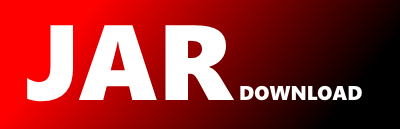
io.qt.qt3d.render.QRenderTargetOutput Maven / Gradle / Ivy
package io.qt.qt3d.render;
import io.qt.*;
/**
* Allows the specification of an attachment of a render target (whether it is a color texture, a depth texture, etc... )
* Java wrapper for Qt class Qt3DRender::QRenderTargetOutput
*/
public class QRenderTargetOutput extends io.qt.qt3d.core.QNode
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QRenderTargetOutput.class);
/**
* Java wrapper for Qt enum Qt3DRender::QRenderTargetOutput::AttachmentPoint
*/
public enum AttachmentPoint implements QtEnumerator {
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color0
*/
Color0(0),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color1
*/
Color1(1),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color2
*/
Color2(2),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color3
*/
Color3(3),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color4
*/
Color4(4),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color5
*/
Color5(5),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color6
*/
Color6(6),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color7
*/
Color7(7),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color8
*/
Color8(8),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color9
*/
Color9(9),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color10
*/
Color10(10),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color11
*/
Color11(11),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color12
*/
Color12(12),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color13
*/
Color13(13),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color14
*/
Color14(14),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Color15
*/
Color15(15),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Depth
*/
Depth(16),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Stencil
*/
Stencil(17),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: DepthStencil
*/
DepthStencil(18),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Left
*/
Left(19),
/**
* Representing Qt3DRender:: QRenderTargetOutput:: Right
*/
Right(20);
static {
QtJambi_LibraryUtilities.initialize();
}
private AttachmentPoint(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull AttachmentPoint resolve(int value) {
switch (value) {
case 0: return Color0;
case 1: return Color1;
case 2: return Color2;
case 3: return Color3;
case 4: return Color4;
case 5: return Color5;
case 6: return Color6;
case 7: return Color7;
case 8: return Color8;
case 9: return Color9;
case 10: return Color10;
case 11: return Color11;
case 12: return Color12;
case 13: return Color13;
case 14: return Color14;
case 15: return Color15;
case 16: return Depth;
case 17: return Stencil;
case 18: return DepthStencil;
case 19: return Left;
case 20: return Right;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
*
*/
@QtPropertyNotify(name="attachmentPoint")
public final @NonNull Signal1 attachmentPointChanged = new Signal1<>();
/**
* See Qt3DRender::QRenderTargetOutput:: faceChanged(Qt3DRender::QAbstractTexture::CubeMapFace)
*/
@QtPropertyNotify(name="face")
public final @NonNull Signal1 faceChanged = new Signal1<>();
/**
* See Qt3DRender::QRenderTargetOutput:: layerChanged(int)
*/
@QtPropertyNotify(name="layer")
public final @NonNull Signal1 layerChanged = new Signal1<>();
/**
* See Qt3DRender::QRenderTargetOutput:: mipLevelChanged(int)
*/
@QtPropertyNotify(name="mipLevel")
public final @NonNull Signal1 mipLevelChanged = new Signal1<>();
/**
* See Qt3DRender::QRenderTargetOutput:: textureChanged(Qt3DRender::QAbstractTexture*)
*/
@QtPropertyNotify(name="texture")
public final @NonNull Signal1 textureChanged = new Signal1<>();
/**
* See Qt3DRender::QRenderTargetOutput:: QRenderTargetOutput(Qt3DCore::QNode*)
* @param parent
*/
@SuppressWarnings({"exports"})
public QRenderTargetOutput(io.qt.qt3d.core.@Nullable QNode parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QRenderTargetOutput instance, io.qt.qt3d.core.QNode parent);
/**
* See Qt3DRender::QRenderTargetOutput:: attachmentPoint()const
* @return
*/
@QtPropertyReader(name="attachmentPoint")
@QtUninvokable
public final io.qt.qt3d.render.QRenderTargetOutput.@NonNull AttachmentPoint attachmentPoint(){
return io.qt.qt3d.render.QRenderTargetOutput.AttachmentPoint.resolve(attachmentPoint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int attachmentPoint_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QRenderTargetOutput:: face()const
* @return
*/
@QtPropertyReader(name="face")
@QtUninvokable
public final io.qt.qt3d.render.QAbstractTexture.@NonNull CubeMapFace face(){
return io.qt.qt3d.render.QAbstractTexture.CubeMapFace.resolve(face_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int face_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QRenderTargetOutput:: layer()const
* @return
*/
@QtPropertyReader(name="layer")
@QtUninvokable
public final int layer(){
return layer_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int layer_native_constfct(long __this__nativeId);
/**
* See Qt3DRender::QRenderTargetOutput:: mipLevel()const
* @return
*/
@QtPropertyReader(name="mipLevel")
@QtUninvokable
public final int mipLevel(){
return mipLevel_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int mipLevel_native_constfct(long __this__nativeId);
/**
*
* @param attachmentPoint
*/
@QtPropertyWriter(name="attachmentPoint")
public final void setAttachmentPoint(io.qt.qt3d.render.QRenderTargetOutput.@NonNull AttachmentPoint attachmentPoint){
setAttachmentPoint_native_Qt3DRender_QRenderTargetOutput_AttachmentPoint(QtJambi_LibraryUtilities.internal.nativeId(this), attachmentPoint.value());
}
private native void setAttachmentPoint_native_Qt3DRender_QRenderTargetOutput_AttachmentPoint(long __this__nativeId, int attachmentPoint);
/**
* See Qt3DRender::QRenderTargetOutput:: setFace(Qt3DRender::QAbstractTexture::CubeMapFace)
* @param face
*/
@QtPropertyWriter(name="face")
public final void setFace(io.qt.qt3d.render.QAbstractTexture.@NonNull CubeMapFace face){
setFace_native_Qt3DRender_QAbstractTexture_CubeMapFace(QtJambi_LibraryUtilities.internal.nativeId(this), face.value());
}
private native void setFace_native_Qt3DRender_QAbstractTexture_CubeMapFace(long __this__nativeId, int face);
/**
* See Qt3DRender::QRenderTargetOutput:: setLayer(int)
* @param layer
*/
@QtPropertyWriter(name="layer")
public final void setLayer(int layer){
setLayer_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), layer);
}
private native void setLayer_native_int(long __this__nativeId, int layer);
/**
* See Qt3DRender::QRenderTargetOutput:: setMipLevel(int)
* @param level
*/
@QtPropertyWriter(name="mipLevel")
public final void setMipLevel(int level){
setMipLevel_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), level);
}
private native void setMipLevel_native_int(long __this__nativeId, int level);
/**
* See Qt3DRender::QRenderTargetOutput:: setTexture(Qt3DRender::QAbstractTexture*)
* @param texture
*/
@QtPropertyWriter(name="texture")
public final void setTexture(io.qt.qt3d.render.@Nullable QAbstractTexture texture){
setTexture_native_Qt3DRender_QAbstractTexture_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(texture));
}
private native void setTexture_native_Qt3DRender_QAbstractTexture_ptr(long __this__nativeId, long texture);
/**
* See Qt3DRender::QRenderTargetOutput:: texture()const
* @return
*/
@QtPropertyReader(name="texture")
@QtUninvokable
public final io.qt.qt3d.render.@Nullable QAbstractTexture texture(){
return texture_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.qt3d.render.QAbstractTexture texture_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QRenderTargetOutput(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QRenderTargetOutput(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QRenderTargetOutput instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QRenderTargetOutput(io.qt.qt3d.core.QNode)}
* with parent = null
.
*/
public QRenderTargetOutput() {
this((io.qt.qt3d.core.QNode)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #attachmentPoint()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.qt3d.render.QRenderTargetOutput.@NonNull AttachmentPoint getAttachmentPoint() {
return attachmentPoint();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #face()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.qt3d.render.QAbstractTexture.@NonNull CubeMapFace getFace() {
return face();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #layer()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getLayer() {
return layer();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #mipLevel()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final int getMipLevel() {
return mipLevel();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #texture()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.qt3d.render.@Nullable QAbstractTexture getTexture() {
return texture();
}
}