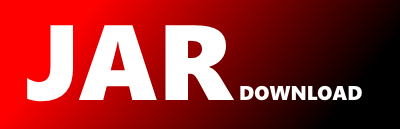
io.qt.gui.QIntValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtjambi Show documentation
Show all versions of qtjambi Show documentation
QtJambi base module containing QtCore, QtGui and QtWidgets.
package io.qt.gui;
/**
* Validator that ensures a string contains a valid integer within a specified range
* Java wrapper for Qt class QIntValidator
*/
public class QIntValidator extends io.qt.gui.QValidator
{
/**
* This variable stores the meta-object for the class.
*/
public static final io.qt.core.QMetaObject staticMetaObject = io.qt.core.QMetaObject.forType(QIntValidator.class);
/**
* See QIntValidator::bottomChanged(int)
*/
@io.qt.QtPropertyNotify(name="bottom")
public final Signal1<@io.qt.QtPrimitiveType Integer> bottomChanged = new Signal1<>();
/**
* See QIntValidator::topChanged(int)
*/
@io.qt.QtPropertyNotify(name="top")
public final Signal1<@io.qt.QtPrimitiveType Integer> topChanged = new Signal1<>();
/**
* Overloaded constructor for {@link #QIntValidator(io.qt.core.QObject)}
* with parent = null
.
*/
public QIntValidator() {
this((io.qt.core.QObject)null);
}
/**
* See QIntValidator::QIntValidator(QObject*)
*/
public QIntValidator(io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, parent);
}
private native static void initialize_native(QIntValidator instance, io.qt.core.QObject parent);
/**
* Overloaded constructor for {@link #QIntValidator(int, int, io.qt.core.QObject)}
* with parent = null
.
*/
public QIntValidator(int bottom, int top) {
this(bottom, top, (io.qt.core.QObject)null);
}
/**
* See QIntValidator::QIntValidator(int,int,QObject*)
*/
public QIntValidator(int bottom, int top, io.qt.core.QObject parent){
super((QPrivateConstructor)null);
initialize_native(this, bottom, top, parent);
}
private native static void initialize_native(QIntValidator instance, int bottom, int top, io.qt.core.QObject parent);
/**
* See QIntValidator::bottom()const
*/
@io.qt.QtPropertyReader(name="bottom")
@io.qt.QtUninvokable
public final int bottom(){
return bottom_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int bottom_native_constfct(long __this__nativeId);
/**
* See QIntValidator::setBottom(int)
*/
@io.qt.QtPropertyWriter(name="bottom")
@io.qt.QtUninvokable
public final void setBottom(int arg__1){
setBottom_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setBottom_native_int(long __this__nativeId, int arg__1);
/**
* See QIntValidator::setRange(int,int)
*/
@io.qt.QtUninvokable
public final void setRange(int bottom, int top){
setRange_native_int_int(QtJambi_LibraryUtilities.internal.nativeId(this), bottom, top);
}
@io.qt.QtUninvokable
private native void setRange_native_int_int(long __this__nativeId, int bottom, int top);
/**
* See QIntValidator::setTop(int)
*/
@io.qt.QtPropertyWriter(name="top")
@io.qt.QtUninvokable
public final void setTop(int arg__1){
setTop_native_int(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1);
}
@io.qt.QtUninvokable
private native void setTop_native_int(long __this__nativeId, int arg__1);
/**
*
*/
@io.qt.QtPropertyReader(name="top")
@io.qt.QtUninvokable
public final int top(){
return top_native_constfct(QtJambi_LibraryUtilities.internal.nativeId(this));
}
@io.qt.QtUninvokable
private native int top_native_constfct(long __this__nativeId);
/**
* See QValidator::fixup(QString&)const
*/
@io.qt.QtUninvokable
public java.lang.String fixup(java.lang.String input){
return fixup_native_ref_QString_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), input);
}
@io.qt.QtUninvokable
private native java.lang.String fixup_native_ref_QString_constfct(long __this__nativeId, java.lang.String input);
/**
* See QValidator::validate(QString&,int&)const
*/
@io.qt.QtUninvokable
public io.qt.gui.QValidator.State validate(io.qt.gui.QValidator.QValidationData arg__1){
return io.qt.gui.QValidator.State.resolve(validate_native_ref_QString_ref_int_constfct(QtJambi_LibraryUtilities.internal.nativeId(this), arg__1));
}
@io.qt.QtUninvokable
private native int validate_native_ref_QString_ref_int_constfct(long __this__nativeId, io.qt.gui.QValidator.QValidationData arg__1);
/**
* Constructor for internal use only.
* @param p expected to be null
.
*/
@io.qt.NativeAccess
protected QIntValidator(QPrivateConstructor p) { super(p); }
/**
* Constructor for internal use only.
* It is not allowed to call the declarative constructor from inside Java.
*/
@io.qt.NativeAccess
protected QIntValidator(QDeclarativeConstructor constructor) {
super((QPrivateConstructor)null);
initialize_native(this, constructor);
}
@io.qt.QtUninvokable
private static native void initialize_native(QIntValidator instance, QDeclarativeConstructor constructor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy