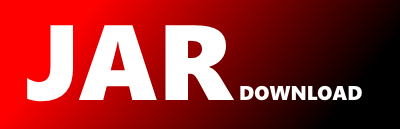
io.qt.core.QLockFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Locking between processes using a file
* Java wrapper for Qt class QLockFile
*/
public class QLockFile extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QLockFile::LockError
*/
public enum LockError implements io.qt.QtEnumerator {
NoError(0),
LockFailedError(1),
PermissionError(2),
UnknownError(3);
private LockError(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static LockError resolve(int value) {
switch (value) {
case 0: return NoError;
case 1: return LockFailedError;
case 2: return PermissionError;
case 3: return UnknownError;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QLockFile::QLockFile(QString)
*/
public QLockFile(java.lang.String fileName){
super((QPrivateConstructor)null);
initialize_native(this, fileName);
}
private native static void initialize_native(QLockFile instance, java.lang.String fileName);
/**
*
*/
@io.qt.QtUninvokable
public final io.qt.core.QLockFile.LockError error(){
return io.qt.core.QLockFile.LockError.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QLockFile::fileName()const
*/
@io.qt.QtUninvokable
public final java.lang.String fileName(){
return fileName_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String fileName_native_constfct(long __this__nativeId);
/**
* See QLockFile::getLockInfo(qint64*,QString*,QString*)const
*/
@io.qt.QtUninvokable
public final io.qt.core.QLockFile.LockInfo getLockInfo(){
return getLockInfo_native_long_long_ptr_QString_ptr_QString_ptr_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QLockFile.LockInfo getLockInfo_native_long_long_ptr_QString_ptr_QString_ptr_constfct(long __this__nativeId);
/**
* See QLockFile::isLocked()const
*/
@io.qt.QtUninvokable
public final boolean isLocked(){
return isLocked_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean isLocked_native_constfct(long __this__nativeId);
/**
*
*/
@io.qt.QtUninvokable
public final boolean lock(){
return lock_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean lock_native(long __this__nativeId);
/**
* See QLockFile::removeStaleLockFile()
*/
@io.qt.QtUninvokable
public final boolean removeStaleLockFile(){
return removeStaleLockFile_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean removeStaleLockFile_native(long __this__nativeId);
/**
* See QLockFile::setStaleLockTime(int)
*/
@io.qt.QtUninvokable
public final void setStaleLockTime(int arg__1){
setStaleLockTime_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setStaleLockTime_native_int(long __this__nativeId, int arg__1);
/**
* See QLockFile::staleLockTime()const
*/
@io.qt.QtUninvokable
public final int staleLockTime(){
return staleLockTime_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int staleLockTime_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #tryLock(int)}
* with timeout = 0
.
*/
@io.qt.QtUninvokable
public final boolean tryLock() {
return tryLock((int)0);
}
/**
*
*/
@io.qt.QtUninvokable
public final boolean tryLock(int timeout){
return tryLock_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), timeout);
}
@io.qt.QtUninvokable
private native boolean tryLock_native_int(long __this__nativeId, int timeout);
/**
*
*/
@io.qt.QtUninvokable
public final void unlock(){
unlock_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void unlock_native(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QLockFile(QPrivateConstructor p) { super(p); }
/**
* Result class for {@link #getLockInfo()}
*/
public static class LockInfo {
private LockInfo(long pid, String hostname, String appname) {
this.pid = pid;
this.hostname = hostname;
this.appname = appname;
}
public final long pid;
public final String hostname;
public final String appname;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy