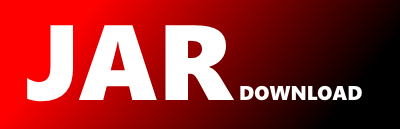
io.qt.core.QFutureWatcherBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Java wrapper for Qt class QFutureWatcherBase
* The following private function is pure virtual in Qt and thus has to
* be implemented in derived Java classes by using the @QtPrivateOverride annotation:
*
*
* @io.qt.QtPrivateOverride
* @io.qt.QtUninvokable
* private io.qt.core.QFutureInterfaceBase futureInterface() {...}
*
*/
public abstract class QFutureWatcherBase extends io.qt.core.QObject
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QFutureWatcherBase.class);
@io.qt.NativeAccess
private static final class ConcreteWrapper extends QFutureWatcherBase {
@io.qt.NativeAccess
private ConcreteWrapper(QPrivateConstructor p) { super(p); }
}
public final Signal0 canceled = new Signal0();
public final Signal0 finished = new Signal0();
/**
* @deprecated Use suspending() instead.
*/
@Deprecated
public final Signal0 paused = new Signal0();
public final Signal2<@io.qt.QtPrimitiveType Integer, @io.qt.QtPrimitiveType Integer> progressRangeChanged = new Signal2<>();
public final Signal1 progressTextChanged = new Signal1<>();
public final Signal1<@io.qt.QtPrimitiveType Integer> progressValueChanged = new Signal1<>();
public final Signal1<@io.qt.QtPrimitiveType Integer> resultReadyAt = new Signal1<>();
public final Signal2<@io.qt.QtPrimitiveType Integer, @io.qt.QtPrimitiveType Integer> resultsReadyAt = new Signal2<>();
public final Signal0 resumed = new Signal0();
public final Signal0 started = new Signal0();
public final Signal0 suspended = new Signal0();
public final Signal0 suspending = new Signal0();
/**
* Overloaded constructor for {@link #QFutureWatcherBase(io.qt.core.QObject)}
* with parent = null
.
*/
public QFutureWatcherBase() {
this((io.qt.core.QObject)null);
}
public QFutureWatcherBase(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QFutureWatcherBase instance, io.qt.core.QObject parent);
public final void cancel(){
cancel_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void cancel_native(long __this__nativeId);
@io.qt.QtUninvokable
protected final void connectOutputInterface(){
connectOutputInterface_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void connectOutputInterface_native(long __this__nativeId);
/**
* Overloaded function for {@link #disconnectOutputInterface(boolean)}
* with pendingAssignment = false
.
*/
@io.qt.QtUninvokable
protected final void disconnectOutputInterface() {
disconnectOutputInterface((boolean)false);
}
@io.qt.QtUninvokable
protected final void disconnectOutputInterface(boolean pendingAssignment){
disconnectOutputInterface_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), pendingAssignment);
}
@io.qt.QtUninvokable
private native void disconnectOutputInterface_native_bool(long __this__nativeId, boolean pendingAssignment);
@io.qt.QtUninvokable
public final boolean isCanceled(){
return isCanceled_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isCanceled_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isFinished(){
return isFinished_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isFinished_native_constfct(long __this__nativeId);
/**
* @deprecated Use isSuspending() or isSuspended() instead.
*/
@Deprecated
@io.qt.QtUninvokable
public final boolean isPaused(){
return isPaused_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
@io.qt.QtUninvokable
private native boolean isPaused_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isRunning(){
return isRunning_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isRunning_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isStarted(){
return isStarted_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isStarted_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isSuspended(){
return isSuspended_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isSuspended_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean isSuspending(){
return isSuspending_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isSuspending_native_constfct(long __this__nativeId);
/**
* @deprecated Use suspended() instead.
*/
@Deprecated
public final void pause(){
pause_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
private native void pause_native(long __this__nativeId);
@io.qt.QtUninvokable
public final int progressMaximum(){
return progressMaximum_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int progressMaximum_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final int progressMinimum(){
return progressMinimum_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int progressMinimum_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final java.lang.String progressText(){
return progressText_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String progressText_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final int progressValue(){
return progressValue_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int progressValue_native_constfct(long __this__nativeId);
public final void resume(){
resume_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void resume_native(long __this__nativeId);
/**
* @deprecated Use setSuspended() instead.
*/
@Deprecated
public final void setPaused(boolean paused){
setPaused_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), paused);
}
@Deprecated
private native void setPaused_native_bool(long __this__nativeId, boolean paused);
@io.qt.QtUninvokable
public final void setPendingResultsLimit(int limit){
setPendingResultsLimit_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), limit);
}
@io.qt.QtUninvokable
private native void setPendingResultsLimit_native_int(long __this__nativeId, int limit);
public final void setSuspended(boolean suspend){
setSuspended_native_bool(QtJambi_LibraryUtilities.internal.nativeId(this), suspend);
}
private native void setSuspended_native_bool(long __this__nativeId, boolean suspend);
public final void suspend(){
suspend_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void suspend_native(long __this__nativeId);
/**
* @deprecated Use toggleSuspended() instead.
*/
@Deprecated
public final void togglePaused(){
togglePaused_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@Deprecated
private native void togglePaused_native(long __this__nativeId);
public final void toggleSuspended(){
toggleSuspended_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native void toggleSuspended_native(long __this__nativeId);
@io.qt.QtUninvokable
public final void waitForFinished(){
waitForFinished_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void waitForFinished_native(long __this__nativeId);
@io.qt.QtUninvokable
protected void connectNotify(io.qt.core.QMetaMethod signal){
connectNotify_native_cref_QMetaMethod(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(signal));
}
@io.qt.QtUninvokable
private native void connectNotify_native_cref_QMetaMethod(long __this__nativeId, long signal);
@io.qt.QtUninvokable
protected void disconnectNotify(io.qt.core.QMetaMethod signal){
disconnectNotify_native_cref_QMetaMethod(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(signal));
}
@io.qt.QtUninvokable
private native void disconnectNotify_native_cref_QMetaMethod(long __this__nativeId, long signal);
@io.qt.QtUninvokable
public boolean event(io.qt.core.QEvent event){
return event_native_QEvent_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(event));
}
@io.qt.QtUninvokable
private native boolean event_native_QEvent_ptr(long __this__nativeId, long event);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QFutureWatcherBase(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QFutureWatcherBase(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QFutureWatcherBase instance, QDeclarativeConstructor constructor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy