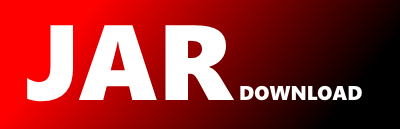
io.qt.core.QSystemSemaphore Maven / Gradle / Ivy
package io.qt.core;
/**
* General counting system semaphore
* Java wrapper for Qt class QSystemSemaphore
*/
public class QSystemSemaphore extends io.qt.QtObject
{
static {
QtJambi_LibraryUtilities.initialize();
}
/**
* Java wrapper for Qt enum QSystemSemaphore::AccessMode
*/
public enum AccessMode implements io.qt.QtEnumerator {
Open(0),
Create(1);
private AccessMode(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static AccessMode resolve(int value) {
switch (value) {
case 0: return Open;
case 1: return Create;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Java wrapper for Qt enum QSystemSemaphore::SystemSemaphoreError
*/
public enum SystemSemaphoreError implements io.qt.QtEnumerator {
NoError(0),
PermissionDenied(1),
KeyError(2),
AlreadyExists(3),
NotFound(4),
OutOfResources(5),
UnknownError(6);
private SystemSemaphoreError(int value) {
this.value = value;
}
/**
* {@inheritDoc}
*/
public int value() {
return value;
}
/**
* Returns the corresponding enum entry for the given value.
* @param value
* @return enum entry
*/
public static SystemSemaphoreError resolve(int value) {
switch (value) {
case 0: return NoError;
case 1: return PermissionDenied;
case 2: return KeyError;
case 3: return AlreadyExists;
case 4: return NotFound;
case 5: return OutOfResources;
case 6: return UnknownError;
default: throw new io.qt.QNoSuchEnumValueException(value);
}
}
private final int value;
}
/**
* Overloaded constructor for {@link #QSystemSemaphore(java.lang.String, int, io.qt.core.QSystemSemaphore.AccessMode)}
* with mode = io.qt.core.QSystemSemaphore.AccessMode.Open
.
*/
public QSystemSemaphore(java.lang.String key, int initialValue) {
this(key, initialValue, io.qt.core.QSystemSemaphore.AccessMode.Open);
}
/**
* Overloaded constructor for {@link #QSystemSemaphore(java.lang.String, int, io.qt.core.QSystemSemaphore.AccessMode)}
* with:
* initialValue = 0
* mode = io.qt.core.QSystemSemaphore.AccessMode.Open
*
*/
public QSystemSemaphore(java.lang.String key) {
this(key, (int)0, io.qt.core.QSystemSemaphore.AccessMode.Open);
}
/**
* See QSystemSemaphore::QSystemSemaphore(QString,int,QSystemSemaphore::AccessMode)
*/
public QSystemSemaphore(java.lang.String key, int initialValue, io.qt.core.QSystemSemaphore.AccessMode mode){
super((QPrivateConstructor)null);
initialize_native(this, key, initialValue, mode);
}
private native static void initialize_native(QSystemSemaphore instance, java.lang.String key, int initialValue, io.qt.core.QSystemSemaphore.AccessMode mode);
/**
* See QSystemSemaphore::acquire()
*/
@io.qt.QtUninvokable
public final boolean acquire(){
return acquire_native(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native boolean acquire_native(long __this__nativeId);
/**
* See QSystemSemaphore::error()const
*/
@io.qt.QtUninvokable
public final io.qt.core.QSystemSemaphore.SystemSemaphoreError error(){
return io.qt.core.QSystemSemaphore.SystemSemaphoreError.resolve(error_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this)));
}
@io.qt.QtUninvokable
private native int error_native_constfct(long __this__nativeId);
/**
* See QSystemSemaphore::errorString()const
*/
@io.qt.QtUninvokable
public final java.lang.String errorString(){
return errorString_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String errorString_native_constfct(long __this__nativeId);
/**
* See QSystemSemaphore::key()const
*/
@io.qt.QtUninvokable
public final java.lang.String key(){
return key_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native java.lang.String key_native_constfct(long __this__nativeId);
/**
* Overloaded function for {@link #release(int)}
* with n = 1
.
*/
@io.qt.QtUninvokable
public final boolean release() {
return release((int)1);
}
/**
* See QSystemSemaphore::release(int)
*/
@io.qt.QtUninvokable
public final boolean release(int n){
return release_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), n);
}
@io.qt.QtUninvokable
private native boolean release_native_int(long __this__nativeId, int n);
/**
* Overloaded function for {@link #setKey(java.lang.String, int, io.qt.core.QSystemSemaphore.AccessMode)}
* with mode = io.qt.core.QSystemSemaphore.AccessMode.Open
.
*/
@io.qt.QtUninvokable
public final void setKey(java.lang.String key, int initialValue) {
setKey(key, initialValue, io.qt.core.QSystemSemaphore.AccessMode.Open);
}
/**
* Overloaded function for {@link #setKey(java.lang.String, int, io.qt.core.QSystemSemaphore.AccessMode)}
* with:
* initialValue = 0
* mode = io.qt.core.QSystemSemaphore.AccessMode.Open
*
*/
@io.qt.QtUninvokable
public final void setKey(java.lang.String key) {
setKey(key, (int)0, io.qt.core.QSystemSemaphore.AccessMode.Open);
}
/**
* See QSystemSemaphore::setKey(QString,int,QSystemSemaphore::AccessMode)
*/
@io.qt.QtUninvokable
public final void setKey(java.lang.String key, int initialValue, io.qt.core.QSystemSemaphore.AccessMode mode){
setKey_native_cref_QString_int_QSystemSemaphore_AccessMode(QtJambi_LibraryUtilities.internal.nativeId(this), key, initialValue, mode.value());
}
@io.qt.QtUninvokable
private native void setKey_native_cref_QString_int_QSystemSemaphore_AccessMode(long __this__nativeId, java.lang.String key, int initialValue, int mode);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QSystemSemaphore(QPrivateConstructor p) { super(p); }
}