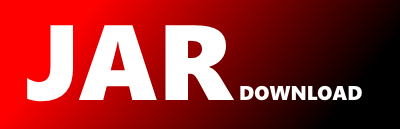
io.qt.quick.widgets.QQuickWidget Maven / Gradle / Ivy
Show all versions of qtjambi-quickwidgets Show documentation
package io.qt.quick.widgets;
import io.qt.*;
/**
* Widget for displaying a Qt Quick user interface
* Java wrapper for Qt class QQuickWidget
*/
public class QQuickWidget extends io.qt.widgets.QWidget
{
static {
QtJambi_LibraryUtilities.initialize();
}
@QtPropertyMember(enabled=false)
private Object __rcQmlComponent;
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QQuickWidget.class);
/**
* Java wrapper for Qt enum QQuickWidget::ResizeMode
*/
public enum ResizeMode implements QtEnumerator {
/**
* Representing QQuickWidget:: SizeViewToRootObject
*/
SizeViewToRootObject(0),
/**
* Representing QQuickWidget:: SizeRootObjectToView
*/
SizeRootObjectToView(1);
static {
QtJambi_LibraryUtilities.initialize();
}
private ResizeMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull ResizeMode resolve(int value) {
switch (value) {
case 0: return SizeViewToRootObject;
case 1: return SizeRootObjectToView;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QQuickWidget::Status
*/
public enum Status implements QtEnumerator {
/**
* Representing QQuickWidget:: Null
*/
Null(0),
/**
* Representing QQuickWidget:: Ready
*/
Ready(1),
/**
* Representing QQuickWidget:: Loading
*/
Loading(2),
/**
* Representing QQuickWidget:: Error
*/
Error(3);
static {
QtJambi_LibraryUtilities.initialize();
}
private Status(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
@Override
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static @NonNull Status resolve(int value) {
switch (value) {
case 0: return Null;
case 1: return Ready;
case 2: return Loading;
case 3: return Error;
default: throw new QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QQuickWidget:: sceneGraphError(QQuickWindow::SceneGraphError, QString)
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal2 sceneGraphError = new Signal2<>();
/**
* See QQuickWidget:: statusChanged(QQuickWidget::Status)
*/
@QtPropertyNotify(name="status")
public final @NonNull Signal1 statusChanged = new Signal1<>();
/**
* See QQuickWidget:: QQuickWidget(QQmlEngine*, QWidget*)
* @param engine
* @param parent
*/
@SuppressWarnings({"exports"})
public QQuickWidget(io.qt.qml.@Nullable QQmlEngine engine, io.qt.widgets.@Nullable QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, engine, parent);
}
private native static void initialize_native(QQuickWidget instance, io.qt.qml.QQmlEngine engine, io.qt.widgets.QWidget parent);
/**
* See QQuickWidget:: QQuickWidget(QUrl, QWidget*)
* @param source
* @param parent
*/
public QQuickWidget(io.qt.core.@NonNull QUrl source, io.qt.widgets.@Nullable QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, source, parent);
}
private native static void initialize_native(QQuickWidget instance, io.qt.core.QUrl source, io.qt.widgets.QWidget parent);
/**
* See QQuickWidget:: QQuickWidget(QWidget*)
* @param parent
*/
public QQuickWidget(io.qt.widgets.@Nullable QWidget parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QQuickWidget instance, io.qt.widgets.QWidget parent);
/**
* See QQuickWidget:: engine()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.qml.@Nullable QQmlEngine engine(){
return engine_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.qml.QQmlEngine engine_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: errors()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.core.@NonNull QList errors(){
return errors_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QList errors_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: format()const
* @return
*/
@QtUninvokable
public final io.qt.gui.@NonNull QSurfaceFormat format(){
return format_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.gui.QSurfaceFormat format_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: grabFramebuffer()const
* @return
*/
@QtUninvokable
public final io.qt.gui.@NonNull QImage grabFramebuffer(){
return grabFramebuffer_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.gui.QImage grabFramebuffer_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: initialSize()const
* @return
*/
@QtUninvokable
public final io.qt.core.@NonNull QSize initialSize(){
return initialSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QSize initialSize_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: quickWindow()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.quick.@Nullable QQuickWindow quickWindow(){
return quickWindow_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.quick.QQuickWindow quickWindow_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: resizeMode()const
* @return
*/
@QtPropertyReader(name="resizeMode")
@QtUninvokable
public final io.qt.quick.widgets.QQuickWidget.@NonNull ResizeMode resizeMode(){
return io.qt.quick.widgets.QQuickWidget.ResizeMode.resolve(resizeMode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int resizeMode_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: rootContext()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.qml.@Nullable QQmlContext rootContext(){
return rootContext_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.qml.QQmlContext rootContext_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: rootObject()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.quick.@Nullable QQuickItem rootObject(){
return rootObject_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.quick.QQuickItem rootObject_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: setClearColor(QColor)
* @param color
*/
@QtUninvokable
public final void setClearColor(io.qt.gui.@NonNull QColor color){
setClearColor_native_cref_QColor(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(color));
}
@QtUninvokable
private native void setClearColor_native_cref_QColor(long __this__nativeId, long color);
/**
* See QQuickWidget:: setContent(QUrl, QQmlComponent*, QObject*)
* @param url
* @param component
* @param item
*/
@SuppressWarnings({"exports"})
public final void setContent(io.qt.core.@NonNull QUrl url, io.qt.qml.@Nullable QQmlComponent component, io.qt.core.@Nullable QObject item){
InternalAccess.NativeIdInfo __component__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(component);
setContent_native_cref_QUrl_QQmlComponent_ptr_QObject_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(url), __component__NativeIdInfo.nativeId(), QtJambi_LibraryUtilities.internal.checkedNativeId(item));
if (__component__NativeIdInfo.needsReferenceCounting()) {
__rcQmlComponent = component;
}else{
__rcQmlComponent = null;
}
}
private native void setContent_native_cref_QUrl_QQmlComponent_ptr_QObject_ptr(long __this__nativeId, long url, long component, long item);
/**
* See QQuickWidget:: setFormat(QSurfaceFormat)
* @param format
*/
@QtUninvokable
public final void setFormat(io.qt.gui.@NonNull QSurfaceFormat format){
setFormat_native_cref_QSurfaceFormat(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(format));
}
@QtUninvokable
private native void setFormat_native_cref_QSurfaceFormat(long __this__nativeId, long format);
/**
* See QQuickWidget:: setResizeMode(QQuickWidget::ResizeMode)
* @param arg__1
*/
@QtPropertyWriter(name="resizeMode")
@QtUninvokable
public final void setResizeMode(io.qt.quick.widgets.QQuickWidget.@NonNull ResizeMode arg__1){
setResizeMode_native_QQuickWidget_ResizeMode(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1.value());
}
@QtUninvokable
private native void setResizeMode_native_QQuickWidget_ResizeMode(long __this__nativeId, int arg__1);
/**
* See QQuickWidget:: setSource(QUrl)
* @param arg__1
*/
@QtPropertyWriter(name="source")
public final void setSource(io.qt.core.@NonNull QUrl arg__1){
setSource_native_cref_QUrl(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
private native void setSource_native_cref_QUrl(long __this__nativeId, long arg__1);
/**
* See QQuickWidget:: source()const
* @return
*/
@QtPropertyReader(name="source")
@QtPropertyDesignable
@QtUninvokable
public final io.qt.core.@NonNull QUrl source(){
return source_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QUrl source_native_constfct(long __this__nativeId);
/**
* See QQuickWidget:: status()const
* @return
*/
@QtPropertyReader(name="status")
@QtUninvokable
public final io.qt.quick.widgets.QQuickWidget.@NonNull Status status(){
return io.qt.quick.widgets.QQuickWidget.Status.resolve(status_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int status_native_constfct(long __this__nativeId);
/**
* See QWidget:: dragEnterEvent(QDragEnterEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void dragEnterEvent(io.qt.gui.@Nullable QDragEnterEvent arg__1){
dragEnterEvent_native_QDragEnterEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void dragEnterEvent_native_QDragEnterEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: dragLeaveEvent(QDragLeaveEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void dragLeaveEvent(io.qt.gui.@Nullable QDragLeaveEvent arg__1){
dragLeaveEvent_native_QDragLeaveEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void dragLeaveEvent_native_QDragLeaveEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: dragMoveEvent(QDragMoveEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void dragMoveEvent(io.qt.gui.@Nullable QDragMoveEvent arg__1){
dragMoveEvent_native_QDragMoveEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void dragMoveEvent_native_QDragMoveEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: dropEvent(QDropEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void dropEvent(io.qt.gui.@Nullable QDropEvent arg__1){
dropEvent_native_QDropEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void dropEvent_native_QDropEvent_ptr(long __this__nativeId, long arg__1);
/**
*
* @param arg__1
* @return
*/
@QtUninvokable
@Override
public boolean event(io.qt.core.@Nullable QEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: focusInEvent(QFocusEvent*)
* @param event
*/
@QtUninvokable
@Override
protected void focusInEvent(io.qt.gui.@Nullable QFocusEvent event){
focusInEvent_native_QFocusEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void focusInEvent_native_QFocusEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget:: focusNextPrevChild(bool)
* @param next
* @return
*/
@QtUninvokable
@Override
protected boolean focusNextPrevChild(boolean next){
return focusNextPrevChild_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), next);
}
@QtUninvokable
private native boolean focusNextPrevChild_native_bool(long __this__nativeId, boolean next);
/**
* See QWidget:: focusOutEvent(QFocusEvent*)
* @param event
*/
@QtUninvokable
@Override
protected void focusOutEvent(io.qt.gui.@Nullable QFocusEvent event){
focusOutEvent_native_QFocusEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void focusOutEvent_native_QFocusEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget:: hideEvent(QHideEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void hideEvent(io.qt.gui.@Nullable QHideEvent arg__1){
hideEvent_native_QHideEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void hideEvent_native_QHideEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: keyPressEvent(QKeyEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void keyPressEvent(io.qt.gui.@Nullable QKeyEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
keyPressEvent_native_QKeyEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void keyPressEvent_native_QKeyEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: keyReleaseEvent(QKeyEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void keyReleaseEvent(io.qt.gui.@Nullable QKeyEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
keyReleaseEvent_native_QKeyEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void keyReleaseEvent_native_QKeyEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: mouseDoubleClickEvent(QMouseEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void mouseDoubleClickEvent(io.qt.gui.@Nullable QMouseEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
mouseDoubleClickEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void mouseDoubleClickEvent_native_QMouseEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: mouseMoveEvent(QMouseEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void mouseMoveEvent(io.qt.gui.@Nullable QMouseEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
mouseMoveEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void mouseMoveEvent_native_QMouseEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: mousePressEvent(QMouseEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void mousePressEvent(io.qt.gui.@Nullable QMouseEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
mousePressEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void mousePressEvent_native_QMouseEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: mouseReleaseEvent(QMouseEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void mouseReleaseEvent(io.qt.gui.@Nullable QMouseEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
mouseReleaseEvent_native_QMouseEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void mouseReleaseEvent_native_QMouseEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: paintEvent(QPaintEvent*)
* @param event
*/
@QtUninvokable
@Override
protected void paintEvent(io.qt.gui.@Nullable QPaintEvent event){
paintEvent_native_QPaintEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@QtUninvokable
private native void paintEvent_native_QPaintEvent_ptr(long __this__nativeId, long event);
/**
* See QWidget:: resizeEvent(QResizeEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void resizeEvent(io.qt.gui.@Nullable QResizeEvent arg__1){
resizeEvent_native_QResizeEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void resizeEvent_native_QResizeEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: showEvent(QShowEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void showEvent(io.qt.gui.@Nullable QShowEvent arg__1){
showEvent_native_QShowEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void showEvent_native_QShowEvent_ptr(long __this__nativeId, long arg__1);
/**
*
* @return
*/
@QtUninvokable
@Override
public io.qt.core.@NonNull QSize sizeHint(){
return sizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QSize sizeHint_native_constfct(long __this__nativeId);
/**
* See QObject:: timerEvent(QTimerEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void timerEvent(io.qt.core.@Nullable QTimerEvent arg__1){
timerEvent_native_QTimerEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void timerEvent_native_QTimerEvent_ptr(long __this__nativeId, long arg__1);
/**
* See QWidget:: wheelEvent(QWheelEvent*)
* @param arg__1
*/
@QtUninvokable
@Override
protected void wheelEvent(io.qt.gui.@Nullable QWheelEvent arg__1){
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
wheelEvent_native_QWheelEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(arg__1));
}
@QtUninvokable
private native void wheelEvent_native_QWheelEvent_ptr(long __this__nativeId, long arg__1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QQuickWidget(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QQuickWidget(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QQuickWidget instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QQuickWidget(io.qt.core.QUrl, io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QQuickWidget(io.qt.core.@NonNull QUrl source) {
this(source, (io.qt.widgets.QWidget)null);
}
/**
* Overloaded constructor for {@link #QQuickWidget(java.lang.String, io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QQuickWidget(java.lang.@NonNull String source) {
this(source, (io.qt.widgets.QWidget)null);
}
/**
* Overloaded constructor for {@link #QQuickWidget(io.qt.core.QUrl, io.qt.widgets.QWidget)}.
*/
public QQuickWidget(java.lang.@NonNull String source, io.qt.widgets.@Nullable QWidget parent) {
this(new io.qt.core.QUrl(source), parent);
}
/**
* Overloaded constructor for {@link #QQuickWidget(io.qt.widgets.QWidget)}
* with parent = null
.
*/
public QQuickWidget() {
this((io.qt.widgets.QWidget)null);
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #resizeMode()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.quick.widgets.QQuickWidget.@NonNull ResizeMode getResizeMode() {
return resizeMode();
}
/**
* Overloaded function for {@link #setClearColor(io.qt.gui.QColor)}.
*/
@QtUninvokable
public final void setClearColor(io.qt.core.Qt.@NonNull GlobalColor color) {
setClearColor(new io.qt.gui.QColor(color));
}
/**
* Overloaded function for {@link #setClearColor(io.qt.gui.QColor)}.
*/
@QtUninvokable
public final void setClearColor(io.qt.gui.@NonNull QRgba64 color) {
setClearColor(new io.qt.gui.QColor(color));
}
/**
* Overloaded function for {@link #setClearColor(io.qt.gui.QColor)}.
*/
@QtUninvokable
public final void setClearColor(java.lang.@NonNull String color) {
setClearColor(new io.qt.gui.QColor(color));
}
/**
* Overloaded function for {@link #setContent(io.qt.core.QUrl, io.qt.qml.QQmlComponent, io.qt.core.QObject)}.
*/
@SuppressWarnings({"exports"})
public final void setContent(java.lang.@NonNull String url, io.qt.qml.@Nullable QQmlComponent component, io.qt.core.@Nullable QObject item) {
setContent(new io.qt.core.QUrl(url), component, item);
}
/**
* Overloaded function for {@link #setFormat(io.qt.gui.QSurfaceFormat)}.
*/
@QtUninvokable
public final void setFormat(io.qt.gui.QSurfaceFormat.@NonNull FormatOptions format) {
setFormat(new io.qt.gui.QSurfaceFormat(format));
}
/**
* Overloaded function for {@link #setSource(io.qt.core.QUrl)}.
*/
public final void setSource(java.lang.@NonNull String arg__1) {
setSource(new io.qt.core.QUrl(arg__1));
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #source()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.core.@NonNull QUrl getSource() {
return source();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #status()} instead.
*/
@QtPropertyReader(enabled=false)
@QtUninvokable
public final io.qt.quick.widgets.QQuickWidget.@NonNull Status getStatus() {
return status();
}
}