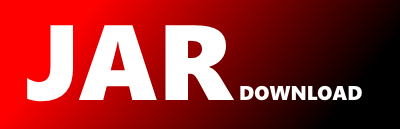
io.qt.scxml.QScxmlCompiler Maven / Gradle / Ivy
package io.qt.scxml;
import io.qt.*;
/**
* Compiler for SCXML files
* Java wrapper for Qt class QScxmlCompiler
*/
public class QScxmlCompiler extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
@QtPropertyMember(enabled=false)
private Object __rcLoader;
/**
* URI resolver and resource loader for an SCXML compiler
* Java wrapper for Qt class QScxmlCompiler::Loader
*/
public static interface Loader extends QtObjectInterface
{
/**
* @hidden
* Implementor class for interface {@link io.qt.scxml.QScxmlCompiler.Loader}
*/
public static abstract class Impl extends QtObject
implements io.qt.scxml.QScxmlCompiler.Loader
{
static {
QtJambi_LibraryUtilities.initialize();
}
@NativeAccess
private static final class ConcreteWrapper extends QScxmlCompiler.Loader.Impl {
@NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
@QtUninvokable
public io.qt.core.@NonNull QByteArray load(java.lang.@NonNull String name, java.lang.@NonNull String baseDir, java.util.@Nullable Collection errors){
return load_native_cref_QString_cref_QString_QStringList_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), name, baseDir, errors);
}
private native static io.qt.core.QByteArray load_native_cref_QString_cref_QString_QStringList_ptr(long __this__nativeId, java.lang.String name, java.lang.String baseDir, java.util.Collection errors);
}
/**
* See QScxmlCompiler::Loader:: Loader()
*/
public Impl(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(Loader instance);
/**
* See QScxmlCompiler::Loader:: load(QString, QString, QStringList*)
* @param name
* @param baseDir
* @param errors
* @return
*/
@QtUninvokable
public abstract io.qt.core.@NonNull QByteArray load(java.lang.@NonNull String name, java.lang.@NonNull String baseDir, java.util.@Nullable Collection errors);
private native static io.qt.core.QByteArray load_native_cref_QString_cref_QString_QStringList_ptr(long __this__nativeId, java.lang.String name, java.lang.String baseDir, java.util.Collection errors);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected Impl(QPrivateConstructor p) { super(p); }
}
/**
* See QScxmlCompiler::Loader:: load(QString, QString, QStringList*)
* @param name
* @param baseDir
* @param errors
* @return
*/
@QtUninvokable
public io.qt.core.@NonNull QByteArray load(java.lang.@NonNull String name, java.lang.@NonNull String baseDir, java.util.@Nullable Collection errors);
}
/**
* See QScxmlCompiler:: QScxmlCompiler(QScxmlCompiler)
* @param other
*/
public QScxmlCompiler(io.qt.scxml.@StrictNonNull QScxmlCompiler other){
super((QPrivateConstructor)null);
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
initialize_native(this, other);
}
private native static void initialize_native(QScxmlCompiler instance, io.qt.scxml.QScxmlCompiler other);
/**
* See QScxmlCompiler:: QScxmlCompiler(QXmlStreamReader*)
* @param xmlReader
*/
public QScxmlCompiler(io.qt.core.@Nullable QXmlStreamReader xmlReader){
super((QPrivateConstructor)null);
initialize_native(this, xmlReader);
}
private native static void initialize_native(QScxmlCompiler instance, io.qt.core.QXmlStreamReader xmlReader);
/**
*
* @return
*/
@QtUninvokable
public final io.qt.scxml.@Nullable QScxmlStateMachine compile(){
return compile_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.scxml.QScxmlStateMachine compile_native(long __this__nativeId);
/**
* See QScxmlCompiler:: errors()const
* @return
*/
@QtUninvokable
public final io.qt.core.@NonNull QList errors(){
return errors_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QList errors_native_constfct(long __this__nativeId);
/**
* See QScxmlCompiler:: fileName()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String fileName(){
return fileName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String fileName_native_constfct(long __this__nativeId);
/**
* See QScxmlCompiler:: loader()const
* @return
*/
@QtUninvokable
public final io.qt.scxml.QScxmlCompiler.@Nullable Loader loader(){
return loader_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.scxml.QScxmlCompiler.Loader loader_native_constfct(long __this__nativeId);
/**
* See QScxmlCompiler:: setFileName(QString)
* @param fileName
*/
@QtUninvokable
public final void setFileName(java.lang.@NonNull String fileName){
setFileName_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), fileName);
}
@QtUninvokable
private native void setFileName_native_cref_QString(long __this__nativeId, java.lang.String fileName);
/**
* See QScxmlCompiler:: setLoader(QScxmlCompiler::Loader*)
* @param newLoader
*/
@QtUninvokable
public final void setLoader(io.qt.scxml.QScxmlCompiler.@Nullable Loader newLoader){
InternalAccess.NativeIdInfo __newLoader__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(newLoader);
setLoader_native_QScxmlCompiler_Loader_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __newLoader__NativeIdInfo.nativeId());
if (__newLoader__NativeIdInfo.needsReferenceCounting()) {
__rcLoader = newLoader;
}else{
__rcLoader = null;
}
}
@QtUninvokable
private native void setLoader_native_QScxmlCompiler_Loader_ptr(long __this__nativeId, long newLoader);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QScxmlCompiler(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
*/
@QtUninvokable
@Override
public QScxmlCompiler clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native QScxmlCompiler clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy