* @param id
* @param distance
* @param localIntersect
* @param worldIntersect
* @param primitiveIndex
* @param v1
* @param v2
* @param v3
*/
@SuppressWarnings({"exports"})
public QRayCasterHit(io.qt.qt3d.render.QRayCasterHit.@NonNull HitType type, io.qt.qt3d.core.@NonNull QNodeId id, float distance, io.qt.gui.@NonNull QVector3D localIntersect, io.qt.gui.@NonNull QVector3D worldIntersect, int primitiveIndex, int v1, int v2, int v3){
super((QPrivateConstructor)null);
initialize_native(this, type, id, distance, localIntersect, worldIntersect, primitiveIndex, v1, v2, v3);
}
private native static void initialize_native(QRayCasterHit instance, io.qt.qt3d.render.QRayCasterHit.HitType type, io.qt.qt3d.core.QNodeId id, float distance, io.qt.gui.QVector3D localIntersect, io.qt.gui.QVector3D worldIntersect, int primitiveIndex, int v1, int v2, int v3);
/**
* Returns the distance between the origin of the ray and the intersection point
* See Qt3DRender::QRayCasterHit:: distance()const
* @return
*/
@QtPropertyReader(name="distance")
@QtPropertyConstant
@QtUninvokable
public final float distance(){
return distance_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native float distance_native_constfct(long __this__nativeId);
/**
* Returns a pointer to the entity that was hit
* See Qt3DRender::QRayCasterHit:: entity()const
* @return
*/
@QtPropertyReader(name="entity")
@QtPropertyConstant
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.qt3d.core.@Nullable QEntity entity(){
return entity_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.qt3d.core.QEntity entity_native_constfct(long __this__nativeId);
/**
* Returns the id of the entity that was hit
* See Qt3DRender::QRayCasterHit:: entityId()const
* @return
*/
@QtPropertyReader(name="entityId")
@QtPropertyConstant
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.qt3d.core.@NonNull QNodeId entityId(){
return entityId_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.qt3d.core.QNodeId entityId_native_constfct(long __this__nativeId);
/**
* Returns the coordinates of the intersection point in the entity's coordinate system
* See Qt3DRender::QRayCasterHit:: localIntersection()const
* @return
*/
@QtPropertyReader(name="localIntersection")
@QtPropertyConstant
@QtUninvokable
public final io.qt.gui.@NonNull QVector3D localIntersection(){
return localIntersection_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.gui.QVector3D localIntersection_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QRayCasterHit:: operator=(Qt3DRender::QRayCasterHit)
* @param other
*/
@QtUninvokable
public final void assign(io.qt.qt3d.render.@NonNull QRayCasterHit other){
assign_native_cref_Qt3DRender_QRayCasterHit(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@QtUninvokable
private native void assign_native_cref_Qt3DRender_QRayCasterHit(long __this__nativeId, long other);
/**
* Returns the index of the picked primitive
* See Qt3DRender::QRayCasterHit:: primitiveIndex()const
* @return
*/
@QtPropertyReader(name="primitiveIndex")
@QtPropertyConstant
@QtUninvokable
public final int primitiveIndex(){
return primitiveIndex_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int primitiveIndex_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QRayCasterHit:: toString()
* @return
*/
public final java.lang.@NonNull String toString(){
return toString_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native java.lang.String toString_native(long __this__nativeId);
/**
* Returns the type of the hit
* See Qt3DRender::QRayCasterHit:: type()const
* @return
*/
@QtPropertyReader(name="type")
@QtPropertyConstant
@QtUninvokable
public final io.qt.qt3d.render.QRayCasterHit.@NonNull HitType type(){
return io.qt.qt3d.render.QRayCasterHit.HitType.resolve(type_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int type_native_constfct(long __this__nativeId);
/**
* Returns the index of the first vertex of the picked primitive
* See Qt3DRender::QRayCasterHit:: vertex1Index()const
* @return
*/
@QtPropertyReader(name="vertex1Index")
@QtPropertyConstant
@QtUninvokable
public final int vertex1Index(){
return vertex1Index_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int vertex1Index_native_constfct(long __this__nativeId);
/**
* Returns the index of the second vertex of the picked primitive
* See Qt3DRender::QRayCasterHit:: vertex2Index()const
* @return
*/
@QtPropertyReader(name="vertex2Index")
@QtPropertyConstant
@QtUninvokable
public final int vertex2Index(){
return vertex2Index_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int vertex2Index_native_constfct(long __this__nativeId);
/**
* Returns the index of the third vertex of the picked primitive
* See Qt3DRender::QRayCasterHit:: vertex3Index()const
* @return
*/
@QtPropertyReader(name="vertex3Index")
@QtPropertyConstant
@QtUninvokable
public final int vertex3Index(){
return vertex3Index_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int vertex3Index_native_constfct(long __this__nativeId);
/**
* Returns the coordinates of the intersection point in the model's coordinate system
* See Qt3DRender::QRayCasterHit:: worldIntersection()const
* @return
*/
@QtPropertyReader(name="worldIntersection")
@QtPropertyConstant
@QtUninvokable
public final io.qt.gui.@NonNull QVector3D worldIntersection(){
return worldIntersection_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.gui.QVector3D worldIntersection_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QRayCasterHit(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See Qt3DRender::QRayCasterHit:: QRayCasterHit(Qt3DRender::QRayCasterHit)
*/
@QtUninvokable
@Override
public QRayCasterHit clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native QRayCasterHit clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #distance()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final float getDistance() {
return distance();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #entity()} instead.
*/
@QtPropertyReader(enabled=false)
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.qt3d.core.@Nullable QEntity getEntity() {
return entity();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #entityId()} instead.
*/
@QtPropertyReader(enabled=false)
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.qt3d.core.@NonNull QNodeId getEntityId() {
return entityId();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #localIntersection()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.gui.@NonNull QVector3D getLocalIntersection() {
return localIntersection();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #primitiveIndex()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getPrimitiveIndex() {
return primitiveIndex();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #type()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.qt3d.render.QRayCasterHit.@NonNull HitType getType() {
return type();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #vertex1Index()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getVertex1Index() {
return vertex1Index();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #vertex2Index()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getVertex2Index() {
return vertex2Index();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #vertex3Index()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getVertex3Index() {
return vertex3Index();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #worldIntersection()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.gui.@NonNull QVector3D getWorldIntersection() {
return worldIntersection();
}
}