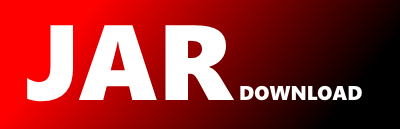
io.qt.core.QItemSelection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Manages information about selected items in a model
* Java wrapper for Qt class QList
*/
public class QItemSelection extends io.qt.core.QList
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* See QItemSelection::QItemSelection(QModelIndex,QModelIndex)
*/
public QItemSelection(io.qt.core.QModelIndex topLeft, io.qt.core.QModelIndex bottomRight){
super((QPrivateConstructor)null);
initialize_native(this, topLeft, bottomRight);
}
private native static void initialize_native(QItemSelection instance, io.qt.core.QModelIndex topLeft, io.qt.core.QModelIndex bottomRight);
/**
* See QItemSelection::contains(QModelIndex)const
*/
@io.qt.QtUninvokable
public final boolean contains(io.qt.core.QModelIndex index){
return contains_native_cref_QModelIndex_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), index);
}
@io.qt.QtUninvokable
private native boolean contains_native_cref_QModelIndex_constfct(long __this__nativeId, io.qt.core.QModelIndex index);
/**
* See QItemSelection::indexes()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QList indexes(){
return indexes_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QList indexes_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #merge(io.qt.core.QItemSelection, io.qt.core.QItemSelectionModel.SelectionFlags)}.
*/
@io.qt.QtUninvokable
public final void merge(io.qt.core.QItemSelection other, io.qt.core.QItemSelectionModel.SelectionFlag ... command){
merge(other, new io.qt.core.QItemSelectionModel.SelectionFlags(command));
}
/**
* See QItemSelection::merge(QItemSelection,QItemSelectionModel::SelectionFlags)
*/
@io.qt.QtUninvokable
public final void merge(io.qt.core.QItemSelection other, io.qt.core.QItemSelectionModel.SelectionFlags command){
merge_native_cref_QItemSelection_QFlags_QItemSelectionModel_SelectionFlag_(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(other), command.value());
}
@io.qt.QtUninvokable
private native void merge_native_cref_QItemSelection_QFlags_QItemSelectionModel_SelectionFlag_(long __this__nativeId, long other, int command);
/**
* See QItemSelection::select(QModelIndex,QModelIndex)
*/
@io.qt.QtUninvokable
public final void select(io.qt.core.QModelIndex topLeft, io.qt.core.QModelIndex bottomRight){
select_native_cref_QModelIndex_cref_QModelIndex(QtJambi_LibraryUtilities.internal.nativeId(this), topLeft, bottomRight);
}
@io.qt.QtUninvokable
private native void select_native_cref_QModelIndex_cref_QModelIndex(long __this__nativeId, io.qt.core.QModelIndex topLeft, io.qt.core.QModelIndex bottomRight);
/**
* See QItemSelection::split(QItemSelectionRange,QItemSelectionRange,QItemSelection*)
*/
public static io.qt.core.QItemSelection split(io.qt.core.QItemSelectionRange range, io.qt.core.QItemSelectionRange other){
return split_native_cref_QItemSelectionRange_cref_QItemSelectionRange_QItemSelection_ptr(QtJambi_LibraryUtilities.internal.checkedNativeId(range), QtJambi_LibraryUtilities.internal.checkedNativeId(other));
}
private native static io.qt.core.QItemSelection split_native_cref_QItemSelectionRange_cref_QItemSelectionRange_QItemSelection_ptr(long range, long other);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QItemSelection(QPrivateConstructor p) { super(p); }
@io.qt.QtUninvokable
@Override
public int hashCode() {
return hashCode_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native static int hashCode_native(long __this_nativeId);
public QItemSelection(java.util.Collection other) {
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QItemSelection instance, java.util.Collection other);
public QItemSelection() {
super((QPrivateConstructor)null);
initialize_native(this, null);
}
@Override
public QItemSelection clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QItemSelection clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy