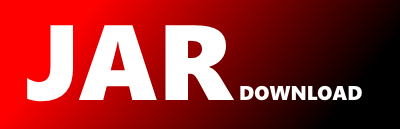
io.qt.websockets.QWebSocket Maven / Gradle / Ivy
Show all versions of qtjambi-websockets Show documentation
package io.qt.websockets;
import io.qt.*;
/**
* Implements a TCP socket that talks the WebSocket protocol
* Java wrapper for Qt class QWebSocket
*/
public class QWebSocket extends io.qt.core.QObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
@QtPropertyMember(enabled=false)
private Object __rcMaskGenerator;
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.@NonNull QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QWebSocket.class);
/**
* See QWebSocket:: aboutToClose()
*/
public final @NonNull Signal0 aboutToClose = new Signal0();
/**
* See QWebSocket:: alertReceived(QSsl::AlertLevel, QSsl::AlertType, QString)
* @since This signal was introduced in Qt 6.2.
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal3 alertReceived = new Signal3<>();
/**
* See QWebSocket:: alertSent(QSsl::AlertLevel, QSsl::AlertType, QString)
* @since This signal was introduced in Qt 6.2.
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal3 alertSent = new Signal3<>();
/**
* See QWebSocket:: authenticationRequired(QAuthenticator*)
* @since This signal was introduced in Qt 6.6.
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 authenticationRequired = new Signal1<>();
/**
* See QWebSocket:: binaryFrameReceived(QByteArray, bool)
*/
public final @NonNull Signal2 binaryFrameReceived = new Signal2<>();
/**
* See QWebSocket:: binaryMessageReceived(QByteArray)
*/
public final @NonNull Signal1 binaryMessageReceived = new Signal1<>();
/**
* See QWebSocket:: bytesWritten(qint64)
*/
public final @NonNull Signal1 bytesWritten = new Signal1<>();
/**
* Emitted when a connection is successfully established. A connection is successfully established when the socket is connected and the handshake was successful
*
*/
public final @NonNull Signal0 connected = new Signal0();
/**
* Emitted when the socket is disconnected
* See QWebSocket:: disconnected()
*/
public final @NonNull Signal0 disconnected = new Signal0();
/**
* See QWebSocket:: error(QAbstractSocket::SocketError)
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 error = new Signal1<>();
/**
* This signal is emitted after an error occurred
* See QWebSocket:: errorOccurred(QAbstractSocket::SocketError)
* @since This signal was introduced in Qt 6.5.
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 errorOccurred = new Signal1<>();
/**
* See QWebSocket:: handshakeInterruptedOnError(QSslError)
* @since This signal was introduced in Qt 6.2.
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 handshakeInterruptedOnError = new Signal1<>();
/**
* See QWebSocket:: peerVerifyError(QSslError)
* @since This signal was introduced in Qt 6.2.
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 peerVerifyError = new Signal1<>();
/**
* See QWebSocket:: pong(quint64, QByteArray)
*/
public final @NonNull Signal2 pong = new Signal2<>();
/**
* See QWebSocket:: preSharedKeyAuthenticationRequired(QSslPreSharedKeyAuthenticator*)
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 preSharedKeyAuthenticationRequired = new Signal1<>();
/**
* See QWebSocket:: proxyAuthenticationRequired(QNetworkProxy, QAuthenticator*)
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal2 proxyAuthenticationRequired = new Signal2<>();
/**
* See QWebSocket:: readChannelFinished()
*/
public final @NonNull Signal0 readChannelFinished = new Signal0();
/**
* See QWebSocket:: sslErrors(QList<QSslError>)
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1> sslErrors = new Signal1<>();
/**
* See QWebSocket:: stateChanged(QAbstractSocket::SocketState)
*/
@SuppressWarnings({"exports"})
public final @NonNull Signal1 stateChanged = new Signal1<>();
/**
* See QWebSocket:: textFrameReceived(QString, bool)
*/
public final @NonNull Signal2 textFrameReceived = new Signal2<>();
/**
* See QWebSocket:: textMessageReceived(QString)
*/
public final @NonNull Signal1 textMessageReceived = new Signal1<>();
/**
* Creates a new QWebSocket with the given origin, the version of the protocol to use and parent
* See QWebSocket:: QWebSocket(QString, QWebSocketProtocol::Version, QObject*)
* @param origin
* @param version
* @param parent
*/
public QWebSocket(java.lang.@NonNull String origin, io.qt.websockets.QWebSocketProtocol.@NonNull Version version, io.qt.core.@Nullable QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, origin, version, parent);
}
private native static void initialize_native(QWebSocket instance, java.lang.String origin, io.qt.websockets.QWebSocketProtocol.Version version, io.qt.core.QObject parent);
/**
* Aborts the current socket and resets the socket. Unlike close(), this function immediately closes the socket, discarding any pending data in the write buffer
*
*/
@QtUninvokable
public final void abort(){
abort_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void abort_native(long __this__nativeId);
/**
* See QWebSocket:: bytesToWrite()const
* @return
*/
@QtUninvokable
public final long bytesToWrite(){
return bytesToWrite_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long bytesToWrite_native_constfct(long __this__nativeId);
/**
* Gracefully closes the socket with the given closeCode and reason
* See QWebSocket:: close(QWebSocketProtocol::CloseCode, QString)
* @param closeCode
* @param reason
*/
public final void close(io.qt.websockets.QWebSocketProtocol.@NonNull CloseCode closeCode, java.lang.@NonNull String reason){
close_native_QWebSocketProtocol_CloseCode_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), closeCode.value(), reason);
}
private native void close_native_QWebSocketProtocol_CloseCode_cref_QString(long __this__nativeId, int closeCode, java.lang.String reason);
/**
* Returns the code indicating why the socket was closed
* See QWebSocket:: closeCode()const
* @return
*/
@QtUninvokable
public final io.qt.websockets.QWebSocketProtocol.@NonNull CloseCode closeCode(){
return io.qt.websockets.QWebSocketProtocol.CloseCode.resolve(closeCode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int closeCode_native_constfct(long __this__nativeId);
/**
* Returns the reason why the socket was closed
* See QWebSocket:: closeReason()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String closeReason(){
return closeReason_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String closeReason_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: continueInterruptedHandshake()
* @since This function was introduced in Qt 6.2.
*/
@QtUninvokable
public final void continueInterruptedHandshake(){
continueInterruptedHandshake_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void continueInterruptedHandshake_native(long __this__nativeId);
/**
*
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.QAbstractSocket.@NonNull SocketError error(){
return io.qt.network.QAbstractSocket.SocketError.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: errorString()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String errorString(){
return errorString_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String errorString_native_constfct(long __this__nativeId);
/**
*
* @return
*/
@QtUninvokable
public final boolean flush(){
return flush_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean flush_native(long __this__nativeId);
/**
* Returns the handshake options that were used to open this socket
* See QWebSocket:: handshakeOptions()const
* @since This function was introduced in Qt 6.4.
* @return
*/
@QtUninvokable
public final io.qt.websockets.@NonNull QWebSocketHandshakeOptions handshakeOptions(){
return handshakeOptions_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.websockets.QWebSocketHandshakeOptions handshakeOptions_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: ignoreSslErrors()
*/
public final void ignoreSslErrors(){
ignoreSslErrors_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void ignoreSslErrors_native(long __this__nativeId);
/**
* See QWebSocket:: ignoreSslErrors(QList<QSslError>)
* @param errors
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final void ignoreSslErrors(java.util.@NonNull Collection extends io.qt.network.@NonNull QSslError> errors){
ignoreSslErrors_native_cref_QList(QtJambi_LibraryUtilities.internal.nativeId(this), errors);
}
@QtUninvokable
private native void ignoreSslErrors_native_cref_QList(long __this__nativeId, java.util.Collection extends io.qt.network.QSslError> errors);
/**
* See QWebSocket:: isValid()const
* @return
*/
@QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: localAddress()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.@NonNull QHostAddress localAddress(){
return localAddress_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.network.QHostAddress localAddress_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: localPort()const
* @return
*/
@QtUninvokable
public final short localPort(){
return localPort_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native short localPort_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: maskGenerator()const
* @return
*/
@QtUninvokable
public final io.qt.websockets.@Nullable QMaskGenerator maskGenerator(){
return maskGenerator_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.websockets.QMaskGenerator maskGenerator_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: maxAllowedIncomingFrameSize()const
* @return
*/
@QtUninvokable
public final long maxAllowedIncomingFrameSize(){
return maxAllowedIncomingFrameSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long maxAllowedIncomingFrameSize_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: maxAllowedIncomingMessageSize()const
* @return
*/
@QtUninvokable
public final long maxAllowedIncomingMessageSize(){
return maxAllowedIncomingMessageSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long maxAllowedIncomingMessageSize_native_constfct(long __this__nativeId);
/**
* Opens a WebSocket connection using the given request
* See QWebSocket:: open(QNetworkRequest)
* @param request
*/
@SuppressWarnings({"exports"})
public final void open(io.qt.network.@NonNull QNetworkRequest request){
open_native_cref_QNetworkRequest(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(request));
}
private native void open_native_cref_QNetworkRequest(long __this__nativeId, long request);
/**
* Opens a WebSocket connection using the given request and options
* See QWebSocket:: open(QNetworkRequest, QWebSocketHandshakeOptions)
* @since This function was introduced in Qt 6.4.
* @param request
* @param options
*/
@SuppressWarnings({"exports"})
public final void open(io.qt.network.@NonNull QNetworkRequest request, io.qt.websockets.@NonNull QWebSocketHandshakeOptions options){
open_native_cref_QNetworkRequest_cref_QWebSocketHandshakeOptions(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(request), QtJambi_LibraryUtilities.internal.checkedNativeId(options));
}
private native void open_native_cref_QNetworkRequest_cref_QWebSocketHandshakeOptions(long __this__nativeId, long request, long options);
/**
* Opens a WebSocket connection using the given url
*
* @param url
*/
public final void open(io.qt.core.@NonNull QUrl url){
open_native_cref_QUrl(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(url));
}
private native void open_native_cref_QUrl(long __this__nativeId, long url);
/**
* Opens a WebSocket connection using the given url and options
* See QWebSocket:: open(QUrl, QWebSocketHandshakeOptions)
* @since This function was introduced in Qt 6.4.
* @param url
* @param options
*/
public final void open(io.qt.core.@NonNull QUrl url, io.qt.websockets.@NonNull QWebSocketHandshakeOptions options){
open_native_cref_QUrl_cref_QWebSocketHandshakeOptions(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(url), QtJambi_LibraryUtilities.internal.checkedNativeId(options));
}
private native void open_native_cref_QUrl_cref_QWebSocketHandshakeOptions(long __this__nativeId, long url, long options);
/**
* Returns the current origin
*
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String origin(){
return origin_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String origin_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: outgoingFrameSize()const
* @return
*/
@QtUninvokable
public final long outgoingFrameSize(){
return outgoingFrameSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long outgoingFrameSize_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: pauseMode()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.QAbstractSocket.@NonNull PauseModes pauseMode(){
return new io.qt.network.QAbstractSocket.PauseModes(pauseMode_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int pauseMode_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: peerAddress()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.@NonNull QHostAddress peerAddress(){
return peerAddress_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.network.QHostAddress peerAddress_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: peerName()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String peerName(){
return peerName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String peerName_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: peerPort()const
* @return
*/
@QtUninvokable
public final short peerPort(){
return peerPort_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native short peerPort_native_constfct(long __this__nativeId);
/**
* Pings the server to indicate that the connection is still alive. Additional payload can be sent along the ping message
* See QWebSocket:: ping(QByteArray)
* @param payload
*/
public final void ping(io.qt.core.@NonNull QByteArray payload){
ping_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(payload));
}
private native void ping_native_cref_QByteArray(long __this__nativeId, long payload);
/**
*
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.@NonNull QNetworkProxy proxy(){
return proxy_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.network.QNetworkProxy proxy_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: readBufferSize()const
* @return
*/
@QtUninvokable
public final long readBufferSize(){
return readBufferSize_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native long readBufferSize_native_constfct(long __this__nativeId);
/**
* Returns the request that was or will be used to open this socket
* See QWebSocket:: request()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.@NonNull QNetworkRequest request(){
return request_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.network.QNetworkRequest request_native_constfct(long __this__nativeId);
/**
* Returns the url the socket is connected to or will connect to
* See QWebSocket:: requestUrl()const
* @return
*/
@QtUninvokable
public final io.qt.core.@NonNull QUrl requestUrl(){
return requestUrl_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.core.QUrl requestUrl_native_constfct(long __this__nativeId);
/**
* Returns the name of the resource currently accessed
* See QWebSocket:: resourceName()const
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String resourceName(){
return resourceName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String resourceName_native_constfct(long __this__nativeId);
/**
*
*/
@QtUninvokable
public final void resume(){
resume_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native void resume_native(long __this__nativeId);
/**
* Sends the given data over the socket as a binary message and returns the number of bytes actually sent
* See QWebSocket:: sendBinaryMessage(QByteArray)
* @param data
* @return
*/
@QtUninvokable
public final long sendBinaryMessage(io.qt.core.@NonNull QByteArray data){
return sendBinaryMessage_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(data));
}
@QtUninvokable
private native long sendBinaryMessage_native_cref_QByteArray(long __this__nativeId, long data);
/**
* Sends the given message over the socket as a text message and returns the number of bytes actually sent
* See QWebSocket:: sendTextMessage(QString)
* @param message
* @return
*/
@QtUninvokable
public final long sendTextMessage(java.lang.@NonNull String message){
return sendTextMessage_native_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), message);
}
@QtUninvokable
private native long sendTextMessage_native_cref_QString(long __this__nativeId, java.lang.String message);
/**
* See QWebSocket:: setMaskGenerator(const QMaskGenerator*)
* @param maskGenerator
*/
@QtUninvokable
public final void setMaskGenerator(io.qt.websockets.@Nullable QMaskGenerator maskGenerator){
InternalAccess.NativeIdInfo __maskGenerator__NativeIdInfo = QtJambi_LibraryUtilities.internal.checkedNativeIdInfo(maskGenerator);
setMaskGenerator_native_const_QMaskGenerator_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), __maskGenerator__NativeIdInfo.nativeId());
if (__maskGenerator__NativeIdInfo.needsReferenceCounting()) {
__rcMaskGenerator = maskGenerator;
}else{
__rcMaskGenerator = null;
}
}
@QtUninvokable
private native void setMaskGenerator_native_const_QMaskGenerator_ptr(long __this__nativeId, long maskGenerator);
/**
* See QWebSocket:: setMaxAllowedIncomingFrameSize(quint64)
* @param maxAllowedIncomingFrameSize
*/
@QtUninvokable
public final void setMaxAllowedIncomingFrameSize(long maxAllowedIncomingFrameSize){
setMaxAllowedIncomingFrameSize_native_quint64(QtJambi_LibraryUtilities.internal.nativeId(this), maxAllowedIncomingFrameSize);
}
@QtUninvokable
private native void setMaxAllowedIncomingFrameSize_native_quint64(long __this__nativeId, long maxAllowedIncomingFrameSize);
/**
* See QWebSocket:: setMaxAllowedIncomingMessageSize(quint64)
* @param maxAllowedIncomingMessageSize
*/
@QtUninvokable
public final void setMaxAllowedIncomingMessageSize(long maxAllowedIncomingMessageSize){
setMaxAllowedIncomingMessageSize_native_quint64(QtJambi_LibraryUtilities.internal.nativeId(this), maxAllowedIncomingMessageSize);
}
@QtUninvokable
private native void setMaxAllowedIncomingMessageSize_native_quint64(long __this__nativeId, long maxAllowedIncomingMessageSize);
/**
* See QWebSocket:: setOutgoingFrameSize(quint64)
* @param outgoingFrameSize
*/
@QtUninvokable
public final void setOutgoingFrameSize(long outgoingFrameSize){
setOutgoingFrameSize_native_quint64(QtJambi_LibraryUtilities.internal.nativeId(this), outgoingFrameSize);
}
@QtUninvokable
private native void setOutgoingFrameSize_native_quint64(long __this__nativeId, long outgoingFrameSize);
/**
* See QWebSocket:: setPauseMode(QAbstractSocket::PauseModes)
* @param pauseMode
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final void setPauseMode(io.qt.network.QAbstractSocket.@NonNull PauseModes pauseMode){
setPauseMode_native_QAbstractSocket_PauseModes(QtJambi_LibraryUtilities.internal.nativeId(this), pauseMode.value());
}
@QtUninvokable
private native void setPauseMode_native_QAbstractSocket_PauseModes(long __this__nativeId, int pauseMode);
/**
* See QWebSocket:: setProxy(QNetworkProxy)
* @param networkProxy
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final void setProxy(io.qt.network.@NonNull QNetworkProxy networkProxy){
setProxy_native_cref_QNetworkProxy(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(networkProxy));
}
@QtUninvokable
private native void setProxy_native_cref_QNetworkProxy(long __this__nativeId, long networkProxy);
/**
* See QWebSocket:: setReadBufferSize(qint64)
* @param size
*/
@QtUninvokable
public final void setReadBufferSize(long size){
setReadBufferSize_native_qint64(QtJambi_LibraryUtilities.internal.nativeId(this), size);
}
@QtUninvokable
private native void setReadBufferSize_native_qint64(long __this__nativeId, long size);
/**
* See QWebSocket:: setSslConfiguration(QSslConfiguration)
* @param sslConfiguration
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final void setSslConfiguration(io.qt.network.@NonNull QSslConfiguration sslConfiguration){
setSslConfiguration_native_cref_QSslConfiguration(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(sslConfiguration));
}
@QtUninvokable
private native void setSslConfiguration_native_cref_QSslConfiguration(long __this__nativeId, long sslConfiguration);
/**
* See QWebSocket:: sslConfiguration()const
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.@NonNull QSslConfiguration sslConfiguration(){
return sslConfiguration_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native io.qt.network.QSslConfiguration sslConfiguration_native_constfct(long __this__nativeId);
/**
* Returns the current state of the socket
*
* @return
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final io.qt.network.QAbstractSocket.@NonNull SocketState state(){
return io.qt.network.QAbstractSocket.SocketState.resolve(state_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int state_native_constfct(long __this__nativeId);
/**
* Returns the used WebSocket protocol
* See QWebSocket:: subprotocol()const
* @since This function was introduced in Qt 6.4.
* @return
*/
@QtUninvokable
public final java.lang.@NonNull String subprotocol(){
return subprotocol_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@QtUninvokable
private native java.lang.String subprotocol_native_constfct(long __this__nativeId);
/**
* Returns the version the socket is currently using
* See QWebSocket:: version()const
* @return
*/
@QtUninvokable
public final io.qt.websockets.QWebSocketProtocol.@NonNull Version version(){
return io.qt.websockets.QWebSocketProtocol.Version.resolve(version_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@QtUninvokable
private native int version_native_constfct(long __this__nativeId);
/**
* See QWebSocket:: maxIncomingFrameSize()
* @return
*/
public native static long maxIncomingFrameSize();
/**
* See QWebSocket:: maxIncomingMessageSize()
* @return
*/
public native static long maxIncomingMessageSize();
/**
* See QWebSocket:: maxOutgoingFrameSize()
* @return
*/
public native static long maxOutgoingFrameSize();
/**
* Constructor for internal use only.
* @param p expected to be null
.
* @hidden
*/
@NativeAccess
protected QWebSocket(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
* @hidden
*/
@NativeAccess
protected QWebSocket(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@QtUninvokable
private static native void initialize_native(QWebSocket instance, QDeclarativeConstructor constructor);
/**
* Overloaded constructor for {@link #QWebSocket(java.lang.String, io.qt.websockets.QWebSocketProtocol.Version, io.qt.core.QObject)}
* with parent = null
.
*/
public QWebSocket(java.lang.@NonNull String origin, io.qt.websockets.QWebSocketProtocol.@NonNull Version version) {
this(origin, version, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QWebSocket(java.lang.String, io.qt.websockets.QWebSocketProtocol.Version, io.qt.core.QObject)}
* with:
* version = io.qt.websockets.QWebSocketProtocol.Version.VersionLatest
* parent = null
*
*/
public QWebSocket(java.lang.@NonNull String origin) {
this(origin, io.qt.websockets.QWebSocketProtocol.Version.VersionLatest, (io.qt.core.QObject)null);
}
/**
* Overloaded constructor for {@link #QWebSocket(java.lang.String, io.qt.websockets.QWebSocketProtocol.Version, io.qt.core.QObject)}
* with:
* origin = (String)null
* version = io.qt.websockets.QWebSocketProtocol.Version.VersionLatest
* parent = null
*
*/
public QWebSocket() {
this((String)null, io.qt.websockets.QWebSocketProtocol.Version.VersionLatest, (io.qt.core.QObject)null);
}
/**
* Overloaded function for {@link #close(io.qt.websockets.QWebSocketProtocol.CloseCode, java.lang.String)}
* with reason = (String)null
.
*/
public final void close(io.qt.websockets.QWebSocketProtocol.@NonNull CloseCode closeCode) {
close(closeCode, (String)null);
}
/**
* Overloaded function for {@link #close(io.qt.websockets.QWebSocketProtocol.CloseCode, java.lang.String)}
* with:
* closeCode = io.qt.websockets.QWebSocketProtocol.CloseCode.CloseCodeNormal
* reason = (String)null
*
*/
public final void close() {
close(io.qt.websockets.QWebSocketProtocol.CloseCode.CloseCodeNormal, (String)null);
}
/**
* Overloaded function for {@link #open(io.qt.core.QUrl)}.
*/
public final void open(java.lang.@NonNull String url) {
open(new io.qt.core.QUrl(url));
}
/**
* Overloaded function for {@link #open(io.qt.core.QUrl, io.qt.websockets.QWebSocketHandshakeOptions)}.
*/
public final void open(java.lang.@NonNull String url, io.qt.websockets.@NonNull QWebSocketHandshakeOptions options) {
open(new io.qt.core.QUrl(url), options);
}
/**
* Overloaded function for {@link #ping(io.qt.core.QByteArray)}
* with payload = new io.qt.core.QByteArray()
.
*/
public final void ping() {
ping(new io.qt.core.QByteArray());
}
/**
* Overloaded function for {@link #ping(io.qt.core.QByteArray)}.
*/
public final void ping(byte @NonNull[] payload) {
ping(new io.qt.core.QByteArray(payload));
}
/**
* Overloaded function for {@link #sendBinaryMessage(io.qt.core.QByteArray)}.
*/
@QtUninvokable
public final long sendBinaryMessage(byte @NonNull[] data) {
return sendBinaryMessage(new io.qt.core.QByteArray(data));
}
/**
* Overloaded function for {@link #setPauseMode(io.qt.network.QAbstractSocket.PauseModes)}.
*/
@SuppressWarnings({"exports"})
@QtUninvokable
public final void setPauseMode(io.qt.network.QAbstractSocket.@NonNull PauseMode @NonNull... pauseMode) {
setPauseMode(new io.qt.network.QAbstractSocket.PauseModes(pauseMode));
}
}