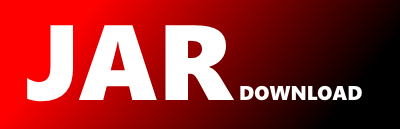
io.qt.core.QPropertyBindingError Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.core;
/**
* Java wrapper for Qt class QPropertyBindingError
*/
public class QPropertyBindingError extends io.qt.QtObject
implements java.lang.Cloneable
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QPropertyBindingError::Type
*/
public enum Type implements io.qt.QtEnumerator {
NoError(0),
BindingLoop(1),
EvaluationError(2),
UnknownError(3);
private Type(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static Type resolve(int value) {
switch (value) {
case 0: return NoError;
case 1: return BindingLoop;
case 2: return EvaluationError;
case 3: return UnknownError;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* See QPropertyBindingError::QPropertyBindingError()
*/
public QPropertyBindingError(){
super((QPrivateConstructor)null);
initialize_native(this);
}
private native static void initialize_native(QPropertyBindingError instance);
/**
* Overloaded constructor for {@link #QPropertyBindingError(io.qt.core.QPropertyBindingError.Type, java.lang.String)}
* with description = ""
.
*/
public QPropertyBindingError(io.qt.core.QPropertyBindingError.Type type) {
this(type, "");
}
/**
* See QPropertyBindingError::QPropertyBindingError(QPropertyBindingError::Type,QString)
*/
public QPropertyBindingError(io.qt.core.QPropertyBindingError.Type type, java.lang.String description){
super((QPrivateConstructor)null);
initialize_native(this, type, description);
}
private native static void initialize_native(QPropertyBindingError instance, io.qt.core.QPropertyBindingError.Type type, java.lang.String description);
/**
* See QPropertyBindingError::QPropertyBindingError(QPropertyBindingError)
*/
public QPropertyBindingError(io.qt.core.QPropertyBindingError other){
super((QPrivateConstructor)null);
initialize_native(this, other);
}
private native static void initialize_native(QPropertyBindingError instance, io.qt.core.QPropertyBindingError other);
/**
* See QPropertyBindingError::description()const
*/
@io.qt.QtUninvokable
public final java.lang.String description(){
return description_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String description_native_constfct(long __this__nativeId);
@io.qt.QtUninvokable
public final boolean hasError(){
return hasError_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean hasError_native_constfct(long __this__nativeId);
/**
* See QPropertyBindingError::type()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QPropertyBindingError.Type type(){
return io.qt.core.QPropertyBindingError.Type.resolve(type_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int type_native_constfct(long __this__nativeId);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QPropertyBindingError(QPrivateConstructor p) { super(p); }
@Override
public QPropertyBindingError clone() {
return clone_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
private native QPropertyBindingError clone_native(long __this_nativeId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy