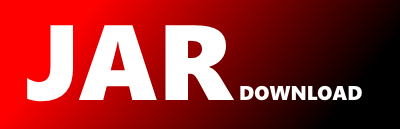
io.qt.websockets.QWebSocket Maven / Gradle / Ivy
package io.qt.websockets;
/**
* Implements a TCP socket that talks the WebSocket protocol
* Java wrapper for Qt class QWebSocket
*/
public class QWebSocket extends io.qt.core.QObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
@io.qt.QtPropertyMember(enabled=false)
private Object __rcMaskGenerator = null;
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QWebSocket.class);
/**
* See QWebSocket::aboutToClose()
*/
public final Signal0 aboutToClose = new Signal0();
/**
* See QWebSocket::binaryFrameReceived(QByteArray,bool)
*/
public final Signal2 binaryFrameReceived = new Signal2<>();
/**
* See QWebSocket::binaryMessageReceived(QByteArray)
*/
public final Signal1 binaryMessageReceived = new Signal1<>();
/**
* See QWebSocket::bytesWritten(qint64)
*/
public final Signal1<@io.qt.QtPrimitiveType Long> bytesWritten = new Signal1<>();
/**
* Emitted when a connection is successfully established. A connection is successfully established when the socket is connected and the handshake was successful
*
*/
public final Signal0 connected = new Signal0();
/**
* Emitted when the socket is disconnected
* See QWebSocket::disconnected()
*/
public final Signal0 disconnected = new Signal0();
/**
* See QWebSocket::error(QAbstractSocket::SocketError)
*/
@SuppressWarnings({"exports"})
public final Signal1 error = new Signal1<>();
/**
* See QWebSocket::pong(quint64,QByteArray)
*/
public final Signal2<@io.qt.QtPrimitiveType Long, io.qt.core.QByteArray> pong = new Signal2<>();
/**
* See QWebSocket::preSharedKeyAuthenticationRequired(QSslPreSharedKeyAuthenticator*)
*/
@SuppressWarnings({"exports"})
public final Signal1 preSharedKeyAuthenticationRequired = new Signal1<>();
/**
* See QWebSocket::proxyAuthenticationRequired(QNetworkProxy,QAuthenticator*)
*/
@SuppressWarnings({"exports"})
public final Signal2 proxyAuthenticationRequired = new Signal2<>();
/**
* See QWebSocket::readChannelFinished()
*/
public final Signal0 readChannelFinished = new Signal0();
/**
* See QWebSocket::sslErrors(QList<QSslError>)
*/
@SuppressWarnings({"exports"})
public final Signal1> sslErrors = new Signal1<>();
/**
* See QWebSocket::stateChanged(QAbstractSocket::SocketState)
*/
@SuppressWarnings({"exports"})
public final Signal1 stateChanged = new Signal1<>();
/**
* See QWebSocket::textFrameReceived(QString,bool)
*/
public final Signal2 textFrameReceived = new Signal2<>();
/**
* See QWebSocket::textMessageReceived(QString)
*/
public final Signal1 textMessageReceived = new Signal1<>();
/**
* Overloaded constructor for {@link #QWebSocket(java.lang.String, io.qt.websockets.QWebSocketProtocol.Version, io.qt.core.QObject)}
* with parent = null
.
*/
public QWebSocket(java.lang.String origin, io.qt.websockets.QWebSocketProtocol.Version version) {
this(origin, version, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QWebSocket(java.lang.String, io.qt.websockets.QWebSocketProtocol.Version, io.qt.core.QObject)}
* with:
* version = io.qt.websockets.QWebSocketProtocol.Version.VersionLatest
* parent = null
*
*/
public QWebSocket(java.lang.String origin) {
this(origin, io.qt.websockets.QWebSocketProtocol.Version.VersionLatest, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QWebSocket(java.lang.String, io.qt.websockets.QWebSocketProtocol.Version, io.qt.core.QObject)}
* with:
* origin = ""
* version = io.qt.websockets.QWebSocketProtocol.Version.VersionLatest
* parent = null
*
*/
public QWebSocket() {
this("", io.qt.websockets.QWebSocketProtocol.Version.VersionLatest, (io.qt.core.QObject)null);
}
/**
* Creates a new QWebSocket with the given origin, the version of the protocol to use and parent
* See QWebSocket::QWebSocket(QString,QWebSocketProtocol::Version,QObject*)
*/
public QWebSocket(java.lang.String origin, io.qt.websockets.QWebSocketProtocol.Version version, io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, origin, version, parent);
}
private native static void initialize_native(QWebSocket instance, java.lang.String origin, io.qt.websockets.QWebSocketProtocol.Version version, io.qt.core.QObject parent);
/**
* Aborts the current socket and resets the socket. Unlike close(), this function immediately closes the socket, discarding any pending data in the write buffer
*
*/
@io.qt.QtUninvokable
public final void abort(){
abort_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void abort_native(long __this__nativeId);
/**
* See QWebSocket::bytesToWrite()const
*/
@io.qt.QtUninvokable
public final long bytesToWrite(){
return bytesToWrite_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long bytesToWrite_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #close(io.qt.websockets.QWebSocketProtocol.CloseCode, java.lang.String)}
* with reason = ""
.
*/
public final void close(io.qt.websockets.QWebSocketProtocol.CloseCode closeCode) {
close(closeCode, "");
}
/**
* Overloaded function for {@link #close(io.qt.websockets.QWebSocketProtocol.CloseCode, java.lang.String)}
* with:
* closeCode = io.qt.websockets.QWebSocketProtocol.CloseCode.CloseCodeNormal
* reason = ""
*
*/
public final void close() {
close(io.qt.websockets.QWebSocketProtocol.CloseCode.CloseCodeNormal, "");
}
/**
* Gracefully closes the socket with the given closeCode and reason
* See QWebSocket::close(QWebSocketProtocol::CloseCode,QString)
*/
public final void close(io.qt.websockets.QWebSocketProtocol.CloseCode closeCode, java.lang.String reason){
close_native_QWebSocketProtocol_CloseCode_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), closeCode.value(), reason);
}
private native void close_native_QWebSocketProtocol_CloseCode_cref_QString(long __this__nativeId, int closeCode, java.lang.String reason);
/**
* Returns the code indicating why the socket was closed
* See QWebSocket::closeCode()const
*/
@io.qt.QtUninvokable
public final io.qt.websockets.QWebSocketProtocol.CloseCode closeCode(){
return io.qt.websockets.QWebSocketProtocol.CloseCode.resolve(closeCode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int closeCode_native_constfct(long __this__nativeId);
/**
* Returns the reason why the socket was closed
* See QWebSocket::closeReason()const
*/
@io.qt.QtUninvokable
public final java.lang.String closeReason(){
return closeReason_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String closeReason_native_constfct(long __this__nativeId);
/**
*
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QAbstractSocket.SocketError error(){
return io.qt.network.QAbstractSocket.SocketError.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QWebSocket::errorString()const
*/
@io.qt.QtUninvokable
public final java.lang.String errorString(){
return errorString_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String errorString_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final boolean flush(){
return flush_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean flush_native(long __this__nativeId);
/**
* See QWebSocket::ignoreSslErrors()
*/
public final void ignoreSslErrors(){
ignoreSslErrors_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void ignoreSslErrors_native(long __this__nativeId);
/**
* See QWebSocket::ignoreSslErrors(QList<QSslError>)
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final void ignoreSslErrors(java.util.Collection errors){
ignoreSslErrors_native_cref_QList(QtJambi_LibraryUtilities.internal.nativeId(this), errors);
}
@io.qt.QtUninvokable
private native void ignoreSslErrors_native_cref_QList(long __this__nativeId, java.util.Collection errors);
/**
* See QWebSocket::isValid()const
*/
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QWebSocket::localAddress()const
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QHostAddress localAddress(){
return localAddress_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.network.QHostAddress localAddress_native_constfct(long __this__nativeId);
/**
* See QWebSocket::localPort()const
*/
@io.qt.QtUninvokable
public final short localPort(){
return localPort_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native short localPort_native_constfct(long __this__nativeId);
/**
* See QWebSocket::maskGenerator()const
*/
@io.qt.QtUninvokable
public final io.qt.websockets.QMaskGenerator maskGenerator(){
return maskGenerator_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.websockets.QMaskGenerator maskGenerator_native_constfct(long __this__nativeId);
/**
* See QWebSocket::maxAllowedIncomingFrameSize()const
*/
@io.qt.QtUninvokable
public final long maxAllowedIncomingFrameSize(){
return maxAllowedIncomingFrameSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long maxAllowedIncomingFrameSize_native_constfct(long __this__nativeId);
/**
* See QWebSocket::maxAllowedIncomingMessageSize()const
*/
@io.qt.QtUninvokable
public final long maxAllowedIncomingMessageSize(){
return maxAllowedIncomingMessageSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long maxAllowedIncomingMessageSize_native_constfct(long __this__nativeId);
/**
* Opens a WebSocket connection using the given request
* See QWebSocket::open(QNetworkRequest)
*/
@SuppressWarnings({"exports"})
public final void open(io.qt.network.QNetworkRequest request){
open_native_cref_QNetworkRequest(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(request));
}
private native void open_native_cref_QNetworkRequest(long __this__nativeId, long request);
/**
* Opens a WebSocket connection using the given url
*
*/
public final void open(io.qt.core.QUrl url){
open_native_cref_QUrl(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(url));
}
private native void open_native_cref_QUrl(long __this__nativeId, long url);
