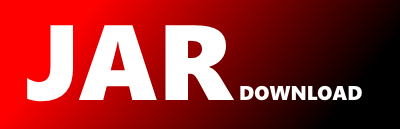
io.qt.core.QFutureInterfaceBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Java wrapper for Qt class QFutureInterfaceBase
*/
public class QFutureInterfaceBase extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
@io.qt.QtPropertyMember(enabled=false)
private Object __rcThreadPool = null;
@io.qt.QtPropertyMember(enabled=false)
private Object __rcRunnable = null;
/**
* Java wrapper for Qt enum QFutureInterfaceBase::State
*/
@io.qt.QtExtensibleEnum
public enum State implements io.qt.QtEnumerator {
NoState(0),
Running(1),
Started(2),
Finished(4),
Canceled(8),
Suspending(16),
Suspended(32),
Throttled(64),
Pending(128);
private State(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
* @throws io.qt.QNoSuchEnumValueException if value not existent in the enum
*/
public static State resolve(int value) {
switch (value) {
case 0: return NoState;
case 1: return Running;
case 2: return Started;
case 4: return Finished;
case 8: return Canceled;
case 16: return Suspending;
case 32: return Suspended;
case 64: return Throttled;
case 128: return Pending;
default: return QtJambi_LibraryUtilities.internal.resolveEnum(State.class, value, null);
}
}
/**
* Returns the corresponding enum entry for the given value and name.
* @param value
* @param name
* @return enum entry
* @throws io.qt.QNoSuchEnumValueException if value not existent in the enum or name does not match.
*/
public static State resolve(int value, String name) {
if(name==null || name.isEmpty())
return resolve(value);
else
return QtJambi_LibraryUtilities.internal.resolveEnum(State.class, value, name);
}
private final int value;
}
/**
* Overloaded constructor for {@link #QFutureInterfaceBase(io.qt.core.QFutureInterfaceBase.State)}
* with initialState = io.qt.core.QFutureInterfaceBase.State.NoState
.
*/
public QFutureInterfaceBase() {
this(io.qt.core.QFutureInterfaceBase.State.NoState);
}
public QFutureInterfaceBase(io.qt.core.QFutureInterfaceBase.State initialState){
super((QPrivateConstructor)null);
initialize_native(this, initialState);
}
private native static void initialize_native(QFutureInterfaceBase instance, io.qt.core.QFutureInterfaceBase.State initialState);
public QFutureInterfaceBase(io.qt.core.QFutureInterfaceBase other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QFutureInterfaceBase instance, io.qt.core.QFutureInterfaceBase other);
@io.qt.QtUninvokable
public final void cancel(){
cancel_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void cancel_native(long __this__nativeId);
@io.qt.QtUninvokable
protected final void cleanContinuation(){
cleanContinuation_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void cleanContinuation_native(long __this__nativeId);
@io.qt.QtUninvokable
protected final boolean derefT(){
return derefT_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean derefT_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final int expectedResultCount(){
return expectedResultCount_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int expectedResultCount_native(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isCanceled(){
return isCanceled_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isCanceled_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isChainCanceled(){
return isChainCanceled_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isChainCanceled_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isFinished(){
return isFinished_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isFinished_native_constfct(long __this__nativeId);
/**
* @deprecated Use isSuspending() or isSuspended() instead.
*/
@Deprecated
@io.qt.QtUninvokable
public final boolean isPaused(){
return isPaused_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native boolean isPaused_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isProgressUpdateNeeded(){
return isProgressUpdateNeeded_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isProgressUpdateNeeded_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isResultReadyAt(int index){
return isResultReadyAt_native_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), index);
}
@io.qt.QtUninvokable
private native boolean isResultReadyAt_native_int_constfct(long __this__nativeId, int index);
@io.qt.QtUninvokable
public final boolean isRunning(){
return isRunning_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isRunning_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final boolean isRunningOrPending(){
return isRunningOrPending_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isRunningOrPending_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isStarted(){
return isStarted_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isStarted_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isSuspended(){
return isSuspended_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isSuspended_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isSuspending(){
return isSuspending_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isSuspending_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isThrottled(){
return isThrottled_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isThrottled_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isValid(){
return isValid_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isValid_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final boolean launchAsync(){
return launchAsync_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean launchAsync_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final int loadState(){
return loadState_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int loadState_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final io.qt.core.QMutex mutex(){
return mutex_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QMutex mutex_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
private final boolean operator_equal(io.qt.core.QFutureInterfaceBase other){
return operator_equal_native_cref_QFutureInterfaceBase_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native boolean operator_equal_native_cref_QFutureInterfaceBase_constfct(long __this__nativeId, long other);
@io.qt.QtUninvokable
public final int progressMaximum(){
return progressMaximum_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int progressMaximum_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final int progressMinimum(){
return progressMinimum_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int progressMinimum_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final java.lang.String progressText(){
return progressText_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String progressText_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final int progressValue(){
return progressValue_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int progressValue_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean queryState(io.qt.core.QFutureInterfaceBase.State state){
return queryState_native_QFutureInterfaceBase_State_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), state.value());
}
@io.qt.QtUninvokable
private native boolean queryState_native_QFutureInterfaceBase_State_constfct(long __this__nativeId, int state);
@io.qt.QtUninvokable
protected final boolean refT(){
return refT_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean refT_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final void reportCanceled(){
reportCanceled_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void reportCanceled_native(long __this__nativeId);
@io.qt.QtDeclaredFinal
@io.qt.QtUninvokable
public void reportFinished(){
reportFinished_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void reportFinished_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void reportResultsReady(int beginIndex, int endIndex){
reportResultsReady_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), beginIndex, endIndex);
}
@io.qt.QtUninvokable
private native void reportResultsReady_native_int_int(long __this__nativeId, int beginIndex, int endIndex);
@io.qt.QtUninvokable
public final void reportStarted(){
reportStarted_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void reportStarted_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void reportSuspended(){
reportSuspended_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void reportSuspended_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final void reset(){
reset_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void reset_native(long __this__nativeId);
@io.qt.QtUninvokable
public final int resultCount(){
return resultCount_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int resultCount_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final void rethrowPossibleException(){
rethrowPossibleException_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void rethrowPossibleException_native(long __this__nativeId);
@io.qt.QtUninvokable
protected final void runContinuation(){
runContinuation_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void runContinuation_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
protected final void setContinuation(java.util.function.Consumer func){
setContinuation_native_QFutureInterfaceBase_Continuation(QtJambi_LibraryUtilities.internal.nativeId(this), func);
}
@io.qt.QtUninvokable
private native void setContinuation_native_QFutureInterfaceBase_Continuation(long __this__nativeId, java.util.function.Consumer func);
@io.qt.QtUninvokable
public final void setExpectedResultCount(int resultCount){
setExpectedResultCount_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), resultCount);
}
@io.qt.QtUninvokable
private native void setExpectedResultCount_native_int(long __this__nativeId, int resultCount);
@io.qt.QtUninvokable
public final void setFilterMode(boolean enable){
setFilterMode_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), enable);
}
@io.qt.QtUninvokable
private native void setFilterMode_native_bool(long __this__nativeId, boolean enable);
@io.qt.QtUninvokable
protected final void setLaunchAsync(boolean value){
setLaunchAsync_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), value);
}
@io.qt.QtUninvokable
private native void setLaunchAsync_native_bool(long __this__nativeId, boolean value);
/**
* @deprecated Use setSuspended() instead.
*/
@Deprecated
@io.qt.QtUninvokable
public final void setPaused(boolean paused){
setPaused_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), paused);
}
@Deprecated
@io.qt.QtUninvokable
private native void setPaused_native_bool(long __this__nativeId, boolean paused);
@io.qt.QtUninvokable
public final void setProgressRange(int minimum, int maximum){
setProgressRange_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), minimum, maximum);
}
@io.qt.QtUninvokable
private native void setProgressRange_native_int_int(long __this__nativeId, int minimum, int maximum);
@io.qt.QtUninvokable
public final void setProgressValue(int progressValue){
setProgressValue_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), progressValue);
}
@io.qt.QtUninvokable
private native void setProgressValue_native_int(long __this__nativeId, int progressValue);
@io.qt.QtUninvokable
public final void setProgressValueAndText(int progressValue, java.lang.String progressText){
setProgressValueAndText_native_int_cref_QString(QtJambi_LibraryUtilities.internal.nativeId(this), progressValue, progressText);
}
@io.qt.QtUninvokable
private native void setProgressValueAndText_native_int_cref_QString(long __this__nativeId, int progressValue, java.lang.String progressText);
@io.qt.QtUninvokable
public final void setRunnable(io.qt.core.QRunnable runnable){
setRunnable_native_QRunnable_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), runnable);
__rcRunnable = runnable;
}
@io.qt.QtUninvokable
private native void setRunnable_native_QRunnable_ptr(long __this__nativeId, io.qt.core.QRunnable runnable);
@io.qt.QtUninvokable
public final void setSuspended(boolean suspend){
setSuspended_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), suspend);
}
@io.qt.QtUninvokable
private native void setSuspended_native_bool(long __this__nativeId, boolean suspend);
@io.qt.QtUninvokable
public final void setThreadPool(io.qt.core.QThreadPool pool){
setThreadPool_native_QThreadPool_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(pool));
__rcThreadPool = pool;
}
@io.qt.QtUninvokable
private native void setThreadPool_native_QThreadPool_ptr(long __this__nativeId, long pool);
@io.qt.QtUninvokable
public final void setThrottled(boolean enable){
setThrottled_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), enable);
}
@io.qt.QtUninvokable
private native void setThrottled_native_bool(long __this__nativeId, boolean enable);
@io.qt.QtUninvokable
public final void suspendIfRequested(){
suspendIfRequested_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void suspendIfRequested_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void swap(io.qt.core.QFutureInterfaceBase other){
java.util.Objects.requireNonNull(other, "Argument 'other': null not expected.");
swap_native_ref_QFutureInterfaceBase(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
@io.qt.QtUninvokable
private native void swap_native_ref_QFutureInterfaceBase(long __this__nativeId, long other);
@io.qt.QtUninvokable
public final io.qt.core.QThreadPool threadPool(){
return threadPool_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QThreadPool threadPool_native_constfct(long __this__nativeId);
/**
* @deprecated Use toggleSuspended() instead.
*/
@Deprecated
@io.qt.QtUninvokable
public final void togglePaused(){
togglePaused_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native void togglePaused_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void toggleSuspended(){
toggleSuspended_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void toggleSuspended_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void waitForFinished(){
waitForFinished_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void waitForFinished_native(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean waitForNextResult(){
return waitForNextResult_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean waitForNextResult_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void waitForResult(int resultIndex){
waitForResult_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), resultIndex);
}
@io.qt.QtUninvokable
private native void waitForResult_native_int(long __this__nativeId, int resultIndex);
@io.qt.QtUninvokable
public final void waitForResume(){
waitForResume_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void waitForResume_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QFutureInterfaceBase(QPrivateConstructor p) { super(p); }
@Override
@io.qt.QtUninvokable
public boolean equals(Object other) {
if (other instanceof io.qt.core.QFutureInterfaceBase) {
return operator_equal((io.qt.core.QFutureInterfaceBase) other);
}
return false;
}
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
@io.qt.QtUninvokable
public native final void reportException(Throwable e);
@Override
public QFutureInterfaceBase clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QFutureInterfaceBase clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy