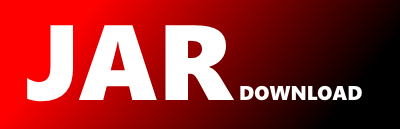
io.qt.core.QAbstractListModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Abstract model that can be subclassed to create one-dimensional list models
* Java wrapper for Qt class QAbstractListModel
*/
public abstract class QAbstractListModel extends io.qt.core.QAbstractItemModel
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QAbstractListModel.class);
@io.qt.NativeAccess
private static final class ConcreteWrapper extends QAbstractListModel {
@io.qt.NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
@Override
public java.lang.Object data(io.qt.core.QModelIndex index, int role){
return data_native_cref_QModelIndex_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), index, role);
}
private native java.lang.Object data_native_cref_QModelIndex_int_constfct(long __this__nativeId, io.qt.core.QModelIndex index, int role);
@Override
public int rowCount(io.qt.core.QModelIndex parent){
return rowCount_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), parent);
}
private native int rowCount_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex parent);
}
/**
* Overloaded constructor for {@link #QAbstractListModel(io.qt.core.QObject)}
* with parent = null
.
*/
public QAbstractListModel() {
this((io.qt.core.QObject)null);
}
/**
* See QAbstractListModel::QAbstractListModel(QObject*)
*/
public QAbstractListModel(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QAbstractListModel instance, io.qt.core.QObject parent);
/**
* See QAbstractItemModel::columnCount(QModelIndex)const
*/
@Deprecated
@io.qt.QtUninvokable
public final int columnCount(io.qt.core.QModelIndex parent) throws io.qt.QNoImplementationException {
return columnCount_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), parent);
}
@Deprecated
private static int columnCount_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex parent) throws io.qt.QNoImplementationException {
throw new io.qt.QNoImplementationException();
}
/**
* See QAbstractItemModel::hasChildren(QModelIndex)const
*/
@Deprecated
@io.qt.QtUninvokable
public final boolean hasChildren(io.qt.core.QModelIndex parent) throws io.qt.QNoImplementationException {
return hasChildren_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), parent);
}
@Deprecated
private static boolean hasChildren_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex parent) throws io.qt.QNoImplementationException {
throw new io.qt.QNoImplementationException();
}
/**
* See QAbstractItemModel::parent(QModelIndex)const
*/
@Deprecated
@io.qt.QtUninvokable
public final io.qt.core.QModelIndex parent(io.qt.core.QModelIndex child) throws io.qt.QNoImplementationException {
return parent_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), child);
}
@Deprecated
private static io.qt.core.QModelIndex parent_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex child) throws io.qt.QNoImplementationException {
throw new io.qt.QNoImplementationException();
}
/**
* See QAbstractItemModel::dropMimeData(const QMimeData*,Qt::DropAction,int,int,QModelIndex)
*/
@io.qt.QtUninvokable
public boolean dropMimeData(io.qt.core.QMimeData data, io.qt.core.Qt.DropAction action, int row, int column, io.qt.core.QModelIndex parent){
return dropMimeData_native_const_QMimeData_ptr_Qt_DropAction_int_int_cref_QModelIndex(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(data), action.value(), row, column, parent);
}
@io.qt.QtUninvokable
private native boolean dropMimeData_native_const_QMimeData_ptr_Qt_DropAction_int_int_cref_QModelIndex(long __this__nativeId, long data, int action, int row, int column, io.qt.core.QModelIndex parent);
/**
* See QAbstractItemModel::flags(QModelIndex)const
*/
@io.qt.QtUninvokable
public io.qt.core.Qt.ItemFlags flags(io.qt.core.QModelIndex index){
return new io.qt.core.Qt.ItemFlags(flags_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), index));
}
@io.qt.QtUninvokable
private native int flags_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex index);
/**
* See QAbstractItemModel::index(int,int,QModelIndex)const
*/
@io.qt.QtUninvokable
public io.qt.core.QModelIndex index(int row, int column, io.qt.core.QModelIndex parent){
return index_native_int_int_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), row, column, parent);
}
@io.qt.QtUninvokable
private native io.qt.core.QModelIndex index_native_int_int_cref_QModelIndex_constfct(long __this__nativeId, int row, int column, io.qt.core.QModelIndex parent);
/**
* See QAbstractItemModel::sibling(int,int,QModelIndex)const
*/
@io.qt.QtUninvokable
public io.qt.core.QModelIndex sibling(int row, int column, io.qt.core.QModelIndex idx){
return sibling_native_int_int_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), row, column, idx);
}
@io.qt.QtUninvokable
private native io.qt.core.QModelIndex sibling_native_int_int_cref_QModelIndex_constfct(long __this__nativeId, int row, int column, io.qt.core.QModelIndex idx);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QAbstractListModel(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QAbstractListModel(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QAbstractListModel instance, QDeclarativeConstructor constructor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy