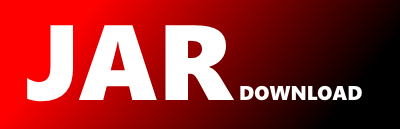
io.qt.uic.ui4.DomCustomWidget Maven / Gradle / Ivy
Show all versions of qtjambi-uic Show documentation
package io.qt.uic.ui4;
import io.qt.*;
/**
* Java wrapper for Qt class DomCustomWidget
* @hidden
*/
public class DomCustomWidget extends QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See DomCustomWidget:: DomCustomWidget()
*/
public DomCustomWidget(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(DomCustomWidget instance);
/**
* See DomCustomWidget:: clearElementAddPageMethod()
*/
@QtUninvokable
public final void clearElementAddPageMethod(){
clearElementAddPageMethod_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementAddPageMethod_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementClass()
*/
@QtUninvokable
public final void clearElementClass(){
clearElementClass_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementClass_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementContainer()
*/
@QtUninvokable
public final void clearElementContainer(){
clearElementContainer_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementContainer_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementExtends()
*/
@QtUninvokable
public final void clearElementExtends(){
clearElementExtends_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementExtends_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementHeader()
*/
@QtUninvokable
public final void clearElementHeader(){
clearElementHeader_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementHeader_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementPixmap()
*/
@QtUninvokable
public final void clearElementPixmap(){
clearElementPixmap_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementPixmap_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementPropertyspecifications()
*/
@QtUninvokable
public final void clearElementPropertyspecifications(){
clearElementPropertyspecifications_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementPropertyspecifications_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementSizeHint()
*/
@QtUninvokable
public final void clearElementSizeHint(){
clearElementSizeHint_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementSizeHint_native(long __this__nativeId);
/**
* See DomCustomWidget:: clearElementSlots()
*/
@QtUninvokable
public final void clearElementSlots(){
clearElementSlots_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void clearElementSlots_native(long __this__nativeId);
/**
* See DomCustomWidget:: elementAddPageMethod()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String elementAddPageMethod(){
return elementAddPageMethod_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String elementAddPageMethod_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementClass()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String elementClass(){
return elementClass_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String elementClass_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementContainer()const
* @return
*/
@QtUninvokable
public final int elementContainer(){
return elementContainer_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native int elementContainer_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementExtends()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String elementExtends(){
return elementExtends_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String elementExtends_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementHeader()const
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomHeader elementHeader(){
return elementHeader_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomHeader elementHeader_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementPixmap()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String elementPixmap(){
return elementPixmap_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String elementPixmap_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementPropertyspecifications()const
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomPropertySpecifications elementPropertyspecifications(){
return elementPropertyspecifications_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomPropertySpecifications elementPropertyspecifications_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementSizeHint()const
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomSize elementSizeHint(){
return elementSizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomSize elementSizeHint_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: elementSlots()const
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomSlots elementSlots(){
return elementSlots_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomSlots elementSlots_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementAddPageMethod()const
* @return
*/
@QtUninvokable
public final boolean hasElementAddPageMethod(){
return hasElementAddPageMethod_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementAddPageMethod_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementClass()const
* @return
*/
@QtUninvokable
public final boolean hasElementClass(){
return hasElementClass_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementClass_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementContainer()const
* @return
*/
@QtUninvokable
public final boolean hasElementContainer(){
return hasElementContainer_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementContainer_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementExtends()const
* @return
*/
@QtUninvokable
public final boolean hasElementExtends(){
return hasElementExtends_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementExtends_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementHeader()const
* @return
*/
@QtUninvokable
public final boolean hasElementHeader(){
return hasElementHeader_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementHeader_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementPixmap()const
* @return
*/
@QtUninvokable
public final boolean hasElementPixmap(){
return hasElementPixmap_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementPixmap_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementPropertyspecifications()const
* @return
*/
@QtUninvokable
public final boolean hasElementPropertyspecifications(){
return hasElementPropertyspecifications_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementPropertyspecifications_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementSizeHint()const
* @return
*/
@QtUninvokable
public final boolean hasElementSizeHint(){
return hasElementSizeHint_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementSizeHint_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: hasElementSlots()const
* @return
*/
@QtUninvokable
public final boolean hasElementSlots(){
return hasElementSlots_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean hasElementSlots_native_constfct(long __this__nativeId);
/**
* See DomCustomWidget:: read(QXmlStreamReader&)
* @param reader
*/
@QtUninvokable
public final void read(io.qt.core.@StrictNonNull QXmlStreamReader reader){
java.util.Objects.requireNonNull(reader, "Argument 'reader': null not expected.");
read_native_ref_QXmlStreamReader(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(reader));
}
@QtUninvokable
private native void read_native_ref_QXmlStreamReader(long __this__nativeId, long reader);
/**
* See DomCustomWidget:: setElementAddPageMethod(QString)
* @param a
*/
@QtUninvokable
public final void setElementAddPageMethod(java.lang.@NonNull String a){
setElementAddPageMethod_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), a);
}
@QtUninvokable
private native void setElementAddPageMethod_native_cref_QString(long __this__nativeId, java.lang.String a);
/**
* See DomCustomWidget:: setElementClass(QString)
* @param a
*/
@QtUninvokable
public final void setElementClass(java.lang.@NonNull String a){
setElementClass_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), a);
}
@QtUninvokable
private native void setElementClass_native_cref_QString(long __this__nativeId, java.lang.String a);
/**
* See DomCustomWidget:: setElementContainer(int)
* @param a
*/
@QtUninvokable
public final void setElementContainer(int a){
setElementContainer_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), a);
}
@QtUninvokable
private native void setElementContainer_native_int(long __this__nativeId, int a);
/**
* See DomCustomWidget:: setElementExtends(QString)
* @param a
*/
@QtUninvokable
public final void setElementExtends(java.lang.@NonNull String a){
setElementExtends_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), a);
}
@QtUninvokable
private native void setElementExtends_native_cref_QString(long __this__nativeId, java.lang.String a);
/**
* See DomCustomWidget:: setElementHeader(DomHeader*)
* @param a
*/
@QtUninvokable
public final void setElementHeader(io.qt.uic.ui4.@Nullable DomHeader a){
setElementHeader_native_DomHeader_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(a));
}
@QtUninvokable
private native void setElementHeader_native_DomHeader_ptr(long __this__nativeId, long a);
/**
* See DomCustomWidget:: setElementPixmap(QString)
* @param a
*/
@QtUninvokable
public final void setElementPixmap(java.lang.@NonNull String a){
setElementPixmap_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), a);
}
@QtUninvokable
private native void setElementPixmap_native_cref_QString(long __this__nativeId, java.lang.String a);
/**
* See DomCustomWidget:: setElementPropertyspecifications(DomPropertySpecifications*)
* @param a
*/
@QtUninvokable
public final void setElementPropertyspecifications(io.qt.uic.ui4.@Nullable DomPropertySpecifications a){
setElementPropertyspecifications_native_DomPropertySpecifications_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(a));
}
@QtUninvokable
private native void setElementPropertyspecifications_native_DomPropertySpecifications_ptr(long __this__nativeId, long a);
/**
* See DomCustomWidget:: setElementSizeHint(DomSize*)
* @param a
*/
@QtUninvokable
public final void setElementSizeHint(io.qt.uic.ui4.@Nullable DomSize a){
setElementSizeHint_native_DomSize_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(a));
}
@QtUninvokable
private native void setElementSizeHint_native_DomSize_ptr(long __this__nativeId, long a);
/**
* See DomCustomWidget:: setElementSlots(DomSlots*)
* @param a
*/
@QtUninvokable
public final void setElementSlots(io.qt.uic.ui4.@Nullable DomSlots a){
setElementSlots_native_DomSlots_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(a));
}
@QtUninvokable
private native void setElementSlots_native_DomSlots_ptr(long __this__nativeId, long a);
/**
* See DomCustomWidget:: takeElementHeader()
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomHeader takeElementHeader(){
return takeElementHeader_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomHeader takeElementHeader_native(long __this__nativeId);
/**
* See DomCustomWidget:: takeElementPropertyspecifications()
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomPropertySpecifications takeElementPropertyspecifications(){
return takeElementPropertyspecifications_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomPropertySpecifications takeElementPropertyspecifications_native(long __this__nativeId);
/**
* See DomCustomWidget:: takeElementSizeHint()
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomSize takeElementSizeHint(){
return takeElementSizeHint_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomSize takeElementSizeHint_native(long __this__nativeId);
/**
* See DomCustomWidget:: takeElementSlots()
* @return
*/
@QtUninvokable
public final io.qt.uic.ui4.@Nullable DomSlots takeElementSlots(){
return takeElementSlots_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.uic.ui4.DomSlots takeElementSlots_native(long __this__nativeId);
/**
* See DomCustomWidget:: write(QXmlStreamWriter&, QString)const
* @param writer
* @param tagName
*/
@QtUninvokable
public final void write(io.qt.core.@StrictNonNull QXmlStreamWriter writer, java.lang.@NonNull String tagName){
java.util.Objects.requireNonNull(writer, "Argument 'writer': null not expected.");
write_native_ref_QXmlStreamWriter_cref_QString_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(writer), tagName);
}
@QtUninvokable
private native void write_native_ref_QXmlStreamWriter_cref_QString_constfct(long __this__nativeId, long writer, java.lang.String tagName);
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected DomCustomWidget(QPrivateConstructor p) { super(p); }
/**
* Overloaded function for {@link #write(io.qt.core.QXmlStreamWriter, java.lang.String)}
* with tagName = (String)null
.
*/
@QtUninvokable
public final void write(io.qt.core.@StrictNonNull QXmlStreamWriter writer) {
write(writer, (String)null);
}
}