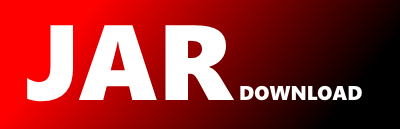
io.qt.core.QElapsedTimer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Fast way to calculate elapsed times
* Java wrapper for Qt class QElapsedTimer
*/
public class QElapsedTimer extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QElapsedTimer::ClockType
*/
public enum ClockType implements io.qt.QtEnumerator {
SystemTime(0),
MonotonicClock(1),
TickCounter(2),
MachAbsoluteTime(3),
PerformanceCounter(4);
private ClockType(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static ClockType resolve(int value) {
switch (value) {
case 0: return SystemTime;
case 1: return MonotonicClock;
case 2: return TickCounter;
case 3: return MachAbsoluteTime;
case 4: return PerformanceCounter;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QElapsedTimer::QElapsedTimer()
*/
public QElapsedTimer(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QElapsedTimer instance);
/**
* See QElapsedTimer::elapsed()const
*/
@io.qt.QtUninvokable
public final long elapsed(){
return elapsed_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long elapsed_native_constfct(long __this__nativeId);
/**
* See QElapsedTimer::hasExpired(qint64)const
*/
@io.qt.QtUninvokable
public final boolean hasExpired(long timeout){
return hasExpired_native_long_long_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), timeout);
}
@io.qt.QtUninvokable
private native boolean hasExpired_native_long_long_constfct(long __this__nativeId, long timeout);
/**
* See QElapsedTimer::invalidate()
*/
@io.qt.QtUninvokable
public final void invalidate(){
invalidate_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void invalidate_native(long __this__nativeId);
/**
* See QElapsedTimer::isValid()const
*/
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
/**
* See QElapsedTimer::msecsSinceReference()const
*/
@io.qt.QtUninvokable
public final long msecsSinceReference(){
return msecsSinceReference_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long msecsSinceReference_native_constfct(long __this__nativeId);
/**
* See QElapsedTimer::msecsTo(QElapsedTimer)const
*/
@io.qt.QtUninvokable
public final long msecsTo(io.qt.core.QElapsedTimer other){
return msecsTo_native_cref_QElapsedTimer_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native long msecsTo_native_cref_QElapsedTimer_constfct(long __this__nativeId, long other);
/**
* See QElapsedTimer::nsecsElapsed()const
*/
@io.qt.QtUninvokable
public final long nsecsElapsed(){
return nsecsElapsed_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long nsecsElapsed_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.core.QElapsedTimer rhs){
return operator_equal_native_cref_QElapsedTimer(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(rhs));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QElapsedTimer(long __this__nativeId, long rhs);
/**
*
*/
@io.qt.QtUninvokable
public final long restart(){
return restart_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native long restart_native(long __this__nativeId);
/**
* See QElapsedTimer::secsTo(QElapsedTimer)const
*/
@io.qt.QtUninvokable
public final long secsTo(io.qt.core.QElapsedTimer other){
return secsTo_native_cref_QElapsedTimer_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native long secsTo_native_cref_QElapsedTimer_constfct(long __this__nativeId, long other);
/**
*
*/
@io.qt.QtUninvokable
public final void start(){
start_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void start_native(long __this__nativeId);
/**
* See QElapsedTimer::clockType()
*/
public static io.qt.core.QElapsedTimer.ClockType clockType(){
return io.qt.core.QElapsedTimer.ClockType.resolve(clockType_native());
}
private native static int clockType_native();
/**
* See QElapsedTimer::isMonotonic()
*/
public native static boolean isMonotonic();
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QElapsedTimer(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.core.QElapsedTimer) {
return operator_equal((io.qt.core.QElapsedTimer) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@Override
public QElapsedTimer clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QElapsedTimer clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy