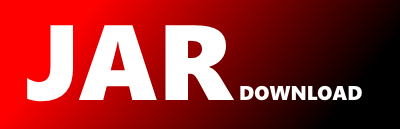
io.qt.core.QMessageAuthenticationCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Way to generate hash-based message authentication codes
* Java wrapper for Qt class QMessageAuthenticationCode
*/
public class QMessageAuthenticationCode extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Overloaded constructor for {@link #QMessageAuthenticationCode(io.qt.core.QCryptographicHash.Algorithm, io.qt.core.QByteArray)}
* with key = new io.qt.core.QByteArray()
.
*/
public QMessageAuthenticationCode(io.qt.core.QCryptographicHash.Algorithm method) {
this(method, new io.qt.core.QByteArray());
}
/**
* See QMessageAuthenticationCode::QMessageAuthenticationCode(QCryptographicHash::Algorithm,QByteArray)
*/
public QMessageAuthenticationCode(io.qt.core.QCryptographicHash.Algorithm method, io.qt.core.QByteArray key){
super((QPrivateConstructor)null);
initialize_native(this, method, key);
}
private native static void initialize_native(QMessageAuthenticationCode instance, io.qt.core.QCryptographicHash.Algorithm method, io.qt.core.QByteArray key);
/**
* See QMessageAuthenticationCode::addData(QIODevice*)
*/
@io.qt.QtUninvokable
public final boolean addData(io.qt.core.QIODevice device){
return addData_native_QIODevice_ptr(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(device));
}
@io.qt.QtUninvokable
private native boolean addData_native_QIODevice_ptr(long __this__nativeId, long device);
/**
* See QMessageAuthenticationCode::addData(QByteArray)
*/
@io.qt.QtUninvokable
public final void addData(io.qt.core.QByteArray data){
addData_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(data));
}
@io.qt.QtUninvokable
private native void addData_native_cref_QByteArray(long __this__nativeId, long data);
@io.qt.QtUninvokable
public final void addData(java.nio.ByteBuffer data){
addData_native_const_char_ptr_qsizetype(QtJambi_LibraryUtilities.internal.nativeId(this), data);
}
@io.qt.QtUninvokable
private native void addData_native_const_char_ptr_qsizetype(long __this__nativeId, java.nio.ByteBuffer data);
/**
* See QMessageAuthenticationCode::reset()
*/
@io.qt.QtUninvokable
public final void reset(){
reset_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native void reset_native(long __this__nativeId);
/**
* See QMessageAuthenticationCode::result()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QByteArray result(){
return result_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native io.qt.core.QByteArray result_native_constfct(long __this__nativeId);
/**
* See QMessageAuthenticationCode::setKey(QByteArray)
*/
@io.qt.QtUninvokable
public final void setKey(io.qt.core.QByteArray key){
setKey_native_cref_QByteArray(QtJambi_LibraryUtilities.internal.nativeId(this), QtJambi_LibraryUtilities.internal.checkedNativeId(key));
}
@io.qt.QtUninvokable
private native void setKey_native_cref_QByteArray(long __this__nativeId, long key);
/**
* See QMessageAuthenticationCode::hash(QByteArray,QByteArray,QCryptographicHash::Algorithm)
*/
public static io.qt.core.QByteArray hash(io.qt.core.QByteArray message, io.qt.core.QByteArray key, io.qt.core.QCryptographicHash.Algorithm method){
return hash_native_cref_QByteArray_cref_QByteArray_QCryptographicHash_Algorithm(QtJambi_LibraryUtilities.internal.checkedNativeId(message), QtJambi_LibraryUtilities.internal.checkedNativeId(key), method.value());
}
private native static io.qt.core.QByteArray hash_native_cref_QByteArray_cref_QByteArray_QCryptographicHash_Algorithm(long message, long key, int method);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QMessageAuthenticationCode(QPrivateConstructor p) { super(p); }
@io.qt.QtUninvokable
public final void addData(byte[] array){
addData(java.nio.ByteBuffer.wrap(array));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy