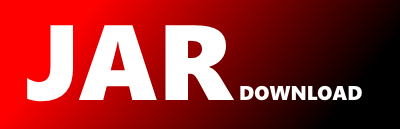
io.qt.scxml.QScxmlExecutableContent Maven / Gradle / Ivy
Show all versions of qtjambi-scxml Show documentation
package io.qt.scxml;
import io.qt.*;
/**
* Contains various types used to interpret executable content in state machines
* Java wrapper for Qt header file QScxmlExecutableContent
*/
public final class QScxmlExecutableContent
{
static {
QtJambi_LibraryUtilities.initialize();
}
private QScxmlExecutableContent() throws java.lang.InstantiationError { throw new java.lang.InstantiationError("Cannot instantiate namespace QScxmlExecutableContent."); }
/**
* Represents a unit of executable content
* Java wrapper for Qt class QScxmlExecutableContent::EvaluatorInfo
*/
public static class EvaluatorInfo extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QScxmlExecutableContent::EvaluatorInfo:: EvaluatorInfo()
*/
public EvaluatorInfo(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(EvaluatorInfo instance);
/**
* See QScxmlExecutableContent::EvaluatorInfo:: EvaluatorInfo(QScxmlExecutableContent::EvaluatorInfo)
* @param other
*/
public EvaluatorInfo(io.qt.scxml.QScxmlExecutableContent.@NonNull EvaluatorInfo other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(EvaluatorInfo instance, io.qt.scxml.QScxmlExecutableContent.EvaluatorInfo other);
/**
* See QScxmlExecutableContent::EvaluatorInfo:: EvaluatorInfo{qint32, qint32}
* @param expr
* @param context
*/
public EvaluatorInfo(int expr, int context){
super((QPrivateConstructor)null);
initialize_native(this, expr, context);
}
private native static void initialize_native(EvaluatorInfo instance, int expr, int context);
/**
* For evaluating the expression
* See QScxmlExecutableContent::EvaluatorInfo:: context
* @param context
*/
@QtUninvokable
public final void setContext(int context){
setContext_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), context);
}
@QtUninvokable
private native void setContext_native_qint32(long __this__nativeId, int context);
/**
* For evaluating the expression
* See QScxmlExecutableContent::EvaluatorInfo:: context
* @return
*/
@QtUninvokable
public final int context(){
return context_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int context_native(long __this__nativeId);
/**
* Expression to be evaluated
* See QScxmlExecutableContent::EvaluatorInfo:: expr
* @param expr
*/
@QtUninvokable
public final void setExpr(int expr){
setExpr_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), expr);
}
@QtUninvokable
private native void setExpr_native_qint32(long __this__nativeId, int expr);
/**
* Expression to be evaluated
* See QScxmlExecutableContent::EvaluatorInfo:: expr
* @return
*/
@QtUninvokable
public final int expr(){
return expr_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int expr_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected EvaluatorInfo(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See QScxmlExecutableContent::EvaluatorInfo:: EvaluatorInfo(QScxmlExecutableContent::EvaluatorInfo)
*/
@QtUninvokable
@Override
public EvaluatorInfo clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native EvaluatorInfo clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #context()} instead.
*/
@QtUninvokable
public final int getContext() {
return context();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #expr()} instead.
*/
@QtUninvokable
public final int getExpr() {
return expr();
}
}
/**
* AssingmentInfo class represents a data assignment
* Java wrapper for Qt class QScxmlExecutableContent::AssignmentInfo
*/
public static class AssignmentInfo extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QScxmlExecutableContent::AssignmentInfo:: AssignmentInfo()
*/
public AssignmentInfo(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(AssignmentInfo instance);
/**
* See QScxmlExecutableContent::AssignmentInfo:: AssignmentInfo(QScxmlExecutableContent::AssignmentInfo)
* @param other
*/
public AssignmentInfo(io.qt.scxml.QScxmlExecutableContent.@NonNull AssignmentInfo other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(AssignmentInfo instance, io.qt.scxml.QScxmlExecutableContent.AssignmentInfo other);
/**
* See QScxmlExecutableContent::AssignmentInfo:: AssignmentInfo{qint32, qint32, qint32}
* @param dest
* @param expr
* @param context
*/
public AssignmentInfo(int dest, int expr, int context){
super((QPrivateConstructor)null);
initialize_native(this, dest, expr, context);
}
private native static void initialize_native(AssignmentInfo instance, int dest, int expr, int context);
/**
* For evaluating the expression
* See QScxmlExecutableContent::AssignmentInfo:: context
* @param context
*/
@QtUninvokable
public final void setContext(int context){
setContext_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), context);
}
@QtUninvokable
private native void setContext_native_qint32(long __this__nativeId, int context);
/**
* For evaluating the expression
* See QScxmlExecutableContent::AssignmentInfo:: context
* @return
*/
@QtUninvokable
public final int context(){
return context_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int context_native(long __this__nativeId);
/**
* Name of the data item to assign to
* See QScxmlExecutableContent::AssignmentInfo:: dest
* @param dest
*/
@QtUninvokable
public final void setDest(int dest){
setDest_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), dest);
}
@QtUninvokable
private native void setDest_native_qint32(long __this__nativeId, int dest);
/**
* Name of the data item to assign to
* See QScxmlExecutableContent::AssignmentInfo:: dest
* @return
*/
@QtUninvokable
public final int dest(){
return dest_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int dest_native(long __this__nativeId);
/**
* Expression to be evaluated
* See QScxmlExecutableContent::AssignmentInfo:: expr
* @param expr
*/
@QtUninvokable
public final void setExpr(int expr){
setExpr_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), expr);
}
@QtUninvokable
private native void setExpr_native_qint32(long __this__nativeId, int expr);
/**
* Expression to be evaluated
* See QScxmlExecutableContent::AssignmentInfo:: expr
* @return
*/
@QtUninvokable
public final int expr(){
return expr_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int expr_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected AssignmentInfo(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See QScxmlExecutableContent::AssignmentInfo:: AssignmentInfo(QScxmlExecutableContent::AssignmentInfo)
*/
@QtUninvokable
@Override
public AssignmentInfo clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native AssignmentInfo clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #context()} instead.
*/
@QtUninvokable
public final int getContext() {
return context();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #dest()} instead.
*/
@QtUninvokable
public final int getDest() {
return dest();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #expr()} instead.
*/
@QtUninvokable
public final int getExpr() {
return expr();
}
}
/**
* Represents a foreach construct
* Java wrapper for Qt class QScxmlExecutableContent::ForeachInfo
*/
public static class ForeachInfo extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QScxmlExecutableContent::ForeachInfo:: ForeachInfo()
*/
public ForeachInfo(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(ForeachInfo instance);
/**
* See QScxmlExecutableContent::ForeachInfo:: ForeachInfo(QScxmlExecutableContent::ForeachInfo)
* @param other
*/
public ForeachInfo(io.qt.scxml.QScxmlExecutableContent.@NonNull ForeachInfo other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(ForeachInfo instance, io.qt.scxml.QScxmlExecutableContent.ForeachInfo other);
/**
* See QScxmlExecutableContent::ForeachInfo:: ForeachInfo{qint32, qint32, qint32, qint32}
* @param array
* @param item
* @param index
* @param context
*/
public ForeachInfo(int array, int item, int index, int context){
super((QPrivateConstructor)null);
initialize_native(this, array, item, index, context);
}
private native static void initialize_native(ForeachInfo instance, int array, int item, int index, int context);
/**
* Name of the array that is iterated over
* See QScxmlExecutableContent::ForeachInfo:: array
* @param array
*/
@QtUninvokable
public final void setArray(int array){
setArray_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), array);
}
@QtUninvokable
private native void setArray_native_qint32(long __this__nativeId, int array);
/**
* Name of the array that is iterated over
* See QScxmlExecutableContent::ForeachInfo:: array
* @return
*/
@QtUninvokable
public final int array(){
return array_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int array_native(long __this__nativeId);
/**
* For evaluating the expression
* See QScxmlExecutableContent::ForeachInfo:: context
* @param context
*/
@QtUninvokable
public final void setContext(int context){
setContext_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), context);
}
@QtUninvokable
private native void setContext_native_qint32(long __this__nativeId, int context);
/**
* For evaluating the expression
* See QScxmlExecutableContent::ForeachInfo:: context
* @return
*/
@QtUninvokable
public final int context(){
return context_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int context_native(long __this__nativeId);
/**
* Name of the index variable
* See QScxmlExecutableContent::ForeachInfo:: index
* @param index
*/
@QtUninvokable
public final void setIndex(int index){
setIndex_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), index);
}
@QtUninvokable
private native void setIndex_native_qint32(long __this__nativeId, int index);
/**
* Name of the index variable
* See QScxmlExecutableContent::ForeachInfo:: index
* @return
*/
@QtUninvokable
public final int index(){
return index_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int index_native(long __this__nativeId);
/**
* Name of the iteration variable
* See QScxmlExecutableContent::ForeachInfo:: item
* @param item
*/
@QtUninvokable
public final void setItem(int item){
setItem_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), item);
}
@QtUninvokable
private native void setItem_native_qint32(long __this__nativeId, int item);
/**
* Name of the iteration variable
* See QScxmlExecutableContent::ForeachInfo:: item
* @return
*/
@QtUninvokable
public final int item(){
return item_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int item_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected ForeachInfo(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See QScxmlExecutableContent::ForeachInfo:: ForeachInfo(QScxmlExecutableContent::ForeachInfo)
*/
@QtUninvokable
@Override
public ForeachInfo clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native ForeachInfo clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #array()} instead.
*/
@QtUninvokable
public final int getArray() {
return array();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #context()} instead.
*/
@QtUninvokable
public final int getContext() {
return context();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #index()} instead.
*/
@QtUninvokable
public final int getIndex() {
return index();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #item()} instead.
*/
@QtUninvokable
public final int getItem() {
return item();
}
}
/**
* Represents a parameter to a service invocation
* Java wrapper for Qt class QScxmlExecutableContent::ParameterInfo
*/
public static class ParameterInfo extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QScxmlExecutableContent::ParameterInfo:: ParameterInfo()
*/
public ParameterInfo(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(ParameterInfo instance);
/**
* See QScxmlExecutableContent::ParameterInfo:: ParameterInfo(QScxmlExecutableContent::ParameterInfo)
* @param other
*/
public ParameterInfo(io.qt.scxml.QScxmlExecutableContent.@NonNull ParameterInfo other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(ParameterInfo instance, io.qt.scxml.QScxmlExecutableContent.ParameterInfo other);
/**
* See QScxmlExecutableContent::ParameterInfo:: ParameterInfo{qint32, qint32, qint32}
* @param name
* @param expr
* @param location
*/
public ParameterInfo(int name, int expr, int location){
super((QPrivateConstructor)null);
initialize_native(this, name, expr, location);
}
private native static void initialize_native(ParameterInfo instance, int name, int expr, int location);
/**
* Expression to be evaluated
* See QScxmlExecutableContent::ParameterInfo:: expr
* @param expr
*/
@QtUninvokable
public final void setExpr(int expr){
setExpr_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), expr);
}
@QtUninvokable
private native void setExpr_native_qint32(long __this__nativeId, int expr);
/**
* Expression to be evaluated
* See QScxmlExecutableContent::ParameterInfo:: expr
* @return
*/
@QtUninvokable
public final int expr(){
return expr_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int expr_native(long __this__nativeId);
/**
* Data model name of the item to be passed as a parameter
* See QScxmlExecutableContent::ParameterInfo:: location
* @param location
*/
@QtUninvokable
public final void setLocation(int location){
setLocation_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), location);
}
@QtUninvokable
private native void setLocation_native_qint32(long __this__nativeId, int location);
/**
* Data model name of the item to be passed as a parameter
* See QScxmlExecutableContent::ParameterInfo:: location
* @return
*/
@QtUninvokable
public final int location(){
return location_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int location_native(long __this__nativeId);
/**
* Of the parameter
* See QScxmlExecutableContent::ParameterInfo:: name
* @param name
*/
@QtUninvokable
public final void setName(int name){
setName_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), name);
}
@QtUninvokable
private native void setName_native_qint32(long __this__nativeId, int name);
/**
* Of the parameter
* See QScxmlExecutableContent::ParameterInfo:: name
* @return
*/
@QtUninvokable
public final int name(){
return name_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int name_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected ParameterInfo(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See QScxmlExecutableContent::ParameterInfo:: ParameterInfo(QScxmlExecutableContent::ParameterInfo)
*/
@QtUninvokable
@Override
public ParameterInfo clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native ParameterInfo clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #expr()} instead.
*/
@QtUninvokable
public final int getExpr() {
return expr();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #location()} instead.
*/
@QtUninvokable
public final int getLocation() {
return location();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #name()} instead.
*/
@QtUninvokable
public final int getName() {
return name();
}
}
/**
* Represents a service invocation
* Java wrapper for Qt class QScxmlExecutableContent::InvokeInfo
*/
public static class InvokeInfo extends QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QScxmlExecutableContent::InvokeInfo:: InvokeInfo()
*/
public InvokeInfo(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(InvokeInfo instance);
/**
* See QScxmlExecutableContent::InvokeInfo:: InvokeInfo(QScxmlExecutableContent::InvokeInfo)
* @param other
*/
public InvokeInfo(io.qt.scxml.QScxmlExecutableContent.@NonNull InvokeInfo other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(InvokeInfo instance, io.qt.scxml.QScxmlExecutableContent.InvokeInfo other);
/**
* See QScxmlExecutableContent::InvokeInfo:: InvokeInfo{qint32, qint32, qint32, qint32, qint32, qint32, bool}
* @param id
* @param prefix
* @param location
* @param context
* @param expr
* @param finalize
* @param autoforward
*/
public InvokeInfo(int id, int prefix, int location, int context, int expr, int finalize, boolean autoforward){
super((QPrivateConstructor)null);
initialize_native(this, id, prefix, location, context, expr, finalize, autoforward);
}
private native static void initialize_native(InvokeInfo instance, int id, int prefix, int location, int context, int expr, int finalize, boolean autoforward);
/**
* Whether events should automatically be forwarded to the invoked service
* See QScxmlExecutableContent::InvokeInfo:: autoforward
* @param autoforward
*/
@QtUninvokable
public final void setAutoforward(boolean autoforward){
setAutoforward_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), autoforward);
}
@QtUninvokable
private native void setAutoforward_native_bool(long __this__nativeId, boolean autoforward);
/**
* Whether events should automatically be forwarded to the invoked service
* See QScxmlExecutableContent::InvokeInfo:: autoforward
* @return
*/
@QtUninvokable
public final boolean autoforward(){
return autoforward_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean autoforward_native(long __this__nativeId);
/**
* To interpret the location in
* See QScxmlExecutableContent::InvokeInfo:: context
* @param context
*/
@QtUninvokable
public final void setContext(int context){
setContext_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), context);
}
@QtUninvokable
private native void setContext_native_qint32(long __this__nativeId, int context);
/**
* To interpret the location in
* See QScxmlExecutableContent::InvokeInfo:: context
* @return
*/
@QtUninvokable
public final int context(){
return context_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int context_native(long __this__nativeId);
/**
* Expression representing the srcexpr of the invoke element
* See QScxmlExecutableContent::InvokeInfo:: expr
* @param expr
*/
@QtUninvokable
public final void setExpr(int expr){
setExpr_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), expr);
}
@QtUninvokable
private native void setExpr_native_qint32(long __this__nativeId, int expr);
/**
* Expression representing the srcexpr of the invoke element
* See QScxmlExecutableContent::InvokeInfo:: expr
* @return
*/
@QtUninvokable
public final int expr(){
return expr_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int expr_native(long __this__nativeId);
/**
* ID of the container of executable content to be run on finalizing the invocation
* See QScxmlExecutableContent::InvokeInfo:: finalize
* @param finalizeId
*/
@QtUninvokable
public final void setFinalizeId(int finalizeId){
setFinalizeId_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), finalizeId);
}
@QtUninvokable
private native void setFinalizeId_native_qint32(long __this__nativeId, int finalizeId);
/**
* ID of the container of executable content to be run on finalizing the invocation
* See QScxmlExecutableContent::InvokeInfo:: finalize
* @return
*/
@QtUninvokable
public final int finalizeId(){
return finalizeId_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int finalizeId_native(long __this__nativeId);
/**
* ID specified by the id attribute in the <invoke> element
* See QScxmlExecutableContent::InvokeInfo:: id
* @param id
*/
@QtUninvokable
public final void setId(int id){
setId_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), id);
}
@QtUninvokable
private native void setId_native_qint32(long __this__nativeId, int id);
/**
* ID specified by the id attribute in the <invoke> element
* See QScxmlExecutableContent::InvokeInfo:: id
* @return
*/
@QtUninvokable
public final int id(){
return id_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int id_native(long __this__nativeId);
/**
* Data model location to write the invocation ID to
* See QScxmlExecutableContent::InvokeInfo:: location
* @param location
*/
@QtUninvokable
public final void setLocation(int location){
setLocation_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), location);
}
@QtUninvokable
private native void setLocation_native_qint32(long __this__nativeId, int location);
/**
* Data model location to write the invocation ID to
* See QScxmlExecutableContent::InvokeInfo:: location
* @return
*/
@QtUninvokable
public final int location(){
return location_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int location_native(long __this__nativeId);
/**
* Unique prefix for this invocation in the context of the state from which it is called
* See QScxmlExecutableContent::InvokeInfo:: prefix
* @param prefix
*/
@QtUninvokable
public final void setPrefix(int prefix){
setPrefix_native_qint32(QtJambi_LibraryUtilities.internal.nativeId(this), prefix);
}
@QtUninvokable
private native void setPrefix_native_qint32(long __this__nativeId, int prefix);
/**
* Unique prefix for this invocation in the context of the state from which it is called
* See QScxmlExecutableContent::InvokeInfo:: prefix
* @return
*/
@QtUninvokable
public final int prefix(){
return prefix_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int prefix_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected InvokeInfo(QPrivateConstructor p) { super(p); }
/**
* Creates and returns a copy of this object.
See QScxmlExecutableContent::InvokeInfo:: InvokeInfo(QScxmlExecutableContent::InvokeInfo)
*/
@QtUninvokable
@Override
public InvokeInfo clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private static native InvokeInfo clone_native(long __this_nativeId);
/**
* @hidden
* Kotlin property getter. In Java use {@link #autoforward()} instead.
*/
@QtUninvokable
public final boolean getAutoforward() {
return autoforward();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #context()} instead.
*/
@QtUninvokable
public final int getContext() {
return context();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #expr()} instead.
*/
@QtUninvokable
public final int getExpr() {
return expr();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #finalizeId()} instead.
*/
@QtUninvokable
public final int getFinalizeId() {
return finalizeId();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #id()} instead.
*/
@QtUninvokable
public final int getId() {
return id();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #location()} instead.
*/
@QtUninvokable
public final int getLocation() {
return location();
}
/**
* @hidden
* Kotlin property getter. In Java use {@link #prefix()} instead.
*/
@QtUninvokable
public final int getPrefix() {
return prefix();
}
}
public final static int NoContainer = -1;
public final static int NoString = -1;
public final static int NoInstruction = -1;
public final static int NoEvaluator = -1;
}