/**
* Returns the current origin
*
*/
@io.qt.QtUninvokable
public final java.lang.String origin(){
return origin_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String origin_native_constfct(long __this__nativeId);
/**
* See QWebSocket::outgoingFrameSize()const
*/
@io.qt.QtUninvokable
public final long outgoingFrameSize(){
return outgoingFrameSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long outgoingFrameSize_native_constfct(long __this__nativeId);
/**
* See QWebSocket::pauseMode()const
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QAbstractSocket.PauseModes pauseMode(){
return new io.qt.network.QAbstractSocket.PauseModes(pauseMode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int pauseMode_native_constfct(long __this__nativeId);
/**
* See QWebSocket::peerAddress()const
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QHostAddress peerAddress(){
return peerAddress_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.network.QHostAddress peerAddress_native_constfct(long __this__nativeId);
/**
* See QWebSocket::peerName()const
*/
@io.qt.QtUninvokable
public final java.lang.String peerName(){
return peerName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String peerName_native_constfct(long __this__nativeId);
/**
* See QWebSocket::peerPort()const
*/
@io.qt.QtUninvokable
public final short peerPort(){
return peerPort_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native short peerPort_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #ping(io.qt.core.QByteArray)}
* with payload = new io.qt.core.QByteArray()
.
*/
public final void ping() {
ping(new io.qt.core.QByteArray());
}
/**
* Pings the server to indicate that the connection is still alive. Additional payload can be sent along the ping message
* See QWebSocket::ping(QByteArray)
*/
public final void ping(io.qt.core.QByteArray payload){
ping_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(payload));
}
private native void ping_native_cref_QByteArray(long __this__nativeId, long payload);
/**
*
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QNetworkProxy proxy(){
return proxy_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.network.QNetworkProxy proxy_native_constfct(long __this__nativeId);
/**
* See QWebSocket::readBufferSize()const
*/
@io.qt.QtUninvokable
public final long readBufferSize(){
return readBufferSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long readBufferSize_native_constfct(long __this__nativeId);
/**
* Returns the request that was or will be used to open this socket
* See QWebSocket::request()const
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QNetworkRequest request(){
return request_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.network.QNetworkRequest request_native_constfct(long __this__nativeId);
/**
* Returns the url the socket is connected to or will connect to
* See QWebSocket::requestUrl()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QUrl requestUrl(){
return requestUrl_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QUrl requestUrl_native_constfct(long __this__nativeId);
/**
* Returns the name of the resource currently accessed
* See QWebSocket::resourceName()const
*/
@io.qt.QtUninvokable
public final java.lang.String resourceName(){
return resourceName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String resourceName_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final void resume(){
resume_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void resume_native(long __this__nativeId);
/**
* Sends the given data over the socket as a binary message and returns the number of bytes actually sent
* See QWebSocket::sendBinaryMessage(QByteArray)
*/
@io.qt.QtUninvokable
public final long sendBinaryMessage(io.qt.core.QByteArray data){
return sendBinaryMessage_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(data));
}
@io.qt.QtUninvokable
private native long sendBinaryMessage_native_cref_QByteArray(long __this__nativeId, long data);
/**
* Sends the given message over the socket as a text message and returns the number of bytes actually sent
* See QWebSocket::sendTextMessage(QString)
*/
@io.qt.QtUninvokable
public final long sendTextMessage(java.lang.String message){
return sendTextMessage_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), message);
}
@io.qt.QtUninvokable
private native long sendTextMessage_native_cref_QString(long __this__nativeId, java.lang.String message);
/**
* See QWebSocket::setMaskGenerator(const QMaskGenerator*)
*/
@io.qt.QtUninvokable
public final void setMaskGenerator(io.qt.websockets.QMaskGenerator maskGenerator){
setMaskGenerator_native_const_QMaskGenerator_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(maskGenerator));
__rcMaskGenerator = maskGenerator;
}
@io.qt.QtUninvokable
private native void setMaskGenerator_native_const_QMaskGenerator_ptr(long __this__nativeId, long maskGenerator);
/**
* See QWebSocket::setMaxAllowedIncomingFrameSize(quint64)
*/
@io.qt.QtUninvokable
public final void setMaxAllowedIncomingFrameSize(long maxAllowedIncomingFrameSize){
setMaxAllowedIncomingFrameSize_native_unsigned_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), maxAllowedIncomingFrameSize);
}
@io.qt.QtUninvokable
private native void setMaxAllowedIncomingFrameSize_native_unsigned_long_long(long __this__nativeId, long maxAllowedIncomingFrameSize);
/**
* See QWebSocket::setMaxAllowedIncomingMessageSize(quint64)
*/
@io.qt.QtUninvokable
public final void setMaxAllowedIncomingMessageSize(long maxAllowedIncomingMessageSize){
setMaxAllowedIncomingMessageSize_native_unsigned_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), maxAllowedIncomingMessageSize);
}
@io.qt.QtUninvokable
private native void setMaxAllowedIncomingMessageSize_native_unsigned_long_long(long __this__nativeId, long maxAllowedIncomingMessageSize);
/**
* See QWebSocket::setOutgoingFrameSize(quint64)
*/
@io.qt.QtUninvokable
public final void setOutgoingFrameSize(long outgoingFrameSize){
setOutgoingFrameSize_native_unsigned_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), outgoingFrameSize);
}
@io.qt.QtUninvokable
private native void setOutgoingFrameSize_native_unsigned_long_long(long __this__nativeId, long outgoingFrameSize);
/**
* Overloaded function for {@link #setPauseMode(io.qt.network.QAbstractSocket.PauseModes)}.
*/
@io.qt.QtUninvokable
public final void setPauseMode(io.qt.network.QAbstractSocket.PauseMode ... pauseMode){
setPauseMode(new io.qt.network.QAbstractSocket.PauseModes(pauseMode));
}
/**
* See QWebSocket::setPauseMode(QAbstractSocket::PauseModes)
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final void setPauseMode(io.qt.network.QAbstractSocket.PauseModes pauseMode){
setPauseMode_native_QFlags_QAbstractSocket_PauseMode_(QtJambi_LibraryUtilities.internal.nativeId(this), pauseMode.value());
}
@io.qt.QtUninvokable
private native void setPauseMode_native_QFlags_QAbstractSocket_PauseMode_(long __this__nativeId, int pauseMode);
/**
* See QWebSocket::setProxy(QNetworkProxy)
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final void setProxy(io.qt.network.QNetworkProxy networkProxy){
setProxy_native_cref_QNetworkProxy(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(networkProxy));
}
@io.qt.QtUninvokable
private native void setProxy_native_cref_QNetworkProxy(long __this__nativeId, long networkProxy);
/**
* See QWebSocket::setReadBufferSize(qint64)
*/
@io.qt.QtUninvokable
public final void setReadBufferSize(long size){
setReadBufferSize_native_long_long(QtJambi_LibraryUtilities.internal.nativeId(this), size);
}
@io.qt.QtUninvokable
private native void setReadBufferSize_native_long_long(long __this__nativeId, long size);
/**
* See QWebSocket::setSslConfiguration(QSslConfiguration)
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final void setSslConfiguration(io.qt.network.QSslConfiguration sslConfiguration){
setSslConfiguration_native_cref_QSslConfiguration(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(sslConfiguration));
}
@io.qt.QtUninvokable
private native void setSslConfiguration_native_cref_QSslConfiguration(long __this__nativeId, long sslConfiguration);
/**
* See QWebSocket::sslConfiguration()const
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QSslConfiguration sslConfiguration(){
return sslConfiguration_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.network.QSslConfiguration sslConfiguration_native_constfct(long __this__nativeId);
/**
* Returns the current state of the socket
*
*/
@SuppressWarnings({"exports"})
@io.qt.QtUninvokable
public final io.qt.network.QAbstractSocket.SocketState state(){
return io.qt.network.QAbstractSocket.SocketState.resolve(state_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int state_native_constfct(long __this__nativeId);
/**
* Returns the version the socket is currently using
* See QWebSocket::version()const
*/
@io.qt.QtUninvokable
public final io.qt.websockets.QWebSocketProtocol.Version version(){
return io.qt.websockets.QWebSocketProtocol.Version.resolve(version_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int version_native_constfct(long __this__nativeId);
/**
* See QWebSocket::maxIncomingFrameSize()
*/
public native static long maxIncomingFrameSize();
/**
* See QWebSocket::maxIncomingMessageSize()
*/
public native static long maxIncomingMessageSize();
/**
* See QWebSocket::maxOutgoingFrameSize()
*/
public native static long maxOutgoingFrameSize();
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QWebSocket(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QWebSocket(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QWebSocket instance, QDeclarativeConstructor constructor);
}