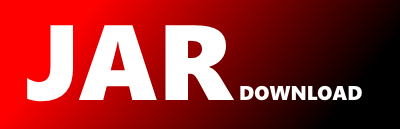
io.qt.core.QEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* The base class of all event classes. Event objects contain event parameters
* Java wrapper for Qt class QEvent
*/
public class QEvent extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QEvent.class);
/**
* Java wrapper for Qt enum QEvent::Type
*/
@io.qt.QtExtensibleEnum
@io.qt.QtRejectedEntries({"ApplicationActivated", "ApplicationDeactivated"})
public enum Type implements io.qt.QtEnumerator {
None(0),
Timer(1),
MouseButtonPress(2),
MouseButtonRelease(3),
MouseButtonDblClick(4),
MouseMove(5),
KeyPress(6),
KeyRelease(7),
FocusIn(8),
FocusOut(9),
FocusAboutToChange(23),
Enter(10),
Leave(11),
Paint(12),
Move(13),
Resize(14),
Create(15),
Destroy(16),
Show(17),
Hide(18),
Close(19),
Quit(20),
ParentChange(21),
ParentAboutToChange(131),
ThreadChange(22),
WindowActivate(24),
WindowDeactivate(25),
ShowToParent(26),
HideToParent(27),
Wheel(31),
WindowTitleChange(33),
WindowIconChange(34),
ApplicationWindowIconChange(35),
ApplicationFontChange(36),
ApplicationLayoutDirectionChange(37),
ApplicationPaletteChange(38),
PaletteChange(39),
Clipboard(40),
Speech(42),
MetaCall(43),
SockAct(50),
WinEventAct(132),
DeferredDispose(52),
DragEnter(60),
DragMove(61),
DragLeave(62),
Drop(63),
DragResponse(64),
ChildAdded(68),
ChildPolished(69),
ChildRemoved(71),
ShowWindowRequest(73),
PolishRequest(74),
Polish(75),
LayoutRequest(76),
UpdateRequest(77),
UpdateLater(78),
EmbeddingControl(79),
ActivateControl(80),
DeactivateControl(81),
ContextMenu(82),
InputMethod(83),
TabletMove(87),
LocaleChange(88),
LanguageChange(89),
LayoutDirectionChange(90),
Style(91),
TabletPress(92),
TabletRelease(93),
OkRequest(94),
HelpRequest(95),
IconDrag(96),
FontChange(97),
EnabledChange(98),
ActivationChange(99),
StyleChange(100),
IconTextChange(101),
ModifiedChange(102),
MouseTrackingChange(109),
WindowBlocked(103),
WindowUnblocked(104),
WindowStateChange(105),
ReadOnlyChange(106),
ToolTip(110),
WhatsThis(111),
StatusTip(112),
ActionChanged(113),
ActionAdded(114),
ActionRemoved(115),
FileOpen(116),
Shortcut(117),
ShortcutOverride(51),
WhatsThisClicked(118),
ToolBarChange(120),
ApplicationActivate(121),
ApplicationActivated(121),
ApplicationDeactivate(122),
ApplicationDeactivated(122),
QueryWhatsThis(123),
EnterWhatsThisMode(124),
LeaveWhatsThisMode(125),
ZOrderChange(126),
HoverEnter(127),
HoverLeave(128),
HoverMove(129),
AcceptDropsChange(152),
ZeroTimerEvent(154),
GraphicsSceneMouseMove(155),
GraphicsSceneMousePress(156),
GraphicsSceneMouseRelease(157),
GraphicsSceneMouseDoubleClick(158),
GraphicsSceneContextMenu(159),
GraphicsSceneHoverEnter(160),
GraphicsSceneHoverMove(161),
GraphicsSceneHoverLeave(162),
GraphicsSceneHelp(163),
GraphicsSceneDragEnter(164),
GraphicsSceneDragMove(165),
GraphicsSceneDragLeave(166),
GraphicsSceneDrop(167),
GraphicsSceneWheel(168),
GraphicsSceneLeave(220),
KeyboardLayoutChange(169),
DynamicPropertyChange(170),
TabletEnterProximity(171),
TabletLeaveProximity(172),
NonClientAreaMouseMove(173),
NonClientAreaMouseButtonPress(174),
NonClientAreaMouseButtonRelease(175),
NonClientAreaMouseButtonDblClick(176),
MacSizeChange(177),
ContentsRectChange(178),
MacGLWindowChange(179),
FutureCallOut(180),
GraphicsSceneResize(181),
GraphicsSceneMove(182),
CursorChange(183),
ToolTipChange(184),
NetworkReplyUpdated(185),
GrabMouse(186),
UngrabMouse(187),
GrabKeyboard(188),
UngrabKeyboard(189),
StateMachineSignal(192),
StateMachineWrapped(193),
TouchBegin(194),
TouchUpdate(195),
TouchEnd(196),
NativeGesture(197),
RequestSoftwareInputPanel(199),
CloseSoftwareInputPanel(200),
WinIdChange(203),
Gesture(198),
GestureOverride(202),
ScrollPrepare(204),
Scroll(205),
Expose(206),
InputMethodQuery(207),
OrientationChange(208),
TouchCancel(209),
ThemeChange(210),
SockClose(211),
PlatformPanel(212),
StyleAnimationUpdate(213),
ApplicationStateChange(214),
WindowChangeInternal(215),
ScreenChangeInternal(216),
PlatformSurface(217),
Pointer(218),
TabletTrackingChange(219),
User(1000),
MaxUser(65535);
private Type(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
* @throws io.qt.QNoSuchEnumValueException if value not existent in the enum
*/
public static Type resolve(int value) {
switch (value) {
case 0: return None;
case 1: return Timer;
case 2: return MouseButtonPress;
case 3: return MouseButtonRelease;
case 4: return MouseButtonDblClick;
case 5: return MouseMove;
case 6: return KeyPress;
case 7: return KeyRelease;
case 8: return FocusIn;
case 9: return FocusOut;
case 23: return FocusAboutToChange;
case 10: return Enter;
case 11: return Leave;
case 12: return Paint;
case 13: return Move;
case 14: return Resize;
case 15: return Create;
case 16: return Destroy;
case 17: return Show;
case 18: return Hide;
case 19: return Close;
case 20: return Quit;
case 21: return ParentChange;
case 131: return ParentAboutToChange;
case 22: return ThreadChange;
case 24: return WindowActivate;
case 25: return WindowDeactivate;
case 26: return ShowToParent;
case 27: return HideToParent;
case 31: return Wheel;
case 33: return WindowTitleChange;
case 34: return WindowIconChange;
case 35: return ApplicationWindowIconChange;
case 36: return ApplicationFontChange;
case 37: return ApplicationLayoutDirectionChange;
case 38: return ApplicationPaletteChange;
case 39: return PaletteChange;
case 40: return Clipboard;
case 42: return Speech;
case 43: return MetaCall;
case 50: return SockAct;
case 132: return WinEventAct;
case 52: return DeferredDispose;
case 60: return DragEnter;
case 61: return DragMove;
case 62: return DragLeave;
case 63: return Drop;
case 64: return DragResponse;
case 68: return ChildAdded;
case 69: return ChildPolished;
case 71: return ChildRemoved;
case 73: return ShowWindowRequest;
case 74: return PolishRequest;
case 75: return Polish;
case 76: return LayoutRequest;
case 77: return UpdateRequest;
case 78: return UpdateLater;
case 79: return EmbeddingControl;
case 80: return ActivateControl;
case 81: return DeactivateControl;
case 82: return ContextMenu;
case 83: return InputMethod;
case 87: return TabletMove;
case 88: return LocaleChange;
case 89: return LanguageChange;
case 90: return LayoutDirectionChange;
case 91: return Style;
case 92: return TabletPress;
case 93: return TabletRelease;
case 94: return OkRequest;
case 95: return HelpRequest;
case 96: return IconDrag;
case 97: return FontChange;
case 98: return EnabledChange;
case 99: return ActivationChange;
case 100: return StyleChange;
case 101: return IconTextChange;
case 102: return ModifiedChange;
case 109: return MouseTrackingChange;
case 103: return WindowBlocked;
case 104: return WindowUnblocked;
case 105: return WindowStateChange;
case 106: return ReadOnlyChange;
case 110: return ToolTip;
case 111: return WhatsThis;
case 112: return StatusTip;
case 113: return ActionChanged;
case 114: return ActionAdded;
case 115: return ActionRemoved;
case 116: return FileOpen;
case 117: return Shortcut;
case 51: return ShortcutOverride;
case 118: return WhatsThisClicked;
case 120: return ToolBarChange;
case 121: return ApplicationActivate;
case 122: return ApplicationDeactivate;
case 123: return QueryWhatsThis;
case 124: return EnterWhatsThisMode;
case 125: return LeaveWhatsThisMode;
case 126: return ZOrderChange;
case 127: return HoverEnter;
case 128: return HoverLeave;
case 129: return HoverMove;
case 152: return AcceptDropsChange;
case 154: return ZeroTimerEvent;
case 155: return GraphicsSceneMouseMove;
case 156: return GraphicsSceneMousePress;
case 157: return GraphicsSceneMouseRelease;
case 158: return GraphicsSceneMouseDoubleClick;
case 159: return GraphicsSceneContextMenu;
case 160: return GraphicsSceneHoverEnter;
case 161: return GraphicsSceneHoverMove;
case 162: return GraphicsSceneHoverLeave;
case 163: return GraphicsSceneHelp;
case 164: return GraphicsSceneDragEnter;
case 165: return GraphicsSceneDragMove;
case 166: return GraphicsSceneDragLeave;
case 167: return GraphicsSceneDrop;
case 168: return GraphicsSceneWheel;
case 220: return GraphicsSceneLeave;
case 169: return KeyboardLayoutChange;
case 170: return DynamicPropertyChange;
case 171: return TabletEnterProximity;
case 172: return TabletLeaveProximity;
case 173: return NonClientAreaMouseMove;
case 174: return NonClientAreaMouseButtonPress;
case 175: return NonClientAreaMouseButtonRelease;
case 176: return NonClientAreaMouseButtonDblClick;
case 177: return MacSizeChange;
case 178: return ContentsRectChange;
case 179: return MacGLWindowChange;
case 180: return FutureCallOut;
case 181: return GraphicsSceneResize;
case 182: return GraphicsSceneMove;
case 183: return CursorChange;
case 184: return ToolTipChange;
case 185: return NetworkReplyUpdated;
case 186: return GrabMouse;
case 187: return UngrabMouse;
case 188: return GrabKeyboard;
case 189: return UngrabKeyboard;
case 192: return StateMachineSignal;
case 193: return StateMachineWrapped;
case 194: return TouchBegin;
case 195: return TouchUpdate;
case 196: return TouchEnd;
case 197: return NativeGesture;
case 199: return RequestSoftwareInputPanel;
case 200: return CloseSoftwareInputPanel;
case 203: return WinIdChange;
case 198: return Gesture;
case 202: return GestureOverride;
case 204: return ScrollPrepare;
case 205: return Scroll;
case 206: return Expose;
case 207: return InputMethodQuery;
case 208: return OrientationChange;
case 209: return TouchCancel;
case 210: return ThemeChange;
case 211: return SockClose;
case 212: return PlatformPanel;
case 213: return StyleAnimationUpdate;
case 214: return ApplicationStateChange;
case 215: return WindowChangeInternal;
case 216: return ScreenChangeInternal;
case 217: return PlatformSurface;
case 218: return Pointer;
case 219: return TabletTrackingChange;
case 1000: return User;
case 65535: return MaxUser;
default: return QtJambi_LibraryUtilities.internal.resolveEnum(Type.class, value, null);
}
}
/**
* Returns the corresponding enum entry for the given value and name.
* @param value
* @param name
* @return enum entry
* @throws io.qt.QNoSuchEnumValueException if value not existent in the enum or name does not match.
*/
public static Type resolve(int value, String name) {
if(name==null || name.isEmpty())
return resolve(value);
else
return QtJambi_LibraryUtilities.internal.resolveEnum(Type.class, value, name);
}
private final int value;
}
/**
* See QEvent::QEvent(QEvent::Type)
*/
public QEvent(io.qt.core.QEvent.Type type){
super((QPrivateConstructor)null);
initialize_native(this, type);
}
private native static void initialize_native(QEvent instance, io.qt.core.QEvent.Type type);
/**
*
*/
protected QEvent(io.qt.core.QEvent arg__1){
super((QPrivateConstructor)null);
java.util.Objects.requireNonNull(arg__1, "Argument 'arg__1': null not expected.");
initialize_native(this, arg__1);
}
private native static void initialize_native(QEvent instance, io.qt.core.QEvent arg__1);
/**
* See QEvent::accept()
*/
@io.qt.QtUninvokable
public final void accept(){
accept_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void accept_native(long __this__nativeId);
/**
* See QEvent::ignore()
*/
@io.qt.QtUninvokable
public final void ignore(){
ignore_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void ignore_native(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final boolean isAccepted(){
return isAccepted_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isAccepted_native_constfct(long __this__nativeId);
/**
* See QEvent::isInputEvent()const
*/
@io.qt.QtUninvokable
public final boolean isInputEvent(){
return isInputEvent_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isInputEvent_native_constfct(long __this__nativeId);
/**
* See QEvent::isPointerEvent()const
*/
@io.qt.QtUninvokable
public final boolean isPointerEvent(){
return isPointerEvent_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isPointerEvent_native_constfct(long __this__nativeId);
/**
* See QEvent::isSinglePointEvent()const
*/
@io.qt.QtUninvokable
public final boolean isSinglePointEvent(){
return isSinglePointEvent_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isSinglePointEvent_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final void set(io.qt.core.QEvent other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
set_native_cref_QEvent(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native void set_native_cref_QEvent(long __this__nativeId, long other);
/**
* See QEvent::spontaneous()const
*/
@io.qt.QtUninvokable
public final boolean spontaneous(){
return spontaneous_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean spontaneous_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.core.QEvent.Type type(){
return io.qt.core.QEvent.Type.resolve(type_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int type_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public io.qt.core.QEvent clone(){
return clone_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QEvent clone_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public void setAccepted(boolean accepted){
setAccepted_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), accepted);
}
@io.qt.QtUninvokable
private native void setAccepted_native_bool(long __this__nativeId, boolean accepted);
/**
* Overloaded function for {@link #registerEventType(int)}
* with hint = -1
.
*/
public static int registerEventType() {
return registerEventType((int)-1);
}
/**
* See QEvent::registerEventType(int)
*/
public native static int registerEventType(int hint);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QEvent(QPrivateConstructor p) { super(p); }
@Override
public String toString() {
return getClass().getSimpleName() + "(type=" + type().name() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy