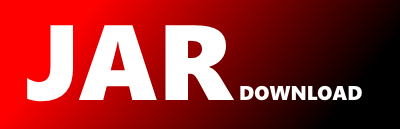
io.qt.websockets.QWebSocketProtocol Maven / Gradle / Ivy
package io.qt.websockets;
/**
* Contains constants related to the WebSocket standard
* Java wrapper for Qt class QWebSocketProtocol
*/
public final class QWebSocketProtocol
{
static {
QtJambi_LibraryUtilities.initialize();
}
private QWebSocketProtocol() throws java.lang.InstantiationError { throw new java.lang.InstantiationError("Cannot instantiate namespace QWebSocketProtocol."); }
/**
* Java wrapper for Qt enum QWebSocketProtocol::CloseCode
*/
public enum CloseCode implements io.qt.QtEnumerator {
CloseCodeNormal(1000),
CloseCodeGoingAway(1001),
CloseCodeProtocolError(1002),
CloseCodeDatatypeNotSupported(1003),
CloseCodeReserved1004(1004),
CloseCodeMissingStatusCode(1005),
CloseCodeAbnormalDisconnection(1006),
CloseCodeWrongDatatype(1007),
CloseCodePolicyViolated(1008),
CloseCodeTooMuchData(1009),
CloseCodeMissingExtension(1010),
CloseCodeBadOperation(1011),
CloseCodeTlsHandshakeFailed(1015);
private CloseCode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static CloseCode resolve(int value) {
switch (value) {
case 1000: return CloseCodeNormal;
case 1001: return CloseCodeGoingAway;
case 1002: return CloseCodeProtocolError;
case 1003: return CloseCodeDatatypeNotSupported;
case 1004: return CloseCodeReserved1004;
case 1005: return CloseCodeMissingStatusCode;
case 1006: return CloseCodeAbnormalDisconnection;
case 1007: return CloseCodeWrongDatatype;
case 1008: return CloseCodePolicyViolated;
case 1009: return CloseCodeTooMuchData;
case 1010: return CloseCodeMissingExtension;
case 1011: return CloseCodeBadOperation;
case 1015: return CloseCodeTlsHandshakeFailed;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QWebSocketProtocol::Version
*/
@io.qt.QtRejectedEntries({"VersionLatest"})
public enum Version implements io.qt.QtEnumerator {
VersionUnknown(-1),
Version0(0),
Version4(4),
Version5(5),
Version6(6),
Version7(7),
Version8(8),
Version13(13),
VersionLatest(13);
private Version(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Version resolve(int value) {
switch (value) {
case -1: return VersionUnknown;
case 0: return Version0;
case 4: return Version4;
case 5: return Version5;
case 6: return Version6;
case 7: return Version7;
case 8: return Version8;
case 13: return Version13;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy